Updating Product Special Prices in Magento 2
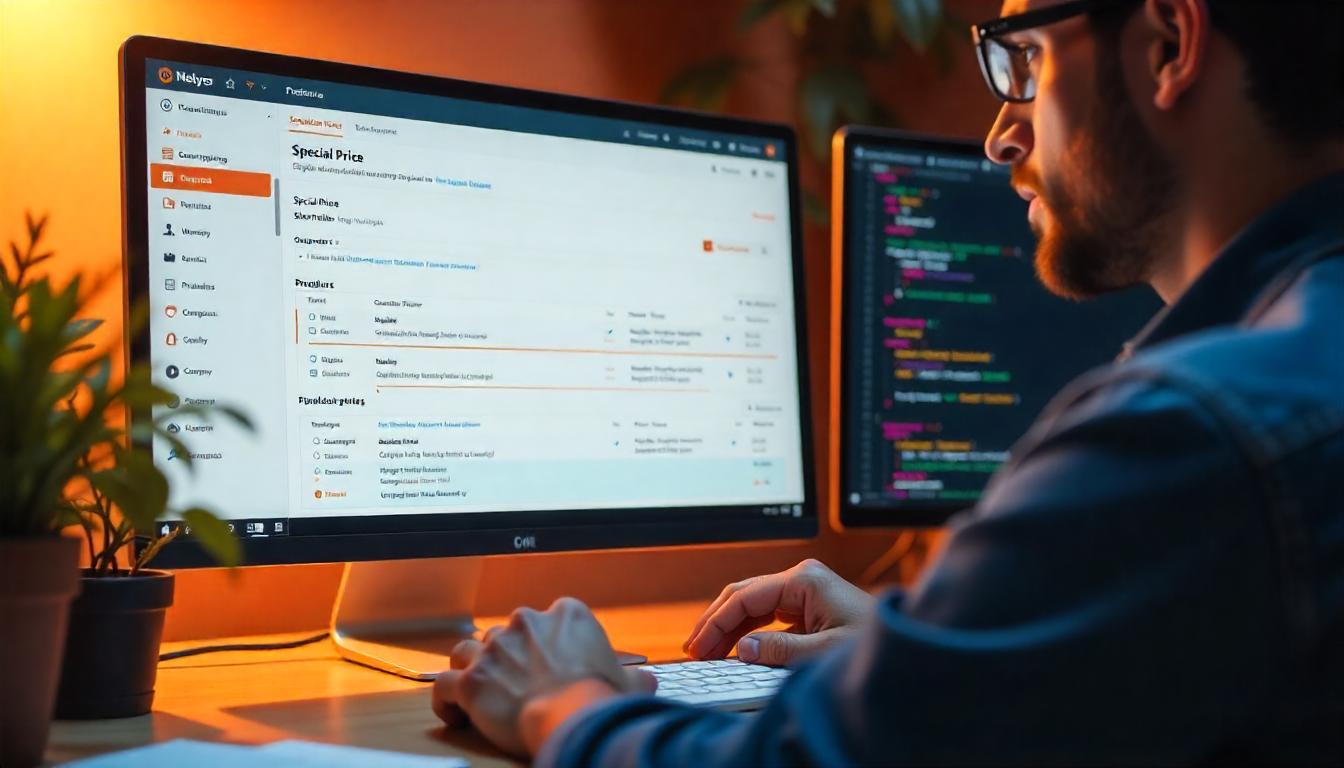
Updating Product Special Prices in Magento 2
Updating product special prices in Magento 2 allows you to apply time-sensitive discounts or promotional pricing. Using the SpecialPriceInterface, you can programmatically set, update, or remove special prices for specific products. This ensures price adjustments are automated and accurately scheduled.
Table Of Content
Updating Product Special Prices in Magento 2
To set or change a product's special price in Magento 2, you can use the SpecialPriceInterface. This interface allows you to programmatically manage special pricing for products.
Method Definition:
Parameters
Key | Type | Description |
---|---|---|
store_id |
int | The ID of the store where the special price applies. |
sku |
string | The Stock Keeping Unit of the product. |
price |
float | The special price value. |
price_from |
string | The start date for the special price in 'Y-m-d H:i:s' format (UTC). |
price_to |
string | The end date for the special price in 'Y-m-d H:i:s' format (UTC). |
Example Code to Update Special Price:
<?php
namespace Vendor\Module\Model;
use Psr\Log\LoggerInterface;
use Magento\Catalog\Api\SpecialPriceManagementInterface;
use Magento\Catalog\Api\Data\SpecialPriceInterfaceFactory;
use Magento\Framework\Exception\CouldNotSaveException;
class UpdateSpecialPrice
{
private $specialPriceManagement;
private $specialPriceFactory;
private $logger;
public function __construct(
SpecialPriceManagementInterface $specialPriceManagement,
SpecialPriceInterfaceFactory $specialPriceFactory,
LoggerInterface $logger
) {
$this->specialPriceManagement = $specialPriceManagement;
$this->specialPriceFactory = $specialPriceFactory;
$this->logger = $logger;
}
public function applySpecialPrice()
{
$sku = '24-MB01';
$price = 10.99;
$priceFrom = '2025-02-01';
$priceTo = '2025-02-28';
try {
$specialPrice = $this->specialPriceFactory->create();
$specialPrice->setSku($sku)
->setStoreId(0)
->setPrice($price)
->setPriceFrom($priceFrom)
->setPriceTo($priceTo);
$this->specialPriceManagement->update([$specialPrice]);
return true;
} catch (CouldNotSaveException $e) {
$this->logger->error(__('Error updating special price: %1', $e->getMessage()));
} catch (\Exception $e) {
$this->logger->error(__('Unexpected error: %1', $e->getMessage()));
}
return false;
}
}
In this example, replace '24-MB01' with your product's SKU. The price_from and price_to fields define the period during which the special price is active. If these fields are omitted, the special price will be applied immediately and remain until changed.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Update Product Special Prices in Magento 2?
You can update product special prices using the SpecialPriceInterface
. The update(array $prices)
method allows you to set special prices for products programmatically.
What Parameters Are Required to Update Special Prices?
To update a special price, you need the following parameters:
store_id
: The store ID where the price applies.sku
: The product's SKU.price
: The special price value.price_from
(optional): Start date in 'Y-m-d H:i:s' format.price_to
(optional): End date in 'Y-m-d H:i:s' format.
How Do I Use PHP to Update Special Prices?
You can use the following PHP class to update special prices:
$prices[] = $this->specialPriceFactory->create()
->setSku('24-MB01')
->setStoreId(0)
->setPrice(10.99)
->setPriceFrom('2025-02-01')
->setPriceTo('2025-02-28');
$this->specialPrice->update($prices);
What Happens If I Don't Set a Start or End Date?
If you omit the price_from
and price_to
parameters, the special price will be applied immediately and remain until manually changed.
Can I Update Special Prices for Multiple Products at Once?
Yes, you can update multiple product special prices by passing an array of special price objects to the update
method.
What Is the Output Format When Updating Special Prices?
The update
method applies the special prices without returning a response. However, you should handle exceptions to catch any errors.
How Can I Optimize Performance When Updating Special Prices?
To improve performance, use dependency injection for SpecialPriceInterface
instead of using the object manager. This reduces processing overhead.
Where Are Special Prices Stored in Magento 2?
Special price data is stored in the catalog_product_entity_decimal
table, linked to product attributes.
Can I Remove a Special Price Programmatically?
Yes, you can remove a special price by updating the product with an empty or null value for the special price attribute.