Access Product Gallery Images by SKU in Magento 2
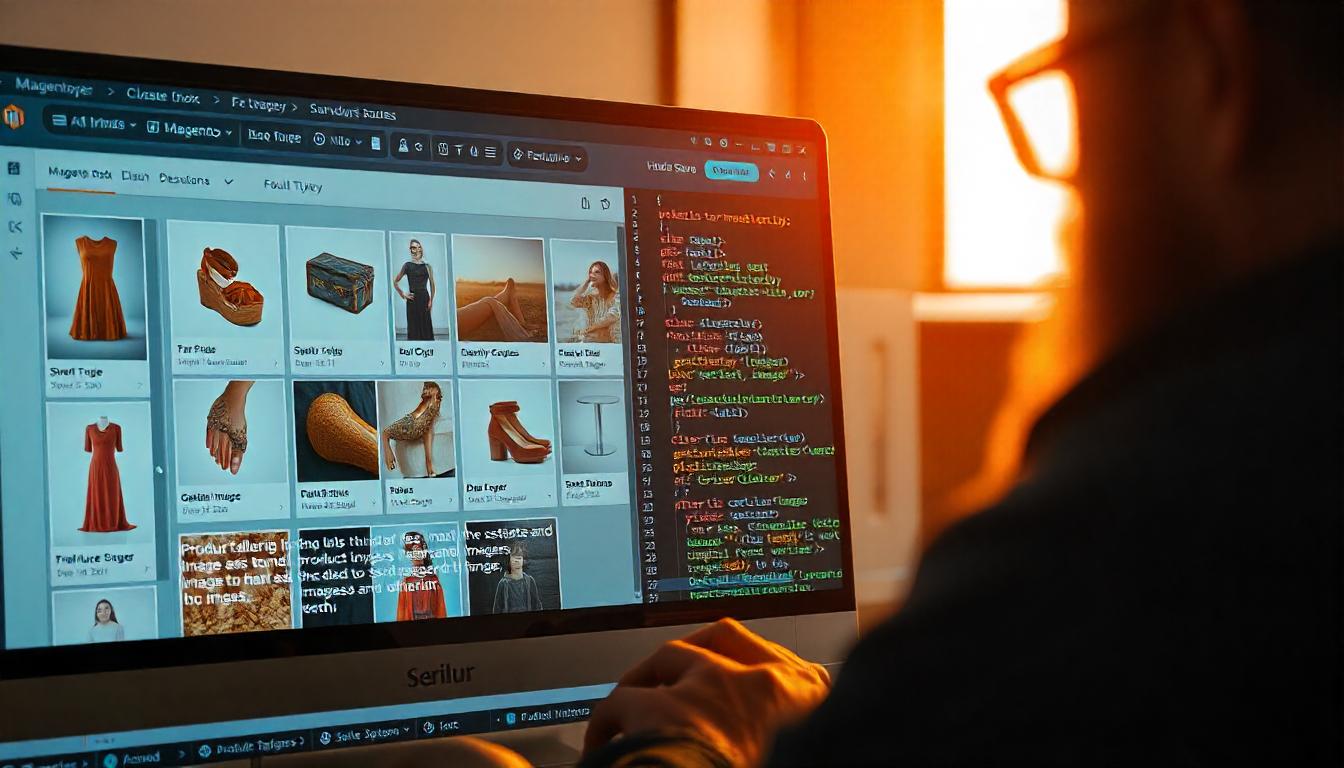
Access Product Gallery Images by SKU in Magento 2
Learn how to retrieve all product gallery images in Magento 2 using the SKU. This guide covers using the ProductAttributeMediaGalleryManagementInterface to fetch image details, including file paths, labels, positions, and assigned types like thumbnails and small images.
Table Of Content
Access Product Gallery Images by SKU in Magento 2
This guide shows you how to get all Magento 2 gallery images for a product using its SKU. You can quickly retrieve Magento 2 product gallery images with the ProductAttributeMediaGalleryManagementInterface
. This method returns an array of image details such as file path, position, label, and image types (e.g., small image, thumbnail).
