How to Check if a Customer is Subscribed to the Newsletter in Magento 2.4.7
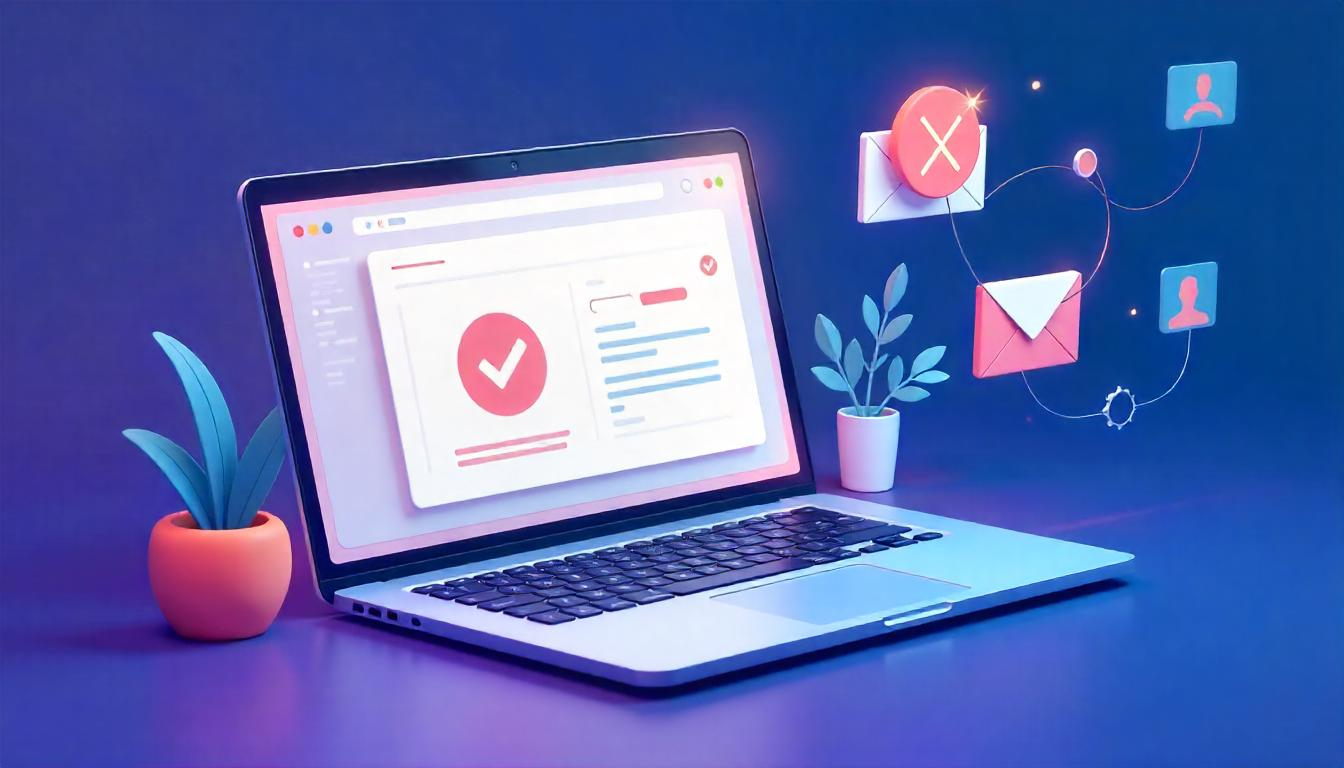
How to Check if a Customer is Subscribed to the Newsletter in Magento 2.4.7
Magento 2 provides a built-in Newsletter module that allows customers to subscribe and receive email updates. You may need to check if a specific customer is subscribed to the newsletter using their customer ID or email address.
Magento provides Magento\Newsletter\Model\SubscriberFactory
, which allows you to retrieve subscription status programmatically.
