How to Customize the Ship-To Section in Order Summary Checkout in Magento 2.4.7
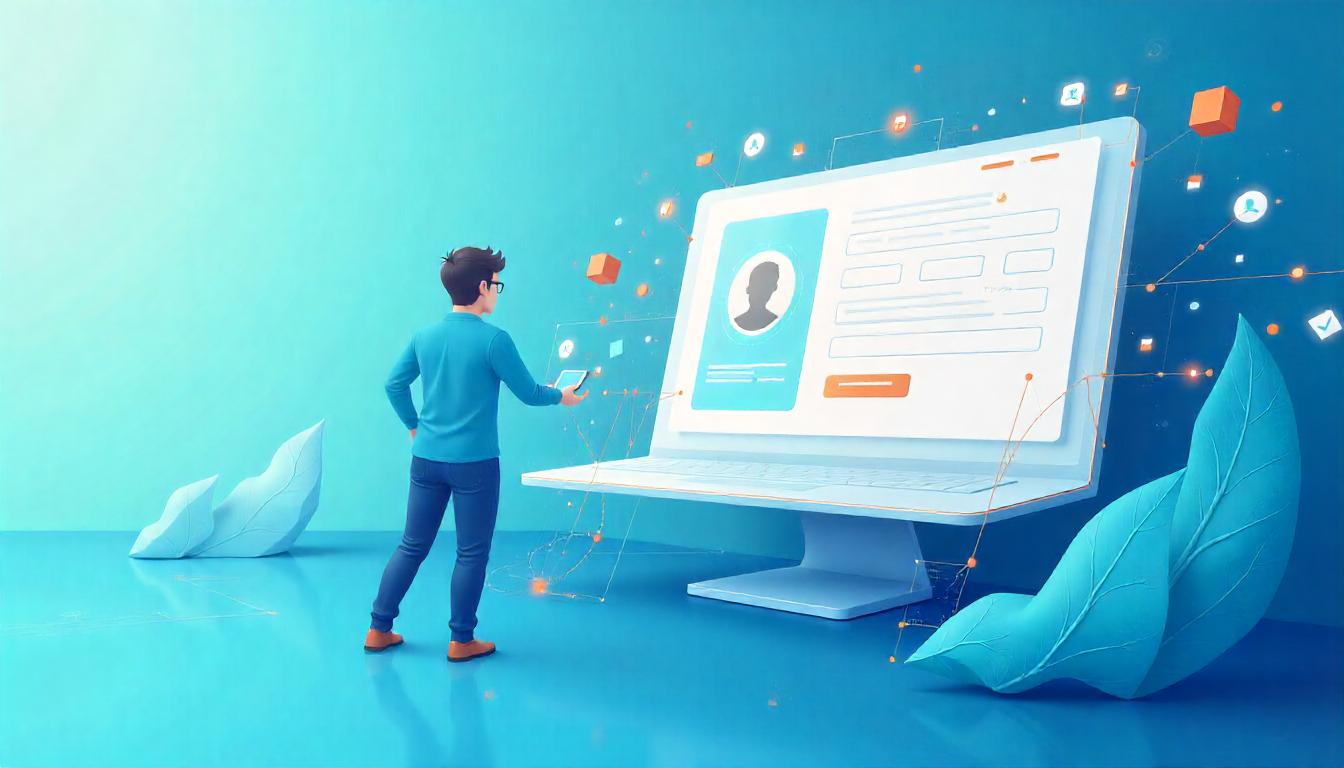
How to Customize the Ship-To Section in Order Summary Checkout in Magento 2.4.7
Magento 2 supports a two-step checkout process, where the Ship-To section appears in the order summary sidebar on the Payment & Review step. This section displays the customer's chosen shipping address from the Shipping step. Clicking the edit icon redirects the user back to the Shipping step for modifications.
Customizing this section allows you to tailor the shipping information display, add custom attributes, or modify the address format to suit your store’s requirements.
Table Of Content
Ship-To Section Overview
The Ship-To section in Magento 2 checkout displays the selected shipping address and method in the order summary sidebar. It is dynamically rendered using JavaScript components and is defined in the checkout_index_index.xml file under the sidebar area.
XML Definition in checkout_index_index.xml
<item name="ship-to" xsi:type="array">
<item name="component" xsi:type="string">Magento_Checkout/js/view/shipping-information/list</item>
<item name="displayArea" xsi:type="string">ship-to</item>
</item>
This XML configuration defines:
- The JavaScript component responsible for rendering the Ship-To section.
- The display area where the component is inserted in the checkout sidebar.