Below is a sample implementation. This PHP class uses the getList($sku)
method to fetch the gallery images. If the SKU exists, the method returns an array of image entries. If not, it throws an exception.
<?php
namespace Jesadiya\MediaGallery\Model;
use Magento\Catalog\Api\ProductAttributeMediaGalleryManagementInterface;
use Magento\Catalog\Api\Data\ProductAttributeMediaGalleryEntryInterface;
use Psr\Log\LoggerInterface;
class GetMediaGallery {
/**
* @var ProductAttributeMediaGalleryManagementInterface
*/
private $productAttributeMediaGallery;
/**
* @var LoggerInterface
*/
private $logger;
public function __construct(
ProductAttributeMediaGalleryManagementInterface $productAttributeMediaGallery,
LoggerInterface $logger
) {
$this->productAttributeMediaGallery = $productAttributeMediaGallery;
$this->logger = $logger;
}
/**
* Retrieve gallery images by SKU.
*
* @param string $sku
* @return ProductAttributeMediaGalleryEntryInterface[]|null
*/
public function getMediaGallery(string $sku): ?array {
try {
return $this->productAttributeMediaGallery->getList($sku);
} catch (\Exception $exception) {
$this->logger->error(__('Error fetching media gallery: %1', $exception->getMessage()));
return null;
}
}
}
To fetch image details, call the method with a valid SKU. For example, use the sample SKU 24-MB03:
$sku = '24-MB03';
$gallery = $this->getMediaGallery($sku);
foreach ($gallery as $image) {
echo "<pre>";
print_r($image->getData());
}
The output is an array of image details. Here’s a summary table of the common keys in the result:
Key | Description |
---|---|
file | The path to the image file |
media_type | The type of media (typically "image") |
label | The label assigned to the image |
position | The display order of the image |
disabled | Status flag (0 for active, 1 for disabled) |
types | Roles assigned to the image (e.g., image, small_image, thumbnail) |
id | Unique identifier for the image |
This approach helps you efficiently retrieve Magento 2 gallery images. Use the focus keywords—Magento 2 gallery images, Magento 2 product gallery, and get gallery images Magento 2—to optimize your SEO and make the content easier to find.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Product Gallery Images in Magento 2?
You can retrieve all gallery images for a product using the ProductAttributeMediaGalleryManagementInterface
. The getList($sku)
method fetches all media entries associated with a product SKU.
What Details Can I Get from the Product Gallery?
Each gallery image entry includes details like file path, position, label, media type, and assigned roles such as thumbnail, small image, or swatch.
How Do I Use PHP to Fetch Gallery Images?
You can use the following PHP class to retrieve images:
$gallery = $this->productAttributeMediaGallery->getList($sku);
foreach ($gallery as $image) {
echo $image->getFile();
}
What Happens If a Product Has No Gallery Images?
If a product has no assigned images, the getList($sku)
method will return an empty array. Always check for an empty result before processing images.
Can I Retrieve Product Images for Multiple SKUs?
Yes, you can iterate through an array of SKUs and call getList($sku)
for each one to fetch their gallery images.
What Is the Output Format of the Gallery Images?
The output is an array containing image details. Example:
Array
(
[file] => /m/b/product-image.jpg
[media_type] => image
[label] => Main Image
[position] => 1
[types] => Array ( [0] => image [1] => thumbnail )
)
How Can I Improve Performance When Fetching Gallery Images?
To optimize performance, use dependency injection for ProductAttributeMediaGalleryManagementInterface
instead of the object manager. This reduces overhead and improves efficiency.
Where Is the Product Gallery Data Stored in Magento 2?
Magento 2 stores product gallery data in the catalog_product_entity_media_gallery
and catalog_product_entity_media_gallery_value
database tables.
Can I Modify the Image Roles Programmatically?
Yes, you can assign or modify image roles (such as base image, small image, or thumbnail) using the Magento 2 repository methods.