How to Add or Update Product Tier Price Programmatically in Magento 2.4.7
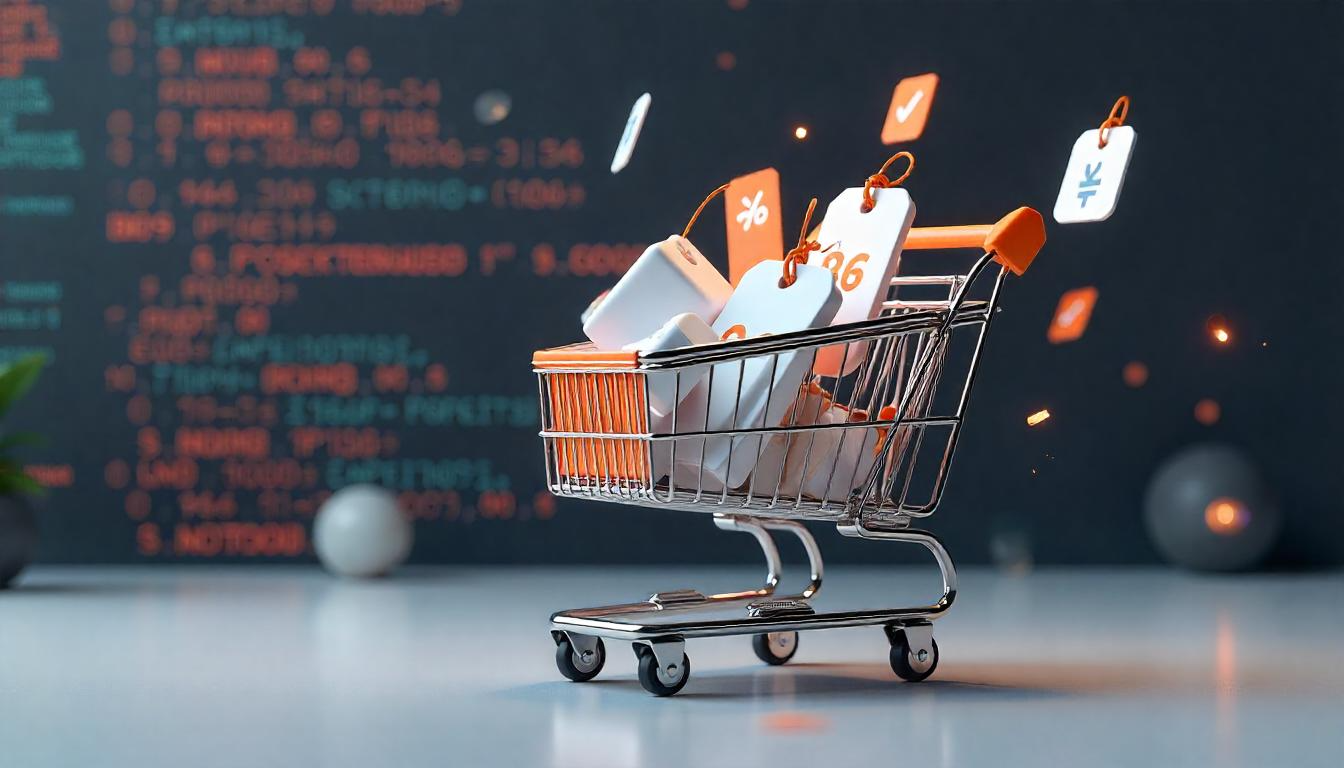
How to Add or Update Product Tier Price Programmatically in Magento 2.4.7
Magento 2 allows you to set tier pricing to offer discounts based on quantity. This can be done manually from the admin panel or programmatically. In this guide, we will cover how to add or update tier prices programmatically in Magento 2.4.7 using ScopedProductTierPriceManagementInterface
.
Table Of Content
Adding Tier Price from Magento 2 Admin Panel
Magento 2 allows store owners to set tier pricing to offer quantity-based discounts, encouraging bulk purchases and increasing customer retention.
