How to Write a Text File and Create a Directory in Magento 2.4.7 (2025)
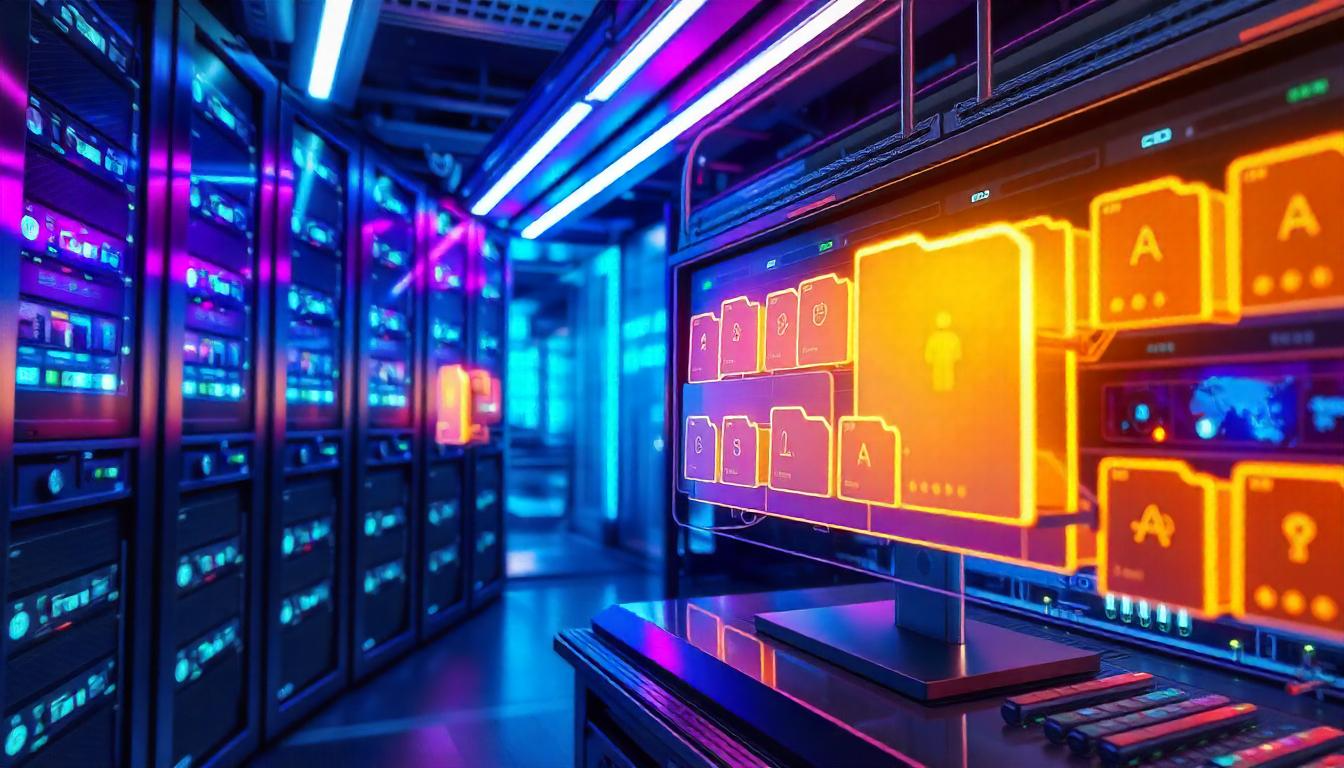
How to Write a Text File and Create a Directory in Magento 2.4.7 (2025)
In Magento 2.4.7, writing a text file programmatically and creating directories in a standardized Magento way is essential for logging, exporting data, and integration purposes.
Magento provides the Magento\Framework\Filesystem
component to handle file operations securely. This method is useful for:
Storing logs
Recording last processed orders
Exporting data for third-party integrations
This guide will show you how to:
- Create a custom directory inside
var/
- Write a text (.txt) file programmatically
- Use Magento’s best practices for file handling
Table Of Content
Why Use Magento’s Standard File System Approach?
Magento’s built-in Filesystem interface ensures:
- Security – Prevents unauthorized file access and enforces strict permissions.
- Scalability – Works seamlessly across various environments (Cloud, Docker, Local).
- Best Practices – Compliant with Magento’s coding standards, ensuring maintainability.
- Performance – Optimized for speed and efficiency in handling file operations.
- Reliability – Reduces errors by using Magento’s core mechanisms instead of direct file access.
