How to Add Product Attributes Programmatically in Magento 2
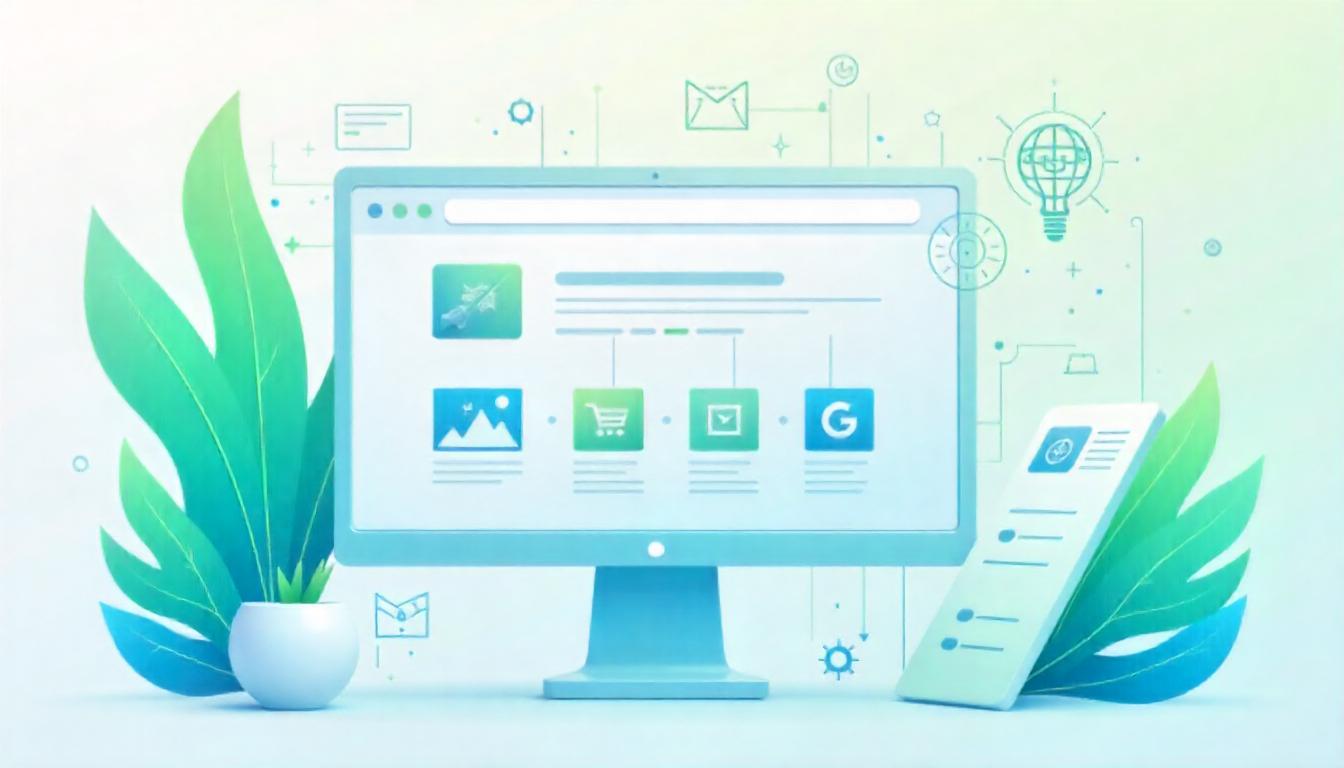
How to Add Product Attributes Programmatically in Magento 2
Adding product attributes in Magento 2 is essential for improving customer experience and increasing conversions. Attributes such as size, color, fabric type, and weight help customers make quick decisions by providing all the relevant product information. For stores with large catalogs, adding attributes manually is inefficient. By adding them programmatically, you save time and streamline the process.
In this guide, we’ll define product attributes, explain their benefits, and provide step-by-step instructions for adding them programmatically in Magento 2.
Table Of Content
What Are Product Attributes?
Product attributes define specific characteristics of a product. These can be simple fields like text boxes or dropdowns that display details such as color, size, material, or weight.
Attribute Type | Examples | Use Case |
---|---|---|
Text Field | Weight, Dimensions | Specific measurements like "1kg" |
Dropdown | Size, Color | Options like "Red," "Blue," "Small" |
Radio Buttons | Material | Choices like "Cotton," "Polyester" |
Price | Special Pricing, Discounts | Custom pricing attributes |
Boolean (Yes/No) | Availability, Warranty | Options like "Yes," "No" |
Attributes play a crucial role in product filtering, layered navigation, and search functionality. By clearly displaying these attributes, customers can quickly determine whether the product meets their needs.
