Adding a 'Pre-Order' Option to Magento 2's Backorder Settings
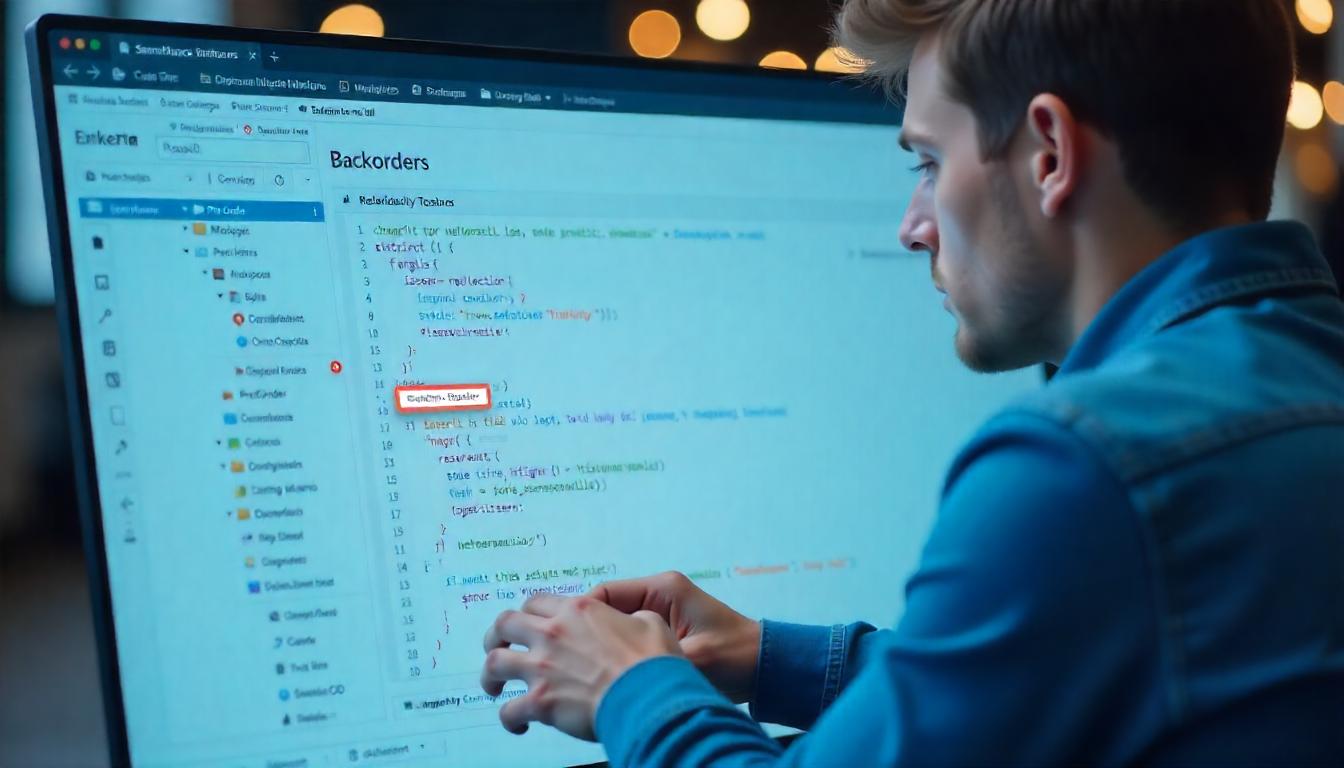
Adding a 'Pre-Order' Option to Magento 2's Backorder Settings
Magento 2 allows backorders, but it doesn’t include a built-in 'Pre-Order' option. By customizing the backorder settings, you can add a 'Pre-Order' status, letting customers place orders for out-of-stock products in advance.
Table Of Content
How to Retrieve Applied Rule IDs for an Item in a Magento 2 Order?
To introduce a 'Pre-Order' option in Magento 2's backorder settings, you'll need to modify the system by creating a plugin that adds this new option to the existing backorder statuses.
Steps to Add 'Pre-Order' to Backorder Options:
Access Advanced Inventory Settings:
- In the Magento admin panel, navigate to the product page.
- Click on the 'Advanced Inventory' link to open the inventory settings modal.
Create a Plugin to Add 'Pre-Order':
- Develop a plugin that extends the backorder options by adding a 'Pre-Order' status.
- This involves creating specific files in your Magento installation:
- di.xml: Defines the plugin and its target.
- PreOrderOption.php: Contains the logic to append the 'Pre-Order' option.
Sample Code Implementation:
di.xml (located at app/code/YourVendor/Preorder/etc/di.xml
):
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\CatalogInventory\Model\Source\Backorders">
<plugin name="YourVendor_Preorder::addOptionArray" type="YourVendor\Preorder\Plugin\PreOrderOption" />
</type>
</config>
PreOrderOption.php (located at app/code/YourVendor/Preorder/Plugin/PreOrderOption.php
):
<?php
namespace YourVendor\Preorder\Plugin;
use Magento\CatalogInventory\Model\Source\Backorders;
class PreOrderOption
{
/**
* Add new 'Pre-Order' option to backorders
*
* @param Backorders $subject
* @param array $options
* @return array
*/
public function afterToOptionArray(Backorders $subject, array $options)
{
$options[] = [
'value' => 50,
'label' => __('Allow Pre-Orders')
];
return $options;
}
}
Important Considerations:
- Option Value: The 'value' (e.g., 50) assigned to the 'Pre-Order' option should be unique and not conflict with existing backorder statuses.
- Customization: You can modify the 'value' and 'label' to fit your specific requirements.
- File Paths: Ensure that the file paths match your Magento installation's directory structure.
By implementing this plugin, the 'Pre-Order' option will become available in the backorders dropdown on the product inventory settings page, allowing you to manage pre-order statuses directly from the admin panel.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Add a 'Pre-Order' Option to Magento 2's Backorder Settings?
To introduce a 'Pre-Order' option in Magento 2's backorder settings, you need to create a plugin that extends the backorder options.
Where Can I Access Advanced Inventory Settings?
Navigate to the Magento admin panel, go to the product page, and click on 'Advanced Inventory' to open the inventory settings modal.
How Do I Create a Plugin to Add 'Pre-Order'?
You need to create specific files in your Magento installation, including di.xml
and PreOrderOption.php
.
What Code is Required to Modify Backorder Options?
Add the following code to di.xml
:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\CatalogInventory\Model\Source\Backorders">
<plugin name="YourVendor_Preorder::addOptionArray" type="YourVendor\Preorder\Plugin\PreOrderOption" />
</type>
</config>
How Do I Implement the 'Pre-Order' Option in PHP?
Create a PreOrderOption.php
file with this code:
namespace YourVendor\Preorder\Plugin;
use Magento\CatalogInventory\Model\Source\Backorders;
class PreOrderOption
{
public function afterToOptionArray(Backorders $subject, array $options)
{
$options[] = [
'value' => 50,
'label' => __('Allow Pre-Orders')
];
return $options;
}
}
What Should I Consider When Adding 'Pre-Order'?
Ensure the value assigned (e.g., 50) is unique and does not conflict with existing backorder statuses.
Do I Need to Clear Cache After Implementing This?
Yes, after adding or modifying the code, run:
php bin/magento cache:clean