Comparison: Direct File Access vs. Magento’s Filesystem
Feature | Direct File Access | Magento Filesystem |
---|---|---|
Security | Vulnerable to unauthorized access | Enforces access control |
Code Maintainability | Harder to manage and prone to errors | Uses Magento’s structured approach |
Scalability | May fail in cloud-based environments | Works across multiple environments |
Performance | Slower, depends on direct file handling | Optimized for Magento’s architecture |
Best Practices | Not recommended by Magento | Fully compliant |
Best Practices for Using Magento’s Filesystem
Use Magento’s Directory List Constants
Instead of hardcoding file paths, use:
$mediaDirectory = $this->filesystem->getDirectoryRead(\Magento\Framework\App\Filesystem\DirectoryList::MEDIA);
Avoid Direct PHP File Functions
Functions like file_get_contents()
or fopen()
should be avoided in favor of Magento’s filesystem methods.
Implement Proper Permissions
Ensure file permissions follow Magento’s security guidelines (644 for files, 755 for directories).
Always Validate File Inputs
If working with user-uploaded files, validate them to prevent security risks.
Tip:
For better debugging, enable Magento’s built-in logging system when working with files:
$this->logger->debug('File operation successful');
Using Magento’s standard filesystem approach ensures better security, scalability, and maintainability—making it the best choice for handling file operations in Magento 2.
File Writing Process Overview in Magento
Magento's Filesystem API ensures secure and scalable file operations. Follow these steps to implement file writing correctly.
Steps to Write a File in Magento:
Step | Action | Description |
---|---|---|
1. Inject Filesystem Dependency | Use Magento’s Filesystem component | Inject Magento\Framework\Filesystem in the constructor. |
2. Create Directory | Define a custom folder inside var/ |
Use DirectoryWriteInterface to create a directory. |
3. Write Content to File | Store dynamic text data using WriteInterface |
Write content securely using Magento’s API. |
4. Use in Custom Modules | Apply this approach in extensions or integrations | Follow best practices to maintain performance and security. |
Magento File Writing Best Practices
1. Use Magento’s Filesystem API Instead of Direct PHP Methods
Instead of using PHP functions like file_put_contents()
, use:
<?php
// Writing to a file in Magento 2
$directoryWrite = $this->filesystem->getDirectoryWrite(\Magento\Framework\App\Filesystem\DirectoryList::VAR_DIR);
$filePath = 'custom_folder/custom_file.txt';
$directoryWrite->writeFile($filePath, 'Your content here.');
?>
Why?
- Improves security by enforcing Magento’s permission structure.
- Ensures compatibility across different environments (cloud, Docker, local).
2. Implement Proper File and Folder Permissions
Ensure file permissions align with Magento’s security standards:
Type | Recommended Permission |
---|---|
Files | 644 (Read & Write for owner, Read for others) |
Directories | 755 (Read, Write, and Execute for owner, Read & Execute for others) |
Tip:
Run this command to fix permissions:
find var/ pub/static pub/media app/etc -type f -exec chmod 644 {} \;
find var/ pub/static pub/media app/etc -type d -exec chmod 755 {} \;
3. Validate and Sanitize File Inputs
If working with user-uploaded files, always validate them to prevent security risks. Example:
<?php
if (!$file->isFile() || !$file->isReadable()) {
throw new \Magento\Framework\Exception\LocalizedException(__('Invalid file.'));
}
?>
Why?
- Prevents unauthorized file uploads.
- Ensures data integrity before processing.
Debugging File Operations
To log file operations for debugging, use Magento’s logger:
<?php
$this->logger->debug('File operation successful');
?>
Tip:
Check logs in::
var/log/system.log
var/log/debug.log
Magento 2.4.7 Code to Write a Text File
1. Create a Model Class
Create WriteTextFile.php
inside your custom module:
file pathapp/code/Vendor/Module/Model/WriteTextFile.php
<?php
namespace Vendor\Module\Model;
use Magento\Framework\Filesystem;
use Magento\Framework\App\Filesystem\DirectoryList;
use Magento\Framework\Exception\FileSystemException;
use Magento\Framework\Filesystem\Directory\WriteInterface;
class WriteTextFile
{
/**
* @var Filesystem
*/
private $filesystem;
/**
* @var DirectoryList
*/
private $directoryList;
public function __construct(
Filesystem $filesystem,
DirectoryList $directoryList
) {
$this->filesystem = $filesystem;
$this->directoryList = $directoryList;
}
/**
* Create a directory and write text file
*
* @return bool
* @throws FileSystemException
*/
public function createDemoTextFile()
{
$varDirectory = $this->filesystem->getDirectoryWrite(DirectoryList::VAR_DIR);
$filePath = $this->directoryList->getPath('var') . '/custom/demo.txt';
// Ensure directory exists
$varDirectory->create('custom');
// Write Content
return $this->write($varDirectory, $filePath);
}
/**
* Write content to text file
*
* @param WriteInterface $writeDirectory
* @param string $filePath
* @return bool
* @throws FileSystemException
*/
private function write(WriteInterface $writeDirectory, string $filePath)
{
$stream = $writeDirectory->openFile($filePath, 'w+');
$stream->lock();
$stream->write('Last Imported Order ID: 10000001');
$stream->unlock();
$stream->close();
return true;
}
}
Explanation of the Code
Function | Purpose |
---|---|
createDemoTextFile() |
Creates a directory and writes a text file |
write() |
Writes content securely inside the text file |
DirectoryList::VAR_DIR |
Defines the path inside var/ folder |
$varDirectory->create('custom') |
Creates custom/ directory if it doesn’t exist |
2. Where is the File Stored?
The text file will be saved at:
var/custom/demo.txt
in Magento root directory.
