Implementing a Before Place Order Plugin in Magento 2
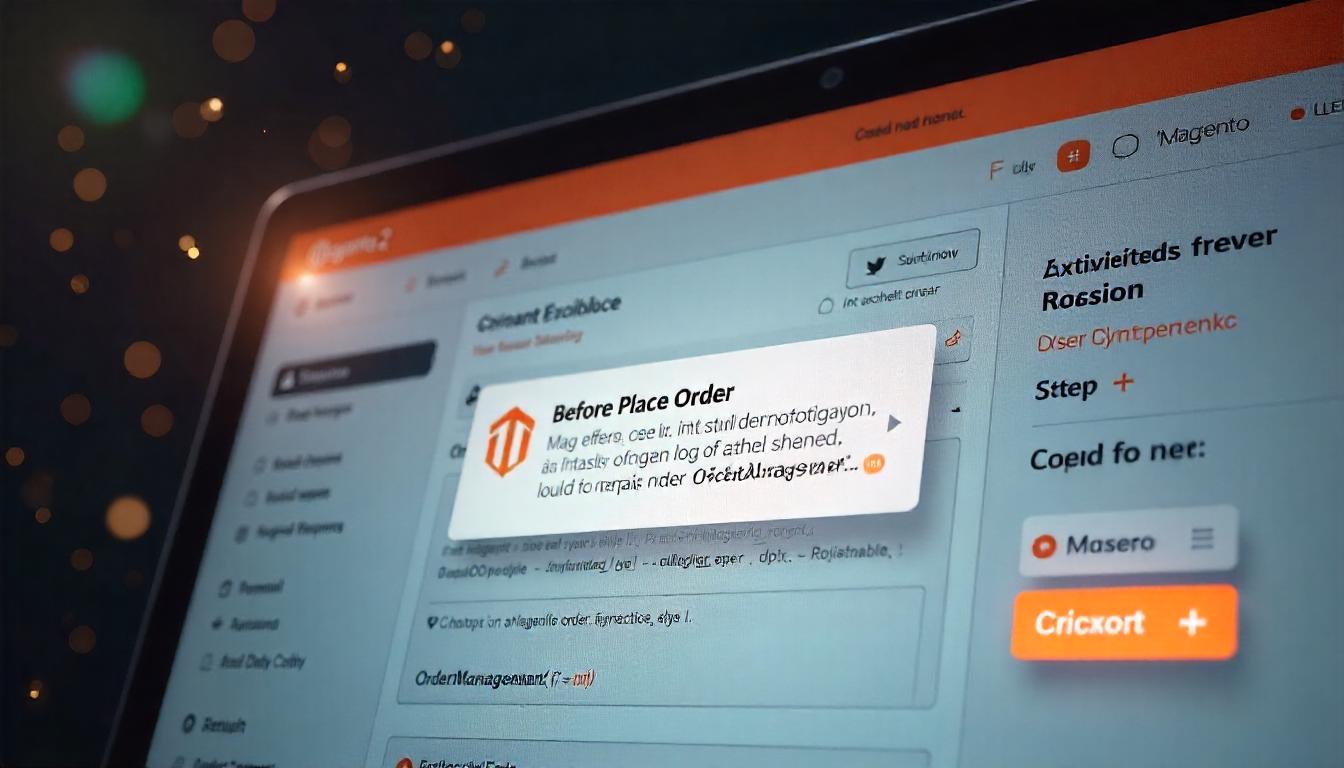
Implementing a Before Place Order Plugin in Magento 2
A Before Place Order plugin in Magento 2 allows you to modify or validate order data before it's finalized. By intercepting the place() method in the OrderManagementInterface, you can enforce business rules, validate order details, or modify order attributes before submission.
Table Of Content
Implementing a Before Place Order Plugin in Magento 2
To modify or validate data before an order is placed in Magento 2, you can create a plugin that intercepts the place()
method of the OrderManagementInterface
. This approach allows you to inject custom logic during the order placement process.
Step 1: Define the Plugin in di.xml
First, declare your plugin in the di.xml file located at app/code/Vendor/Module/etc/di.xml
:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Sales\Api\OrderManagementInterface">
<plugin name="before_place_order_plugin"
type="Vendor\Module\Plugin\OrderManagement"
sortOrder="1" />
</type>
</config>
This configuration specifies that the OrderManagement
plugin will intercept the OrderManagementInterface
. The sortOrder
attribute determines the execution sequence if multiple plugins are applied to the same method.
Step 2: Create the Plugin Class
Next, implement the plugin class at app/code/Vendor/Module/Plugin/OrderManagement.php
:
<?php
namespace Vendor\Module\Plugin;
use Magento\Sales\Api\Data\OrderInterface;
use Magento\Sales\Api\OrderManagementInterface;
use Magento\Framework\Exception\LocalizedException;
class OrderManagement
{
/**
* Before plugin for the place() method.
