Ultimate Magento 2.4.7 Tutorial: How to Create Directories & Write Text Files Step-by-Step
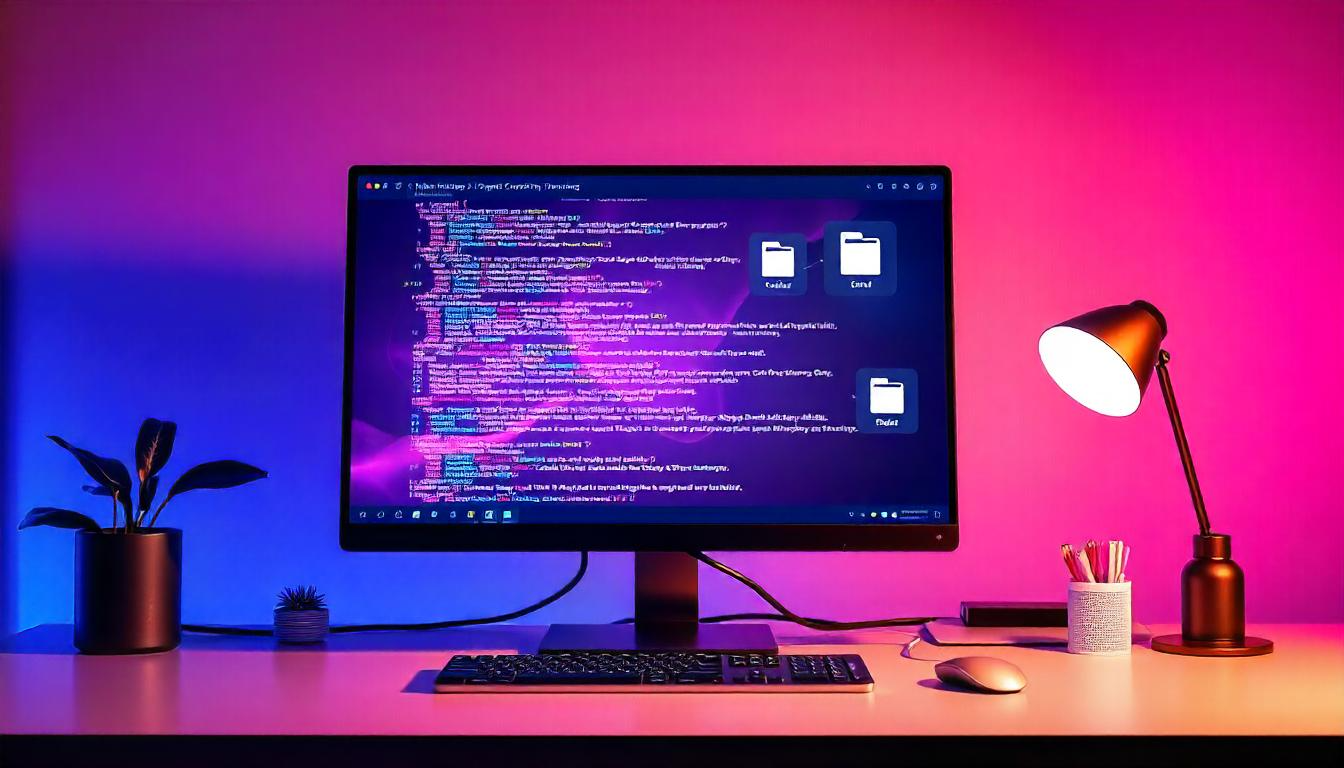
Ultimate Magento 2.4.7 Tutorial: How to Create Directories & Write Text Files Step-by-Step
Writing text files and creating directories programmatically in Magento 2 is essential for tasks such as logging, data synchronization, and integration with third-party tools. In Magento 2.4.7, the process leverages the robust Magento\Framework\Filesystem
component, ensuring secure and efficient file operations within the Magento file system structure.
Proper file handling helps maintain data integrity, improves debugging, and enhances automation. Magento 2 provides a structured approach using dependency injection, making file operations more secure and compliant with best practices.
