How to Retrieve Customer ID from API Header Bearer Token in Magento 2
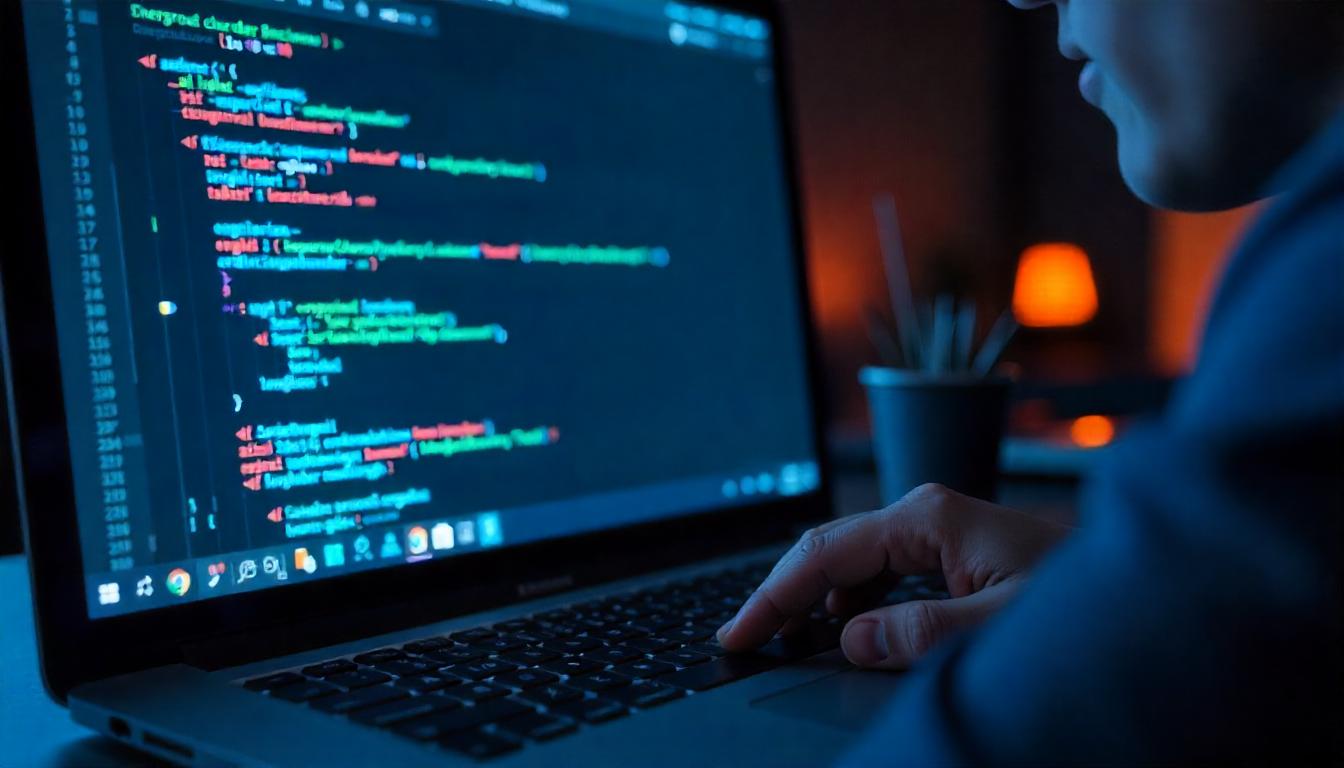
How to Retrieve Customer ID from API Header Bearer Token in Magento 2
In Magento 2, extracting the customer ID from an API header bearer token is crucial for secure and authenticated operations. Here's how you can do it step-by-step:
Table Of Content
How to Retrieve Customer ID from API Header
To retrieve a customer ID from a Bearer token in Magento 2's API, follow these steps:
- Extract the Bearer Token: Access the 'Authorization' header from the API request. Split its value to isolate the token.
