Retrieve Order Entity ID Using Order Increment ID in Magento 2
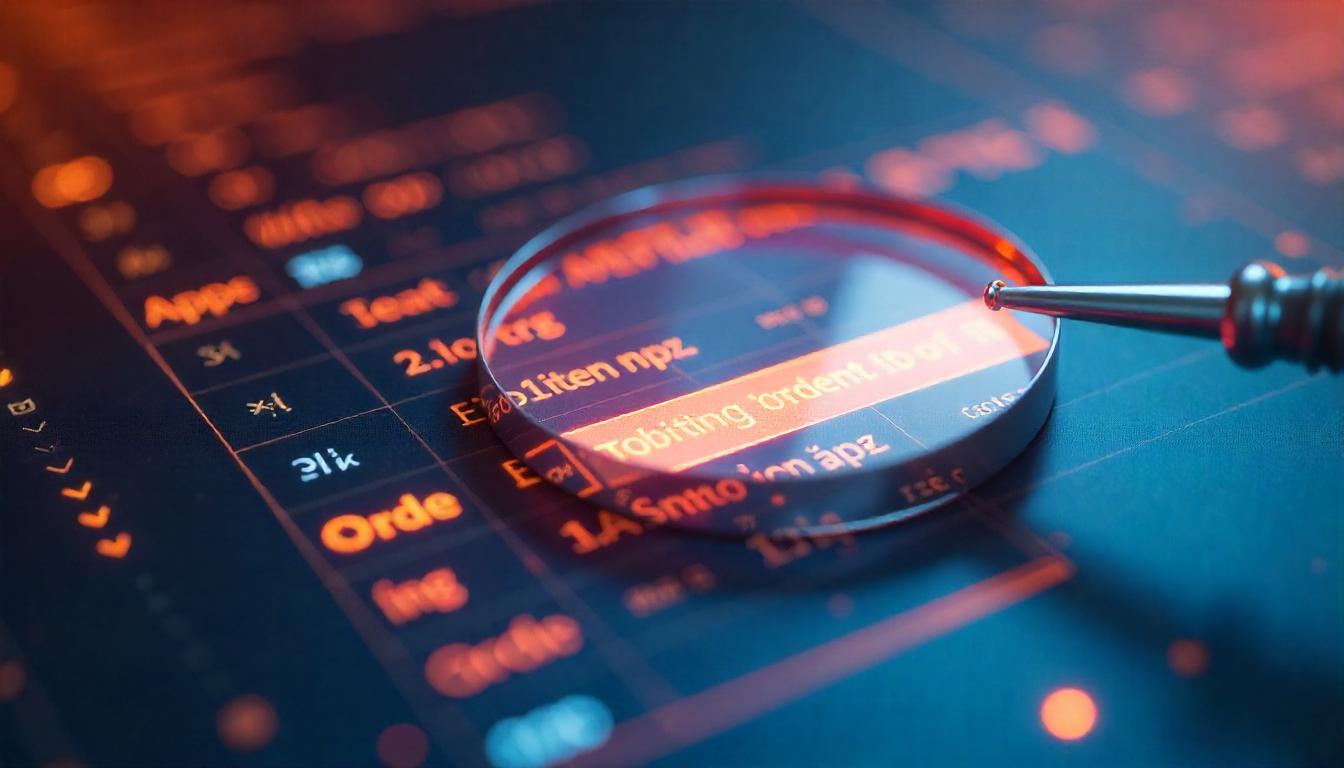
Retrieve Order Entity ID Using Order Increment ID in Magento 2
In Magento 2, the order entity ID is the primary key in the sales_order table, required for various order-related operations. However, if you only have the order increment ID, you'll need a way to retrieve the entity ID. This guide explains how to fetch the order entity ID programmatically using SearchCriteriaBuilder and OrderRepositoryInterface.
Table Of Content
How to Retrieve Applied Rule IDs for an Item in a Magento 2 Order?
To retrieve the applied rule IDs for items in a Magento 2 order, you can access the applied_rule_ids field in the sales_order_item
table. This field stores the IDs of shopping cart rules applied to each item during checkout.
Understanding Applied Rule IDs in Magento 2
When a customer applies a discount code, such as "15Off," during checkout, Magento records the associated rule ID for each item in the order. These rule IDs are stored in the applied_rule_ids field of the sales_order_item table. This allows you to identify which promotional rules were applied to specific items.
Retrieving Applied Rule IDs Programmatically
To fetch the applied rule IDs for a specific order item, you can create a custom model in Magento 2. Below is an example of how to implement this:
<?php
namespace Vendor\Module\Model;
use Magento\Sales\Api\OrderItemRepositoryInterface;
use Psr\Log\LoggerInterface;
class AppliedRuleIds
{
private $orderItemRepository;
private $logger;
public function __construct(
OrderItemRepositoryInterface $orderItemRepository,
LoggerInterface $logger
) {
$this->orderItemRepository = $orderItemRepository;
$this->logger = $logger;
}
public function getAppliedRuleIds(int $itemId): ?string
{
try {
$orderItem = $this->orderItemRepository->get($itemId);
return $orderItem->getAppliedRuleIds();
} catch (\Exception $e) {
$this->logger->error('Error fetching applied rule IDs: ' . $e->getMessage());
return null;
}
}
}
Key Points:
- Dependencies: Ensure you inject
SearchCriteriaBuilder
,OrderRepositoryInterface
, andLoggerInterface
into your class. - Method Functionality: The
getOrderEntityID
method accepts an order increment ID, builds a search criteria to filter orders by this ID, and returns the corresponding entity ID. Error Handling
: Exceptions are logged for troubleshooting purposes.
By utilizing this method, you can efficiently retrieve the order entity ID using the order increment ID in Magento 2.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve the Order Entity ID Using an Increment ID in Magento 2?
You can retrieve the order entity ID by filtering the sales_order
table using the order increment ID. The entity ID serves as the primary key in Magento's order database.
Why Do I Need the Order Entity ID Instead of the Increment ID?
Magento's order repository requires the entity ID when fetching order details using the get($id)
method. Since increment IDs are human-readable and not primary keys, conversion is necessary.
How Do I Retrieve the Order Entity ID Programmatically?
Use the following PHP code to retrieve the order entity ID by increment ID:
$searchCriteria = $this->searchCriteriaBuilder
->addFilter('increment_id', 'YOUR_ORDER_INCREMENT_ID')
->create();
$orderData = $this->orderRepository->getList($searchCriteria)->getItems();
foreach ($orderData as $order) {
$orderId = $order->getId();
}
Which Magento 2 Class Helps in Fetching the Order Entity ID?
The OrderRepositoryInterface
class is used to fetch order details, including the entity ID, by applying filters through SearchCriteriaBuilder
.
What Happens If the Increment ID Does Not Exist?
If no matching order is found for the given increment ID, the query returns an empty result. Logging errors using LoggerInterface
can help debug such cases.
Where Is the Order Entity ID Stored in Magento 2?
The order entity ID is stored in the sales_order
table under the entity_id
column.
Do I Need to Clear the Cache After Fetching Order Data?
Fetching data does not require cache clearing. However, if you modify order-related information, run:
php bin/magento cache:clean
Where Can I Find More Information on Magento 2 Order Processing?
Refer to Magento's official documentation for details on order management, repository usage, and database structure.