Table Of Content
Magento 2.4.7: Creating Directories and Writing Text Files Programmatically Intro
In many scenarios, you might need to create a directory and write a text file dynamically—for instance, logging the last synchronized order ID with an external system or saving temporary data for debugging. Magento 2.4.7 provides standard, secure methods for handling file system operations. This guide outlines how to create a custom directory (e.g., var/custom) and write a text file (e.g., demo.txt) using Magento’s built-in filesystem interfaces.
Magento 2.4.7 provides a secure and structured way to handle file operations using Magento\Framework\Filesystem. Whether you need to log synchronization details, store temporary debugging information, or generate reports, Magento’s built-in filesystem ensures compatibility with multi-server environments and cloud-based hosting solutions.
Why Use Magento’s Filesystem for File Operations?
Magento 2.4.7 provides a robust filesystem API (Magento\Framework\Filesystem
) that enhances file handling capabilities while ensuring security, scalability, and efficiency. Unlike using direct PHP functions like mkdir()
or file_put_contents()
, Magento’s filesystem is optimized for multi-server environments, cloud storage, and large-scale eCommerce operations.
Key Advantages of Using Magento’s Filesystem API:
1. Security & Permission Control
Magento enforces strict file system permissions to protect against unauthorized access. Using Magento\Framework\Filesystem
ensures that files and directories follow Magento’s security model, preventing external threats.
- Restricts unauthorized file modifications.
- Prevents accidental deletion of critical files.
- Ensures compliance with Magento’s read/write rules.
2. Scalability Across Multiple Environments
Magento’s filesystem API allows seamless file management across various hosting environments, including:
- Cloud servers (AWS, Google Cloud, Azure).
- Multi-node setups with shared file storage.
- Distributed file systems (e.g., NFS, GlusterFS).
Using direct PHP file operations may not work correctly in distributed environments, but Magento’s API abstracts storage complexities, making it scalable for enterprise setups.
3. Performance Optimization
Magento’s filesystem is designed for efficiency, reducing file access overhead through:
- Caching & Indexing: Faster read/write operations.
- Lazy Loading: Loads files only when necessary.
- Optimized Storage Management: Avoids excessive I/O operations.
This improves Magento’s overall performance, especially for high-traffic stores that rely on frequent file operations.
4. Flexible Storage Locations
Magento organizes file storage systematically, ensuring flexibility while maintaining structure. Using the filesystem API, developers can store files securely in different locations:
Storage Location | Purpose |
---|---|
var/log/ | Stores custom logs and debugging information. |
var/report/ | System-generated error logs and crash reports. |
var/custom/ | Custom files (recommended for temporary storage). |
media/ | Product images, CMS assets, and downloadable files. |
pub/media/ | Exposed media files accessible from the frontend. |
app/etc/ | Configuration files and XML settings. |
Important: Avoid storing sensitive data in pub/media/, as it is publicly accessible. For secure data storage, use var/ or app/etc/.
Pro Tip:
Always use Dependency Injection (DI) to access Magento\Framework\Filesystem
. This ensures better code maintainability and prevents direct object instantiation, which is discouraged in Magento development.
Where Should You Store Custom Files in Magento 2?
Magento 2 organizes files into predefined directories to ensure security, performance, and maintainability. Choosing the right storage location is crucial for keeping your data organized and protected.
