Retrieve Cart Price Rule Data by ID in Magento 2
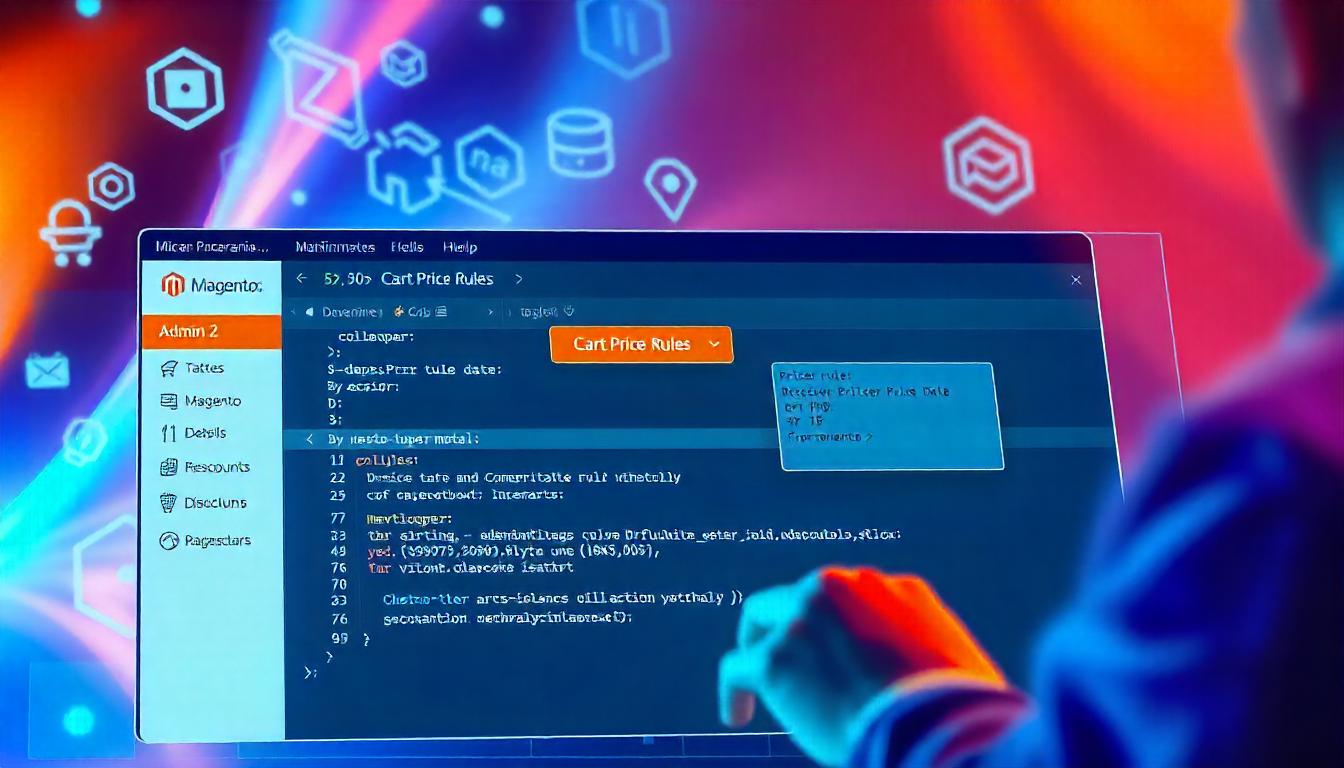
Retrieve Cart Price Rule Data by ID in Magento 2
To retrieve cart price rule data in Magento 2, you need the sales rule ID. Using the RuleRepositoryInterface, you can fetch rule details like name, conditions, discounts, and usage limits.
Table Of Content
Retrieve Cart Price Rule Data by ID in Magento 2
To access cart price rule details in Magento 2, you'll need the specific sales rule ID. Here's a straightforward method to fetch this data:
Code Implementation:
<?php
namespace YourNamespace\SalesRule\Model;
use Magento\SalesRule\Api\RuleRepositoryInterface;
use Psr\Log\LoggerInterface;
use Magento\SalesRule\Api\Data\RuleInterface;
class SalesRuleById
{
private LoggerInterface $logger;
private RuleRepositoryInterface $ruleRepository;
public function __construct(
LoggerInterface $logger,
RuleRepositoryInterface $ruleRepository
) {
$this->logger = $logger;
$this->ruleRepository = $ruleRepository;
}
public function getRuleData(int $ruleId): ?RuleInterface
{
try {
return $this->ruleRepository->getById($ruleId);
} catch (\Exception $e) {
$this->logger->error(__('Error fetching rule: %1', $e->getMessage()));
return null;
}
}
}
Usage:
$ruleId = 1;
$salesRuleData = $this->getRuleData($ruleId);
if ($salesRuleData) {
echo $salesRuleData->getName();
}
Explanation:
- Namespace Declaration: Replace
YourNamespace
with your actual namespace. - Dependencies: The class uses
RuleRepositoryInterface
to fetch rule data andLoggerInterface
for logging errors. - Constructor: Initializes the logger and rule repository.
- getRuleData Method: Attempts to retrieve the sales rule by ID. If successful, it returns the rule data; otherwise, it logs the error and returns null.
Additional Information
To retrieve specific properties of the sales rule, such as the description or conditions, you can use methods like getDescription()
or getConditionsSerialized()
on the $salesRuleData
object. Ensure that the rule ID you provide exists in your Magento 2 setup to avoid exceptions.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Cart Price Rule Data by ID in Magento 2?
You can fetch cart price rule details in Magento 2 using the sales rule ID. The RuleRepositoryInterface
allows you to retrieve rule data programmatically.
What PHP Code Can I Use to Get Cart Price Rule Data?
Use the following PHP code to fetch cart price rule details:
$ruleId = 1;
$salesRuleData = $this->getRuleData($ruleId);
if ($salesRuleData) {
echo $salesRuleData->getName();
}
Which Magento Interface Is Used to Retrieve Sales Rule Data?
The Magento\SalesRule\Api\RuleRepositoryInterface
is used to fetch cart price rule data by rule ID.
How Can I Handle Errors While Fetching Cart Price Rule Data?
Wrap the API call inside a try-catch block to handle exceptions:
try {
$salesRule = $this->ruleRepository->getById($ruleId);
} catch (Exception $e) {
$this->logger->error($e->getMessage());
}
Where Is Cart Price Rule Data Stored in Magento 2?
Cart price rule details are stored in the salesrule
table in the Magento database.
What Happens If the Given Rule ID Does Not Exist?
If the rule ID is invalid or does not exist, the getById()
method will throw an exception. Ensure the ID is correct before calling the method.