How to Retrieve Applied Rule IDs for an Item in a Magento 2 Order?
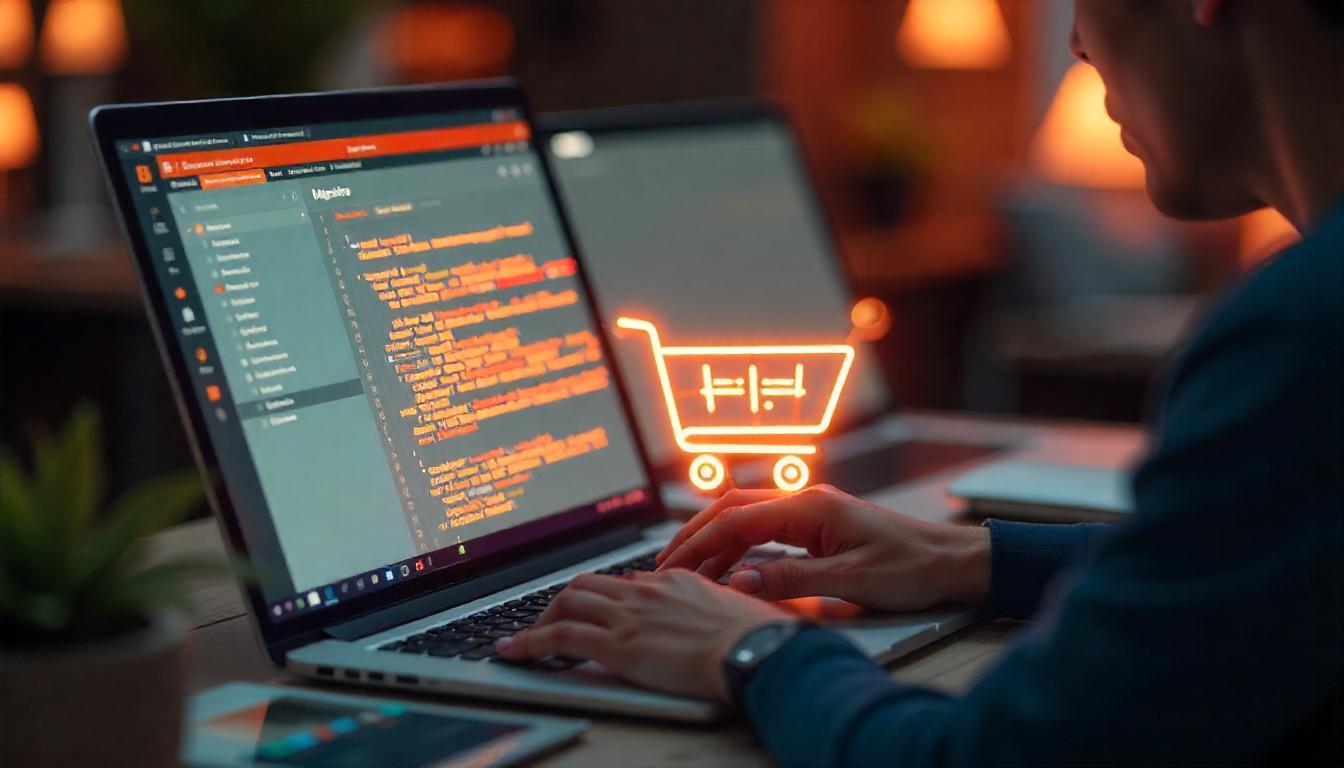
How to Retrieve Applied Rule IDs for an Item in a Magento 2 Order?
To retrieve applied rule IDs for an item in a Magento 2 order, you need to access the applied_rule_ids field in the sales_order_item table. This field stores the shopping cart rule IDs applied to each item during checkout. By fetching these IDs, you can track which promotions or discounts were applied at the item level.
Table Of Content
How to Retrieve Applied Rule IDs for an Item in a Magento 2 Order?
To retrieve the applied rule IDs for items in a Magento 2 order, you can access the applied_rule_ids field in the sales_order_item
table. This field stores the IDs of shopping cart rules applied to each item during checkout.
Understanding Applied Rule IDs in Magento 2
When a customer applies a discount code, such as "15Off," during checkout, Magento records the associated rule ID for each item in the order. These rule IDs are stored in the applied_rule_ids field of the sales_order_item table. This allows you to identify which promotional rules were applied to specific items.
