Checking User Type (Customer or Guest) in Magento 2 REST API
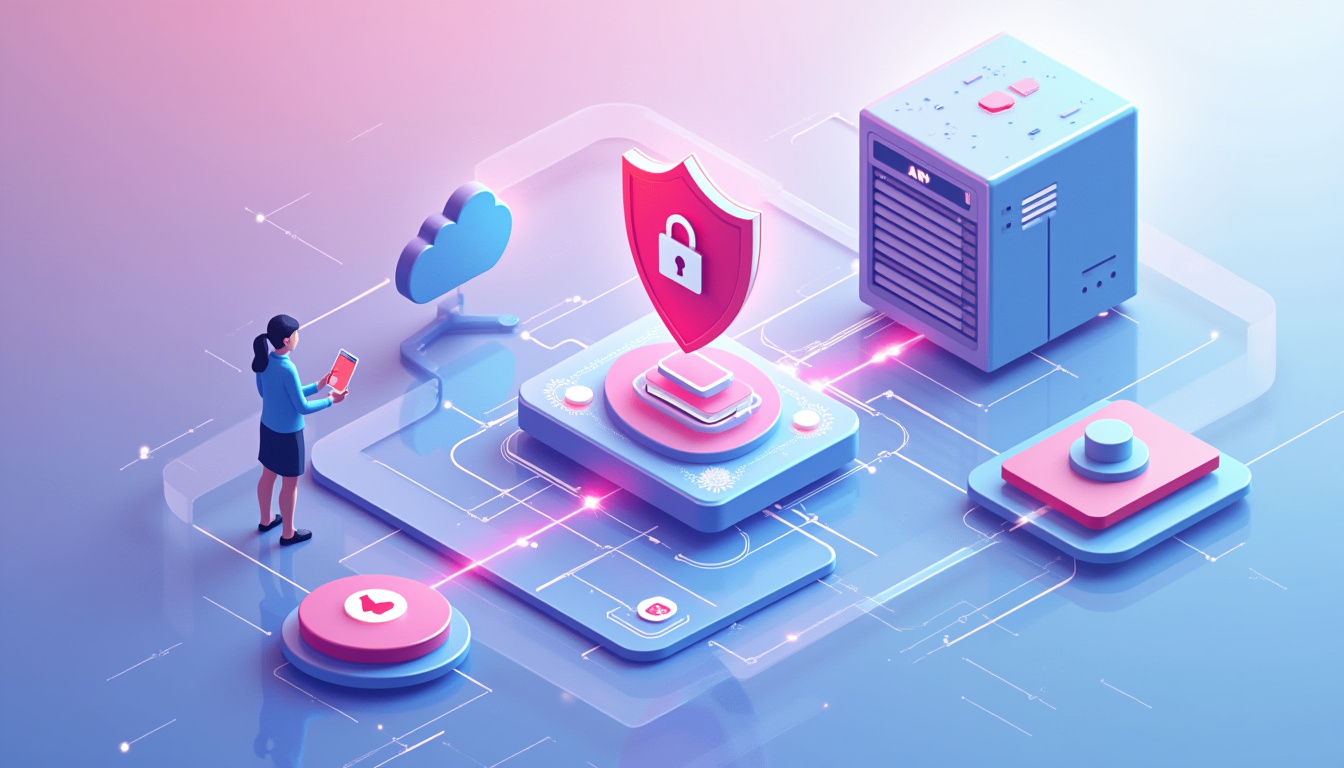
Checking User Type (Customer or Guest) in Magento 2 REST API
When working with Magento 2 REST API, it’s essential to determine whether the current user is a customer or a guest. This helps in managing access permissions effectively.
Magento distinguishes users based on their type, which affects their ability to access resources. Customer users have permissions based on authentication, while guest users can only access resources with anonymous permissions.
Table Of Content
Magento User Type Constants
Magento defines user types using constants stored in Magento\Authorization\Model\UserContextInterface
. These constants help determine user access levels, allowing developers to implement role-based functionalities effectively.
