Retrieving Child Items from Bundle Products in Magento 2
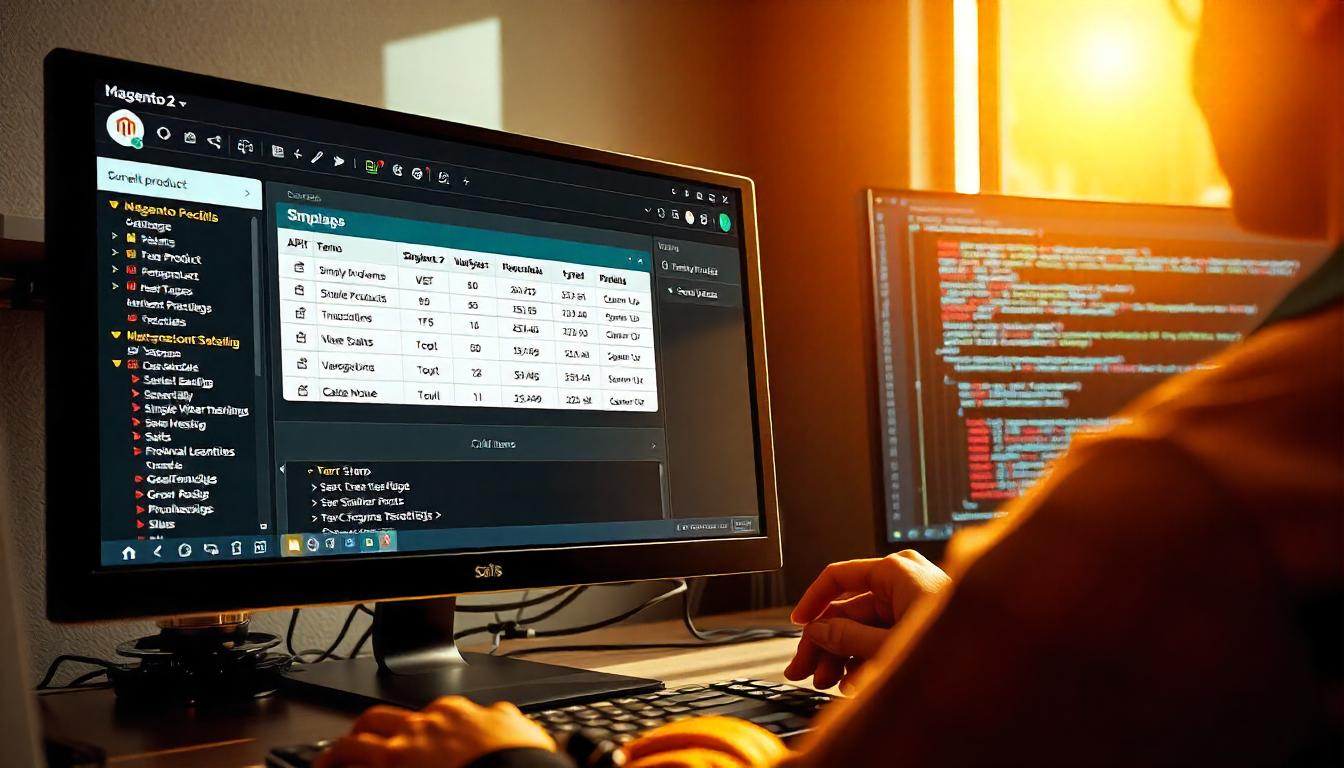
Retrieving Child Items from Bundle Products in Magento 2
In Magento 2, bundle products consist of multiple simple or virtual products grouped together. To fetch the child items of a bundle product, you can use the Magento\Bundle\Api\ProductLinkManagementInterface interface.
Table Of Content
Retrieving Child Items from Bundle Products in Magento 2
In Magento 2, bundle products are collections of simple or virtual products. To access all child items of a bundle product, utilize the Magento\Bundle\Api\ProductLinkManagementInterface
. This interface provides the getChildren()
method, which retrieves child products based on the parent bundle's SKU.