3. How to Use This Code in Magento?
You can call this model method inside a controller, observer, or helper class like this:
$writeTextFile = $this->writeTextFile->createDemoTextFile();
Alternatively, run it in CLI:
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$writeTextFile = $objectManager->create(\Vendor\Module\Model\WriteTextFile::class);
$writeTextFile->createDemoTextFile();
4. Troubleshooting & Debugging
Issue | Possible Cause | Solution |
---|---|---|
Custom Layout Update not appearing | XML file is missing or incorrect | Check if the file exists in the correct path |
Dropdown not showing custom layout | Cache issue | Run php bin/magento cache:flush |
Layout changes not reflecting | Wrong XML structure | Verify the XML syntax |
5. Best Practices for Magento File Handling
- Use Magento’s
Filesystem
class instead of direct PHP file operations likefile_put_contents
for better security and compatibility. - Ensure proper permissions (e.g.,
777
for development or755
for production) on thevar/
directory and its subdirectories. - Use dependency injection instead of
ObjectManager
for improved performance and maintainability. - Always clear cache using
php bin/magento cache:flush
after making file-related changes to ensure updates take effect. - Check Magento error logs (
var/log/system.log
,var/log/exception.log
) if issues occur to diagnose problems quickly.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Managing files and directories in Magento 2 is crucial for maintaining a secure and well-optimized store. Instead of relying on direct PHP functions like file_put_contents, Magento provides the Filesystem class, which ensures compatibility with different environments and enhances security.
When working with files, proper directory permissions are essential. Use 777 only in development environments, while 755 is recommended for production to prevent unauthorized access. Additionally, following Magento’s dependency injection pattern rather than using ObjectManager ensures better performance, cleaner code, and easier debugging.
To avoid caching issues, always flush the cache using php bin/magento cache:flush after making any changes. If problems persist, Magento’s built-in logging system (var/log/system.log, var/log/exception.log) helps identify errors and debug effectively.
By implementing these best practices, you create a scalable, secure, and future-proof Magento 2 store. Whether you’re handling file exports, managing third-party integrations, or optimizing workflows, these techniques will enhance reliability, performance, and maintainability in your eCommerce setup. Stay updated with the latest Magento 2.4.7 (2025) improvements to ensure your store remains efficient and competitive!
FAQs
How do I create a text file in Magento 2 using the standard approach?
You can use Magento's Filesystem
class and Directory\WriteInterface
to create and write text files in a secure and structured way.
Where should I store custom text files in Magento 2?
Magento recommends storing custom text files in the var/
directory under a subfolder, such as var/custom/
, to keep them organized and easily accessible.
How can I ensure my Magento 2 custom directory is created?
Use $varDirectory->create('custom')
to programmatically create a directory if it doesn’t exist.
Why should I use Magento's Filesystem instead of PHP file functions?
Magento’s Filesystem class provides better security, compatibility, and integration with Magento’s architecture, unlike direct PHP file functions like file_put_contents
.
What file permissions should I set for text files in Magento 2?
For development, use 777
, but in production, set permissions to 755
to enhance security and prevent unauthorized access.
How do I flush Magento’s cache after creating a text file?
Run php bin/magento cache:flush
in the terminal to clear the cache and apply file-related changes instantly.
How can I debug file-writing issues in Magento 2?
Check Magento logs located in var/log/system.log
and var/log/exception.log
to identify and resolve any file-writing issues.
What is the purpose of the createDemoTextFile() function?
The createDemoTextFile()
function creates a directory and writes a text file in the Magento 2 var/
folder programmatically.
What does the write() function do in Magento 2 file handling?
The write()
function securely writes content into a text file, ensuring data integrity and preventing concurrency issues using file locking.
Why is my Magento 2 custom layout update not appearing?
Your XML file might be missing or incorrectly structured. Ensure it's placed in the correct path and follows Magento’s layout naming conventions.
How do I fix the dropdown not showing my custom layout update?
This is usually a caching issue. Run php bin/magento cache:flush
to refresh the cache and apply your layout changes.
Why are my Magento 2 layout changes not reflecting on the frontend?
Your XML structure might be incorrect. Verify the syntax and check for any missing or misplaced nodes.
How does dependency injection improve Magento 2 file handling?
Using dependency injection instead of ObjectManager
enhances performance, improves maintainability, and aligns with Magento’s best practices.