Breakdown of Key Attributes
Attribute | Value | Description |
---|---|---|
Component | Magento_Checkout/js/view/shipping-information/list | The JavaScript component that handles the Ship-To section UI and logic. |
Display Area | ship-to | Specifies where the Ship-To section appears in the checkout sidebar. |
How the Ship-To Section Works
Magento’s Checkout UI Components Flow
Magento 2 checkout is built using Knockout.js-based UI components. The Ship-To section is part of the checkout sidebar and dynamically updates when a customer selects or changes the shipping address.
Rendering Process
Checkout Layout Definition:
- The
checkout_index_index.xml
file registers the Ship-To section inside the sidebar.
JavaScript Component Magento_Checkout/js/view/shipping-information/list
- Loads shipping details and displays them dynamically.
Knockout.js Data Binding
- Updates the shipping address and method in real-time without a full-page reload.
Customizing the Ship-To Section
If you need to modify the Ship-To section, you can create a custom module to extend or override the component.
Override the Default Component
Step 1: Create requirejs-config.js
to Map Your Custom Component
var config = {
config: {
mixins: {
'Magento_Checkout/js/view/shipping-information/list': {
'Vendor_Module/js/view/custom-ship-to': true
}
}
}
};
Step 2: Create a Custom Component (custom-ship-to.js
)
define([
'Magento_Checkout/js/view/shipping-information/list',
'ko'
], function (Component, ko) {
'use strict';
return Component.extend({
initialize: function () {
this._super();
console.log('Custom Ship-To section loaded');
}
});
});
Step 3: Clear Cache and Test the Changes
php bin/magento cache:flush
This method allows you to customize the Ship-To section without modifying core files.
Adding Custom Fields to Ship-To Section
You can add extra information like estimated delivery time or a custom message by modifying the template file.
Modify the Template (shipping-information.html
)
Navigate to:
app/design/frontend/Vendor/theme/Magento_Checkout/web/template/shipping-information.html
Add a new section inside the template:
<div class="custom-message">
<strong>Estimated Delivery:</strong> <!-- Bold label -->
<span data-bind="text: estimatedDelivery"></span> <!-- Knockout.js binding -->
</div>
Then, update the custom-ship-to.js
file to include:
<script>
define([
'Magento_Checkout/js/view/shipping-information/list',
'ko'
], function (Component, ko) {
'use strict';
return Component.extend({
estimatedDelivery: ko.observable('2-4 Business Days'), // Observable for estimated delivery
initialize: function () {
this._super();
console.log('Custom Ship-To section with estimated delivery time loaded');
}
});
});
</script>
Debugging and Common Issues
Issue | Possible Cause | Solution |
---|---|---|
Ship-To section is not displaying | JavaScript error or missing layout configuration | Check the browser console and verify checkout_index_index.xml . |
Data is not updating after address change | Knockout.js binding issue | Ensure that getObservable is used correctly in the component. |
Custom modifications are not applying | RequireJS cache is still loading old files | Run php bin/magento cache:clean && static-content:deploy . |
JavaScript Component Controlling Ship-To Section
Magento 2 uses JavaScript components to manage checkout behavior dynamically. The Ship-To section is responsible for displaying the selected shipping address in the checkout sidebar. It is controlled by the following JavaScript component:
JS Component Path
Magento_Checkout/js/view/shipping-information/address-renderer/default
This component ensures that shipping addresses are correctly rendered in the sidebar during checkout.
Key Responsibilities of the Ship-To Component
Feature | Description |
---|---|
Rendering Address | Displays the selected shipping address in the checkout sidebar. |
Observing Changes | Listens for address updates and dynamically updates the displayed address. |
Knockout.js Bindings | Uses Knockout.js observables to reactively update the address without reloading the page. |
Interaction with Shipping Methods | Works in coordination with the shipping method component to reflect the correct shipping selections. |
How the Ship-To Component Works
Retrieves the Selected Shipping Address
- The component fetches the chosen shipping address from Magento's checkout data.
Observes Address Changes
- Whenever the user modifies the shipping address, Knockout.js bindings update the displayed address dynamically.
Ensures Data Persistence
- The component ensures that the selected shipping address persists throughout the checkout process.
Relevant Code Snippet
This is a simplified version of how Magento initializes the Ship-To component:
<script>
define([
'uiComponent',
'Magento_Checkout/js/model/quote'
], function (Component, quote) {
'use strict';
return Component.extend({
defaults: {
template: 'Magento_Checkout/shipping-information/address-renderer/default'
},
quote: quote, // Assigning quote model
getShippingAddress: function () {
return this.quote.shippingAddress();
}
});
});
</script>
Explanation:
- The
quote
model retrieves the current shipping address. - The Knockout.js binding automatically updates the displayed address when the user selects a new one.
