Accessing Customer Date of Birth in Magento 2
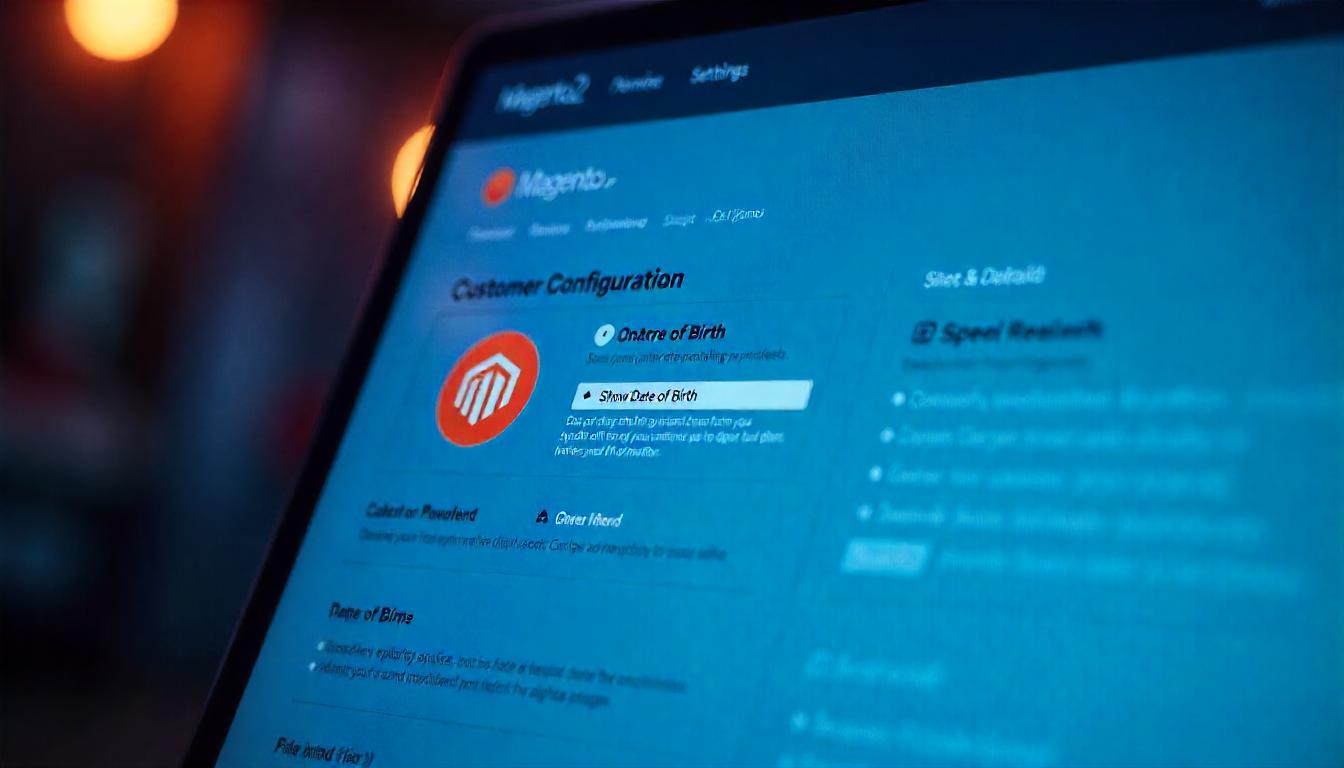
Accessing Customer Date of Birth in Magento 2
Accessing the customer date of birth (DOB) in Magento 2 allows you to retrieve and display important customer information for various use cases, like personalizing experiences or processing age-related settings. By default, the DOB field is disabled in Magento 2's customer registration form.
Table Of Content
Accessing Customer Date of Birth in Magento 2
In Magento 2, retrieving a customer's date of birth (DOB) by their customer ID is straightforward. By default, the DOB field is hidden on the registration page. To display it, you need to enable it in the configuration settings.