Why Add Product Attributes Programmatically?
Adding product attributes manually in the Magento admin panel is time-consuming and error-prone, especially for stores with extensive catalogs or when importing data from external sources. Programmatically creating product attributes is faster, more accurate, and more scalable.
Manual Method | Programmatic Method |
---|---|
Suitable for small catalogs | Best for large catalogs |
Time-intensive for bulk attributes | Quick bulk attribute creation |
Prone to human errors | Reduces risk of errors |
Requires repetitive steps | Automates the entire process |
Limited customization | Full control over attribute properties |
For example, if you're importing thousands of products from a supplier with custom attributes like "Fabric Type," "Season," or "Brand," adding these attributes programmatically is far more efficient.
Prerequisites
Before proceeding, ensure the following:
- Custom Module: A custom module should already be set up. If not, create one under
app/code/Company/Mymodule.
- Terminal Access: SSH access to run Magento commands.
- Basic PHP Knowledge: Familiarity with PHP and Magento's file structure.
Step-by-Step: Add Product Attributes Programmatically
Step 1: Create the Setup File - InstallData.php
In Magento 2, the InstallData
script is executed during the module installation to create or update data, like adding product attributes.
File Path:app/code/Company/Mymodule/Setup/InstallData.php
InstallData File to Add Custom Product Attribute
<?php
namespace Company\Mymodule\Setup;
use Magento\Eav\Setup\EavSetup;
use Magento\Eav\Setup\EavSetupFactory;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class InstallData implements InstallDataInterface
{
private $eavSetupFactory;
/**
* Constructor to inject EavSetupFactory dependency
*
* @param EavSetupFactory $eavSetupFactory
*/
public function __construct(EavSetupFactory $eavSetupFactory)
{
$this->eavSetupFactory = $eavSetupFactory;
}
/**
* Install custom product attribute
*
* @param ModuleDataSetupInterface $setup
* @param ModuleContextInterface $context
*/
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$setup->startSetup();
/** @var EavSetup $eavSetup */
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
// Add product attribute code here
$setup->endSetup();
}
}
Explanation of Code:
- Namespace: Follows Magento's PSR-4 standards for module files.
- InstallDataInterface: Defines the install() method to insert data into the database during module installation.
- EavSetupFactory: Creates and manages product attributes programmatically.
startSetup()
andendSetup()
: Used to start and end database transactions safely.
Step 2: Define the install()
Method
The install()
method contains the actual logic for adding the product attribute. Here, we'll use the addAttribute()
method provided by the EavSetup
class.
Step 3: Add Product Attribute
Adding a Text Field Attribute:
Here’s how to add a custom text field attribute (sample_attribute
) programmatically.
InstallData File to Add Custom Product Attribute
<?php
namespace Company\Mymodule\Setup;
use Magento\Eav\Setup\EavSetup;
use Magento\Eav\Setup\EavSetupFactory;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class InstallData implements InstallDataInterface
{
private $eavSetupFactory;
public function __construct(EavSetupFactory $eavSetupFactory)
{
$this->eavSetupFactory = $eavSetupFactory;
}
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$setup->startSetup();
/** @var EavSetup $eavSetup */
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
$eavSetup->addAttribute(
\Magento\Catalog\Model\Product::ENTITY,
'sample_attribute',
[
'type' => 'varchar',
'backend' => '',
'frontend' => '',
'label' => 'Sample Attribute',
'input' => 'text',
'class' => '',
'source' => '',
'global' => \Magento\Eav\Model\Entity\Attribute\ScopedAttributeInterface::SCOPE_GLOBAL,
'visible' => true,
'required' => false,
'user_defined' => true,
'default' => '',
'searchable' => true,
'filterable' => false,
'comparable' => false,
'visible_on_front' => true,
'used_in_product_listing' => true,
'unique' => false,
'apply_to' => '',
'sort_order' => 50
]
);
$setup->endSetup();
}
}
Code Breakdown
Parameter | Description | Example Value |
---|---|---|
'type' | Database column type for the attribute. | 'varchar', 'int' |
'label' | Name of the attribute shown in the admin. | 'Sample Attribute' |
'input' | Input type (text, dropdown, multiselect). | 'text' |
'required' | Marks the field as required (true/false). | false |
'user_defined' | Marks it as a custom attribute. | true |
'global' | Scope of the attribute (global, website, store view). | SCOPE_GLOBAL |
'visible_on_front' | Show the attribute on the frontend product page. | true |
'used_in_product_listing' | Include the attribute in product listings. | true |
'sort_order' | Determines the display position in the admin panel. | 50 |
Step 4: Run the Upgrade Script
Once your code is ready, run the following commands in your terminal to apply the changes:
Magento CLI Commands
php bin/magento setup:upgrade
php bin/magento setup:static-content:deploy -f
php bin/magento cache:flush
Step 5: Verify the Attribute
In Admin Panel
- Go to Stores > Attributes > Product.