Steps to Add/Update Tier Prices Manually:
Login to Admin Panel
- Go to your Magento 2 Admin Panel and enter your credentials.
Navigate to Products → Catalog
- In the sidebar, go to Catalog under the Products section.
Select the Product to Edit
- Find the product you want to apply tier pricing to and click Edit.
Access Advanced Pricing Settings
- Scroll down to the Price section.
- Click on Advanced Pricing to open pricing configurations.
Add Tier Price Details
- In the Tier Price section, click Add.
- Choose the Customer Group (e.g., General, Wholesale, Retailer, or All Groups).
- Set the Minimum Quantity required for the discount.
- Define the Discount Price (either a fixed amount or a percentage).
Save the Changes
- Click Save to apply the tier pricing to the product.
Example of Tier Pricing:
The following table demonstrates a sample tier pricing structure:
Minimum Quantity | Customer Group | Discount Type | Discounted Price |
---|---|---|---|
5+ | General | Fixed | $90 (original $100) |
10+ | General | Percentage | 15% off ($85) |
20+ | Wholesale | Fixed | $75 (special pricing) |
50+ | Retailer | Percentage | 25% off ($75) |
Additional Customization:
- Different pricing for specific customer groups: Tailor tier pricing for wholesale buyers or loyal customers.
- Set tier prices per store view: Adjust prices for different store views if running a multi-store setup.
- Import Tier Pricing in Bulk: Instead of manually adding tier prices, use CSV import in System → Import to update multiple products efficiently.
Pro Tip:
Use tier pricing strategically to increase your store’s average order value (AOV) and encourage bulk purchasing. Combine it with cart price rules for additional discounts to boost conversions!
Adding/Updating Tier Price Programmatically
Magento 2 provides a way to add or update tier prices programmatically using the ProductTierPriceManagementInterface. This allows developers to set bulk pricing dynamically through custom scripts or modules.
Using ProductTierPriceManagementInterface
To add a tier price, use the following method:
public function add($sku, \Magento\Catalog\Api\Data\ProductTierPriceInterface $tierPrice);
Parameters Explained:
Parameter | Description |
---|---|
$sku |
The SKU (Stock Keeping Unit) of the product for which the tier price is being set. |
$tierPrice |
Instance of ProductTierPriceInterface where you define the Quantity (Qty), Price, and Customer Group ID. |
Example: Adding a Tier Price Programmatically
Below is an example of how to add a tier price for a product using Magento’s API:
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Magento\Catalog\Api\Data\ProductTierPriceInterfaceFactory;
use Magento\Catalog\Api\ProductTierPriceManagementInterface;
class TierPriceManager
{
private $productRepository;
private $tierPriceFactory;
private $tierPriceManagement;
public function __construct(
ProductRepositoryInterface $productRepository,
ProductTierPriceInterfaceFactory $tierPriceFactory,
ProductTierPriceManagementInterface $tierPriceManagement
) {
$this->productRepository = $productRepository;
$this->tierPriceFactory = $tierPriceFactory;
$this->tierPriceManagement = $tierPriceManagement;
}
public function addTierPrice($sku, $qty, $price, $customerGroupId)
{
try {
$tierPrice = $this->tierPriceFactory->create();
$tierPrice->setCustomerGroupId($customerGroupId)
->setQty($qty)
->setValue($price);
$this->tierPriceManagement->add($sku, $tierPrice);
return "Tier Price successfully added for SKU: $sku";
} catch (\Exception $e) {
return 'Error: ' . $e->getMessage();
}
}
}
Advanced Customization
If you need to update existing tier prices instead of adding new ones, use getList() to fetch current tier prices and modify them accordingly before saving.
<?php
$tierPrices = $this->tierPriceManagement->getList($sku);
foreach ($tierPrices as $tierPrice) {
if ($tierPrice->getQty() == 5) {
$tierPrice->setValue(80); // Update price
}
}
$this->tierPriceManagement->add($sku, $tierPrice);
Pro Tip:
Always clear the cache php bin/magento cache:flush
and reindex php bin/magento indexer:reindex
after adding or updating tier prices to ensure changes are reflected correctly on the storefront.
