Steps to Retrieve Bundle Option Type by Option ID:
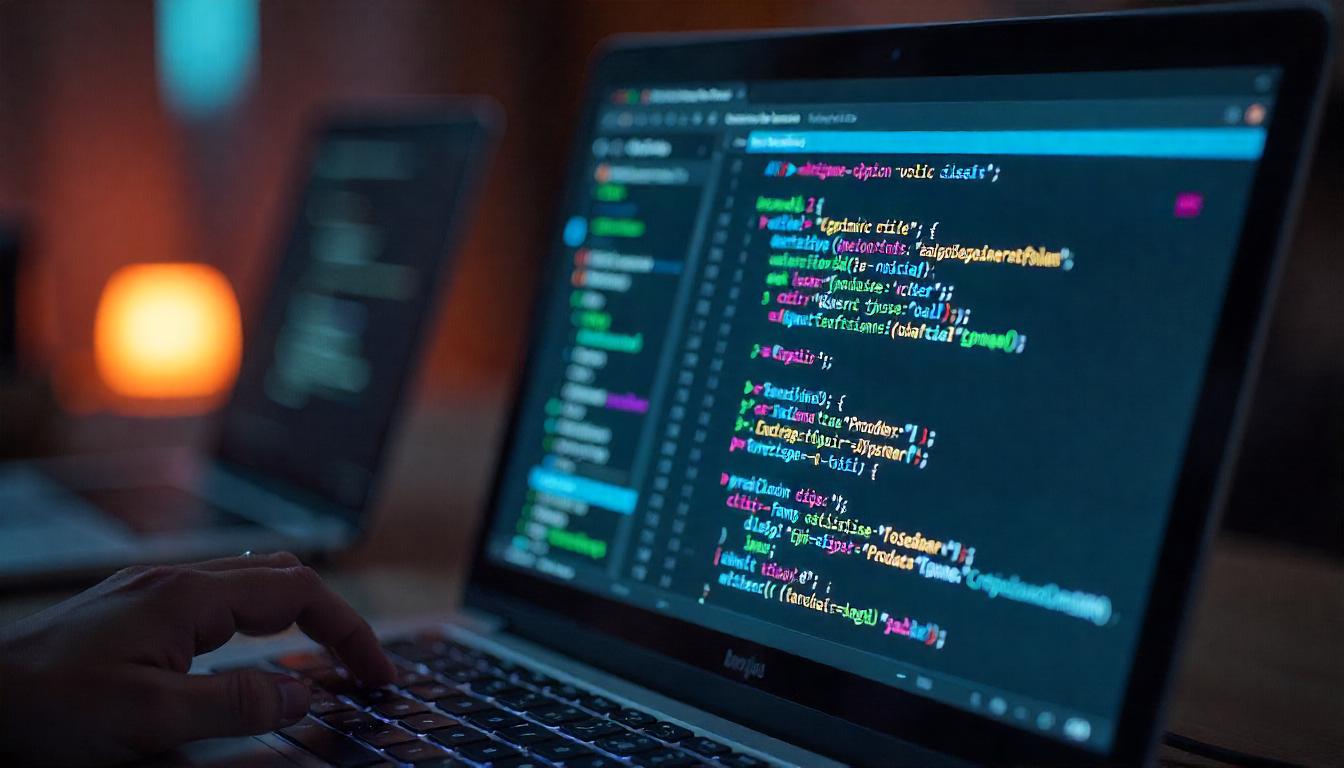
Steps to Retrieve Bundle Option Type by Option ID:
To retrieve the bundle option type by option ID in Magento 2, you need to fetch data from the bundle product options. Magento provides an API to get option details using the ProductOptionRepositoryInterface.
Table Of Content
Steps to Retrieve Bundle Option Type by Option ID:
To programmatically determine the option type of a bundle product in Magento 2, you can utilize the ProductOptionRepositoryInterface. This interface allows you to fetch details about bundle options using the product's SKU and the specific option ID.
- Inject the ProductOptionRepositoryInterface: Begin by injecting the
ProductOptionRepositoryInterface
into your class.
use Magento\Bundle\Api\ProductOptionRepositoryInterface;
use Magento\Framework\Exception\NoSuchEntityException;
class BundleOptionType
{
private $productOptionRepository;
public function __construct(ProductOptionRepositoryInterface $productOptionRepository)
{
$this->productOptionRepository = $productOptionRepository;
}
/**
* Retrieve product option by ID.
*
* @param int $optionId
* @return \Magento\Bundle\Api\Data\OptionInterface|null
* @throws NoSuchEntityException
*/
public function getOptionById(int $optionId)
{
try {
return $this->productOptionRepository->get($optionId);
} catch (NoSuchEntityException $e) {
return null;
}
}
}
- Implement the Method to Get Option Type: Create a method that accepts the product SKU and option ID, then retrieves the corresponding option type.
public function getOptionTypeById(string $sku, int $optionId): ?string
{
try {
$option = $this->productOptionRepository->get($sku, $optionId);
return $option->getType();
} catch (\Magento\Framework\Exception\NoSuchEntityException $e) {
// Option not found, handle accordingly
} catch (\Magento\Framework\Exception\LocalizedException $e) {
// Handle specific Magento exceptions
} catch (\Exception $e) {
// Log other exceptions if needed
}
return null;
}
- Usage Example: You can call the getOptionTypeById method by passing the product SKU and option ID.
$sku = 'your_bundle_product_sku';
$optionId = 1; // Replace with your option ID
$optionType = $this->getOptionTypeById($sku, $optionId);
if ($optionType) {
echo "The option type is: " . $optionType;
} else {
echo "Option type not found.";
}
Available Option Types:
Magento 2 supports the following option types for bundle products:
select
radio
checkbox
multi
These correspond to dropdowns, radio buttons, checkboxes, and multi-selects, respectively.
Ensure that the SKU and option ID you provide are valid and correspond to an existing bundle product and its option. Handling exceptions will help in identifying issues during the retrieval process.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve the Option Type by Option ID in Magento 2?
To retrieve the option type for a bundle product in Magento 2, you can use the ProductOptionRepositoryInterface
. This interface allows fetching details about a specific bundle option by its ID.
What Is the Purpose of ProductOptionRepositoryInterface
?
The ProductOptionRepositoryInterface
manages bundle product options. It provides methods to retrieve and manipulate bundle option data programmatically.
How Do I Retrieve the Option Type Using the Option ID?
You can retrieve the option type by using the following PHP code:
$sku = 'your_bundle_product_sku';
$optionId = 1; // Replace with your option ID
$optionType = $this->getOptionTypeById($sku, $optionId);
if ($optionType) {
echo "The option type is: " . $optionType;
} else {
echo "Option type not found.";
}
What Option Types Are Available for Bundle Products?
Magento 2 supports the following option types for bundle products:
select
- Dropdown selectionradio
- Radio buttoncheckbox
- Checkboxmulti
- Multi-select
What Happens If No Option Type Is Found for the Given ID?
If no option type is found for the specified option ID, the method will return null. To prevent errors, always check if the result is valid before using it in your code.
Can I Optimize the Code for Retrieving Bundle Option Types?
Yes, you should use dependency injection instead of the object manager for better performance. Also, ensure your database is indexed properly for faster query execution.
What Is the Role of getOptionTypeById()
Method?
The getOptionTypeById()
method retrieves the option type by fetching bundle option data from Magento's repository. It returns the type as a string.