Fetching Currency Symbols in Magento 2
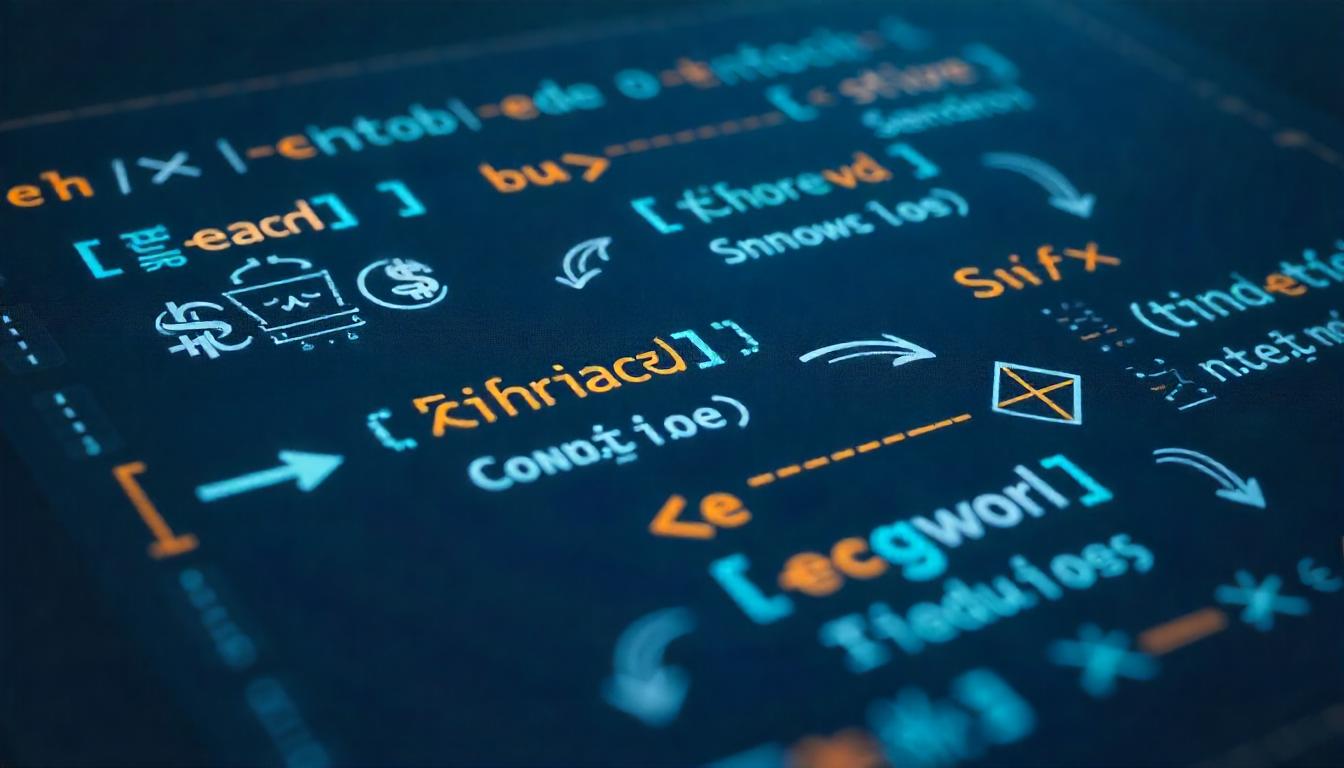
Fetching Currency Symbols in Magento 2
In Magento 2, retrieving the currency symbol for a store or website is straightforward using the PriceCurrencyInterface. This method allows you to fetch the currency symbol for the default store or specify a particular store by ID or code.
Table Of Content
Fetching Currency Symbols in Magento 2
To retrieve the currency symbol in Magento 2, utilize the PriceCurrencyInterface
's getCurrencySymbol()
method. This approach allows you to obtain the symbol for the default store currency or specify a particular store by its ID or code.
