Accessing DHL Shipping Sandbox and Gateway URLs in Magento 2
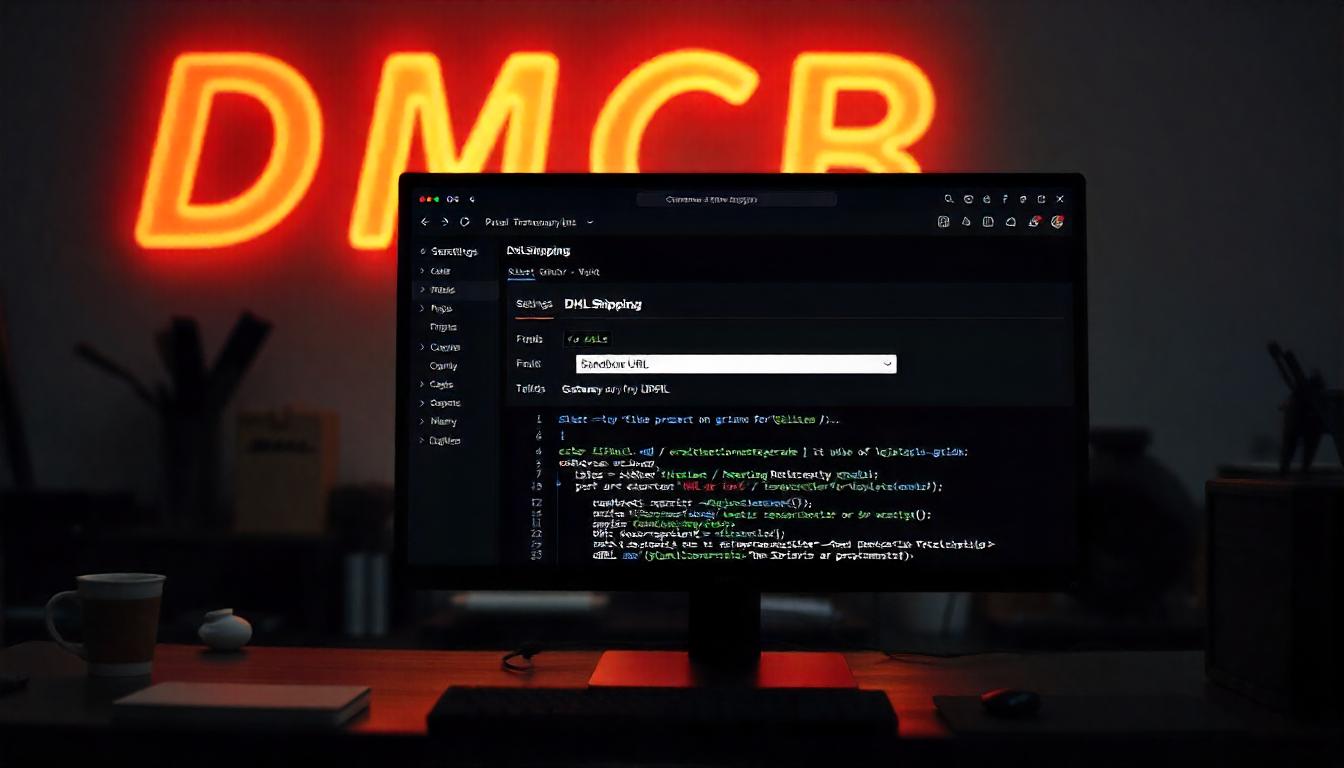
Accessing DHL Shipping Sandbox and Gateway URLs in Magento 2
Accessing the DHL Shipping Sandbox and Gateway URLs in Magento 2 is essential for integrating DHL's shipping services into your store. By retrieving these URLs programmatically, you can easily work with both the testing (sandbox) and live (gateway) environments.