1. Magento User Type Overview
Magento provides predefined user types that determine access levels within the system:
User Type | Constant Name | Integer Value | Description |
---|---|---|---|
Integration User | USER_TYPE_INTEGRATION |
1 | Used for API integrations with Magento. |
Admin User | USER_TYPE_ADMIN |
2 | Represents an admin with backend access. |
Customer User | USER_TYPE_CUSTOMER |
3 | Represents a registered frontend customer. |
Guest User | USER_TYPE_GUEST |
4 | Represents users browsing the site without logging in. |
Understanding User Types in Magento
Each user type serves a specific purpose within Magento:
Integration User
- Used for API authentication and third-party integrations.
- Provides secure communication between external systems and Magento.
- Uses OAuth-based authentication methods.
Admin User
- Has backend access to manage products, orders, and settings.
- Permissions can be controlled via Role Management in Magento.
- Typically has the highest level of control.
Customer User
- Represents users with an account on the store.
- Can place orders, track shipments, and access saved data.
- Managed via Customer Groups for targeted promotions and pricing.
Guest User
- A user browsing the store without logging in.
- Can add items to the cart and proceed to checkout as a guest.
- Magento allows disabling guest checkout for specific stores.
How to Use User Type Constants in Magento Code?
Developers can retrieve the user type programmatically in Magento using:
<?php
use Magento\Authorization\Model\UserContextInterface;
public function getUserType(UserContextInterface $userContext)
{
$userType = $userContext->getUserType();
switch ($userType) {
case UserContextInterface::USER_TYPE_ADMIN:
return 'Admin User';
case UserContextInterface::USER_TYPE_CUSTOMER:
return 'Customer User';
case UserContextInterface::USER_TYPE_GUEST:
return 'Guest User';
case UserContextInterface::USER_TYPE_INTEGRATION:
return 'Integration User';
default:
return 'Unknown User Type';
}
}
Implementing User Type Detection in Magento 2
To determine whether the current user is a customer or guest, implement the following class:
Create a Custom Model for User Type Detection
Create a new model file at:
app/code/Emmo/CustomerGuestUserType/Model/CustomerGuestUserAPI.php
Add the following code:
<?php
namespace Emmo\CustomerGuestUserType\Model;
use Magento\Authorization\Model\UserContextInterface;
class CustomerGuestUserAPI
{
/**
* @var UserContextInterface
*/
private $userContextType;
/**
* Constructor
*
* @param UserContextInterface $userContextType
*/
public function __construct(
UserContextInterface $userContextType
) {
$this->userContextType = $userContextType;
}
/**
* Get Customer or Guest User Type
*
* @return int|null
*/
public function getCustomerOrGuestUserType(): ?int
{
return $this->userContextType->getUserType();
}
}
How to Use the Above Class
Call the method to fetch the user type:
echo $userType = $this->getCustomerOrGuestUserType();
Inject the Class in a Controller or Block
To use this in a controller or block, inject the model and fetch the user type:
use Emmo\CustomerGuestUserType\Model\CustomerGuestUserAPI;
class SomeClass
{
private $customerGuestUserAPI;
public function __construct(CustomerGuestUserAPI $customerGuestUserAPI)
{
$this->customerGuestUserAPI = $customerGuestUserAPI;
}
public function execute()
{
$userType = $this->customerGuestUserAPI->getCustomerOrGuestUserType();
echo "User Type: " . $userType;
}
}
Example Output
If a logged-in customer accesses this method, it will return:
User Type: 3
If a guest user accesses this method, it will return:
User Type: 4
Handling Different User Types in Magento
To take different actions based on the user type, you can use the following switch case:
$userType = $this->customerGuestUserAPI->getCustomerOrGuestUserType();
switch ($userType) {
case UserContextInterface::USER_TYPE_ADMIN:
echo "Admin user detected!";
break;
case UserContextInterface::USER_TYPE_CUSTOMER:
echo "Customer logged in.";
break;
case UserContextInterface::USER_TYPE_GUEST:
echo "Guest browsing the site.";
break;
case UserContextInterface::USER_TYPE_INTEGRATION:
echo "Integration user detected!";
break;
default:
echo "Unknown user type!";
break;
}
Tips for Magento 2 User Type Handling
- Always check user context before applying access restrictions.
- Use dependency injection (DI) to fetch user type instead of directly instantiating classes.
- Store user type values in session if needed for multiple requests.
- Ensure API-based user authentication respects these roles.