Code to Add/Update Tier Price Programmatically in Magento 2
Magento 2 allows you to programmatically set tier pricing for products using its API. This is useful when managing bulk pricing updates or integrating with external systems.
Steps to Implement:
Create a Custom Module
Ensure you have a custom module in place, such as Emmo_AddTierPrice
.
Create a Model File
Place the AddUpdateTierPrice.php
file in Emmo/AddTierPrice/Model/
.
Inject Dependencies
Use ScopedProductTierPriceManagementInterface
and ProductTierPriceInterfaceFactory
to handle tier price management.
Code for Adding/Updating Tier Prices
<?php
namespace Emmo\AddTierPrice\Model;
use Magento\Catalog\Api\Data\ProductTierPriceInterfaceFactory;
use Magento\Catalog\Api\ScopedProductTierPriceManagementInterface;
use Magento\Framework\Exception\CouldNotSaveException;
use Magento\Framework\Exception\NoSuchEntityException;
class AddUpdateTierPrice {
private $tierPrice;
private $productTierPriceFactory;
public function __construct(
ScopedProductTierPriceManagementInterface $tierPrice,
ProductTierPriceInterfaceFactory $productTierPriceFactory
) {
$this->tierPrice = $tierPrice;
$this->productTierPriceFactory = $productTierPriceFactory;
}
public function addTierPrice() {
$qty = 7.00; // Must be a float value
$price = 20.00; // Must be a float value
$customerGroupId = 1; // Change this as needed (1 = General)
$sku = '24-MB02'; // SKU of the product
$tierPrice = false;
try {
$tierPriceData = $this->productTierPriceFactory->create();
$tierPriceData->setCustomerGroupId($customerGroupId)
->setQty($qty)
->setValue($price);
$tierPrice = $this->tierPrice->add($sku, $tierPriceData);
} catch (NoSuchEntityException $exception) {
throw new NoSuchEntityException(__($exception->getMessage()));
} catch (CouldNotSaveException $exception) {
throw new CouldNotSaveException(__("Failed to save tier price: " . $exception->getMessage()));
}
return $tierPrice;
}
}
Additional Details
Parameter | Description |
---|---|
$qty |
The minimum quantity required for the tier price to apply. Must be a float. |
$price |
The discounted price for the specified quantity. |
$customerGroupId |
Defines which customer group the tier price applies to. (1 = General, 2 = Wholesale, etc.) |
$sku |
The SKU of the product for which the tier price is being added. |
Tip:
If tier pricing does not update, make sure to:
- Clear Cache:
php bin/magento cache:flush
- Reindex Data:
php bin/magento indexer:reindex
- Enable Developer Mode:
php bin/magento deploy:mode:set developer
to see detailed error logs.
This ensures that changes reflect properly in the storefront
Calling the Function in a Template or PHP Class
To execute the tier price function in a template or within another PHP class, use the following approach:
echo $tierPriceData = $this->addTierPrice();
Expected Output
The function will return a Boolean value (true/false):
- true → Tier price was successfully added.
- false → The process failed due to an issue (e.g., invalid SKU, missing parameters, or incorrect customer group).
Error Handling
If an error occurs, exceptions like NoSuchEntityException
or CouldNotSaveException
may be thrown. To prevent errors, use a try-catch block:
try {
$tierPriceData = $this->addTierPrice();
echo $tierPriceData ? "Tier price added successfully!" : "Failed to add tier price.";
} catch (\Exception $e) {
echo "Error: " . $e->getMessage();
}
Debugging
If the function does not return the expected result:
- Check if the SKU exists in the catalog.
- Ensure the customer group ID is valid.
- Verify that
$qty
and$price
are correctly set as float values.
Tip:
Always clear the Magento cache after adding or modifying tier prices to ensure the changes reflect correctly:
php bin/magento cache:clean
This guarantees that tier prices are updated instantly without waiting for cache expiration.