Method Syntax:
/**
* @param null|string|bool|int|\Magento\Framework\App\ScopeInterface $scope
* @param \Magento\Framework\Model\AbstractModel|string|null $currency
* @return string
*/
public function getCurrencySymbol($scope = null, $currency = null);
Implementation Steps:
Create a Block or Model Class:
Begin by creating a class that will use the PriceCurrencyInterface
to fetch the currency symbol.
<?php
namespace YourNamespace\Currency\Model;
use Magento\Framework\Pricing\PriceCurrencyInterface;
class CurrencySymbol
{
private PriceCurrencyInterface $priceCurrency;
public function __construct(PriceCurrencyInterface $priceCurrency)
{
$this->priceCurrency = $priceCurrency;
}
public function getCurrencySymbol($scope = null, $currencyCode = null): string
{
$currency = $this->priceCurrency->getCurrency($scope, $currencyCode);
return $currency ? $currency->getCurrencySymbol() : '';
}
}
Usage Examples:
You can call the getCurrencySymbol()
method with different parameters to fetch the desired currency symbol:
// Instantiate the CurrencySymbol class
$currencySymbolModel = new \YourNamespace\Currency\Model\CurrencySymbol($priceCurrency);
// Get the default store currency symbol
echo $currencySymbolModel->getCurrencySymbol();
// Get the currency symbol by store ID
echo $currencySymbolModel->getCurrencySymbol(1);
// Get the currency symbol by store code
echo $currencySymbolModel->getCurrencySymbol('default');
Parameter Details:
- $scope: Accepts null, store ID, or store code. If null, it defaults to the current store.
- $currency: Accepts null or a currency code string. If null, it uses the store's base currency.
Additional Methods:
Alternatively, you can retrieve the current currency symbol using the LocaleCurrency class:
use Magento\Framework\Locale\CurrencyInterface;
class CurrencySymbol
{
private $localeCurrency;
public function __construct(CurrencyInterface $localeCurrency)
{
$this->localeCurrency = $localeCurrency;
}
public function getCurrentCurrencySymbol()
{
$currencyCode = $this->localeCurrency->getCurrency()->getCurrencyCode();
return $this->localeCurrency->getCurrency($currencyCode)->getSymbol();
}
}
In this method, getCurrency()
retrieves the currency object for the current locale, and getSymbol()
fetches its symbol.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve the Currency Symbol in Magento 2?
To retrieve the currency symbol in Magento 2, you can use the PriceCurrencyInterface
with its getCurrencySymbol()
method. This allows fetching the symbol for the default store or any specific store by its ID or code.
What Is the Purpose of PriceCurrencyInterface
?
The PriceCurrencyInterface
handles currency-related operations in Magento 2. It provides the getCurrencySymbol()
method to retrieve the symbol for a specified currency, whether it's the default store's currency or another store's currency.
How Do I Retrieve the Currency Symbol Using Store ID or Code?
You can retrieve the currency symbol by passing the store ID or store code to the getCurrencySymbol()
method like this:
$currencySymbol = $this->getCurrencySymbol(1); // By store ID
$currencySymbol = $this->getCurrencySymbol('default'); // By store code
echo $currencySymbol;
What If I Don't Pass a Store ID or Code?
If you don't pass a store ID or code, the method will return the currency symbol of the current store by default.
Can I Retrieve Currency Symbols for Multiple Stores?
Yes, by passing different store IDs or codes to the getCurrencySymbol()
method, you can fetch the currency symbol for multiple stores.
How Can I Optimize the Code for Retrieving Currency Symbols?
To optimize the code, use dependency injection for the PriceCurrencyInterface
instead of relying on the object manager. This improves performance and ensures cleaner code.
What Is the Role of the getCurrencySymbol()
Method?
The getCurrencySymbol()
method retrieves the currency symbol for the specified store. It can return the symbol for the default store or any other store depending on the parameters passed.