Retrieve Special Price by Product SKU in Magento 2
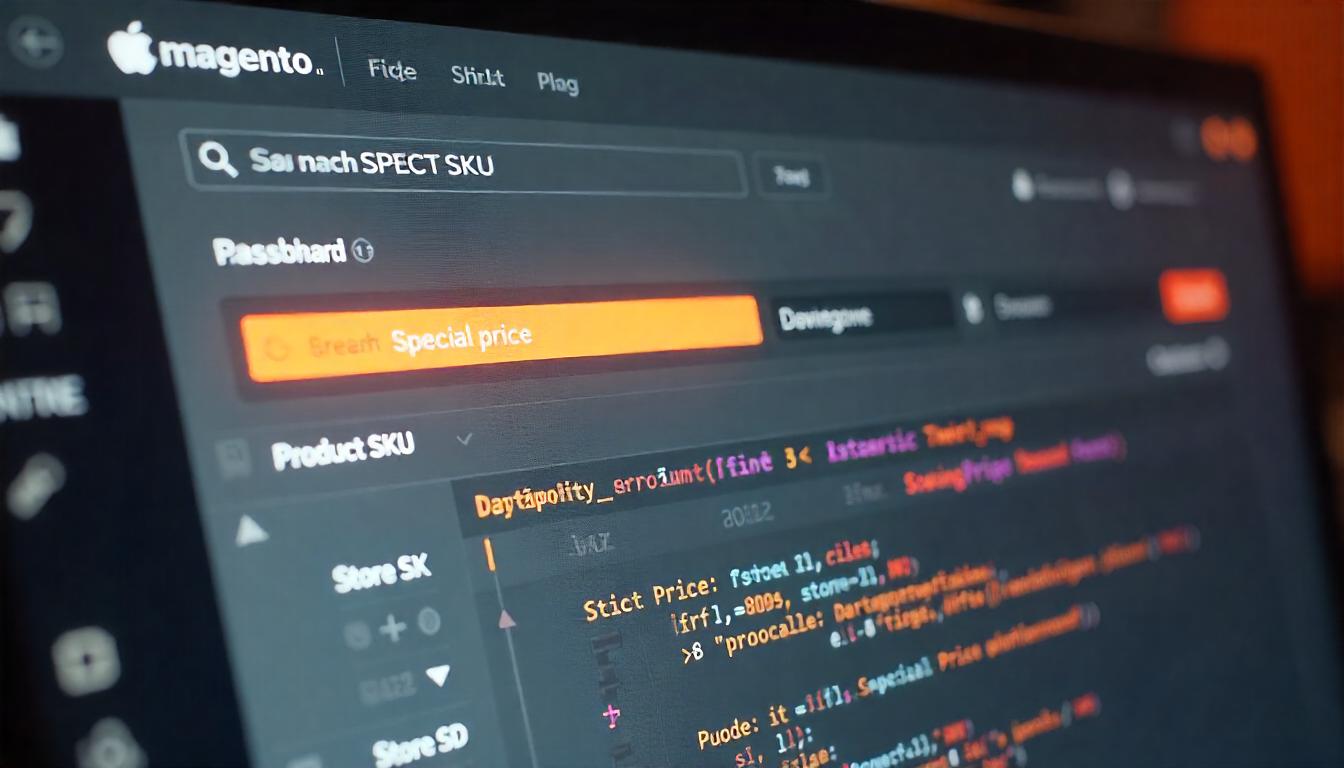
Retrieve Special Price by Product SKU in Magento 2
To get a product's special price in Magento 2 using its SKU, you can use the SpecialPriceInterface. This interface allows you to fetch special pricing details, including the discount value, start and end dates, store ID, and SKU.
Table Of Content
Retrieve Special Price by Product SKU in Magento 2
To retrieve a product's special price in Magento 2 using its SKU, you can utilize the SpecialPriceInterface. This interface allows you to access special pricing details, including the start and end dates, store ID, and SKU.
Implementation Steps:
- Inject the
SpecialPriceInterface
:
Begin by injecting the SpecialPriceInterface into your class's constructor:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Magento\Framework\Exception\NoSuchEntityException;
class SpecialPriceRetriever
{
private $productRepository;
public function __construct(ProductRepositoryInterface $productRepository)
{
$this->productRepository = $productRepository;
}
public function getSpecialPriceBySku(string $sku): ?float
{
try {
$product = $this->productRepository->get($sku);
return $product->getSpecialPrice() ?: null;
} catch (NoSuchEntityException $e) {
return null; // Return null if product does not exist
} catch (\Exception $e) {
throw new \Exception('Error retrieving special price: ' . $e->getMessage());
}
}
}
- Retrieve Special Price Data:
Call the getSpecialPriceBySku
method with the desired SKU:
$sku = 'your-product-sku';
$specialPriceRetriever = new \YourNamespace\YourModule\Model\SpecialPriceRetriever($specialPriceInterface);
$specialPriceData = $specialPriceRetriever->getSpecialPriceBySku($sku);
If the product has a special price, $specialPriceData will contain an array with details like value (the special price
), store_id
, sku
, price_from,
and price_to
. If there's no special price, the array will be empty.
Array
(
[0] => Array
(
[row_id] => 1
[value] => 29.99
[store_id] => 0
[sku] => 24-MB01
[price_from] => 1970-01-01 00:00:01
[price_to] => 2020-01-19 03:14:07
)
)
This method ensures you retrieve the special price data efficiently using the SKU in Magento 2.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Do I Retrieve a Product’s Special Price in Magento 2?
You can use the SpecialPriceInterface
to fetch special prices based on SKU. The get([$sku])
method returns special pricing details, including start and end dates.
What Parameters Are Required to Get a Special Price?
To retrieve a special price, you need:
sku
: The product SKU.store_id
: The store ID (default is 0).price_from
: The start date for the special price.price_to
: The end date for the special price.
How Do I Retrieve a Special Price Using PHP?
Use the following PHP code to fetch a special price:
$productSku = '24-MB01';
$specialPrice = $this->specialPriceInterface->get([$productSku]);
What Is the Output Format for Special Price Data?
If a product has a special price, the output will be an array like this:
Array
(
[0] => Array
(
[row_id] => 1
[value] => 29.99
[store_id] => 0
[sku] => 24-MB01
[price_from] => 1970-01-01 00:00:01
[price_to] => 2025-02-28 23:59:59
)
)
What Happens If a Product Has No Special Price?
If there is no special price set for a product, the returned array will be empty.
Can I Retrieve Special Prices for Multiple Products at Once?
Yes, you can pass multiple SKUs in an array to the get
method to fetch special prices for multiple products.
Where Is Special Price Data Stored in Magento 2?
Special price data is stored in the catalog_product_entity_decimal
table, linked to product attributes.
Can I Filter Special Prices by Date?
Yes, the special price records include price_from
and price_to
dates, which you can use to filter active discounts.
How Can I Handle Errors When Retrieving Special Prices?
Wrap the get
method in a try-catch block to handle exceptions and avoid crashes when retrieving special prices.