Retrieve Active Shipping Methods from Current Quote in Magento 2
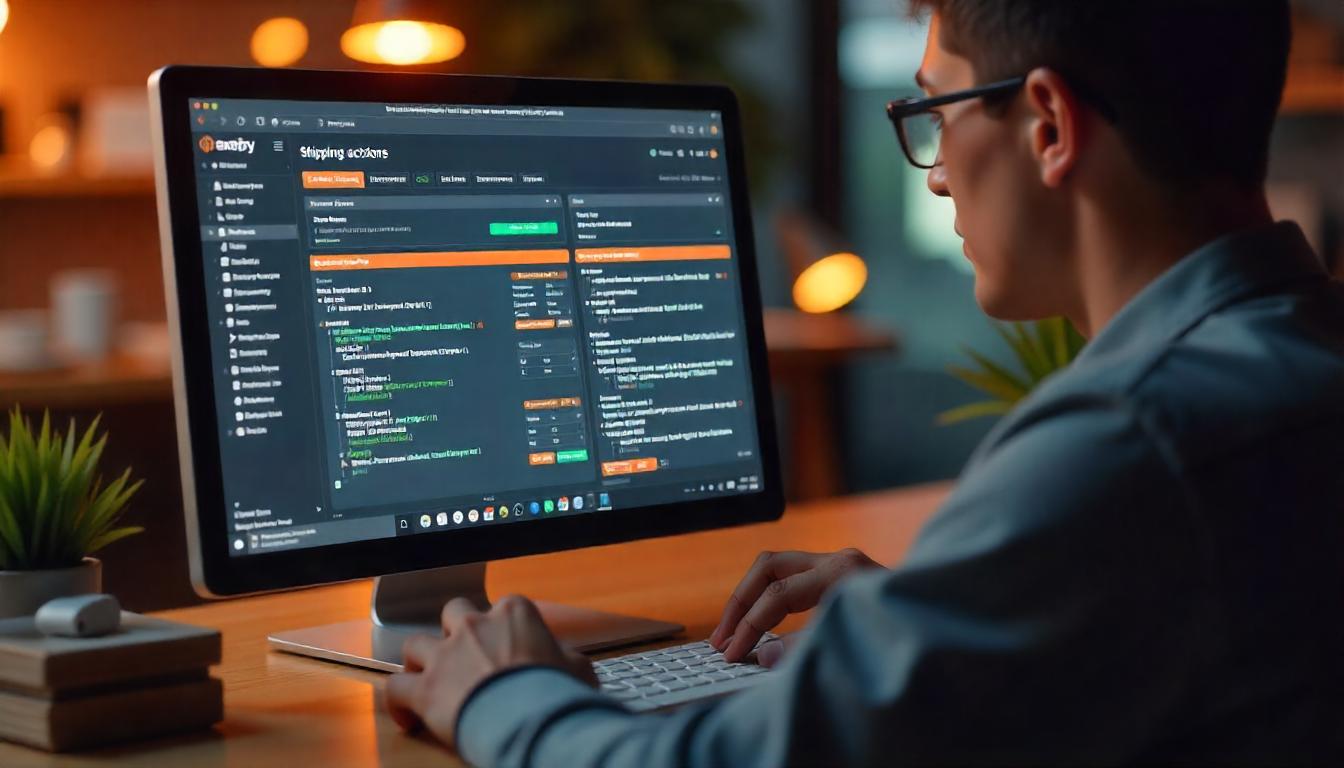
Retrieve Active Shipping Methods from Current Quote in Magento 2
Retrieving active shipping methods from a current quote in Magento 2 allows you to fetch details like carrier codes, method titles, and shipping costs. This is useful for checkout customization, order processing, and shipping calculations. By leveraging Magento\Quote\Api\ShippingMethodManagementInterface
, you can programmatically access available shipping options based on the active quote ID.
Table Of Content
Retrieve Active Shipping Methods from Current Quote in Magento 2
To obtain active shipping methods for a current quote in Magento 2, you can utilize the Magento\Quote\Api\ShippingMethodManagementInterface
. This interface allows you to fetch details like carrier codes, method titles, and shipping amounts associated with a specific quote ID.