- Read and Validate the Token: Utilize Magento's UserTokenReaderInterface to interpret the token. Then, apply UserTokenValidatorInterface to confirm its validity.
- Obtain the Customer ID: After validation, fetch the user context from the token and retrieve the associated customer ID.
Here's a concise PHP code example demonstrating this process:
<?php
declare(strict_types=1);
namespace Vendor\Module\Webapi;
use Magento\Framework\Exception\AuthorizationException;
use Magento\Integration\Api\Exception\UserTokenException;
use Magento\Integration\Api\UserTokenReaderInterface;
use Magento\Integration\Api\UserTokenValidatorInterface;
use Magento\Framework\Webapi\Request;
class TokenCustomerId
{
private Request $request;
private UserTokenReaderInterface $userTokenReader;
private UserTokenValidatorInterface $userTokenValidator;
public function __construct(
Request $request,
UserTokenReaderInterface $userTokenReader,
UserTokenValidatorInterface $userTokenValidator
) {
$this->request = $request;
$this->userTokenReader = $userTokenReader;
$this->userTokenValidator = $userTokenValidator;
}
/**
* Retrieve customer ID from the authorization token.
*
* @return int|null
* @throws AuthorizationException
*/
public function getCustomerIdByBearerToken(): ?int
{
$authorizationHeader = $this->request->getHeader('Authorization');
if (!$authorizationHeader) {
return null;
}
$bearerToken = $headerParts[1];
try {
$token = $this->userTokenReader->read($bearerToken);
$this->userTokenValidator->validate($token);
} catch (UserTokenException | AuthorizationException $e) {
throw new AuthorizationException(__($e->getMessage()));
}
return (int) $token->getUserContext()->getUserId();
}
}
Key Points:
- Authorization Header: Ensure the 'Authorization' header follows the 'Bearer {token}' format.
- Error Handling: Implement appropriate exception handling to manage invalid or expired tokens.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Purpose of Retrieving a Customer ID from a Bearer Token?
Retrieving a customer ID from a Bearer token allows developers to identify the customer making an API request. This is crucial for personalizing responses and ensuring secure access control.
What Prerequisites Are Needed for Fetching a Customer ID?
Ensure you have access to Magento 2’s `Request`, `UserTokenReaderInterface`, and `UserTokenValidatorInterface` classes. Proper API headers, including the Bearer token, must be passed during the request.
How Do You Extract a Bearer Token from the Authorization Header?
The Bearer token can be extracted by accessing the 'Authorization' header in the request. Split the header's value by spaces to isolate the token from the 'Bearer' keyword.
What Are the Key Steps to Validate a Bearer Token in Magento 2?
Use `UserTokenReaderInterface` to read the token and `UserTokenValidatorInterface` to confirm its validity. Ensure proper exception handling to manage invalid or expired tokens.
How Do You Retrieve the Customer ID from a Valid Token?
After validating the token, use the user context associated with the token to fetch the customer ID. This ID identifies the specific customer making the API request.
What Common Issues Arise When Fetching Customer IDs?
Common issues include missing or malformed Authorization headers, invalid tokens, and expired user sessions. Proper error handling and debugging can resolve these problems.
Can This Process Be Used for Admin Tokens?
Yes, a similar approach can be used for admin tokens. However, ensure the token’s context matches the required user type (admin or customer) for accurate results.
Why Is Error Handling Important in Token Validation?
Error handling ensures that invalid or expired tokens don’t compromise system security. It also helps provide clear feedback for debugging and troubleshooting.
What Best Practices Should Be Followed When Using Bearer Tokens?
Always use secure HTTPS connections for API requests, limit token expiration time, and implement proper logging for token access and errors. Follow Magento’s coding standards for security.
How Can This Code Be Extended for Additional Security?
Integrate IP whitelisting, rate limiting, and logging mechanisms. Regularly update Magento and its components to incorporate the latest security patches.