Determining Custom Options in Magento 2 Products
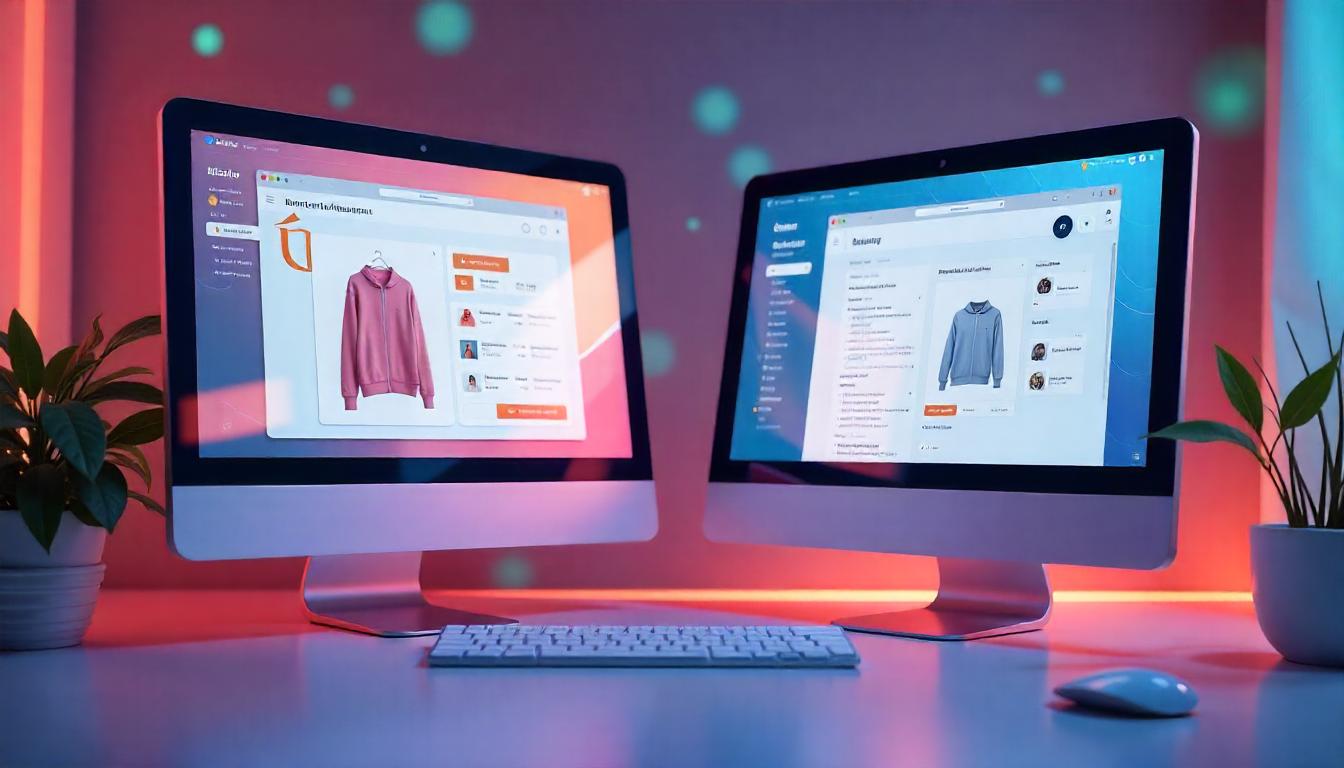
Determining Custom Options in Magento 2 Products
In Magento 2, you can check whether a product has custom options using the hasOptions() method. Custom options allow additional product variations without using complex product types like Configurable or Bundle. Simple, Virtual, Grouped, and Downloadable products typically do not support options, while Bundle and Configurable products do.
Table Of Content
Determining Custom Options in Magento 2 Products
To identify if a product in Magento 2 has custom options, you can utilize the hasOptions()
method on the product object. This method returns a boolean indicating the presence of custom options.
Implementation Steps:
Inject Dependencies:
- ProductRepositoryInterface: Allows retrieval of product information.
- LoggerInterface: Facilitates logging of errors.
Create a Method to Check for Custom Options:
- Use the
hasOptions()
method to determine if the product has custom options.
- Retrieve the product using its SKU.
Sample Code:
namespace Vendor\Module\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Psr\Log\LoggerInterface;
use Magento\Framework\Exception\NoSuchEntityException;
class ProductOptionsChecker
{
private $productRepository;
private $logger;
public function __construct(
ProductRepositoryInterface $productRepository,
LoggerInterface $logger
) {
$this->productRepository = $productRepository;
$this->logger = $logger;
}
public function hasCustomOptions($sku)
{
try {
$product = $this->productRepository->get($sku);
return $product->hasOptions();
} catch (NoSuchEntityException $e) {
$this->logger->error("Product with SKU {$sku} not found.");
return false;
}
}
}
Usage:
$checker = new \Vendor\Module\Model\ProductOptionsChecker($productRepository, $logger);
$sku = 'your-product-sku';
if ($checker->hasCustomOptions($sku)) {
echo "Product has custom options.";
} else {
echo "Product does not have custom options.";
}
Product Types and Custom Options:
Product Type | Supports Custom Options |
---|---|
Simple | Yes |
Configurable | No |
Bundle | No |
Virtual | Yes |
Grouped | No |
Downloadable | Yes |
Gift Card | Yes |
Note: While configurable and bundle products do not support custom options themselves, their associated simple products can have custom options.
Alternative Method:
if ($product->getTypeInstance()->hasOptions($product)) {
// Product has custom options
}
This method checks the product's type instance to determine the presence of options.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Do I Check if a Product Has Custom Options in Magento 2?
You can use the hasOptions()
method to determine if a product has custom options. This method is available in the product object.
Which Product Types Support Custom Options?
Products that support custom options:
- Bundle
- Configurable
Products that do not support custom options:
- Simple
- Virtual
- Grouped
- Downloadable
- Gift Card
How Can I Check for Custom Options in PHP?
Use the following PHP code to check if a product has custom options:
$product = $this->productRepository->get('24-WG080');
$hasOptions = $product->hasOptions();
echo $hasOptions ? 'Product has options' : 'No options available';
What Will the Output Look Like?
The output will be:
Bundle (24-WG080) -> HasOptions: 1
Configurable (MJ08) -> HasOptions: 1
Grouped (24-WG085_Group) -> HasOptions: 0
Downloadable (240-LV04) -> HasOptions: 0
Simple (24-MB01) -> HasOptions: 0
Virtual Product -> HasOptions: 0
What Happens If a Product Doesn’t Have Custom Options?
If a product does not have custom options, hasOptions()
will return false
, and no additional options will be available.
Can I Check Custom Options for Multiple Products at Once?
Yes, by iterating over multiple product SKUs, you can check the custom options status for multiple products.
Where Are Custom Options Stored in Magento 2?
Custom options are stored in the catalog_product_option
and catalog_product_option_type_value
tables in the Magento database.
How Can I Handle Errors When Checking for Custom Options?
Use a try-catch block to handle exceptions and prevent errors if a product does not exist.