Retrieving Order Discount Amounts in Magento 2
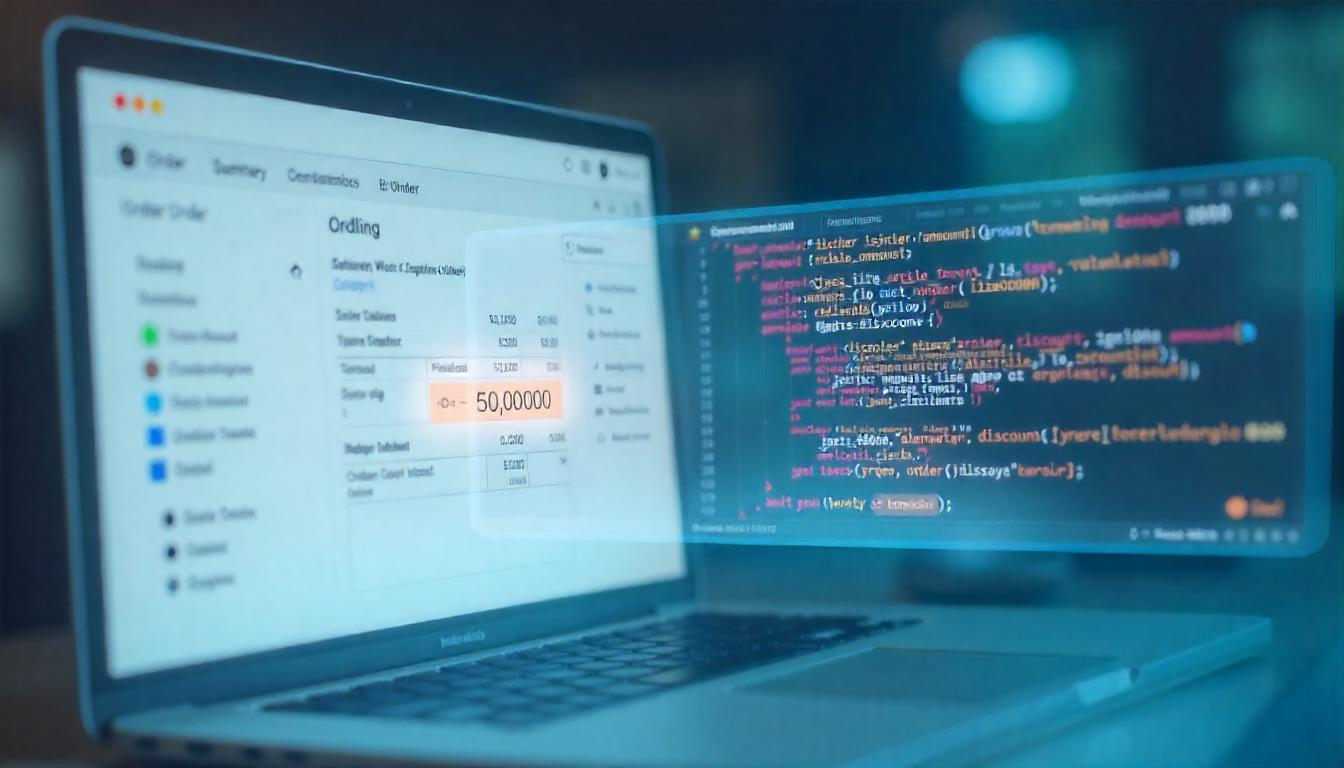
Retrieving Order Discount Amounts in Magento 2
Retrieving the discount amount for an order in Magento 2 is essential for tracking applied promotions and calculating final totals. Magento stores discount values in the sales_order table under the discount_amount field, usually as a negative number.
Table Of Content
Retrieving Order Discount Amounts in Magento 2
In Magento 2, understanding how to access the discount amount applied to an order is essential for accurate order management. The discount_amount
field in the sales_order
table records this value, typically as a negative number. For instance, a $50 discount appears as -50.0000 in the database.