Table Of Content
Why Checking Newsletter Subscription is Important?
Ensuring that a customer's subscription status is verified is essential for maintaining an effective email marketing strategy. Below are key reasons why it matters:
Personalized Marketing
Send promotional emails only to customers who have opted in, increasing engagement and conversion rates.
Data Accuracy
Avoid sending emails to inactive or unsubscribed users, ensuring the right audience receives newsletters.
User Engagement
Prevent redundant or unwanted emails that could lead to customers marking messages as spam.
Legal Compliance
Respect user preferences and comply with GDPR, CAN-SPAM, and other data protection regulations to avoid legal penalties.
Best Practices for Managing Subscriptions
Practice | Benefit |
---|---|
Use Double Opt-in | Ensures genuine subscribers and reduces spam sign-ups. |
Provide Easy Unsubscribe Option | Enhances trust and compliance with regulations. |
Segment Your Audience | Targets users based on their preferences and past interactions. |
Regularly Clean Your Email List | Improves email deliverability by removing inactive subscribers. |
Track Subscription Metrics | Helps monitor engagement rates and optimize future campaigns. |
Pro Tip
Enable automated subscription confirmation emails to verify new sign-ups and keep your email list clean.
Magento 2 User Subscription Status Values
Magento assigns specific values to determine the subscription status of a user. Understanding these values is essential for managing customer subscriptions effectively.
Subscription Status Table
Subscription Status | Constant Name | Integer Value | Description |
---|---|---|---|
Subscribed | STATUS_SUBSCRIBED |
1 | The customer has opted in to receive newsletters and promotional emails. |
Not Subscribed | STATUS_NOT_ACTIVE |
3 | The customer has never subscribed or has manually disabled newsletter subscriptions. |
Unsubscribed | STATUS_UNSUBSCRIBED |
4 | The customer was previously subscribed but has opted out of receiving newsletters. |
Unconfirmed | STATUS_UNCONFIRMED |
2 | The customer signed up for the newsletter but has not yet confirmed their subscription via email verification. |
Best Practices for Managing Subscription Status
- Verify User Confirmation – Ensure users confirm their email before adding them to the subscription list.
- Offer Easy Opt-Out – Always provide a simple unsubscribe option to maintain trust and legal compliance.
- Segment Users Based on Subscription Status – Send different emails based on whether users are subscribed, unconfirmed, or unsubscribed.
- Automate Subscription Updates – Use Magento’s event-driven system to update subscription statuses dynamically.
- Monitor Subscription Metrics – Track open rates, click-through rates, and unsubscribes to optimize engagement.
Method 1: Check Subscription Status Using Customer ID or Email
Magento provides an easy way to check a customer's newsletter subscription status using SubscriberFactory
.
Why Use This Method?
- Fast & Efficient – Retrieves the subscription status directly from the database.
- Flexible – Works with both customer ID and email.
- Easy to Implement – Uses Magento's built-in methods.
Steps to Implement
- Inject
SubscriberFactory
into your custom class. - Use
isSubscribed()
to verify the subscription status. - Check the Response:
true
→ User is subscribedfalse
→ User is not subscribed
PHP Implementation
<?php
<namespace Emmo\Subscriber\Model;>
<use Magento\Framework\Exception\LocalizedException;>
<use Magento\Newsletter\Model\SubscriberFactory;>
<class IsSubscribed>
private $subscriberFactory;
public function __construct(SubscriberFactory $subscriberFactory)
{
$this->subscriberFactory = $subscriberFactory;
}
public function isCustomerSubscribeById($customerId)
{
$status = $this->subscriberFactory->create()
->loadByCustomerId((int)$customerId)
->isSubscribed();
return (bool)$status;
}
public function isCustomerSubscribeByEmail($email)
{
$status = $this->subscriberFactory->create()
->loadByEmail($email)
->isSubscribed();
return (bool)$status;
}
</class>
How to Use This Code?
