Removing an Attribute Set by ID in Magento 2
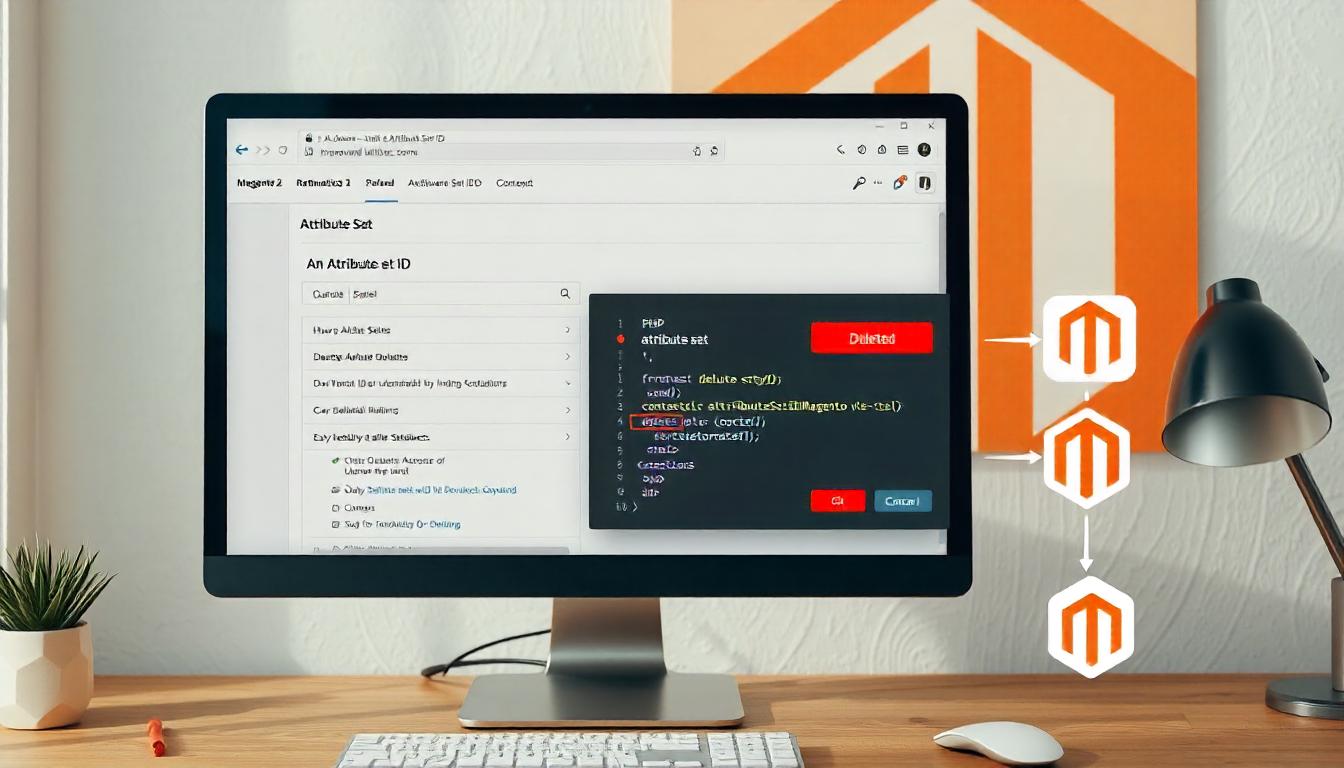
Removing an Attribute Set by ID in Magento 2
Deleting an attribute set by ID in Magento 2 helps keep your store organized by removing unnecessary attribute sets. You can achieve this using the deleteById($attributeSetId) method from the AttributeSetRepositoryInterface.
Table Of Content
Removing an Attribute Set by ID in Magento 2
To delete a specific attribute set in Magento 2, you can use the deleteById($attributeSetId)
method from the AttributeSetRepositoryInterface
. This approach allows you to remove an attribute set using its unique ID.
Implementing the Deletion Method
Here's how you can implement a function to delete an attribute set by its ID:
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Api\AttributeSetRepositoryInterface;
use Magento\Framework\Exception\LocalizedException;
class AttributeSetManager
{
/**
* @var AttributeSetRepositoryInterface
*/
private $attributeSetRepository;
public function __construct(AttributeSetRepositoryInterface $attributeSetRepository)
{
$this->attributeSetRepository = $attributeSetRepository;
}
/**
* Delete attribute set by ID
*
* @param int $attributeSetId
* @return bool
* @throws LocalizedException
*/
public function deleteAttributeSetById($attributeSetId)
{
try {
return $this->attributeSetRepository->deleteById($attributeSetId);
} catch (\Exception $e) {
throw new LocalizedException(__(\'Error deleting attribute set: %1\', $e->getMessage()));
}
}
}
Usage Example
To use this function, instantiate the AttributeSetManager
class and call the deleteAttributeSetById
method with the desired attribute set ID:
<?php
$attributeSetId = 5; // Replace with your attribute set ID
// Assuming $attributeSetRepository is already initialized
$attributeSetManager = new \Vendor\Module\Model\AttributeSetManager($attributeSetRepository);
$result = $attributeSetManager->deleteAttributeSetById($attributeSetId);
if ($result) {
echo "Attribute set deleted successfully.";
} else {
echo "Failed to delete attribute set.";
}
This method returns true if the deletion is successful; otherwise, it throws an exception.
Important Considerations
- Data Integrity: Ensure that the attribute set you're deleting isn't assigned to any products. Deleting an attribute set associated with products can lead to data inconsistencies.
- Error Handling: Implement proper error handling to manage exceptions that may occur during the deletion process.
By following this approach, you can effectively manage and delete attribute sets in your Magento 2 store.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Delete an Attribute Set by ID in Magento 2?
You can remove an attribute set in Magento 2 using its ID. The deleteById($attributeSetId)
method in the AttributeSetRepositoryInterface
allows you to delete it programmatically.
What PHP Code Can I Use to Delete an Attribute Set?
Use the following PHP code to remove an attribute set:
$attributeSetId = 5;
$result = $this->deleteAttributeSetById($attributeSetId);
if ($result) {
echo "Attribute set deleted successfully.";
} else {
echo "Failed to delete attribute set.";
}
Which Magento Interface Is Used to Delete an Attribute Set?
The Magento\Catalog\Api\AttributeSetRepositoryInterface
is responsible for managing and deleting attribute sets in Magento 2.
How Can I Handle Errors While Deleting an Attribute Set?
Wrap the deletion process inside a try-catch block to handle exceptions:
try {
$result = $this->attributeSetRepository->deleteById($attributeSetId);
} catch (Exception $e) {
$this->logger->error($e->getMessage());
}
Where Are Attribute Sets Stored in Magento 2?
Attribute set data is stored in the eav_attribute_set
table in the Magento database.
What Happens If the Given Attribute Set ID Does Not Exist?
If the attribute set ID is invalid or does not exist, the deleteById()
method will throw an exception. Ensure the ID is correct before executing the deletion.