Implementing User Type Check in a REST API Endpoint
To use this logic in a REST API, create a custom endpoint that returns the user type:
Step 1: Define the Web API Route
Create the webapi.xml
file in your module at etc/webapi.xml
:
<?xml version="1.0"?>
<routes xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Webapi:etc/webapi.xsd">
<route url="/V1/custom/get-user-type" method="GET">
<service class="Emmo\CustomerGuestUserType\Api\UserTypeInterface" method="getUserType"/>
<resources>
<resource ref="anonymous"/>
</resources>
</route>
</routes>
Step 2: Create the Interface
Define the API interface in Api/UserTypeInterface.php
:
<?php
<namespace Emmo\CustomerGuestUserType\Api;>
<interface UserTypeInterface>
/**
* Get user type
*
* @return int
*/
public function getUserType();
</interface>
Step 3: Implement the API Interface
Create the API model class Model/UserType.php
:
<?php
<namespace Emmo\CustomerGuestUserType\Model;>
<use Emmo\CustomerGuestUserType\Api\UserTypeInterface;>
<use Magento\Authorization\Model\UserContextInterface;>
<class UserType implements UserTypeInterface>
private $userContext;
public function __construct(UserContextInterface $userContext)
{
$this->userContext = $userContext;
}
public function getUserType()
{
return $this->userContext->getUserType();
}
</class>
Step 4: Declare the API Model as a Service
Register the model as a service in di.xml
:
<preference for="Emmo\CustomerGuestUserType\Api\UserTypeInterface"
type="Emmo\CustomerGuestUserType\Model\UserType" />
Now, calling the API /V1/custom/get-user-type will return the current user type.
Best Practices & Expert Tips for Handling User Types in Magento 2
Magento 2 provides robust user role management, allowing developers to control authentication, permissions, and access dynamically. However, efficiently handling user types requires optimized performance, security considerations, and debugging techniques. Follow these best practices to ensure a smooth implementation.
1. Optimize Performance with User Type Caching
Fetching the user type on every request can slow down performance, especially for logged-in customers. Instead, cache the user type to avoid redundant API calls.
How to Implement User Type Caching in Magento 2
Modify your class to store user type in session storage:
<?php
use Magento\Framework\Session\SessionManagerInterface;
class CustomerGuestUserAPI
{
private $userContextType;
private $session;
public function __construct(
UserContextInterface $userContextType,
SessionManagerInterface $session
) {
$this->userContextType = $userContextType;
$this->session = $session;
}
public function getCustomerOrGuestUserType(): ?int
{
if ($this->session->getUserType()) {
return $this->session->getUserType();
}
$userType = $this->userContextType->getUserType();
$this->session->setUserType($userType);
return $userType;
}
}
Benefits of Caching User Type:
- Reduces API calls and improves response time.
- Enhances session persistence, ensuring data remains available.
- Minimizes database load by storing user type in memory.
2. Restrict API Access Based on User Type
Not all users should have access to every API endpoint. Restricting access based on user type enhances security.
How to Restrict API Access by User Type
Use a plugin to modify API responses:
<route url="/V1/custom-endpoint" method="GET">
<service class="Emmo\CustomerGuestUserType\Api\CustomInterface" method="execute"/>
<resources>
<resource ref="Magento_Customer::group"/>
</resources>
</route>
In your API class:
public function execute()
{
$userType = $this->customerGuestUserAPI->getCustomerOrGuestUserType();
if ($userType !== UserContextInterface::USER_TYPE_CUSTOMER) {
throw new LocalizedException(__('Access Denied'));
}
return ['message' => 'Welcome, Customer!'];
}
Security Advantages
- Prevents unauthorized access by guests or admins.
- Improves API security by enforcing strict authentication rules.
- Enhances performance by restricting unnecessary API calls.
3. Use Middleware for Dynamic Authentication & Access Control
Middleware allows real-time user validation before executing requests, ensuring proper authentication.
Middleware Implementation
In di.xml
, define your custom middleware:
<type name="Magento\Framework\App\FrontController">
<plugin name="custom_auth_middleware" type="Emmo\Middleware\CustomAuthMiddleware" sortOrder="10"/>
</type>
Create CustomAuthMiddleware.php
:
<?php
namespace Emmo\Middleware;
use Magento\Framework\App\RequestInterface;
use Magento\Framework\App\ResponseInterface;
use Magento\Framework\App\ActionInterface;
use Magento\Framework\Exception\LocalizedException;
class CustomAuthMiddleware
{
public function beforeDispatch(ActionInterface $subject, RequestInterface $request)
{
$userType = $this->customerGuestUserAPI->getCustomerOrGuestUserType();
if ($userType === UserContextInterface::USER_TYPE_GUEST && $request->getFullActionName() === 'restricted_page') {
throw new LocalizedException(__('You must be logged in to access this page.'));
}
}
}
Why Use Middleware?