Common Issues & Fixes
Issue | Possible Cause | Solution |
---|---|---|
Ship-To section is empty | JavaScript component not initialized | Ensure that Magento_Checkout/js/view/shipping-information/address-renderer/default is properly loaded. |
Address does not update | Knockout.js binding issue | Check if quote.shippingAddress is correctly observed and refreshed. |
Custom styling not applying | Overridden template not loading | Verify that the custom template path is correct in checkout_index_index.xml . |
Ship-To Section Template Path
Magento 2 dynamically renders the Ship-To section using a Knockout.js-based template system. The default HTML template responsible for displaying the selected shipping address in the checkout sidebar is:
Template Path:
Magento_Checkout/shipping-information/address-renderer/default
How It Works
- This template fetches the
address()
observable, which contains the selected shipping address details. - It binds the shipping address fields dynamically using Knockout.js observables.
- When a customer updates their shipping address, the component re-renders the UI automatically.
Key Elements in the Template
Element | Description |
---|---|
data-bind="text: address().street" |
Displays the street address dynamically. |
data-bind="text: address().city" |
Shows the city of the selected shipping address. |
data-bind="text: address().region" |
Displays the state/region of the address. |
data-bind="text: address().postcode" |
Shows the postal code dynamically. |
data-bind="text: address().telephone" |
Displays the phone number associated with the address. |
Customization Options
To modify the template, override the default template file in your custom theme or module:
Copy the original file from:
vendor/magento/module-checkout/view/frontend/web/template/shipping-information/address-renderer/default.html
Place it inside your custom theme/module at:
app/design/frontend/Vendor/Theme/Magento_Checkout/web/template/shipping-information/address-renderer/default.html
Modify the bindings or layout structure as needed.
Common Issues and Fixes
Issue | Possible Cause | Solution |
---|---|---|
Address does not appear in sidebar | Knockout.js binding issue | Ensure quote.shippingAddress is correctly observed. |
Address formatting is incorrect | Default template is not overridden properly | Verify that the custom template path is correct. |
Changes not reflecting | Cache is still serving old files | Run php bin/magento cache:clean && static-content:deploy . |
Customizing the Ship-To Section
Steps to Modify the Ship-To Section
Override the Default Template
- Create a new
default.html
template inside your custom theme or module. - Modify the address structure to align with your store’s requirements.
Customize default.html
- Add extra address fields, custom attributes, or additional styling.
- Ensure your custom attribute is available in the address object.
Example: Adding a Custom Attribute to the Ship-To Section
Modify the default.html
file:
<div class="shipping-information-content">
<strong class="shipping-information-title">Ship To</strong>
<span data-bind="text: address().firstname"></span>
<span data-bind="text: address().lastname"></span>
<span data-bind="text: address().street"></span>
<span data-bind="text: address().city"></span>
<span data-bind="text: address().region"></span>
<span data-bind="text: address().postcode"></span>
<span data-bind="text: address().telephone"></span>
<span data-bind="text: address().company"></span> <!-- Displays company name -->
<span data-bind="text: address().countryId"></span> <!-- Displays country -->
<span data-bind="text: address().customAttribute"></span> <!-- Custom Attribute -->
</div>
Ensuring customAttribute
Availability
To make sure customAttribute
is available in the address object:
- Extend the
Magento_Checkout/js/view/shipping-information/address-renderer/default
component. - Modify
shipping-information.js
to include the custom attribute.
Example: Extending the View Model
<script>
define([
'Magento_Checkout/js/view/shipping-information'
], function (Component) {
'use strict';
return Component.extend({
defaults: {
template: 'Your_Module/shipping-information'
},
getCustomAttribute: function () {
return this.quote.shippingAddress().customAttribute;
}
});
});
</script>
Tip: Optimize Performance
When adding custom fields, ensure they are indexed properly and use Knockout.js bindings efficiently to avoid unnecessary re-renders in the checkout sidebar.
Advanced Customization: Extending the Ship-To Component
To gain full control over the Ship-To section in Magento 2, you can extend list.js and customize its behavior. This is useful for adding extra fields, modifying the UI, or implementing business-specific logic.
Steps to Extend list.js
1. Create a Custom JavaScript Component
Create a new file in your module:
view/frontend/web/js/view/custom-ship-to.js
<script>
define([
'Magento_Checkout/js/view/shipping-information/list'
], function (Component) {
'use strict';
return Component.extend({
defaults: {
template: 'Vendor_Module/custom-ship-to'
},
/**
* Custom function to add extra processing logic
*/
getCustomField: function () {
if (this.quote.shippingAddress()) {
return this.quote.shippingAddress().customAttribute || 'N/A';
}
return 'N/A';
}
});
});
</script>
This customization:
- Changes the default template for the Ship-To section.