Method Overview
public function getChildren($productSku, $optionId = null);
- $productSku: The SKU of the bundle product.
- $optionId (optional): The specific option ID within the bundle. If omitted, all child items are returned.
Implementation Example
Here's how to implement this in a custom model:
<?php
namespace Vendor\Module\Model;
use Magento\Bundle\Api\ProductLinkManagementInterface;
use Psr\Log\LoggerInterface;
use Exception;
class BundleChildProduct
{
private $logger;
private $productLinkManagement;
public function __construct(
LoggerInterface $logger,
ProductLinkManagementInterface $productLinkManagement
) {
$this->logger = $logger;
$this->productLinkManagement = $productLinkManagement;
}
public function getBundleChildrenItems($sku)
try {
$items = $this->productLinkManagement->getChildren($sku);
return $items;
} catch (Exception $exception) {
$this->logger->error($exception->getMessage());
throw new Exception($exception->getMessage());
}
}
}
Usage:
$sku = 'your_bundle_product_sku';
$bundleChildProduct = new \Vendor\Module\Model\BundleChildProduct($logger, $productLinkManagement);
$items = $bundleChildProduct->getBundleChildrenItems($sku);
foreach ($items as $item) {
var_dump($item->getData());
}
Replace 'your_bundle_product_sku
' with the actual SKU of your bundle product. This code will output the data of each child item associated with the specified bundle product.
Sample Output:
array (size=10)
'entity_id' => string '26' (length=2)
'sku' => string '24-WG081-blue' (length=13)
'option_id' => string '1' (length=1)
'position' => string '1' (length=1)
'is_default' => string '1' (length=1)
'price' => null
'id' => string '1' (length=1)
'qty' => string '1.0000' (length=6)
'selection_can_change_quantity' => string '1' (length=1)
'price_type' => null
This output displays the details of each child item, including the entity ID, SKU, option ID, position, default status, price, quantity, and other relevant information.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Child Items from a Bundle Product in Magento 2?
You can retrieve child items from a bundle product using the Magento\Bundle\Api\ProductLinkManagementInterface
. The getChildren()
method allows you to fetch all child items linked to a bundle product.
What Is the Method to Get Child Items of a Bundle Product?
Use the following method from ProductLinkManagementInterface
to get child items:
public function getChildren($productSku, $optionId = null);
Parameters:
$productSku
- The SKU of the bundle product.$optionId
(optional) - The specific option ID to filter child products.
Can I Retrieve All Child Items Without an Option ID?
Yes, if $optionId
is set to null
, all child items of the bundle product will be retrieved.
What Does the Output Look Like?
The output will be an array containing the child items of the bundle product:
array (size=10)
'entity_id' => string '26' (length=2)
'sku' => string '24-WG081-blue' (length=13)
'option_id' => string '1' (length=1)
'position' => string '1' (length=1)
'is_default' => string '1' (length=1)
'price' => null
'id' => string '1' (length=1)
'qty' => string '1.0000' (length=6)
'selection_can_change_quantity' => string '1' (length=1)
'price_type' => null
How Do I Implement This in PHP?
Use the following PHP code to retrieve child items of a bundle product:
$sku = '24-WG080'; // Bundle product SKU
$items = $this->productLinkManagement->getChildren($sku);
foreach ($items as $item) {
var_dump($item->getData());
}
What Happens If the Bundle Product Has No Child Items?
If the bundle product does not contain any child items, the getChildren()
method will return an empty array.
Where Are Bundle Product Child Items Stored in Magento 2?
Child items of bundle products are stored in the catalog_product_bundle_selection
table in the Magento database.
How Can I Handle Errors When Retrieving Child Items?
Use a try-catch block to catch exceptions and log errors if the bundle product does not exist:
try {
$items = $this->productLinkManagement->getChildren($sku);
} catch (Exception $exception) {
$this->logger->error($exception->getMessage());
}