Retrieving Quote Data by ID in Magento 2
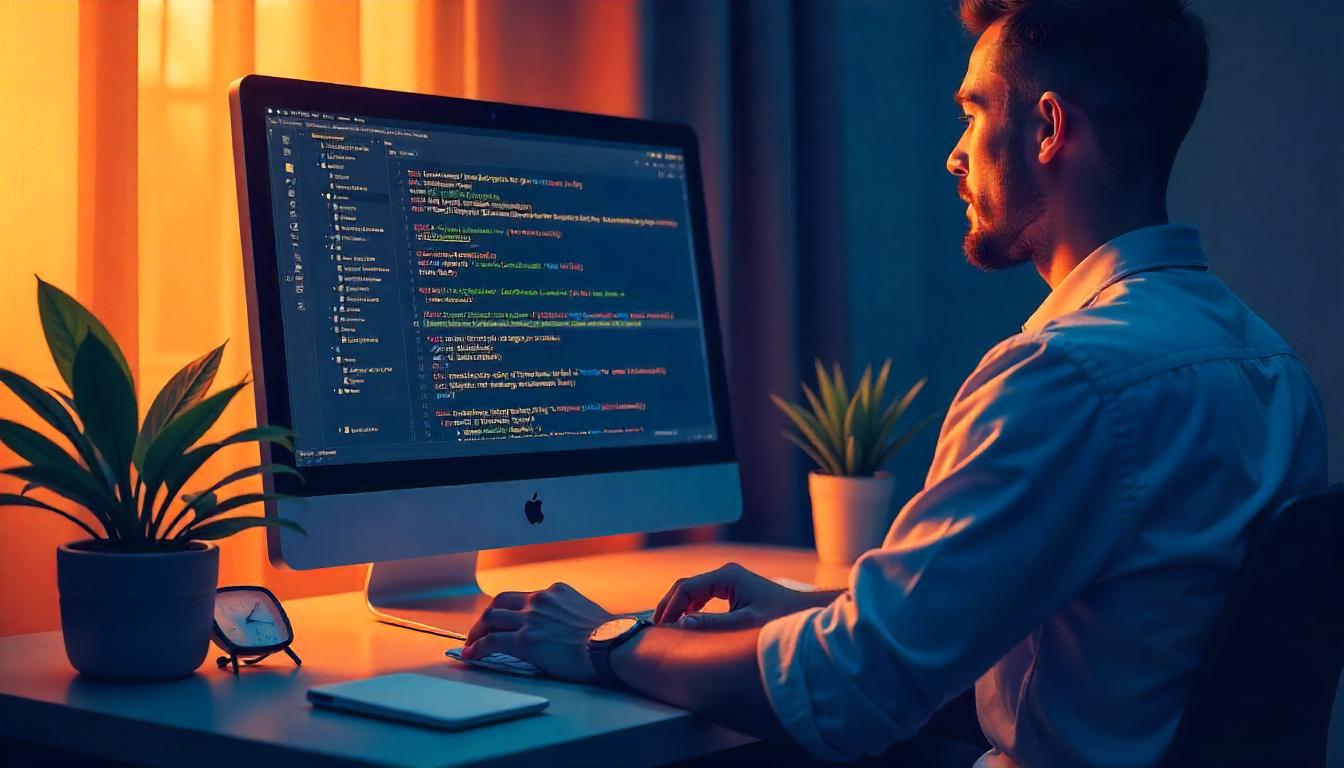
Retrieving Quote Data by ID in Magento 2
Retrieving quote data by ID in Magento 2 is essential for managing cart-related functionality. The best approach is using CartRepositoryInterface, which ensures efficient and reliable access to quote details.
Table Of Content
Retrieving Quote Data by ID in Magento 2
To access a specific quote in Magento 2 using its ID, you can utilize the CartRepositoryInterface. This approach is straightforward and efficient.