Retrieving Applied Rule IDs Programmatically
To fetch the applied rule IDs for a specific order item, you can create a custom model in Magento 2. Below is an example of how to implement this:
<?php
namespace Vendor\Module\Model;
use Magento\Sales\Api\OrderItemRepositoryInterface;
use Psr\Log\LoggerInterface;
class AppliedRuleIds
{
private $orderItemRepository;
private $logger;
public function __construct(
OrderItemRepositoryInterface $orderItemRepository,
LoggerInterface $logger
) {
$this->orderItemRepository = $orderItemRepository;
$this->logger = $logger;
}
public function getAppliedRuleIds(int $itemId): ?string
{
try {
$orderItem = $this->orderItemRepository->get($itemId);
return $orderItem->getAppliedRuleIds();
} catch (\Exception $e) {
$this->logger->error('Error fetching applied rule IDs: ' . $e->getMessage());
return null;
}
}
}
Usage Example
To use this model, instantiate it and call the getAppliedRuleIds method with the desired order item ID:
$itemId = 123; // Replace with your order item ID
$appliedRuleIdsModel = $objectManager->create(\Vendor\Module\Model\AppliedRuleIds::class);
$ruleIds = $appliedRuleIdsModel->getAppliedRuleIds($itemId);
if ($ruleIds) {
echo "Applied Rule IDs: " . $ruleIds;
} else {
echo "No applied rule IDs found for this item.";
}
Important Considerations
Error Handling
: The code includes a try-catch block to handle potential exceptions when retrieving the order item.- Dependencies: Ensure that the
OrderItemRepositoryInterface
andLoggerInterface
are correctly injected into your model.
By implementing this approach, you can effectively retrieve and utilize the applied rule IDs for items within Magento 2 orders, enabling better management and analysis of promotional rules.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Applied Rule IDs for an Order Item in Magento 2?
You can retrieve applied rule IDs from the sales_order_item
table, where the applied_rule_ids
field stores all shopping cart rule IDs applied to each item during checkout.
Why Are Applied Rule IDs Important in Magento 2?
Applied rule IDs help track which discount or promotional rules were applied to specific order items, allowing for better reporting, debugging, and customization of promotional campaigns.
How Do I Retrieve Applied Rule IDs Programmatically?
You can use the OrderItemRepositoryInterface
to fetch the applied rule IDs for a specific order item.
namespace Vendor\Module\Model;
use Magento\Sales\Api\OrderItemRepositoryInterface;
use Psr\Log\LoggerInterface;
class AppliedRuleIds
{
private $orderItemRepository;
private $logger;
public function __construct(
OrderItemRepositoryInterface $orderItemRepository,
LoggerInterface $logger
) {
$this->orderItemRepository = $orderItemRepository;
$this->logger = $logger;
}
public function getAppliedRuleIds(int $itemId): ?string
{
try {
$orderItem = $this->orderItemRepository->get($itemId);
return $orderItem->getAppliedRuleIds();
} catch (\Exception $e) {
$this->logger->error('Error fetching applied rule IDs: ' . $e->getMessage());
return null;
}
}
}
How Do I Use This Method to Fetch Applied Rule IDs?
Call the method by passing an order item ID to retrieve its applied rule IDs.
$itemId = 123; // Replace with your order item ID
$appliedRuleIdsModel = $objectManager->create(\Vendor\Module\Model\AppliedRuleIds::class);
$ruleIds = $appliedRuleIdsModel->getAppliedRuleIds($itemId);
if ($ruleIds) {
echo "Applied Rule IDs: " . $ruleIds;
} else {
echo "No applied rule IDs found for this item.";
}
Do I Need to Handle Errors While Fetching Applied Rule IDs?
Yes, handling errors ensures stability. The example code includes a try-catch block to log errors if the order item cannot be retrieved.
Do I Need to Clear Cache After Implementing This?
Yes, after adding or modifying the code, run:
php bin/magento cache:clean
Can I Retrieve Applied Rule IDs Directly from the Database?
Yes, you can use the following SQL query:
SELECT applied_rule_ids FROM sales_order_item WHERE item_id = {item_id};
Replace {item_id}
with the actual order item ID.