How to Retrieve All Bundle Item Option Details by SKU in Magento 2
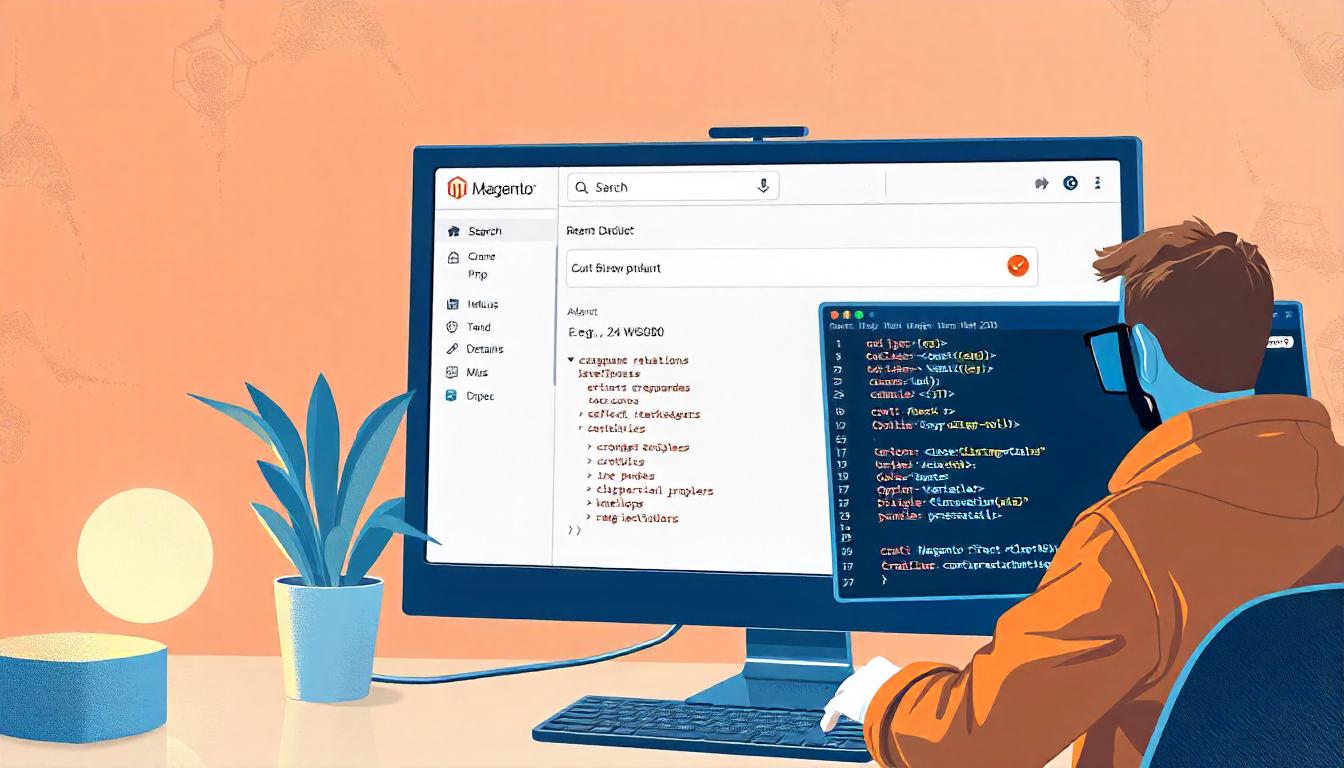
How to Retrieve All Bundle Item Option Details by SKU in Magento 2
Learn how to retrieve all bundle product option details by SKU in Magento 2 using ProductOptionRepositoryInterface. This guide walks you through fetching bundle options programmatically, handling errors, and displaying option details efficiently.
Table Of Content
How to Retrieve All Bundle Item Option Details by SKU in Magento 2
To retrieve all bundle product option details by SKU in Magento 2, you can use the ProductOptionRepositoryInterface
. This interface provides methods to access bundle product options efficiently.