Best Practices and Tips for Adding Tier Prices in Magento 2
When working with tier prices in Magento 2, following best practices ensures smooth functionality and prevents errors. Below are some key recommendations:
Best Practices and Tips
Tip | Description |
---|---|
Use Dependency Injection (DI) | Avoid direct object creation using ObjectManager. Always inject dependencies via the constructor to follow Magento's best practices. |
Validate Product SKU | Before adding a tier price, check if the product exists using Magento's ProductRepositoryInterface to avoid errors. |
Use Float for Qty & Price | Magento requires tier price quantity and value to be floats. Ensure correct data types to prevent unexpected behavior. |
Set Customer Group ID Properly | Use 0 to apply tier pricing for all customers or specify a valid customer group ID (1 = General, 2 = Wholesale, etc.). |
Handle Exceptions Gracefully | Always wrap your code in try-catch blocks to catch NoSuchEntityException, CouldNotSaveException, and other errors. Log exceptions properly for debugging. |
Clear Cache After Changes | Run bin/magento cache:clean and bin/magento cache:flush after modifying tier pricing to ensure changes reflect immediately. |
Use Bulk Tier Price Updates | Instead of adding tier prices one by one, consider batch updates using Magento\Catalog\Api\ProductTierPriceManagementInterface for better performance. |
Test on a Staging Environment | Always test tier price functionality on a staging or development environment before applying changes to production. |
Tip:
For large product catalogs, use Magento’s API or a CSV import to update tier prices efficiently, reducing manual work and potential errors.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Managing tier pricing in Magento 2 effectively enhances your store's pricing strategy, improving customer engagement and sales. Whether configuring tier prices manually via the admin panel or programmatically through custom modules, following Magento’s best practices ensures smooth functionality.
By leveraging Dependency Injection (DI), validating product SKUs, and using float values for quantity and pricing, you maintain data integrity. Implementing proper exception handling prevents system crashes, while bulk updates and caching strategies optimize performance.
For a seamless user experience, always test changes in a staging environment before deploying them live. With a structured approach to tier pricing, you can offer competitive discounts while keeping your Magento store scalable and efficient.
Optimize smartly, automate where possible, and deliver a dynamic pricing experience to your customers!
FAQs
What is tier pricing in Magento 2?
Tier pricing allows store owners to offer quantity-based discounts to customers, encouraging bulk purchases.
How can I manually add tier pricing in Magento 2?
Go to the Admin Panel → Products → Catalog → Edit Product → Advanced Pricing → Add Tier Price.
Which Magento interface is used to add tier pricing programmatically?
The ScopedProductTierPriceManagementInterface
is used to add or update tier pricing programmatically.
What data is required to set tier pricing via code?
You need the product SKU, customer group ID, quantity, and price value.
How can I retrieve the customer group ID for tier pricing?
You can retrieve it using \Magento\Customer\Model\Group
or by checking the Admin Panel under Customer Groups.
Why is my tier pricing not updating?
Ensure that the SKU exists, the values are in float format, and that you’ve cleared the cache after making changes.
How do I apply tier pricing to all customers?
Set the customer group ID to 0
when adding tier pricing.
Can I remove tier pricing programmatically?
Yes, use the remove
method from ScopedProductTierPriceManagementInterface
to delete tier pricing.
Does tier pricing work with Magento multi-store setups?
Yes, but you may need to specify the store ID while setting up tier pricing.
How do I verify if tier pricing is correctly applied?
Check the product page on the frontend or retrieve the tier prices using the Magento API.
Is tier pricing affected by customer group pricing?
Yes, tier pricing applies based on the customer group. Group-specific pricing may override it.
What happens if multiple tier prices are set for the same quantity?
Magento applies the lowest price available among the tier pricing options.
Do I need to reindex after updating tier pricing?
Yes, run bin/magento indexer:reindex
and clear the cache to ensure the updates reflect.