Retrieve Product Attribute Code by Attribute ID in Magento 2
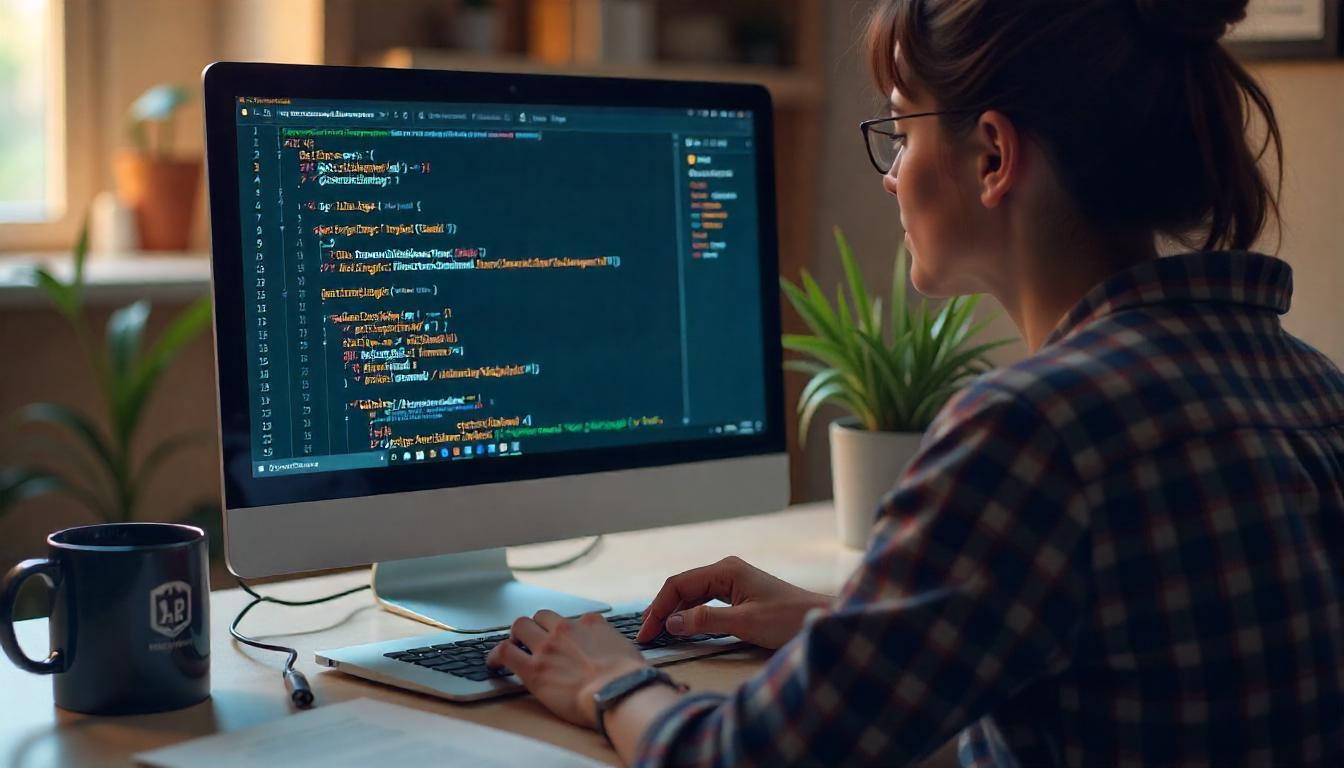
Retrieve Product Attribute Code by Attribute ID in Magento 2
To retrieve a product attribute code by its attribute ID in Magento 2, you can use the ProductAttributeRepositoryInterface class. This method is useful when you're working on custom development or features where you need to fetch the attribute code dynamically.
Table Of Content
Retrieve Product Attribute Code by Attribute ID in Magento 2
In Magento 2, you can obtain a product's attribute code using its attribute ID. Here's how:
Using Dependency Injection
Inject the ProductAttributeRepositoryInterface
into your class:
use Magento\Catalog\Api\ProductAttributeRepositoryInterface;
class YourClass
{
< private $attributeRepository;
< public function __construct(ProductAttributeRepositoryInterface $attributeRepository)
{
$this->attributeRepository = $attributeRepository;
}
public function getAttributeCodeById(int $id)
{
return $this->attributeRepository->get($id)->getAttributeCode();>
}
}
Call the method with the attribute ID:
$attributeCode = $this->getAttributeCodeById(93);
echo $attributeCode; // Outputs: color
Using Object Manager (Not Recommended)
While it's possible to use the Object Manager, it's not recommended due to best practices:
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$attribute = $objectManager->get(\Magento\Catalog\Api\ProductAttributeRepositoryInterface::class)->get(93);
echo $attribute->getAttributeCode(); // Outputs: color
For more details, refer to Rakesh Jesadiya's article on retrieving product attribute codes by attribute ID in Magento 2.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Product Attribute Code by Attribute ID in Magento 2?
To retrieve a product attribute code by its ID in Magento 2, you can use the ProductAttributeRepositoryInterface
service. This allows you to fetch the attribute code associated with a given attribute ID using dependency injection or the object manager.
What Is the Purpose of ProductAttributeRepositoryInterface
?
The ProductAttributeRepositoryInterface
is responsible for managing product attribute data. It provides methods to retrieve, save, and manage product attributes in Magento, including getting an attribute by its ID.
How Do I Retrieve the Attribute Code Using the Attribute ID?
You can retrieve the attribute code by using the following PHP code:
$attributeId = 93;
$attributeCode = $this->getAttributeCodeById($attributeId);
echo $attributeCode; // Outputs: color
What Happens If No Attribute Code Is Found for the ID?
If no attribute code is found for the given ID, the result will be null. It's important to handle this case to prevent errors in your application when working with missing attribute data.
Can I Optimize the Code for Retrieving Product Attribute Codes?
Yes, to improve performance, avoid using the object manager directly. Use dependency injection to fetch the attribute repository, and ensure that the database is properly indexed for better speed when retrieving attribute codes.
What Is the Role of getAttributeCodeById()
Method?
The getAttributeCodeById()
method is used to fetch the attribute code by providing the attribute ID. It uses Magento's attribute repository to retrieve the code and return it as a string.