- Adds a new method
getCustomField()
to fetch a custom attribute. - Ensures that
customAttribute
does not break if missing.
2. Override the Component Using RequireJS Mixin
Now, override the default Magento component in requirejs-config.js
.
view/frontend/requirejs-config.js
<script>
var config = {
config: {
mixins: {
'Magento_Checkout/js/view/shipping-information/list': {
'Vendor_Module/js/view/custom-ship-to': true
}
}
}
};
</script>
3. Create a Custom Template
Now, create a custom template to display the new field.
view/frontend/web/template/custom-ship-to.html
<div class="shipping-information-content">
<strong class="shipping-information-title">Ship To</strong>
<span data-bind="text: address().firstname"></span>
<span data-bind="text: address().lastname"></span>
<span data-bind="text: address().street"></span>
<span data-bind="text: address().city"></span>
<span data-bind="text: address().region"></span>
<span data-bind="text: address().postcode"></span>
<span data-bind="text: address().countryId"></span>
<span data-bind="text: address().telephone"></span>
<span data-bind="text: getCustomField()"></span> <!-- Custom Field -->
</div>
Final Steps
Deploy Static Content:
php bin/magento setup:static-content:deploy -f
Flush Cache:
php bin/magento cache:flush
Test the Checkout Page and confirm that the Ship-To section displays the new field.
Tip: Ensure Compatibility with Third-Party Modules
If you are using third-party checkout extensions (e.g., One Step Checkout), test your changes to prevent conflicts. Use console.log(this.quote.shippingAddress());
in custom-ship-to.js
to debug address data dynamically.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Customizing the Ship-To section in Magento 2 provides greater control over how shipping information is displayed and processed during checkout. By understanding the default structure, modifying the JavaScript component, and extending the template, you can enhance the user experience and ensure it aligns with your store’s needs.
Whether you need to add custom fields, observe dynamic updates, or override Magento's default behavior, implementing these steps will ensure a smooth and efficient checkout process. For advanced modifications, leveraging Knockout.js bindings and RequireJS mixins ensures seamless integration without breaking core functionalities.
By following these best practices and debugging efficiently, you can enhance your Magento 2 checkout experience while maintaining flexibility for future updates and third-party extensions.
FAQs
What is the Ship-To section in Magento 2?
The Ship-To section displays the selected shipping address in the checkout sidebar, allowing customers to verify their shipping details before placing an order.
Where is the Ship-To section defined in Magento 2?
The Ship-To section is defined in the checkout_index_index.xml
file under the sidebar area and is controlled by a JavaScript component.
Which JavaScript file controls the Ship-To section?
The Ship-To section is managed by Magento_Checkout/js/view/shipping-information/list
, which handles address rendering and updates.
How do I customize the Ship-To section template?
You can override the default default.html
template in your custom theme or module by modifying the structure and adding custom attributes.
How can I add a custom field to the Ship-To section?
You can extend the default.html
template and modify Magento_Checkout/js/view/shipping-information/list
to include custom attributes.
Why is my Ship-To section not displaying?
Possible causes include JavaScript errors, missing layout configurations, or a caching issue. Check the browser console and verify the checkout_index_index.xml
file.
Why is the address in the Ship-To section not updating?
This can occur due to Knockout.js binding issues. Ensure that quote.shippingAddress
is properly observed and refreshed in the JavaScript component.
How do I extend the Ship-To section using RequireJS mixins?
You can create a custom JavaScript file and configure requirejs-config.js
to override Magento_Checkout/js/view/shipping-information/list
without modifying core files.
What is the role of Knockout.js in the Ship-To section?
Knockout.js handles dynamic updates by binding address fields to observables, ensuring real-time updates without page refreshes.
How do I debug Ship-To section issues?
Check the browser console for JavaScript errors, verify layout configurations, and clear Magento’s cache using php bin/magento cache:clean
.
How do I add a custom CSS style to the Ship-To section?
You can add styles by modifying Magento_Checkout/web/css/source/module/_checkout.less
or by creating a custom CSS file in your theme.
Can I reposition the Ship-To section in the checkout sidebar?
Yes, you can update the checkout_index_index.xml
file and modify the displayArea
attribute to reposition the Ship-To section.
How do I make the Ship-To section compatible with third-party checkout extensions?
Ensure that your customization follows Magento’s best practices, uses RequireJS mixins, and does not conflict with other checkout modifications.