Setting or Updating Special Prices in Magento 2
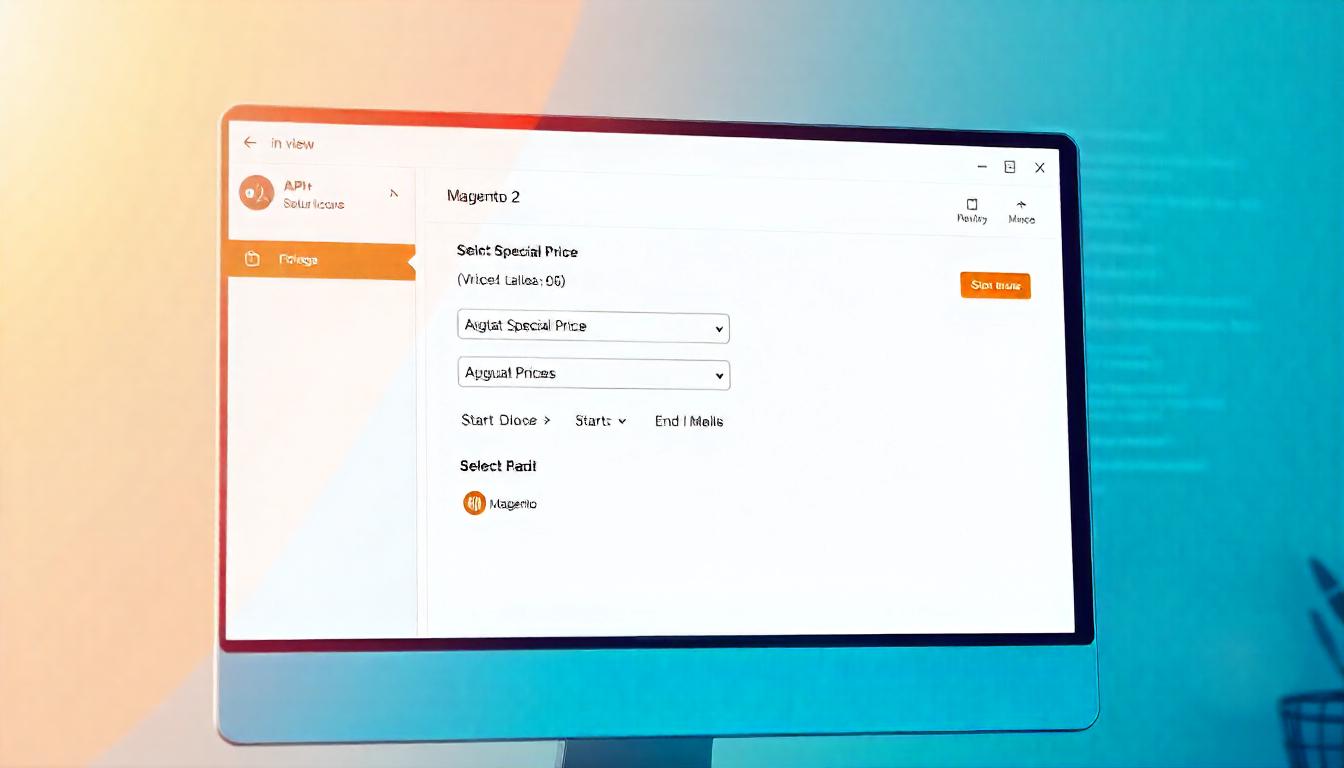
Setting or Updating Special Prices in Magento 2
In Magento 2, you can set or update special prices to offer discounts for a limited time. This can be done programmatically using the SpecialPriceInterface, which allows you to define pricing rules based on SKU, store ID, and date range.
Table Of Content
Setting or Updating Special Prices in Magento 2
To set or update a product's special price in Magento 2, you can use the SpecialPriceInterface
. This interface allows you to define special pricing for products programmatically.
Implementing the Deletion Method
Method Definition:
public function update(array $prices);
Parameters:
Key | Type | Description |
---|---|---|
store_id |
int | The ID of the store where the special price applies. |
sku |
string | The SKU of the product. |
price |
float | The special price value. |
price_from |
string | The start date for the special price in 'Y-m-d H:i:s' format (UTC). |
price_to |
string | The end date for the special price in 'Y-m-d H:i:s' format (UTC). |
Example Code to Update Special Price
<?php
namespace Vendor\Module\Model;
use Exception;
use Magento\Catalog\Api\SpecialPriceInterface;
use Magento\Catalog\Api\Data\SpecialPriceInterfaceFactory;
class UpdateSpecialPrice
{
private $specialPrice;
private $specialPriceFactory;
public function __construct(
SpecialPriceInterface $specialPrice,
SpecialPriceInterfaceFactory $specialPriceFactory
) {
$this->specialPrice = $specialPrice;
$this->specialPriceFactory = $specialPriceFactory;
}
public function applySpecialPrice()
{
$sku = 'example-sku';
$price = 10.99; // Special Price
try {
$priceFrom = '2025-02-15'; // Start date
$priceTo = '2025-03-15'; // End date
$prices[] = $this->specialPriceFactory->create()
->setSku($sku)
->setStoreId(0)
->setPrice($price)
->setPriceFrom($priceFrom)
->setPriceTo($priceTo);
$this->specialPrice->update($prices);
} catch (Exception $exception) {
throw new Exception($exception->getMessage());
}
}
}
In this example, replace 'example-sku' with your product's SKU. The price_from and price_to fields are optional; if omitted, the special price will apply immediately and indefinitely.
For Magento Commerce users, special prices can also be managed through the Admin panel under Scheduled Changes.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Update a Product's Special Price in Magento 2?
You can update a special price in Magento 2 using the SpecialPriceInterface
. The update(array $prices)
method allows you to set or modify special prices programmatically.
What PHP Code Can I Use to Update a Special Price?
Use the following PHP code snippet to update a special price:
$prices[] = $this->specialPriceFactory->create()
->setSku('example-sku')
->setStoreId(0)
->setPrice(10.99)
->setPriceFrom('2025-02-15')
->setPriceTo('2025-03-15');
$this->specialPrice->update($prices);
Which Magento Interface Is Used to Update Special Prices?
The Magento\Catalog\Api\SpecialPriceInterface
manages and updates special prices in Magento 2.
How Can I Handle Errors While Updating a Special Price?
Wrap the price update process inside a try-catch block to handle exceptions:
try {
$this->specialPrice->update($prices);
} catch (Exception $e) {
$this->logger->error($e->getMessage());
}
Where Are Special Prices Stored in Magento 2?
Special price data is stored in the catalog_product_entity
table in the Magento database.
What Happens If the Given SKU Does Not Exist?
If the SKU is invalid or does not exist, the update()
method will throw an exception. Ensure the SKU is correct before executing the update.