Removing Bundle Product Options by Option ID in Magento 2
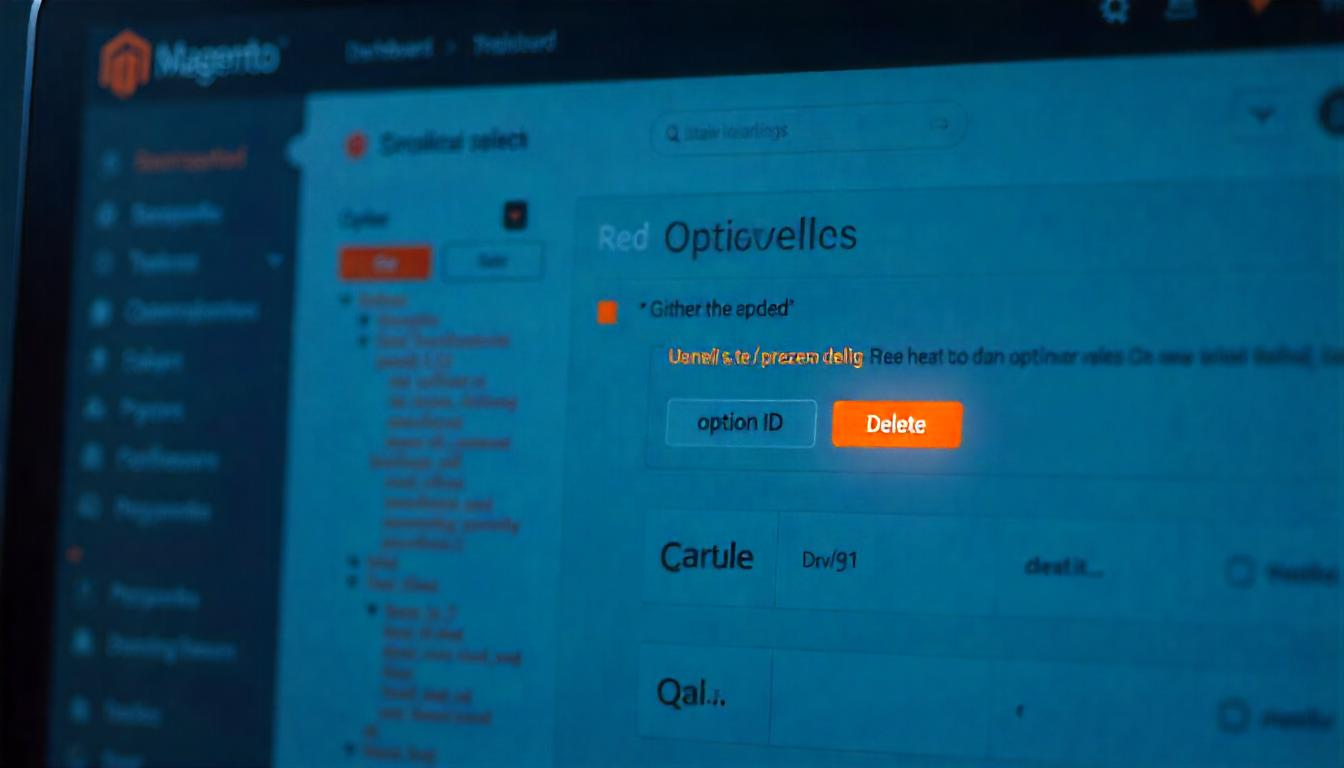
Removing Bundle Product Options by Option ID in Magento 2
Removing bundle product options in Magento 2 requires a programmatic approach using the option ID and the bundle product’s SKU. By leveraging the ProductOptionRepositoryInterface and its deleteById() method, you can efficiently delete specific bundle options.
Table Of Content
Removing Bundle Product Options by Option ID in Magento 2
To delete a specific bundle product option in Magento 2, you can do this programmatically using the option ID and the bundle product's SKU. Here's how:
- Inject the ProductOptionRepositoryInterface: In your class constructor, include the
Magento\Bundle\Api\ProductOptionRepositoryInterface
. - Use the deleteById() Method: Call the
deleteById()
method with the bundle product's SKU and the option ID you wish to remove.
Here's a concise example:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Bundle\Api\ProductOptionRepositoryInterface;
use Psr\Log\LoggerInterface;
use Magento\Framework\Exception\LocalizedException;
class RemoveBundleOption
{
private $productOptionRepository;
private $logger;
public function __construct(
ProductOptionRepositoryInterface $productOptionRepository,
LoggerInterface $logger
)
{
$this->productOptionRepository = $productOptionRepository;
$this->logger = $logger;
}
public function removeOption(string $sku, int $optionId): bool
{
try {
return $this->productOptionRepository->deleteById($sku, $optionId);
} catch (LocalizedException $e) {
$this->logger->error(__('Error removing option: %1', $e->getMessage()));
} catch (\Exception $e) {
$this->logger->critical(__('Unexpected error: %1', $e->getMessage()));
}
return false;
}
}
Parameters:
- $sku: The SKU of the parent bundle product.
- $optionId: The ID of the bundle option you want to delete.
Usage:
$remover = new \YourNamespace\YourModule\Model\RemoveBundleOption($productOptionRepository);
$success = $remover->removeOption('your-bundle-sku', 1);
if ($success) {
// Option was successfully removed
} else {
// Option removal failed
}
Ensure that the SKU and option ID are correct. If they are, the method returns true; otherwise, it throws an exception.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Remove a Specific Bundle Option in Magento 2?
You can remove a bundle product option programmatically using the Magento\Bundle\Api\ProductOptionRepositoryInterface
. The deleteById()
method allows you to delete an option using the bundle product's SKU and the option ID.
What Is the Correct Method to Delete a Bundle Option?
Use the following method from ProductOptionRepositoryInterface
to delete a bundle option:
public function deleteById($productSku, $optionId);
Parameters:
$productSku
- The SKU of the parent bundle product.$optionId
- The ID of the bundle option you want to remove.
Can I Remove Multiple Bundle Options at Once?
No, the deleteById()
method removes one option at a time. If you need to delete multiple options, you must call the method separately for each option ID.
How Do I Implement This in PHP?
Use the following PHP code to delete a bundle option:
$sku = '24-WG080'; // Parent bundle product SKU
$optionId = 1;
try {
$this->productOptionRepository->deleteById($sku, $optionId);
} catch (Exception $e) {
// Handle exception
echo $e->getMessage();
}
What Happens If the Option ID Does Not Exist?
If the provided option ID does not exist, the deleteById()
method will throw an exception. Ensure the option ID is valid before calling the method.
Where Are Bundle Product Options Stored in Magento 2?
Bundle product options are stored in the catalog_product_bundle_option
table in the Magento database.
How Can I Handle Errors When Deleting a Bundle Option?
Use a try-catch block to catch exceptions and log errors if the bundle option cannot be deleted:
try {
$this->productOptionRepository->deleteById($sku, $optionId);
} catch (Exception $exception) {
$this->logger->error($exception->getMessage());
}