Best Practices for Using the insertFromSelect Query in Magento 2 Databases
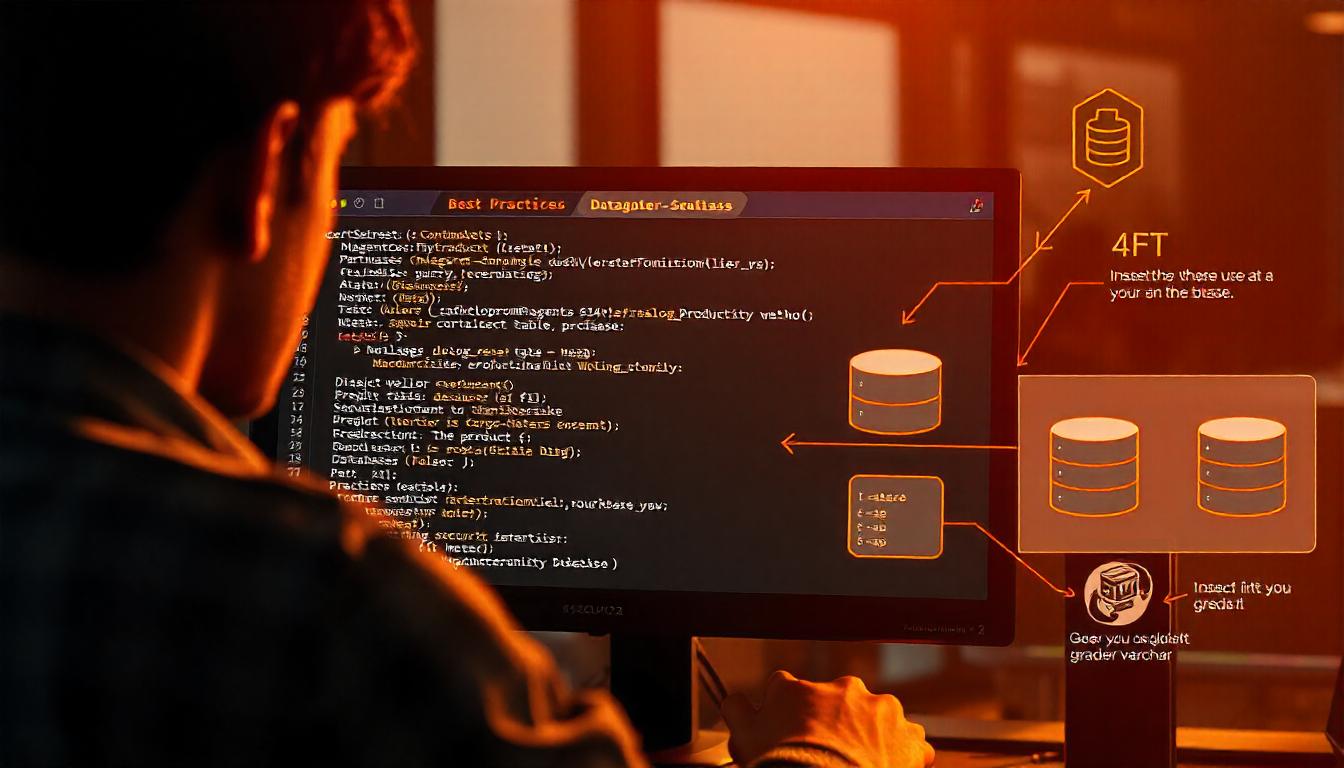
Best Practices for Using the insertFromSelect Query in Magento 2 Databases
IThe insertFromSelect query in Magento 2 is a powerful method for efficiently inserting data from one table into another. This guide explores its best practices, ensuring optimal performance, clean code, and database integrity. Learn how to leverage this technique effectively in your Magento 2 projects while avoiding common pitfalls.
