Understanding Bundle Product Option Types in Magento 2
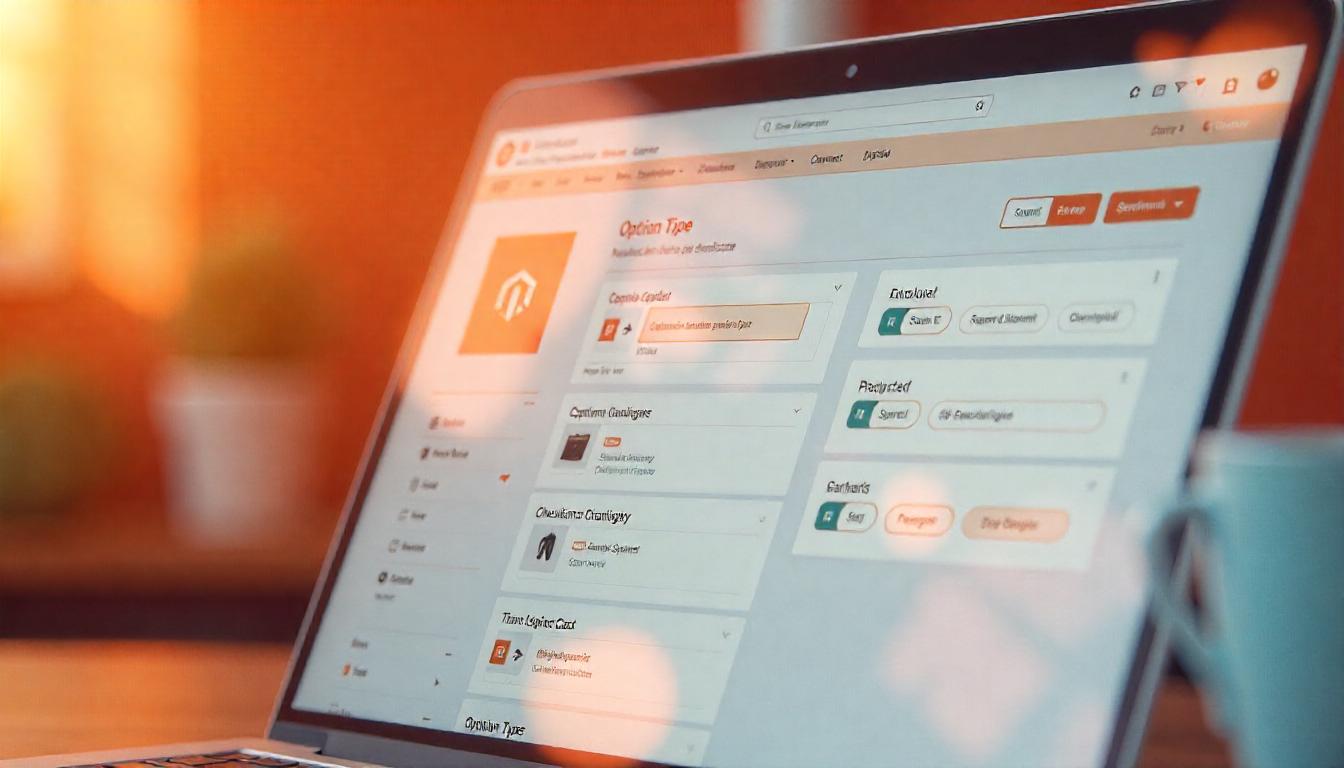
Understanding Bundle Product Option Types in Magento 2
Bundle products in Magento 2 offer flexible purchasing options by allowing customers to customize their selections. These products use different option types like dropdowns, radio buttons, checkboxes, and multi-selects.
Table Of Content
Understanding Bundle Product Option Types in Magento 2
In Magento 2, bundle products offer customizable options to enhance the shopping experience. These options include dropdowns, radio buttons, checkboxes, and multi-selects. To retrieve all available system option types for bundle products programmatically, you can utilize the native interface Magento\Bundle\Api\ProductOptionTypeListInterface
. This interface contains the getItems()
method, which provides a list of option types for bundle products.
Here's how you can implement this:
<?php
namespace YourNamespace\BundleOptions\Model;
use Magento\Bundle\Api\Data\OptionTypeInterface;
use Magento\Bundle\Api\ProductOptionTypeListInterface;
class BundleOptions
{
private ProductOptionTypeListInterface $productOptionType;
public function __construct(ProductOptionTypeListInterface $productOptionType)
{
$this->productOptionType = $productOptionType;
}
public function getOptionsType(): array
{
return $this->productOptionType->getItems();
}
}
- Fetch Bundle Options: Create a method to fetch bundle options using the getList method provided by
ProductOptionRepositoryInterface
.
public function getBundleOptionsBySku(string $sku): array
{
try {
$options = $this->productOptionRepository->getListByProductSku($sku);
return $options->getItems();
} catch (\Magento\Framework\Exception\NoSuchEntityException $e) {
$this->logger->warning("No bundle options found for SKU: {$sku}");
} catch (\Exception $e) {
$this->logger->error("Error fetching bundle options for SKU {$sku}: " . $e->getMessage());
}
return [];
}
To use this in a template or any PHP class:
$items = $this->getOptionsType();
foreach ($items as $item) {
echo $item->getLabel() . ' => ' . $item->getCode();
}
Output:
select
radio
checkbox
multi
This approach allows you to programmatically access all native system option types for bundle products in Magento 2.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Are the Available Option Types for Bundle Products in Magento 2?
Magento 2 bundle products support multiple option types, including dropdown
, radio buttons
, checkboxes
, and multi-select
. These options allow customers to customize their selections when purchasing a bundle product.
How Can I Retrieve All Bundle Product Option Types Programmatically?
You can use the Magento\Bundle\Api\ProductOptionTypeListInterface
to get a list of all option types available for bundle products. The getItems()
method returns all system-defined bundle product options.
What PHP Code Can I Use to Fetch Bundle Option Types?
Use the following PHP code to get all bundle option types:
$items = $this->getOptionsType();
foreach ($items as $item) {
echo $item->getLabel() . ' => ' . $item->getCode();
}
Where Are Bundle Product Options Stored in Magento 2?
Bundle product option details are stored in the catalog_product_bundle_option
table in the Magento database.
How Do I Ensure My SKU Belongs to a Bundle Product?
Before fetching options, verify that the product SKU corresponds to a bundle product type. Non-bundle SKUs will not return valid results when querying for option types.
What Happens If No Options Are Found?
If no options are found for a given SKU, the getItems()
method will return an empty array. Ensure the SKU belongs to a bundle product and has associated options.
How Can I Handle Errors When Fetching Bundle Options?
Wrap the API call in a try-catch block to handle errors gracefully:
try {
$options = $this->productOptionType->getItems();
} catch (Exception $exception) {
$this->logger->error($exception->getMessage());
}