Call the methods to check the subscription status using either Customer ID
or Email
.
$customerId = 1;
echo $this->isCustomerSubscribeById($customerId); // Returns true or false
$email = '[email protected]';
echo $this->isCustomerSubscribeByEmail($email); // Returns true or false
Expected Output
Scenario | Expected Output |
---|---|
Customer is subscribed | true |
Customer is not subscribed | false |
Invalid customer ID or email | false |
Common Issues & Solutions
Issue | Possible Cause | Solution |
---|---|---|
Always returns false |
Invalid email or customer ID | Ensure the provided email or ID exists in the database. |
Returns true for unsubscribed users |
Customer subscription status not updated | Refresh the subscription table and verify database records. |
Performance is slow | Fetching status on every request | Use caching to store the subscription status temporarily. |
Pro Tip
- Optimize Queries: Fetch multiple user subscriptions in a single query if checking in bulk.
- Use Caching: Store results in session or cache to reduce unnecessary database calls.
- Error Handling: Always handle exceptions to avoid breaking API calls.
Method 2: Checking Subscription Status Using SQL Query
For a direct approach, you can check a customer's subscription status using a SQL query.
SQL Query to Fetch Subscription Status
Run the following query in your Magento 2 database to retrieve the subscription status:
This query returns an integer value representing the user's subscription status. Use the previously mentioned status values to interpret the result.
Handling Different Subscription Scenarios
Magento users may have different subscription statuses. Here's how to handle them:
Subscription Status and Recommended Actions
Scenario | Solution |
---|---|
Customer is Subscribed | Allow marketing emails and promotional offers. |
Customer is Unsubscribed | Do not send promotional emails to respect user preferences. |
Customer is Not Active | Check account status before sending any emails. |
Customer is Unconfirmed | Resend the confirmation email to validate the subscription. |
Common Issues & Fixes
Subscription Status Not Updating?
If the subscription status does not update properly, try the following:
- Ensure the
newsletter_subscriber
table is correctly updated. - Flush the Magento cache:
- Reindex Magento:
php bin/magento cache:flush
php bin/magento indexer:reindex
SubscriberFactory
Not Working?
If SubscriberFactory
does not resolve properly, ensure it's correctly injected into your class constructor:
private $subscriberFactory;
public function __construct(SubscriberFactory $subscriberFactory) {
$this->subscriberFactory = $subscriberFactory;
}
Pro Tip
For performance optimization, avoid querying the database on every request. Instead, store the subscription status in a cache or session for faster access.
Best Practices for Handling Magento 2 Newsletter Subscriptions
Managing customer subscriptions effectively ensures better user engagement, compliance, and system performance. Below are key best practices to follow:
General Best Practices
- Use Dependency Injection – Always inject SubscriberFactory instead of instantiating it directly to follow Magento’s best practices.
- Avoid Direct SQL Queries – Use Magento’s models and repository patterns to maintain security and data integrity.
- Respect Customer Privacy – Never expose a user’s subscription status to unauthorized users to comply with GDPR and other regulations.
- Optimize Subscription Queries – Cache subscription status to reduce database calls and improve performance.
- Implement Error Handling – Ensure proper exception handling when retrieving subscription statuses to prevent application crashes.
- Log Subscription Changes – Maintain logs for subscription changes to track user interactions and debug issues.
- Allow Easy Unsubscription – Provide a clear and simple way for customers to unsubscribe to maintain trust and compliance.