Table Of Content
Implementing insertFromSelect in Magento 2
To insert data into a Magento 2 database using the insertFromSelect() method, follow these steps:
Set Up the Class:
Begin by declaring strict types and defining your namespace. Import the necessary Magento classes:
Here's a concise PHP code example demonstrating this process:
<?php
declare(strict_types=1);
namespace Vendor\Module\Index;
use Magento\Framework\App\ResourceConnection;
use Magento\Framework\DB\Adapter\AdapterInterface;
Define the Class and Constructor:
Create the InsertFromSelect class and inject the ResourceConnection dependency:
class InsertFromSelect
}
private const CATALOG_PRODUCT_ENTITY_VARCHAR = 'catalog_product_entity_varchar';
public function __construct(
private readonly ResourceConnection $resourceConnection
) {
}
}
Execute the Insert from Select:
Within the execute method, establish a database connection, define table names, and set attribute IDs:
public function execute()
}
$connection = $this->resourceConnection->getConnection();
$catalogVarcharTable = $connection->getTableName(self::CATALOG_PRODUCT_ENTITY_VARCHAR);
$smallImageAttributeId = 88;
$imageAttributeId = 87;
}
Construct the Select Query:
Build a select query to retrieve data for insertion:
$select = $connection->select()
->from(
$catalogVarcharTable,
[new \Zend_Db_Expr($imageAttributeId), 'store_id', 'value', 'row_id']
)
->where("value != 'no_selection'")
->where('attribute_id = ?', $smallImageAttributeId);
Perform the Insert Operation:
Use the insertFromSelect method to insert data, specifying the desired behavior with the fourth parameter:
$insertFromSelectQuery = $connection->insertFromSelect(
$select,
$catalogVarcharTable,
['attribute_id', 'store_id', 'value', 'row_id'],
AdapterInterface::INSERT_IGNORE
);
$connection->query($insertFromSelectQuery);
By following these steps, you can effectively use the insertFromSelect() method in Magento 2 to insert data into your database while adhering to best practices.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the `insertFromSelect` Query in Magento 2?
The `insertFromSelect` query allows you to insert data into a database table by selecting data from another table. It's an efficient way to copy or manipulate data within Magento 2's database.
When Should You Use the `insertFromSelect` Query?
Use this query when you need to insert large volumes of data into a table from another table. It’s ideal for bulk operations or when working with data transformation during migrations.
How Does the `insertFromSelect` Query Work in Magento 2?
The query selects data from a source table and inserts it into a target table. The `insertFromSelect()` method is used to perform the operation, allowing you to define specific values and behavior (e.g., `INSERT_IGNORE`).
What Are the Key Benefits of Using `insertFromSelect`?
This method helps avoid the need for looping through individual records, improving performance for bulk data operations. It's an optimized approach to data insertion in Magento 2.
What Are the Best Practices for Using `insertFromSelect`?
Follow best practices by validating data before inserting, handling errors gracefully, and using the correct database table names. Additionally, use appropriate constants like `INSERT_IGNORE` or `INSERT_ON_DUPLICATE` based on your needs.
How Do You Handle Errors When Using `insertFromSelect`?
Ensure proper exception handling to manage database connection issues, invalid data, or duplicate entries. Always log errors for easier troubleshooting and maintain system reliability.
What Should Be Considered When Setting Up the `insertFromSelect` Query?
Make sure you are selecting only the necessary data fields, and avoid inserting unnecessary columns into the target table. Also, validate that your select query returns the correct results before executing the insert operation.
Can You Use `insertFromSelect` for Data Migrations?
Yes, `insertFromSelect` is commonly used for data migration tasks, especially when transferring data between Magento 2 tables or from external systems.
How Do You Ensure Data Integrity When Using `insertFromSelect`?
To ensure data integrity, always use valid select queries, validate the data before insertion, and avoid inserting duplicate or incomplete records. Implement appropriate checks and constraints to maintain consistency.
What Are Common Mistakes to Avoid When Using `insertFromSelect`?
Common mistakes include failing to validate data, missing error handling, and incorrectly specifying column mappings. Always test the query in a safe environment before running it in production.