Commonly Used Directories in Magento 2
Directory | Purpose |
---|---|
var/log/ |
Stores custom logs and debugging information. |
var/report/ |
Contains system-generated reports and error logs. |
var/custom/ |
Recommended for user-defined temporary files and custom storage needs. |
media/ |
Holds publicly accessible images, CMS assets, and downloadable files. |
pub/media/ |
Stores media files accessible from the frontend (e.g., product images, category banners). |
app/etc/ |
Contains Magento configuration files like env.php and config.php . |
generated/ |
Holds generated classes, proxies, and metadata files (should not be manually modified). |
pub/static/ |
Stores static assets such as CSS, JavaScript, and theme-related files. |
Best Practices for Storing Custom Files
- Use
var/custom/
for Temporary Data: If you need to store temporary or custom files, this directory is recommended as it is not publicly accessible. - Avoid Storing Sensitive Data in
pub/media/
: Sincepub/media/
is accessible from the frontend, sensitive files should not be stored here. - Log Custom Errors in
var/log/
: If you're implementing custom logging, store logs in this directory to maintain consistency with Magento’s logging system. - Protect Configuration Files: The
app/etc/
directory contains critical configuration files and should not be publicly exposed or modified manually unless necessary. - Use Magento’s Filesystem API: Instead of manually creating directories and files with PHP functions, leverage
Magento\Framework\Filesystem
for better security and compatibility.
Best Practices for Creating Files and Directories in Magento 2.4.7
Before implementing file operations in Magento 2.4.7, follow these essential best practices to ensure security, efficiency, and maintainability:
- Use Dependency Injection (DI): Always inject Magento\Framework\Filesystem via the constructor instead of manually instantiating objects. This improves code maintainability and aligns with Magento’s architecture.
- Error Handling & Logging: Wrap file operations in try-catch blocks to handle exceptions gracefully. Use Magento’s logging system (Psr\Log\LoggerInterface) to capture errors instead of relying on echo or var_dump().
- Ensure Correct Permissions: Magento’s filesystem must have the proper write permissions. Use:
bin/magento setup:perms:apply
- Follow Naming Conventions: Use clear, descriptive filenames relevant to their function.
Examples:sync_orders.log
(good)data.txt
(unclear)
- Use Secure Storage Locations: Store files in appropriate Magento directories to avoid security risks:
- Temporary files:
var/custom/
- Logs:
var/log/
- Reports:
var/report/
- Publicly accessible media:
pub/media/
(for images, not sensitive data)
- Temporary files:
- Enable Periodic Cleanup: Remove outdated logs and temporary files to prevent disk space issues. Consider using Magento’s built-in cron jobs for automated cleanup.
- Use Magento’s Filesystem API Instead of Core PHP Functions: Avoid
mkdir()
orfile_put_contents()
. Instead, leverage:$this->directory->writeFile($filePath, $content);
This ensures compatibility with different storage backends, such as cloud-based file systems.
Magento Filesystem Overview
Magento 2 provides a robust Filesystem API to manage file operations securely and efficiently. Instead of using standard PHP functions like file_put_contents()
or mkdir()
, Magento’s framework-based approach ensures compatibility, security, and scalability.
Key Components of Magento's Filesystem API
Component | Purpose |
---|---|
Magento\Framework\Filesystem |
Core class for handling file operations such as reading, writing, and deleting files. |
Magento\Framework\App\Filesystem\DirectoryList |
Manages predefined directory paths (e.g., var/ , media/ , pub/ ). Helps retrieve paths dynamically. |
Magento\Framework\Filesystem\Directory\WriteInterface |
Provides secure methods for writing, creating, and deleting files and directories. Ensures proper access permissions. |
Magento\Framework\Filesystem\Driver\File |
Handles low-level file system interactions like checking file existence and retrieving contents. |
Magento\Framework\Filesystem\Io\File |
Supports advanced file operations like copying, moving, renaming, and getting file metadata. |
Use Cases and Best Practices for File Operations in Magento 2.4.7
Use Cases
- Logging and Debugging: Store custom logs or temporary data for troubleshooting issues.
- Data Synchronization: Save details such as the last imported order ID for seamless integration with external systems.
- Custom Reports: Generate and store dynamic reports, logs, or analytics in structured text files.
- Temporary Data Storage: Maintain temporary session data or cache information without exposing it to the public.
- Third-Party Integrations: Exchange data files with external platforms for automation and data exchange.