Steps to Retrieve Bundle Product Options by SKU:
- Inject Dependencies: In your class, inject the ProductOptionRepositoryInterface and LoggerInterface for logging purposes.
namespace Vendor\Module\Model;
use Psr\Log\LoggerInterface;
use Magento\Bundle\Api\ProductOptionRepositoryInterface;
class BundleOptions
{
private ProductOptionRepositoryInterface $productOptionRepository;
private LoggerInterface $logger;
public function __construct(
ProductOptionRepositoryInterface $productOptionRepository,
LoggerInterface $logger
) {
$this->productOptionRepository = $productOptionRepository;
$this->logger = $logger;
}
public function getBundleOptions(int $productId)
{
try {
return $this->productOptionRepository->get($productId);
} catch (\Exception $e) {
$this->logger->error(__('Error fetching bundle options: %1', $e->getMessage()));
return null;
}
}
}
- Fetch Bundle Options: Create a method to fetch bundle options using the getList method provided by
ProductOptionRepositoryInterface
.
public function getBundleOptionsBySku(string $sku): array
{
try {
$options = $this->productOptionRepository->getListByProductSku($sku);
return $options->getItems();
} catch (\Magento\Framework\Exception\NoSuchEntityException $e) {
$this->logger->warning("No bundle options found for SKU: {$sku}");
} catch (\Exception $e) {
$this->logger->error("Error fetching bundle options for SKU {$sku}: " . $e->getMessage());
}
return [];
}
- Display Option Details: Iterate through the retrieved options to access their details.
Usage:
$sku = 'your_bundle_product_sku';
$options = $this->getBundleOptionsBySku($sku);
foreach ($options as $option) {
// Access option data
$optionData = $option->getData();
// Example: Output option title
echo 'Option Title: ' . $optionData['title'] . PHP_EOL;
}
Important Considerations:
- Error Handling: Ensure you handle exceptions appropriately to avoid runtime errors. Log any exceptions for debugging purposes.
- Dynamic SKU: Replace
'your_bundle_product_sku'
with the actual SKU of your bundle product. - Option Details: The
$option->getData()
method returns an array containing detailslike option_id
, title, type, and associatedproduct_links
.
By following these steps, you can programmatically retrieve all bundle item option details by SKU in Magento 2. This approach leverages Magento's repository interfaces for efficient data access.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve All Bundle Item Option Details by SKU in Magento 2?
You can retrieve all bundle item options for a specific SKU using the Magento\Bundle\Api\ProductOptionRepositoryInterface
. The getList()
method allows you to fetch all bundle options for a given product SKU.
What Method Retrieves Bundle Options by SKU?
Use the getList()
method from ProductOptionRepositoryInterface
:
public function getList($productSku);
Parameters:
$productSku
- The SKU of the bundle product.
Can I Retrieve Bundle Options for Any Product SKU?
No, the getList()
method only works for bundle products. Ensure the SKU belongs to a bundle product before calling this method.
How Do I Implement This in PHP?
Use the following PHP code to retrieve bundle item options:
$sku = '24-WG080'; // Bundle product SKU
try {
$options = $this->productOptionRepository->getList($sku);
foreach ($options as $option) {
echo "Option Title: " . $option->getTitle() . "
";
}
} catch (Exception $e) {
echo $e->getMessage();
}
What Happens If the SKU Does Not Exist?
If the provided SKU does not exist or is not a bundle product, the getList()
method will return an empty result or throw an exception.
Where Are Bundle Product Options Stored in Magento 2?
Bundle product options are stored in the catalog_product_bundle_option
table in the Magento database.
How Can I Handle Errors When Fetching Bundle Options?
Use a try-catch block to catch exceptions and log errors if bundle options cannot be retrieved:
try {
$options = $this->productOptionRepository->getList($sku);
} catch (Exception $exception) {
$this->logger->error($exception->getMessage());
}