Accessing the Discount Amount Using Order Increment ID
To fetch the discount amount based on an order's increment ID, you can utilize the following code snippet:
Define the Event in events.xml
Create the events.xml
file in your module's etc/frontend
directory:
<?php
namespace YourNamespace\Order\Block;
use Exception;
use Psr\Log\LoggerInterface;
use Magento\Framework\View\Element\Template;
use Magento\Framework\Api\SearchCriteriaBuilder;
use Magento\Sales\Api\OrderRepositoryInterface;
use Magento\Framework\View\Element\Template\Context;
class OrderDiscount extends Template
{
protected $searchCriteriaBuilder;
private $orderRepository;
private $logger;
public function __construct(
Context $context,
SearchCriteriaBuilder $searchCriteriaBuilder,
OrderRepositoryInterface $orderRepository,
LoggerInterface $logger,
array $data = []
) {
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
$this->orderRepository = $orderRepository;
$this->logger = $logger;
parent::__construct($context, $data);
}
public function getDiscountAmountOrder()
{
$orderDiscountAmount = null;
try {
$incrementId = 'YOUR_ORDER_INCREMENT_ID';
$searchCriteria = $this->searchCriteriaBuilder
->addFilter('increment_id', $incrementId)
->create();
$orderData = $this->orderRepository->getList($searchCriteria)->getItems();
foreach ($orderData as $order) {
if ($order->getDiscountAmount()) {
$orderDiscountAmount = abs($order->getDiscountAmount());
}
}
} catch (Exception $exception) {
$this->logger->error($exception->getMessage());
}
return $orderDiscountAmount;
}
}
Replace 'YOUR_ORDER_INCREMENT_ID
' with the actual increment ID of the order. This code retrieves the discount amount and converts it to a positive value using the abs() function.
Accessing the Discount Amount Using Order Entity ID
If you have the order's entity ID, retrieving the discount amount becomes more straightforward:
<?php
namespace YourNamespace\Order\Block;
use Exception;
use Psr\Log\LoggerInterface;
use Magento\Framework\View\Element\Template;
use Magento\Sales\Api\OrderRepositoryInterface;
use Magento\Framework\View\Element\Template\Context;
class OrderDiscount extends Template
{
private $orderRepository;
private $logger;
public function __construct(
Context $context,
OrderRepositoryInterface $orderRepository,
LoggerInterface $logger,
array $data = []
) {
$this->orderRepository = $orderRepository;
$this->logger = $logger;
parent::__construct($context, $data);
}
public function getDiscountAmountById($orderId)
{
$orderDiscountAmount = null;
try {
$order = $this->orderRepository->get($orderId);
if ($order->getDiscountAmount()) {
$orderDiscountAmount = abs($order->getDiscountAmount());
}
} catch (Exception $exception) {
$this->logger->error($exception->getMessage());
}
return $orderDiscountAmount;
}
}
In this example, replace '$orderId' with the specific entity ID of the order. This method directly fetches the order and retrieves the discount amount.
Understanding Discount Amount Storage
Magento 2 stores discount amounts as negative values in the sales_order table. This design choice reflects the discount's subtractive effect on the order total. When retrieving and displaying this data, it's common practice to convert these values to positive numbers for clarity.
Key Database Tables for Sales Orders
Magento 2 utilizes several tables to manage sales order data. Here's a brief overview:
Table Name | Description |
---|---|
sales_order |
Stores main order records, including total amounts and discounts. |
sales_order_item |
Contains individual items within each order. |
sales_order_grid |
Facilitates order management in the admin panel. |
sales_order_address |
Stores billing and shipping addresses associated with orders. |
sales_order_payment |
Details payment information for orders. |
sales_order_status |
Tracks the status history of orders. |
Understanding these tables can aid in more advanced order management and customization within Magento 2.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve the Discount Amount for an Order in Magento 2?
You can retrieve the discount amount by accessing the discount_amount
field in the sales_order
table. This field stores the applied discount as a negative value.
How Do I Get the Discount Amount Using an Order Increment ID?
Use the following code snippet to retrieve the discount amount based on an order's increment ID:
$searchCriteria = $this->searchCriteriaBuilder
->addFilter('increment_id', 'YOUR_ORDER_INCREMENT_ID')
->create();
$orderData = $this->orderRepository->getList($searchCriteria)->getItems();
foreach ($orderData as $order) {
$discountAmount = abs($order->getDiscountAmount());
}
How Do I Retrieve the Discount Amount Using an Order Entity ID?
If you have the order's entity ID, you can directly fetch the discount amount using this code:
$order = $this->orderRepository->get($orderId);
$discountAmount = abs($order->getDiscountAmount());
Why is the Discount Amount Stored as a Negative Value?
Magento 2 stores discounts as negative values in the sales_order
table to reflect the deduction from the order total. When displaying this value, converting it to a positive number improves readability.
Which Database Tables Store Sales Order Discount Information?
Magento 2 stores sales order discount details in several tables, including:
sales_order
- Stores the main order data, including discount amounts.sales_order_item
- Contains individual order items and item-level discounts.sales_order_grid
- Facilitates order management in the admin panel.
Do I Need to Clear the Cache After Modifying Order Discounts?
Yes, after making changes, run the following command to clear the cache:
php bin/magento cache:clean
Where Can I Find More Information on Order Events and Discounts?
You can check Magento's official documentation for more details on order events, discounts, and customization options.