Enabling the DOB Field in Customer Registration
To make the DOB field visible during customer registration:
- Log in to the Admin Panel: Access your Magento 2 admin dashboard.
- Navigate to Configuration: Go to
Stores
>Configuration
. - Access Customer Configuration: Under
Customers
, selectCustomer Configuration
. - Modify Name and Address Options: Expand the
Name and Address Options
section. - Enable DOB Field: Set the
Show Date of Birth
option toRequired
orOptional
, depending on your preference. - Save Changes: Click
Save Config
to apply the changes.
This will display the DOB field on the customer registration page.
Retrieving Customer DOB by Customer ID
To fetch a customer's DOB using their customer ID, you can use the following PHP code:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Customer\Api\CustomerRepositoryInterface;
use Magento\Framework\Exception\LocalizedException;
use Magento\Framework\Exception\NoSuchEntityException;
class CustomerInfo
{
/**
* @var CustomerRepositoryInterface
*/
private $customerRepository;
public function __construct(
CustomerRepositoryInterface $customerRepository
) {
$this->customerRepository = $customerRepository;
}
/**
* Retrieve customer DOB by customer ID
*
* @param int $customerId
* @return string|null
*/
public function getCustomerDob($customerId)
{
try {
$customer = $this->customerRepository->getById($customerId);
return $customer->getDob();
} catch (NoSuchEntityException $exception) {
throw new LocalizedException(__('Customer not found.'));
}
}
}
In this code:
CustomerRepositoryInterface
is used to fetch customer data.- The
getCustomerDob
method retrieves the DOB inY-m-d
format (e.g.,1990-10-10
).
Usage Example
To use this method:
$customerId = 5;
$dob = $customerInfo->getCustomerDob($customerId);
echo $dob; // Outputs: 1990-10-10
Ensure that the CustomerInfo
class is properly instantiated and injected into your context.
Important Considerations
When handling customer DOBs, be mindful of privacy and security best practices. It's recommended to limit the storage of full birth dates and consider using only the year of birth to mitigate potential legal and security risks.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve a Customer's Date of Birth in Magento 2?
To retrieve a customer's date of birth (DOB) in Magento 2, you can use the CustomerRepositoryInterface
to fetch the DOB from the customer's data. The DOB can be accessed programmatically by the customer ID.
Why Is the Date of Birth Field Hidden by Default in Magento 2?
The DOB field is hidden by default during customer registration for privacy reasons. However, you can enable it in the configuration settings if you want to display it to users on the registration page.
How Do I Enable the Date of Birth Field on the Customer Registration Page?
To enable the DOB field, navigate to the configuration settings in Magento 2, under `Stores > Configuration > Customers > Customer Configuration`. From there, set the "Show Date of Birth" option to `Required` or `Optional`, depending on your needs.
How Do I Retrieve a Customer's Date of Birth by Their Customer ID?
You can retrieve a customer's DOB using the following PHP code:
$customerId = 5;
$dob = $customerInfo->getCustomerDob($customerId);
echo $dob; // Outputs: 1990-10-10
What Format Will the Date of Birth Be Returned In?
The customer's DOB will be returned in the format Y-m-d
(e.g., `1990-10-10`). This is the standard date format in Magento 2 for DOB values.
What Happens If the Customer Doesn't Have a Date of Birth Set?
If a customer has not set their date of birth, the getDob()
method will return `null`. You should check for this value in your code to handle cases where the DOB is not available.
Can I Retrieve the Date of Birth for Multiple Customers?
Yes, you can retrieve the DOB for multiple customers by iterating through customer IDs and calling the getCustomerDob()
method for each customer.
How Can I Improve the Performance When Retrieving Customer DOBs?
To improve performance, it's best to use dependency injection for the CustomerRepositoryInterface
and avoid using the object manager directly. This reduces overhead and leads to more efficient code.
What Is the Role of the getCustomerDob()
Method?
The getCustomerDob()
method retrieves the customer's date of birth from the database based on the provided customer ID. This allows you to access and display the DOB as needed in your Magento 2 store.