How to Retrieve a Customer's Remote IP from an Order in Magento 2?
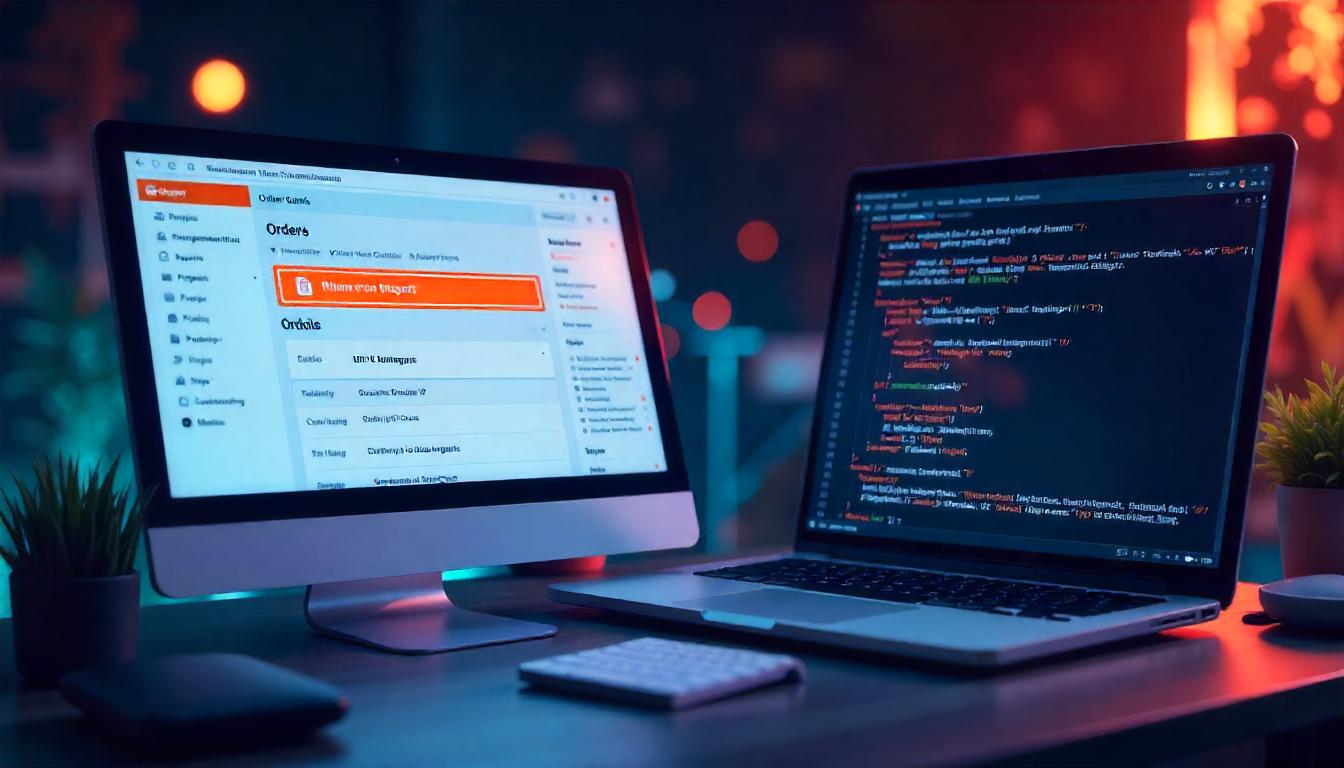
How to Retrieve a Customer's Remote IP from an Order in Magento 2?
In Magento 2, the remote_ip field in the sales_order table stores the customer's IP address when they place an order through the frontend. However, if the order is created from the backend (admin panel), this field remains empty.
Table Of Content
How to Retrieve a Customer's Remote IP from an Order in Magento 2?
To retrieve a customer's remote IP address from an order in Magento 2, you can access the remote_ip
field in the sales_order
table. This field captures the customer's IP when an order is placed through the frontend.
Frontend vs. Backend Order Placement:
- Frontend Orders: The
remote_ip
field is populated, indicating the order was placed by the customer through the storefront. - Backend Orders: When orders are created via the admin panel, the
remote_ip
field remains empty, as the IP address isn't recorded.
To determine the source of an order, check the remote_ip field:
- If
remote_ip
has a value: Order placed from the frontend. - If
remote_ip
is null or empty: Order placed from the backend.
Fetching the Remote IP Using Order Increment ID:
You can retrieve the remote IP address using the order's increment ID with the following code snippet:
<?php
namespace Vendor\Module\Block;
use Magento\Framework\View\Element\Template;
use Magento\Framework\Api\SearchCriteriaBuilder;
use Magento\Sales\Api\OrderRepositoryInterface;
use Psr\Log\LoggerInterface;
class OrderRemoteIp extends Template
{
protected $searchCriteriaBuilder;
private $logger;
private $orderRepository;
public function __construct(
Template\Context $context,
SearchCriteriaBuilder $searchCriteriaBuilder,
LoggerInterface $logger,
OrderRepositoryInterface $orderRepository,
array $data = []
) {
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
$this->logger = $logger;
$this->orderRepository = $orderRepository;
parent::__construct($context, $data);
}
public function getRemoteIp($incrementId)
{
try {
$searchCriteria = $this->searchCriteriaBuilder
->addFilter('increment_id', $incrementId)
->create();
$orders = $this->orderRepository->getList($searchCriteria)->getItems();
foreach ($orders as $order) {
$remoteIp = $order->getRemoteIp();
if ($remoteIp) {
return $remoteIp;
}
}
} catch (\Exception $e) {
$this->logger->error($e->getMessage());
}
return null;
}
}
Usage:
Call the getRemoteIp method with the specific order increment ID to obtain the customer's IP address.
Output:
- Frontend Order: Returns the customer's IP address (e.g., 127.0.0.1).
- Backend Order: Returns
null
since the IP isn't recorded.
This approach helps in identifying the source of an order and obtaining the customer's IP address when available.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve a Customer's Remote IP from an Order in Magento 2?
You can retrieve the customer's remote IP by accessing the remote_ip
field in the sales_order
table. This field stores the IP address of the customer when an order is placed via the frontend.
Why Is the Remote IP Important in Magento 2?
The remote IP helps track customer locations, detect fraud, and analyze traffic sources. It is useful for security and marketing analytics.
Does Magento 2 Store Remote IPs for All Orders?
No. The remote IP is stored only for orders placed from the frontend. Orders created through the admin panel do not have an associated remote IP.
How Can I Retrieve a Remote IP Programmatically?
Use the following PHP code to get the remote IP for an order:
$searchCriteria = $this->searchCriteriaBuilder
->addFilter('increment_id', 'YOUR_ORDER_INCREMENT_ID')
->create();
$orderData = $this->orderRepository->getList($searchCriteria)->getItems();
foreach ($orderData as $order) {
$remoteIp = $order->getRemoteIp();
}
Which Magento 2 Class Helps in Fetching the Remote IP?
The OrderRepositoryInterface
class is used to retrieve order details, including the remote_ip
field, by applying filters via SearchCriteriaBuilder
.
What Happens If an Order Doesn't Have a Remote IP?
If an order was created via the admin panel, the remote_ip
field will be empty. Logging with LoggerInterface
can help debug missing IPs.
Where Is the Remote IP Stored in Magento 2?
The customer's remote IP is stored in the sales_order
table under the remote_ip
column.
Where Can I Find More Information on Magento 2 Order Processing?
Refer to Magento's official documentation for details on order management, customer data retrieval, and database structure.