Method 1: Using CartRepositoryInterface
Here's how you can implement it:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Quote\Api\CartRepositoryInterface;
use Psr\Log\LoggerInterface;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Quote\Api\Data\CartInterface;
class QuoteById
{
private $quoteRepository;
private $logger;
public function __construct(
CartRepositoryInterface $quoteRepository,
LoggerInterface $logger
) {
$this->quoteRepository = $quoteRepository;
$this->logger = $logger;
}
/**
* Retrieve Quote by ID
*
* @param int $quoteId
* @return CartInterface|null
*/
public function getQuoteById(int $quoteId): ?CartInterface
{
try {
return $this->quoteRepository->get($quoteId);
} catch (NoSuchEntityException $e) {
$this->logger->error('Quote not found: ' . $e->getMessage());
return null;
}
}
}
In this code, we inject CartRepositoryInterface and LoggerInterface into the constructor. The getQuoteById method attempts to retrieve the quote by its ID and logs an error if the quote doesn't exist.
Method 2: Using QuoteFactory
Alternatively, you can use QuoteFactory
to load the quote. However, be aware that the load method is deprecated in Magento 2. It's recommended to use the repository pattern as shown above. If you choose to use QuoteFactory
, here's how:
use Magento\Quote\Model\QuoteFactory;
class QuoteById
{
private $quoteFactory;
public function __construct(QuoteFactory $quoteFactory)
{
$this->quoteFactory = $quoteFactory;
}
public function getQuoteById($quoteId)
{
return $this->quoteFactory->create()->load($quoteId);
}
}
This method creates an instance of the quote and loads it by ID. Due to the deprecation of the load method, it's advisable to use CartRepositoryInterface for future-proofing your code.
Summary
To retrieve quote data by ID in Magento 2, the preferred approach is to use CartRepositoryInterface
for its compliance with Magento's best practices. While QuoteFactory
is available, its load method is deprecated and not recommended for use in new developments.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve a Quote by ID in Magento 2?
You can retrieve a quote using the CartRepositoryInterface
. This method ensures proper data access while following Magento best practices.
Why Should I Use CartRepositoryInterface Instead of QuoteFactory?
Magento recommends using CartRepositoryInterface
as it follows the repository pattern, ensuring better performance and maintainability. The load
method in QuoteFactory
is deprecated.
How Do I Retrieve a Quote Programmatically?
Use the following method in your custom module to fetch a quote by its ID:
namespace Vendor\Module\Model;
use Magento\Quote\Api\CartRepositoryInterface;
use Psr\Log\LoggerInterface;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Quote\Api\Data\CartInterface;
class QuoteById
{
private $quoteRepository;
private $logger;
public function __construct(
CartRepositoryInterface $quoteRepository,
LoggerInterface $logger
) {
$this->quoteRepository = $quoteRepository;
$this->logger = $logger;
}
public function getQuoteById(int $quoteId): ?CartInterface
{
try {
return $this->quoteRepository->get($quoteId);
} catch (NoSuchEntityException $e) {
$this->logger->error('Quote not found: ' . $e->getMessage());
return null;
}
}
}
How Do I Use This Method to Fetch a Quote?
Call the method by passing a quote ID:
$quoteId = 1; // Replace with the actual quote ID
$quoteModel = $objectManager->create(\Vendor\Module\Model\QuoteById::class);
$quote = $quoteModel->getQuoteById($quoteId);
if ($quote) {
echo "Quote retrieved successfully.";
} else {
echo "No quote found for this ID.";
}
Should I Handle Errors When Retrieving a Quote?
Yes, handling errors is essential to prevent crashes. The example code includes a try-catch block to log errors if the quote is not found.
Do I Need to Clear Cache After Implementing This?
Yes, after adding or modifying the code, run:
php bin/magento cache:clean
Can I Retrieve Quote Data Directly from the Database?
Yes, you can use the following SQL query:
SELECT * FROM quote WHERE entity_id = {quote_id};
Replace {quote_id}
with the actual quote ID.