Common Mistakes & How to Avoid Them
Common Mistake | Impact | Recommended Solution |
---|---|---|
Directly instantiating SubscriberFactory |
Disrupts Magento’s dependency injection system, making the code harder to manage and extend | Use constructor-based dependency injection to ensure proper object management |
Executing raw SQL queries | Introduces security risks, potential SQL injection vulnerabilities, and data integrity issues | Use Magento’s model and repository patterns to safely interact with the database |
Ignoring subscription errors | Can lead to unexpected application crashes and unreliable subscription status updates | Implement structured error handling and logging for troubleshooting |
Checking subscription status on every request | Increases database load, leading to slow page performance and high server resource usage | Use caching mechanisms to store subscription status temporarily |
Exposing subscription data without restrictions | Compromises customer privacy and violates compliance regulations like GDPR | Restrict subscription data access to authorized users only |
Performance Optimization Tips
- Use Caching for Subscription Checks – Store customer subscription status in session or cache to prevent unnecessary database queries.
- Batch Update Subscription Data – Instead of updating individual subscriptions, use batch updates for efficiency.
- Avoid Fetching Subscription Status on Every Page Load – Only query subscription status when necessary (e.g., during checkout or email campaigns)
- Implement Lazy Loading – Load subscription data asynchronously to enhance frontend performance.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Ensuring a seamless and efficient newsletter subscription management system is crucial for personalized marketing and enhanced customer engagement in Magento 2. With the SubscriberFactory model, checking a customer's subscription status using either their Customer ID or Email Address is simple and efficient.
By leveraging Magento's built-in models instead of direct SQL queries, you maintain a secure, scalable, and high-performance system. Additionally, understanding different subscription statuses—subscribed, unsubscribed, inactive, and unconfirmed—allows for better targeting and compliance with email marketing standards.
To keep your Magento store optimized:
- Use Dependency Injection for better performance.
- Cache subscription status to reduce database queries.
- Respect customer privacy and GDPR compliance.
- Reindex and flush cache regularly to avoid outdated subscription data.
With Magento 2.4.7 (2025) and its continuous improvements, following best practices and optimized workflows ensures that your email marketing strategies remain effective, legal, and customer-centric. Implement these methods today and keep your audience engaged while maximizing the potential of your online store!
FAQs
How can I check if a customer is subscribed to the newsletter using Customer ID?
You can check subscription status by using the isSubscribed()
method of SubscriberFactory
and passing the Customer ID.
How do I check a customer’s subscription status using their email?
Use SubscriberFactory
and call the isSubscribed()
method after loading the customer by email.
Which class is used to check customer subscription status in Magento 2?
The Magento\Newsletter\Model\SubscriberFactory
class is used to check if a customer is subscribed to the newsletter.
What are the different user types in Magento 2?
Magento 2 has four user types: Integration User (1), Admin User (2), Customer User (3), and Guest User (4), as defined in Magento\Authorization\Model\UserContextInterface
.
How do I determine if a user is a guest or a registered customer?
You can use UserContextInterface
to retrieve the user type and determine if they are a guest (USER_TYPE_GUEST
) or a registered customer (USER_TYPE_CUSTOMER
).
How do I retrieve the user type in Magento 2?
Use getUserType()
from UserContextInterface
to retrieve the current user type.
Can I cache the user type result for better performance?
Yes, caching user type results can reduce unnecessary API calls and improve performance, especially for frequently accessed endpoints.
How do I ensure security when checking user types in Magento 2?
Always use authentication tokens and restrict API access based on user roles to prevent unauthorized data access.
What happens if a customer is not subscribed to the newsletter?
If a customer is not subscribed, the isSubscribed()
method will return false
, indicating they are not on the newsletter list.
How do I subscribe a customer to the newsletter programmatically?
Use the Magento\Newsletter\Model\Subscriber
class and call subscribe($email)
to add a customer to the newsletter.
Can a guest user subscribe to the newsletter in Magento 2?
Yes, guest users can subscribe to the newsletter using their email address without needing a registered account.
How do I unsubscribe a customer from the newsletter?
You can use unsubscribe()
method from Magento\Newsletter\Model\Subscriber
to remove a customer from the newsletter.
What should I do if the user type detection fails?
Check authentication tokens, session persistence, and debug the API response to ensure the user type is retrieved correctly.