*
* @param OrderManagementInterface $subject
* @param OrderInterface $order
* @return OrderInterface[]
* @throws LocalizedException
*/
public function beforePlace(
OrderManagementInterface $subject,
OrderInterface $order
): array {
// Example: Validate the order's grand total
$grandTotal = $order->getGrandTotal();
if ($grandTotal < 100) {
throw new LocalizedException(__('Order amount must be at least 100.'));
}
// Additional custom logic can be added here
return [$order];
}
}
In this example, the beforePlace
method checks if the order's grand total is below 100. If so, it throws a LocalizedException
, preventing the order from being placed. You can replace this validation with any other checks or data modifications as needed.
Step 3: Apply the Plugin to Guest Orders (Optional)
If you need to apply similar validations for guest customers, create a plugin for the GuestPaymentInformationManagementInterface
. Update the di.xml
file:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<!-- For Logged-in Customers -->
<type name="Magento\Sales\Api\OrderManagementInterface">
<plugin name="before_place_order_plugin"
type="Vendor\Module\Plugin\OrderManagement"
sortOrder="1" />
</type>
<!-- For Guest Customers -->
<type name="Magento\Checkout\Api\GuestPaymentInformationManagementInterface">
<plugin name="guest_before_place_order_plugin"
type="Vendor\Module\Plugin\GuestOrderManagement"
sortOrder="1" />
</type>
</config>
Then, create the corresponding plugin class at app/code/Vendor/Module/Plugin/GuestOrderManagement.php
:
<?php
namespace Vendor\Module\Plugin;
use Magento\Checkout\Api\GuestPaymentInformationManagementInterface;
use Magento\Quote\Api\Data\PaymentInterface;
use Magento\Quote\Api\Data\AddressInterface;
use Magento\Quote\Api\CartRepositoryInterface;
use Magento\Framework\Exception\LocalizedException;
class GuestOrderManagement
{
/**
* @var CartRepositoryInterface
*/
private $cartRepository;
/**
* Constructor.
*
* @param CartRepositoryInterface $cartRepository
*/
public function __construct(CartRepositoryInterface $cartRepository)
{
$this->cartRepository = $cartRepository;
}
/**
* Before plugin for savePaymentInformationAndPlaceOrder method.
*
* @param GuestPaymentInformationManagementInterface $subject
* @param string $cartId
* @param string $email
* @param PaymentInterface $paymentMethod
* @param AddressInterface|null $billingAddress
* @throws LocalizedException
*/
public function beforeSavePaymentInformationAndPlaceOrder(
GuestPaymentInformationManagementInterface $subject,
$cartId,
$email,
PaymentInterface $paymentMethod,
AddressInterface $billingAddress = null
) {
$cart = $this->cartRepository->get($cartId);
$grandTotal = $cart->getGrandTotal();
if ($grandTotal < 100) {
throw new LocalizedException(__('Order amount must be at least 100.'));
}
// Additional custom logic can be added here
}
}
This plugin performs a similar validation for guest users before the order is placed.
Step 4: Deploy and Verify
After implementing the plugins, run the following commands to deploy and verify the changes:
php bin/magento setup:upgrade
php bin/magento cache:clean
php bin/magento cache:flush
These commands update the Magento system and clear the cache to ensure your plugin functions correctly.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is a Before Place Order Plugin in Magento 2?
A Before Place Order plugin in Magento 2 allows developers to execute custom logic before an order is placed.
Why Use a Before Place Order Plugin?
This plugin helps in modifying order data, validating additional conditions, or implementing custom business logic before an order is finalized.
How Do I Declare a Before Place Order Plugin?
Create a di.xml
file in your module’s etc
directory and define the plugin.
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework/ObjectManager/etc/config.xsd">
<type name="Magento\Checkout\Model\PaymentInformationManagement">
<plugin name="before_place_order_plugin"
type="Vendor\Module\Plugin\BeforePlaceOrder"
sortOrder="10"
disabled="false"/>
</type>
</config>
How Do I Create the Plugin Class?
Create a plugin class in your module’s Plugin
directory.
namespace Vendor\Module\Plugin;
use Magento\Checkout\Model\PaymentInformationManagement;
use Magento\Quote\Api\Data\PaymentInterface;
use Magento\Quote\Api\Data\AddressInterface;
class BeforePlaceOrder
{
public function beforeSavePaymentInformationAndPlaceOrder(
PaymentInformationManagement $subject,
$cartId,
PaymentInterface $paymentMethod,
AddressInterface $billingAddress
) {
// Custom logic before placing an order
}
}
How Do I Apply the Changes?
Run the following commands to apply updates:
php bin/magento setup:upgrade
php bin/magento cache:flush
php bin/magento setup:di:compile
php bin/magento setup:static-content:deploy -f
How Can I Debug a Before Place Order Plugin?
Use Magento's logging system to debug your plugin:
$writer = new \Zend\Log\Writer\Stream(BP . '/var/log/custom.log');
$logger = new \Zend\Log\Logger();
$logger->addWriter($writer);
$logger->info('Before Place Order plugin executed.');
What If My Plugin Doesn’t Work?
Check the following:
- Ensure the
di.xml
configuration is correct. - Verify the plugin class exists and follows Magento’s best practices.
- Flush cache and redeploy static content.
Where Can I Learn More About Magento 2 Plugins?
Refer to the official Magento developer documentation for an in-depth guide on using plugins.