Best Practices
- Use Dependency Injection (DI): Always inject
Magento\Framework\Filesystem
instead of using the Object Manager directly. - Keep File Operations Secure: Restrict file access to non-public directories like
var/
to prevent unauthorized access. - Implement Proper Error Handling: Use
try-catch
blocks to handle exceptions gracefully and avoid system crashes. - Follow Magento’s Directory Structure: Store files in appropriate locations like
var/custom/
for temporary data instead ofpub/media/
. - Use Proper Logging: Record any file operation errors using Magento’s built-in logging framework to track issues effectively.
- Ensure Correct Permissions: Maintain proper read/write permissions using Magento CLI (
bin/magento setup:perms:apply
). - Regular Cleanup: Periodically remove outdated or unnecessary files to optimize storage and maintain performance.
Pro Tip:
By leveraging Magento’s Filesystem API, developers ensure that file operations are consistent, secure, and aligned with Magento’s best practices
Implementation Guide
Step 1: Create a Custom Module (If Needed)
If you don't have an existing module for this functionality, create a new module (e.g., Vendor_Module
). Define the necessary module registration and configuration files.
Step 2: Implementing the Model Class
Create a model class to handle directory creation and file writing. Below is an example implementation:
<?php
namespace Vendor\Module\Model;
use Magento\Framework\Filesystem;
use Magento\Framework\App\Filesystem\DirectoryList;
use Magento\Framework\Exception\FileSystemException;
use Magento\Framework\Filesystem\Directory\WriteInterface;
class WriteTextFile
{
private $filesystem;
private $directoryList;
public function __construct(
Filesystem $filesystem,
DirectoryList $directoryList
) {
$this->filesystem = $filesystem;
$this->directoryList = $directoryList;
}
public function createDemoTextFile(): bool
{
try {
$varDirectory = $this->filesystem->getDirectoryWrite(DirectoryList::VAR_DIR);
$customFolder = 'custom/';
$fileName = 'demo.txt';
if (!$varDirectory->isExist($customFolder)) {
$varDirectory->create($customFolder);
}
$filePath = $customFolder . $fileName;
return $this->write($varDirectory, $filePath);
} catch (FileSystemException $e) {
return false;
}
}
public function write(WriteInterface $writeDirectory, string $filePath): bool
{
try {
$stream = $writeDirectory->openFile($filePath, 'w+');
$stream->lock();
$fileData = 'Last Imported Order ID: 10000001';
$stream->write($fileData);
$stream->unlock();
$stream->close();
return true;
} catch (FileSystemException $e) {
return false;
}
}
}
Step 3: Explanation of the Code
Filesystem Initialization:
The constructor injects Filesystem
and DirectoryList
to handle file paths and write operations.
Creating the Directory:
The createDemoTextFile()
method retrieves the var
directory. It checks if a custom/
folder exists and creates it if not.
Writing the Text File:
The write()
method opens (or creates) a file in write mode, locks it to prevent concurrent access, writes data, then unlocks and closes the file.
Additional Enhancements
Retrieving Dynamic Content
You might want to write dynamic content (e.g., the last order ID fetched from the database):
$fileData = 'Last Imported Order ID: ' . $latestOrderId;
Using Logging for Debugging
Integrate Magento's logger to capture any file system errors:
use Psr\Log\LoggerInterface;
//$this->logger->error('File write error', [$e->getMessage()]);
// Inject LoggerInterface in constructor and use:
Directory and File Permissions
Ensure the web server user has the appropriate permissions to write to the var
directory to avoid permission-related issues.