- Search for the attribute by its code (sample_attribute).
- Confirm the field settings, input type, and visibility.
In Product Edit Page
- Go to Catalog > Products.
- Edit any product and scroll to see the "Sample Attribute" field.
On the Frontend
- View the product page.
- Check if the attribute value appears on the product view page.
Step 6: Customize Input Types
To add other input types like dropdown, yes/no, or multiselect, customize the input and add relevant options:
Dropdown Example:
Attribute with Select Input and Options
'input' => 'select',
'source' => '',
'option' => ['values' => ['Option 1', 'Option 2', 'Option 3']],
Yes/No Field:
Attribute with Boolean Input and Default Value
'input' => 'boolean',
'default' => '1', // Default value: Yes
Multiselect Example:
Attribute with Multiselect Input and Options
'input' => 'multiselect',
'option' => ['values' => ['Value 1', 'Value 2', 'Value 3']],
Final Notes and Best Practices
- Use EAV Attributes: EAV attributes are essential for flexible, scalable product data.
- Test on Development Environment: Always test the setup scripts in a staging or dev environment.
- Clean Cache: Ensure caches are flushed after running the upgrade script.
- Re-indexing: Run php bin/magento indexer:reindex if the attribute doesn't appear immediately.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Adding custom product attributes programmatically in Magento 2 is a powerful way to enhance your product catalog and meet specific business needs. By following the steps outlined above, you can easily create custom attributes with various input types, such as text fields, dropdowns, and multiselect options. This process allows for greater flexibility, scalability, and automation, ensuring that your product data is managed efficiently and consistently across environments.
With a clear understanding of Magento's EAV model and best practices, you can extend the functionality of your store while maintaining a clean, maintainable codebase. Whether you're working on a small custom feature or integrating more complex data structures, programmatically adding product attributes is an essential tool for Magento developers.
Remember to thoroughly test your changes, keep your caches and indexes updated, and follow Magento's standards to ensure smooth implementation. By leveraging these techniques, you’ll be able to offer a more customizable shopping experience, improving both backend management and frontend customer interactions.
Feel free to reach out if you need assistance with more advanced scenarios or further optimization of your Magento store. Happy coding!
FAQs
What are product attributes in Magento 2?
Product attributes in Magento 2 define characteristics of a product, such as size, color, material, and more. These attributes help customers filter and compare products more effectively on your store.
Why should I add product attributes programmatically in Magento 2?
Adding product attributes programmatically in Magento 2 is efficient when you need to create multiple attributes at once or if you're importing attributes from external sources. This method saves time compared to using the admin panel.
How do I create a product attribute in Magento 2 programmatically?
To create a product attribute programmatically, you need to use the EavSetup class and define the attribute's properties, such as label, input type, visibility, and more in a custom InstallData script within your module.
What file do I need to create to add product attributes programmatically in Magento 2?
You need to create an InstallData.php file within your module's Setup directory. This file will contain the logic to add the product attribute using the EavSetup class.
What is the EavSetup class in Magento 2?
The EavSetup class in Magento 2 is used to manage attributes for entities like products, customers, and categories. It provides a convenient API for adding, updating, and deleting attributes programmatically.
Can I add custom product attributes with dropdown options in Magento 2?
Yes, you can add custom product attributes with dropdown options by defining the attribute as a 'select' input type and then specifying the available options in the 'source' field.
What is the purpose of the 'apply_to' field when adding a product attribute?
The 'apply_to' field is used to specify which product types the attribute should apply to, such as simple products, configurable products, or virtual products. This ensures that the attribute is only available for relevant products.
Do I need to run any commands after adding a product attribute programmatically?
Yes, after adding a product attribute programmatically, you need to run the `php bin/magento setup:upgrade` and `php bin/magento setup:static-content:deploy` commands to apply the changes and update the database.
Can I create multiple product attributes at once in Magento 2?
Yes, you can create multiple product attributes at once by using the same InstallData.php file and adding additional `addAttribute` calls within the `install()` method to define each attribute.
What is the 'global' field used for when adding a product attribute in Magento 2?
The 'global' field determines the scope of the attribute. If set to `SCOPE_GLOBAL`, the attribute will be available for all store views. If set to `SCOPE_WEBSITE` or `SCOPE_STORE`, the attribute will be limited to the respective scope.