Modifying Product Final Price in Magento 2 Using the catalog_product_get_final_price Event
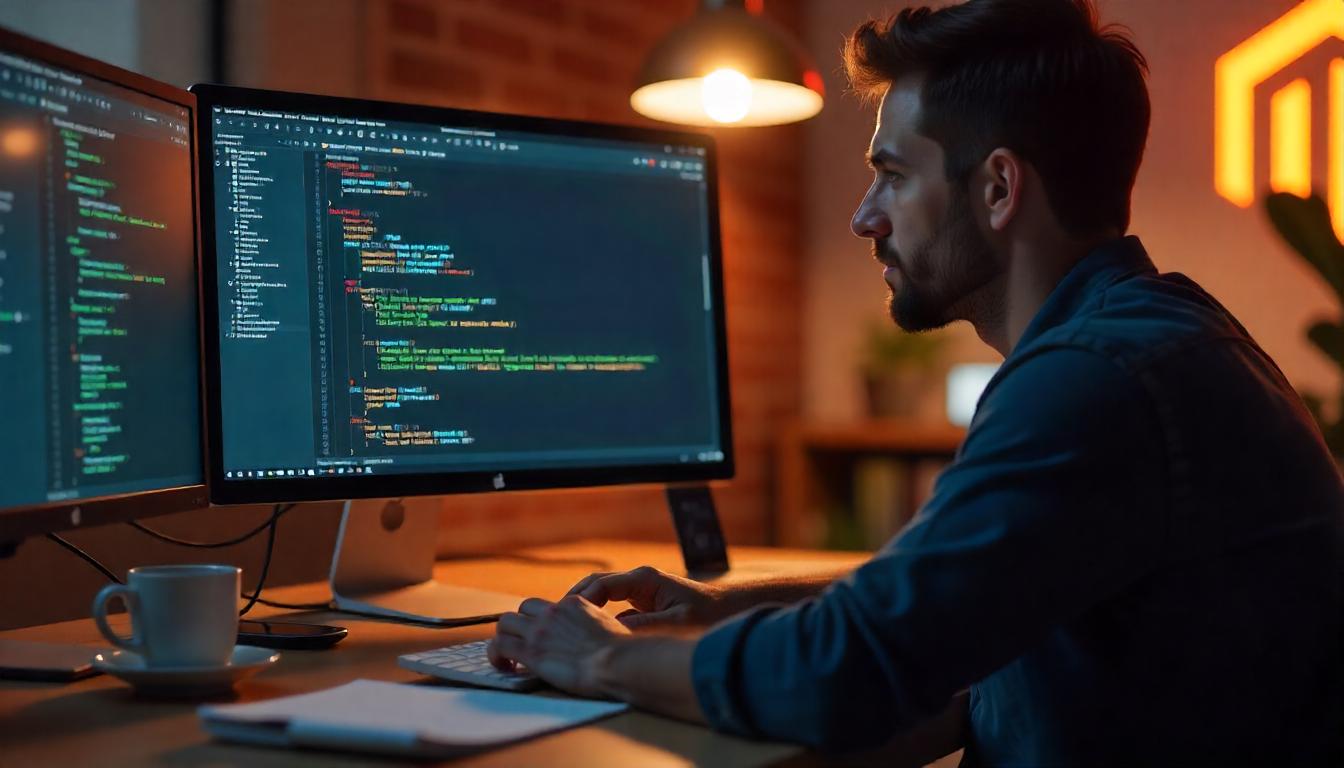
Modifying Product Final Price in Magento 2 Using the catalog_product_get_final_price Event
Learn how to modify a product's final price in Magento 2 using the catalog_product_get_final_price event. This guide walks you through defining the event in events.xml, creating a custom observer, and dynamically adjusting product pricing.
Table Of Content
Modifying Product Final Price in Magento 2 Using the catalog_product_get_final_price Event
In Magento 2, you can adjust a product's final price by leveraging the catalog_product_get_final_price
event. This event, located in the Magento\Catalog\Model\Product\Type\Price
class, allows developers to modify product pricing dynamically.
Implementing the Price Modification Observer
To set a maximum product price, for instance, $100, follow these steps:
Define the Event in events.xml
Create the events.xml
file in your module's etc/frontend
directory:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="catalog_product_get_final_price">
<observer name="custom_price_observer" instance="Vendor\Module\Observer\CustomPriceObserver" />
</event>
</config>
Create the Observer Class
Develop the observer class CustomPriceObserver.php
in the Observer
directory of your module:
<?php
namespace Vendor\Module\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
class CustomPriceObserver implements ObserverInterface
{
public function execute(Observer $observer)
{
$product = $observer->getEvent()->getProduct();
$maxPrice = 100;
$finalPrice = min($product->getFinalPrice(), $maxPrice);
$product->setFinalPrice($finalPrice);
}
}
In this example, the observer checks if the product's final price exceeds $100. If it does, the price is set to $100; otherwise, the original price remains.
Considerations for API Usage
When working with REST or SOAP APIs, define events in the webapi_rest or webapi_soap areas of your module to ensure the events trigger correctly.
Additional Resources
For a comprehensive list of all events in Magento 2, refer to the official Magento documentation.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Modify a Product's Final Price in Magento 2?
You can modify the final price by using the catalog_product_get_final_price
event in Magento 2. This event allows dynamic price adjustments before displaying the final price to customers.
Where is the catalog_product_get_final_price Event Defined?
The event is defined in the Magento\Catalog\Model\Product\Type\Price
class and is used to determine a product's final price.
How Do I Register the Event in My Custom Module?
Create an events.xml
file inside your module's etc/frontend
directory and register the event like this:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="catalog_product_get_final_price">
<observer name="custom_price_observer" instance="Vendor\Module\Observer\CustomPriceObserver" />
</event>
</config>
How Can I Set a Maximum Product Price Using an Observer?
Create an observer class CustomPriceObserver.php
in your module's Observer
directory with the following code:
namespace Vendor\Module\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
class CustomPriceObserver implements ObserverInterface
{
public function execute(Observer $observer)
{
$product = $observer->getEvent()->getProduct();
$maxPrice = 100;
$finalPrice = min($product->getFinalPrice(), $maxPrice);
$product->setFinalPrice($finalPrice);
}
}
Will This Observer Restrict Prices Above the Set Limit?
Yes, the observer checks if the product's final price exceeds $100. If it does, it sets the price to $100. If the price is below $100, it remains unchanged.
Do I Need to Modify Anything for API Requests?
Yes, if you're using REST or SOAP APIs, ensure the event is registered in the webapi_rest
or webapi_soap
areas of your module to trigger properly.
Should I Clear Cache After Implementing These Changes?
Yes, after modifying the event, run the following command to clear the cache:
php bin/magento cache:clean
Where Can I Find More Events for Customization?
You can refer to the official Magento documentation for a complete list of events that can be used for customization.