Retrieving Store Code from Cookies in Magento 2
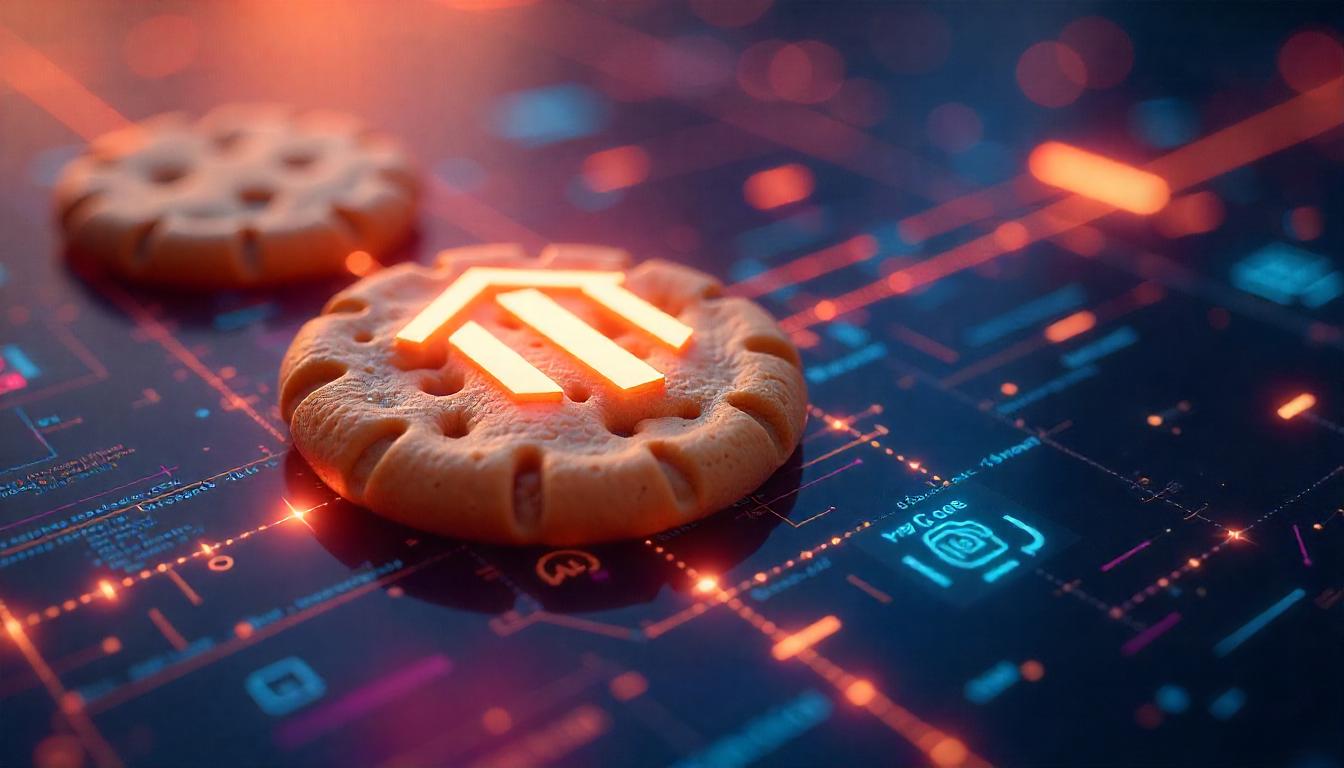
Retrieving Store Code from Cookies in Magento 2
In Magento 2, when you have multiple store views, switching between them sets a cookie named store with the value of the current store's code. This allows you to identify the active store programmatically.
Table Of Content
Retrieving Store Code from Cookies in Magento 2
Accessing the Store Code from the Cookie
To retrieve the store code from this cookie, you can utilize Magento's StoreCookieManagerInterface. Here's how:
declare(strict_types=1);
namespace YourNamespace\YourModule\Model;
use Magento\Store\Api\StoreCookieManagerInterface;
class StoreInfo
{
private StoreCookieManagerInterface $storeCookieManager;
public function __construct(StoreCookieManagerInterface $storeCookieManager)
{
$this->storeCookieManager = $storeCookieManager;
}
public function getStoreCode(): string
{
return $this->storeCookieManager->getStoreCodeFromCookie();
}
}
Explanation:
- Constructor Injection: The StoreCookieManagerInterface is injected into the class, allowing access to store cookie functionalities.
- getStoreCode Method: This method retrieves the store code directly from the store cookie using the getStoreCodeFromCookie() function.
Important Considerations:
- Cookie Availability: The store cookie is set when a user switches store views. If no switch has occurred, the cookie may not be present.
- Default Store View: If the default store view is active, the store cookie might not be set. In such cases, you can retrieve the store code
programmatically using the StoreManagerInterface:
declare(strict_types=1);
namespace YourNamespace\YourModule\Model;
use Magento\Store\Api\StoreCookieManagerInterface;
class StoreInfo
{
private StoreManagerInterface $storeManager;
public function __construct(StoreManagerInterface $storeManager)
{
$this->storeManager = $storeManager;
}
public function getStoreCode(): string
{
return $this->storeManager->getStore()->getCode();
}
}
This approach ensures that you can reliably obtain the store code, whether the store cookie is set or not.
Additional Resources:
For more detailed information on retrieving store information in Magento 2, refer to this guide:
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the `store` Cookie in Magento 2?
The `store` cookie in Magento 2 stores the current store code when a user switches between stores. It helps Magento manage multi-store setups by associating the user session with the selected store.
Why Is the `store` Cookie Important?
The `store` cookie is essential for Magento 2's multi-store functionality. It ensures that the correct store view is loaded and the user’s session is aligned with the appropriate store code, providing a seamless experience across different stores.
What Does the `store` Cookie Contain?
The `store` cookie contains the store code that corresponds to the current active store. For example, if the store code is "default", the cookie will store `store=default`.
How Do You Retrieve the Store Code from the Cookie?
You can retrieve the store code from the `store` cookie using Magento 2’s `StoreCookieManagerInterface`. This interface provides a method `getStoreCodeFromCookie()` that returns the store code stored in the cookie.
How Can You Use the `store` Cookie in Custom Code?
To use the `store` cookie, inject the `StoreCookieManagerInterface` into your custom class and call the `getStoreCodeFromCookie()` method. This will return the store code, allowing you to work with store-specific logic in your Magento 2 application.
What Happens If the `store` Cookie Is Not Set?
If the `store` cookie is not set, Magento will default to the store code of the primary store. You can also retrieve the store code directly from the `StoreManagerInterface` in such cases.
How Can You Ensure Correct Store Code Retrieval?
Ensure that the user switches stores for the cookie to be set. If the cookie is not available, fall back to using the `StoreManagerInterface` to retrieve the store code programmatically.
Can You Modify the `store` Cookie?
While it’s possible to modify the `store` cookie, doing so is not recommended as it can cause issues with store switching functionality. It’s better to rely on Magento’s built-in methods for store code management.
How Can You Debug Issues with the `store` Cookie?
To debug the `store` cookie, check if it is set in the browser’s developer tools. If it’s not present, verify if store switching is properly working on the frontend, or fall back to server-side logic using the `StoreManagerInterface`.
Can You Automate Store Code Retrieval Using the `store` Cookie?
Yes, you can automate the process by using the Magento 2 API or creating custom scripts that automatically retrieve the store code from the cookie for efficient management of store-specific data.