Configuration Settings Overview
To facilitate better understanding and use of configuration settings, here's a summary displayed in a table format:
Parameter | Description | Example Value |
---|---|---|
Directory | Defines the base directory where file operations occur. | var/custom/ |
File Name | Specifies the name of the text file to be created. | demo.txt |
File Data | Details the content to be written inside the text file. | Last Imported Order ID: 10000001 |
File Mode | Specifies the file opening mode, typically for writing. | w+ |
File Permissions | Defines the permissions for the created file (e.g., read/write). | 0644 |
Encoding | Specifies the character encoding for the file content. | UTF-8 |
Line Break | Defines the type of line break to use in the file. | \n |
Backup | Indicates whether a backup of the file should be created before writing. | true |
Overwrite | Determines if an existing file with the same name should be overwritten. | false |
Timestamp | Adds a timestamp at the beginning or end of the file. | true |
Common Troubleshooting Scenarios and Solutions
Here’s a summary of common issues related to file operations and their solutions:
Issue | Possible Cause | Solution |
---|---|---|
File not created or written | Insufficient directory permissions | Ensure that the var directory and its subdirectories have the correct write permissions for the web server user. |
Errors during file write | File system exceptions or path errors | Use try-catch blocks to handle exceptions, and log detailed error messages using Magento's LoggerInterface for easier debugging. |
Directory not found | Custom folder not created before file write | Before attempting to write, check if the directory exists using isExist() . If it doesn't, create the directory using Magento’s file system utilities. |
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Writing text files and creating directories programmatically in Magento 2 is a standard and secure method to handle file operations. By leveraging Magento’s Filesystem API, developers can dynamically create directories, write text files, and store essential data such as order IDs or logs. This guide demonstrates a best-practice approach for Magento 2.4.7, ensuring that your code remains scalable, secure, and aligned with modern development standards.
Key Takeaways:
- Use Dependency Injection: Always inject Filesystem and DirectoryList to manage file paths securely.
- Implement Robust Error Handling: Use try-catch blocks and log errors effectively.
- Customize Content Dynamically: Adapt file content based on your application's needs.
- Follow Best Practices: Ensure proper directory permissions and leverage Magento's built-in logging.
By adopting these methods, your Magento 2 store will efficiently handle dynamic file operations, making it easier to integrate with third-party systems and maintain robust logging and reporting capabilities.
FAQs
How do I write a text file in Magento 2?
In Magento 2, you can use the Magento\Framework\Filesystem
component to write text files by opening a file in write mode and writing the desired content.
How can I create a custom directory for storing files in Magento 2?
You can create a custom directory by using Filesystem
and checking if the directory exists using the isExist()
method. If it doesn’t exist, create it using create()
.
What file permissions are required for file operations in Magento 2?
Ensure that the var
directory and its subdirectories have appropriate write permissions for the web server user to avoid permission errors.
What is the recommended way to handle errors during file write operations in Magento 2?
Use try-catch
blocks to handle exceptions, and log any errors using Magento's LoggerInterface
for debugging and traceability.
How can I dynamically write data, like the last order ID, into a file?
You can retrieve the dynamic content, such as the last order ID, from the database and use it to write to the file. For example: $fileData = 'Last Imported Order ID: ' . $latestOrderId;
What is the purpose of the Magento\Framework\Filesystem\DirectoryList
class?
The DirectoryList
class is used to manage and retrieve directory paths within Magento 2, such as var
, media
, and app
.
How do I ensure the file is written securely in Magento 2?
Ensure that file operations are restricted to appropriate directories like var
, and always implement proper error handling and logging.
How can I prevent file write errors caused by missing directories?
Before writing to the file, check if the target directory exists using isExist()
. If the directory is missing, create it using create()
.
What should I do if I encounter filesystem exceptions while writing a file?
Use try-catch
blocks to catch filesystem exceptions, and log detailed error messages using Magento’s logging system for easy troubleshooting.
How do I open a file in write mode in Magento 2?
To open a file in write mode, you can use the openFile()
method provided by the WriteInterface
class and specify the mode as 'w+'
.
What is the purpose of file locking in Magento 2?
File locking ensures that the file is not concurrently accessed by multiple processes, which helps maintain data integrity. You can use the lock()
method to lock the file before writing.
How do I ensure a file is closed after writing?
Always use the close()
method to close the file after writing to release system resources and prevent memory leaks.
Can I customize the content being written to the file in Magento 2?
Yes, you can customize the content dynamically by fetching data from the database or other sources, and then formatting it before writing it to the file.