Table Of Content
Accessing DHL Shipping Sandbox and Gateway URLs in Magento 2
Magento 2's built-in DHL shipping method allows you to integrate DHL services into your store. For development and testing, you might need the sandbox URL, while for live operations, the gateway URL is essential. You can retrieve both URLs programmatically using the following approach:
Retrieving DHL URLs Programmatically
To access the DHL sandbox and gateway URLs in Magento 2, create a custom model that fetches these values from the configuration:
<?php
namespace YourNamespace\Dhl\Model;
use Magento\Store\Model\ScopeInterface;
use Magento\Framework\App\Config\ScopeConfigInterface;
class ShippingUrl
{
/**
* @var ScopeConfigInterface
*/
private $scopeConfig;
public function __construct(
ScopeConfigInterface $scopeConfig
) {
$this->scopeConfig = $scopeConfig;
}
/**
* Get DHL Sandbox URL
*
* @return string
*/
public function getSandboxUrl()
{
$path = 'carriers/dhl/sandbox_url';
return $this->scopeConfig->getValue(
$path,
ScopeInterface::SCOPE_STORE
);
}
/**
* Get DHL Gateway URL
*
* @return string
*/
public function getGatewayUrl()
{
$path = 'carriers/dhl/gateway_url';
return $this->scopeConfig->getValue(
$path,
ScopeInterface::SCOPE_STORE
);
}
}
</div>
Usage Example
To use this model and retrieve the URLs:
$shippingUrlModel = $this->objectManager->create('YourNamespace\Dhl\Model\ShippingUrl');
$gatewayUrl = $shippingUrlModel->getGatewayUrl();
$sandboxUrl = $shippingUrlModel->getSandboxUrl();
echo 'DHL Gateway URL: ' . $gatewayUrl;
echo 'DHL Sandbox URL: ' . $sandboxUrl;
Understanding the Code
- ScopeConfigInterface: This interface allows access to the configuration values stored in Magento's system.
- ScopeInterface::SCOPE_STORE: This constant specifies that the configuration value is retrieved at the store level.
- getValue(): This method fetches the configuration value based on the provided path.
Additional Configuration
Ensure that the DHL shipping method is enabled in your Magento 2 store:
- Navigate to Stores > Settings > Configuration.
- Under Sales, select Delivery Methods.
- Expand the DHL section.
- Set Enabled for Checkout to
Yes
. - Enter the appropriate Gateway URL and Sandbox URL provided by DHL.
For more detailed information, refer to Adobe's official documentation on configuring DHL shipping methods.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve the DHL Sandbox and Gateway URLs in Magento 2?
To retrieve the DHL Sandbox and Gateway URLs programmatically in Magento 2, you can use the ScopeConfigInterface
to fetch the values stored in the configuration settings for the DHL carrier.
What Is the Purpose of ScopeConfigInterface
in Magento 2?
The ScopeConfigInterface
is used to access configuration values in Magento 2. It allows you to retrieve store-specific configuration data, such as the DHL Sandbox and Gateway URLs.
How Do I Retrieve the DHL Sandbox URL Programmatically?
You can retrieve the DHL Sandbox URL by calling the getSandboxUrl()
method, which fetches the configuration value for the DHL Sandbox URL:
$shippingUrlModel = $this->objectManager->create('YourNamespace\Dhl\Model\ShippingUrl');
$sandboxUrl = $shippingUrlModel->getSandboxUrl();
echo $sandboxUrl;
How Do I Retrieve the DHL Gateway URL?
To retrieve the DHL Gateway URL, use the getGatewayUrl()
method, which fetches the configuration value for the Gateway URL:
$gatewayUrl = $shippingUrlModel->getGatewayUrl();
echo $gatewayUrl;
What If I Don't Specify a Store ID or Code?
If you don't specify a store ID or code, the method will return the URL for the current store by default. This is useful if you are working with a single store environment.
Can I Retrieve URLs for Multiple Stores?
Yes, by specifying different store IDs or store codes, you can retrieve the DHL Sandbox and Gateway URLs for multiple stores in your Magento 2 setup.
How Can I Improve the Performance of Retrieving DHL URLs?
To optimize the code, use dependency injection for the ScopeConfigInterface
and avoid relying on the object manager. This leads to cleaner code and better performance.
What Is the Role of the getSandboxUrl()
and getGatewayUrl()
Methods?
The getSandboxUrl()
and getGatewayUrl()
methods retrieve the respective URLs for the DHL Sandbox and Gateway environments. These methods allow you to programmatically access the URLs based on store configuration.