- Real-time access control for specific routes.
- Reduces security risks by preventing unauthorized access.
- Enhances user experience by guiding users correctly.
4. Debugging: Fix Common Issues with User Type Detection
Issue | Possible Cause | Solution |
---|---|---|
User type returns null |
Session expired or uninitialized | Ensure the user session is started before fetching the user type. |
User type always returns 4 (Guest) |
Cookies disabled or authentication not persisting | Enable cookies and verify the authentication mechanism. |
Admin user detected as a customer | Incorrect user context injected | Use UserContextInterface correctly in dependency injection (DI). |
Slow performance when checking user type | Fetching user type on every request | Implement caching to store user type for the session and reduce repeated lookups. |
Magento API returns unauthorized error | User context is not passed correctly | Ensure the correct authentication headers are included in API requests. |
Custom module not detecting user type | Missing dependency in di.xml |
Verify that UserContextInterface is correctly injected in the module configuration. |
Debugging Steps
Check authentication headers in API requests:
<?php
$headers = getallheaders();
print_r($headers);
?>
Verify session persistence:
echo $_SESSION['user_type'];
Use Magento logs:
$this->logger->info('User type: ' . $userType);
Clear cache & flush sessions:
bin/magento cache:flush
bin/magento cache:clean
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Identifying user types (customer or guest) in Magento 2 REST API is a crucial aspect of managing access control, security, and personalized experiences. By leveraging UserContextInterface, developers can efficiently determine whether a request originates from a logged-in customer, a guest, an admin, or an integration user. This knowledge helps in enforcing authentication rules, optimizing API responses, and securing sensitive data.
With Magento 2.4.7 (2025), ensuring a seamless API experience requires best practices such as caching user sessions, implementing middleware for access control, and logging authentication events to prevent unauthorized access. By structuring API interactions around user types, businesses can create a more secure, efficient, and personalized eCommerce ecosystem.
By following the outlined implementation, best practices, and debugging techniques, you can enhance your Magento 2 store’s API security, improve performance, and deliver a better experience for both customers and guests. Keep refining your API strategies to stay ahead in the evolving eCommerce landscape.
FAQs
What is the purpose of checking user type in Magento 2 REST API?
Checking the user type helps determine whether the request is coming from a logged-in customer, guest, admin, or integration user. This ensures proper access control and security.
How does Magento 2 differentiate between a customer and a guest?
Magento 2 differentiates users based on authentication. Customers have an account and login credentials, whereas guests are unauthenticated users who can only access resources with anonymous permissions.
Where are user type constants defined in Magento 2?
User type constants are stored in Magento\Authorization\Model\UserContextInterface
.
What are the different user types in Magento 2?
Magento 2 has the following user types:
- Integration User (1)
- Admin User (2)
- Customer User (3)
- Guest User (4)
How can I programmatically check if a user is a customer or guest?
You can use the UserContextInterface
in a custom class and call the getUserType()
method to retrieve the user type.
How do I implement user type detection in Magento 2?
Create a custom class that injects UserContextInterface
and use getUserType()
to check the user type.
What is the best way to restrict API access based on user type?
Use middleware to filter API requests based on the user type and deny access to unauthorized users.
How can I improve the performance of user type detection?
Caching the user type result can minimize repeated API calls and improve performance.
How can I display the user type in Magento 2?
You can use a switch case statement to display user types based on the returned integer value from getUserType()
.
What should I do if user type detection fails?
Check for session persistence, token authentication issues, and ensure UserContextInterface
is properly injected in the class.
Can I use user type detection for frontend users?
Yes, you can check user type for frontend users by implementing it in GraphQL or REST API calls.
Is it possible to restrict guest users from accessing specific API endpoints?
Yes, you can configure access control lists (ACLs) or use custom middleware to restrict guest users.
What is the latest version of Magento covered in this guide?
This guide is updated for Magento 2.4.7 (2025), ensuring compatibility with the latest features and security updates.