Implementation Steps:
Inject Dependencies:
In your class, inject the ShippingMethodManagementInterface
to access shipping methods.
<?php
namespace Vendor\Module\Helper;
use Magento\Framework\App\Helper\AbstractHelper;
use Magento\Quote\Api\ShippingMethodManagementInterface;
use Magento\Framework\Exception\LocalizedException;
class Data extends AbstractHelper
{
protected $shippingMethodManagement;
public function __construct(
ShippingMethodManagementInterface $shippingMethodManagement
) {
$this->shippingMethodManagement = $shippingMethodManagement;
}
public function getShippingMethods($quoteId)
{
try {
$shippingMethods = $this->shippingMethodManagement->getList($quoteId);
$methods = [];
foreach ($shippingMethods as $method) {
$methods[] = [
'carrier_code' => $method->getCarrierCode(),
'method_title' => $method->getMethodTitle(),
'amount' => $method->getAmount()
];
}
return $methods;
} catch (\Exception $e) {
throw new LocalizedException(__($e->getMessage()));
}
}
}
?>
Fetch Shipping Methods:
Call the getShippingMethods
function with the desired quote ID to retrieve active shipping methods.
Considerations:
- Error Handling: If the provided quote ID isn't active or valid, an error like "No such entity with cartId = {quoteId}" will be thrown.
- Missing Shipping Address: If no shipping method is selected for the quote, you might encounter: "The shipping address is missing. Set the address and try again."
Example Output:
For a flat rate shipping method selected in the current quote, the output might be:
Array
(
[0] => Array
(
[carrier_code] => flatrate
[method_title] => Flat Rate
[amount] => 5.00
)
)
This approach ensures you retrieve all active shipping methods associated with a specific quote in Magento 2, facilitating customized checkout processes or other functionalities requiring shipping information.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Get Active Shipping Methods from a Quote in Magento 2?
You can use the Magento\Quote\Api\ShippingMethodManagementInterface
to fetch active shipping methods, including carrier codes, method titles, and amounts.
Why Would I Need to Retrieve Shipping Methods from a Quote?
Fetching shipping methods is useful for customizing the checkout process, validating shipping options, or implementing business logic based on available methods.
How Do I Implement This in Magento 2?
Inject the ShippingMethodManagementInterface
into your class and call its getList
method with a valid quote ID.
namespace Vendor\Module\Helper;
use Magento\Quote\Api\ShippingMethodManagementInterface;
use Magento\Framework\Exception\LocalizedException;
class Data
{
protected $shippingMethodManagement;
public function __construct(
ShippingMethodManagementInterface $shippingMethodManagement
) {
$this->shippingMethodManagement = $shippingMethodManagement;
}
public function getShippingMethods($quoteId)
{
try {
$methods = $this->shippingMethodManagement->getList($quoteId);
$result = [];
foreach ($methods as $method) {
$result[] = [
'carrier_code' => $method->getCarrierCode(),
'method_title' => $method->getMethodTitle(),
'amount' => $method->getAmount()
];
}
return $result;
} catch (\Exception $e) {
throw new LocalizedException(__($e->getMessage()));
}
}
}
What Errors Might Occur?
Common errors include:
- No such entity with cartId = X: The provided quote ID is invalid or inactive.
- The shipping address is missing: Ensure a shipping address is set before fetching shipping methods.
How Do I Test If the Code Works?
Call the getShippingMethods()
function with a valid quote ID. Example output for a flat rate shipping method:
Array
(
[0] => Array
(
[carrier_code] => flatrate
[method_title] => Flat Rate
[amount] => 5.00
)
)
How Can I Debug Issues with Shipping Method Retrieval?
Use Magento's logging system to track potential errors in your plugin:
$writer = new \Zend\Log\Writer\Stream(BP . '/var/log/shipping_methods.log');
$logger = new \Zend\Log\Logger();
$logger->addWriter($writer);
$logger->info('Fetching shipping methods for quote ID: ' . $quoteId);
What If Multiple Shipping Methods Are Available?
Magento returns all active shipping methods for the quote, so you may receive multiple options in the response.
Where Can I Learn More?
Check the official Magento developer documentation for details on shipping method retrieval and API usage.