Understanding JavaScript Mixins in Magento 2
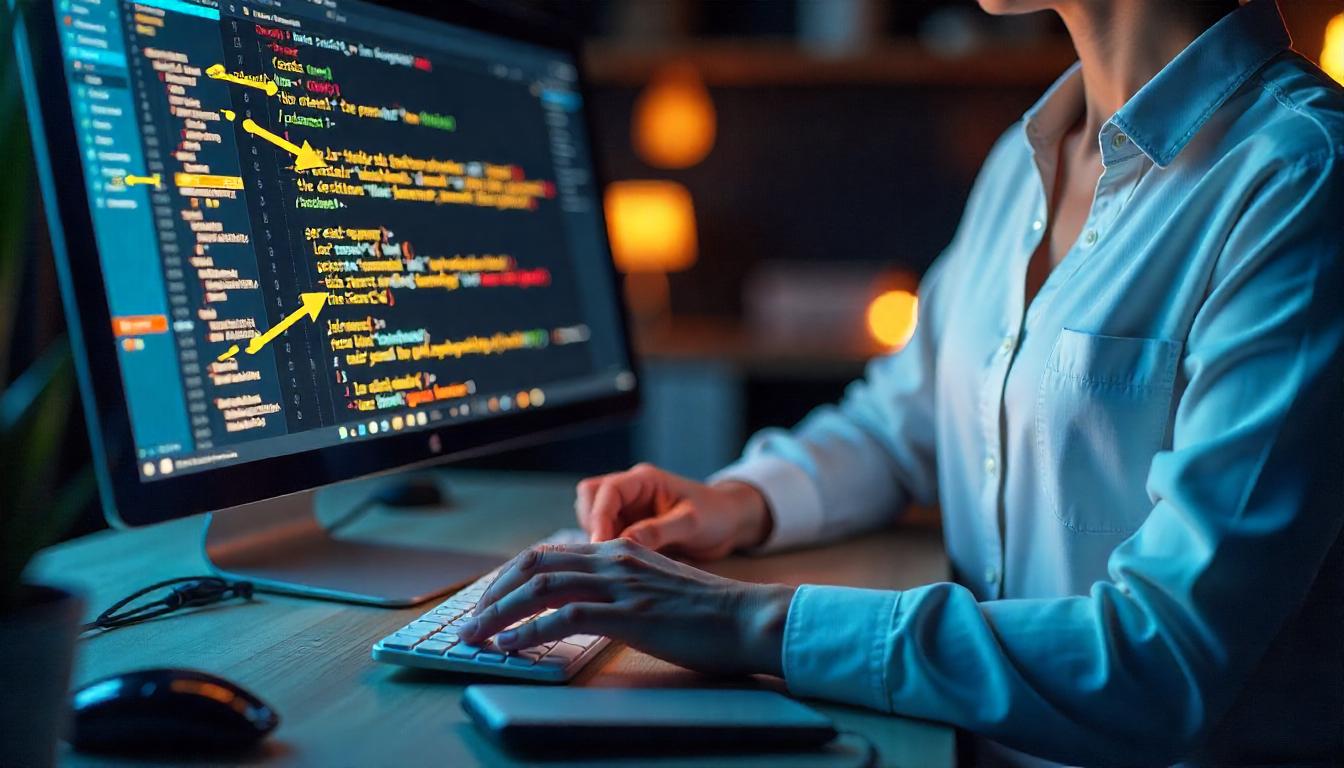
Understanding JavaScript Mixins in Magento 2
JavaScript mixins in Magento 2 allow you to modify or extend core JavaScript functionality without overriding entire files. This approach helps developers customize features while keeping the original code intact, ensuring easier upgrades and maintenance.
Table Of Content
Understanding JavaScript Mixins in Magento 2
In Magento 2, a mixin is a class whose methods can be incorporated into another class without using traditional inheritance. This approach allows you to add or modify methods in existing classes without altering the original code. Mixins are particularly useful in JavaScript for extending or customizing functionality without directly overriding core files.
Overriding the Payment List Template Using mixins.js in Magento 2
To customize the payment list template in Magento 2 using mixins, follow these steps:
Create a requirejs-config.js
File: This configuration file declares the mixin and specifies the target component to override.
var config = {
config: {
mixins: {
'Magento_Checkout/js/view/payment/list': {
'Your_Module/js/view/payment/list-mixin': true
}
}
}
};
In this example, Your_Module/js/view/payment/list-mixin
is the path to your custom mixin file.
Develop the Mixin File: Create the mixin JavaScript file at the specified location. This file will extend the functionality of the target component.
define(['jquery'], function ($) {
'use strict';
return function (PaymentList) {
return PaymentList.extend({
/**
* Returns payment group title
*/
getGroupTitle: function () {
return 'Custom Payment Methods';
}
});
};
});
In this script, the getGroupTitle
method is overridden to return 'Custom Payment Methods' as the payment group title.
Verify the New Column: Check the sales_order table in your database to confirm that the order_country column has been added successfully.
Benefits of Using Mixins
- Non-Intrusive Customization: Mixins allow you to modify or extend functionality without altering core Magento files, preserving upgradability.
- Targeted Enhancements: You can apply mixins to specific components, ensuring precise customization.
- Improved Maintainability: By keeping custom code separate, mixins enhance the maintainability and readability of your codebase.
For a comprehensive guide on using mixins in Magento 2, refer to the official Adobe documentation.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Are JavaScript Mixins in Magento 2?
Mixins in Magento 2 allow you to modify or extend JavaScript components without directly editing core files.
Why Use Mixins Instead of Overriding Core Files?
Mixins provide a non-intrusive way to customize JavaScript components, ensuring better maintainability and compatibility with Magento updates.
How Do I Declare a Mixin in Magento 2?
Create a requirejs-config.js
file in your module's view/frontend
directory and specify the mixin.
var config = {
config: {
mixins: {
'Magento_Checkout/js/view/payment/list': {
'Your_Module/js/view/payment/list-mixin': true
}
}
}
};
How Do I Create a Mixin File?
Create the mixin JavaScript file in your module, for example:
define(['jquery'], function ($) {
'use strict';
return function (PaymentList) {
return PaymentList.extend({
getGroupTitle: function () {
return 'Custom Payment Methods';
}
});
};
});
How Do I Apply the Changes?
Run the following command to clear the cache and apply updates:
php bin/magento cache:flush
Can I Use Mixins for UI Components?
Yes, mixins can modify UI components by extending existing JavaScript classes.
How Can I Debug a Mixin in Magento 2?
Use browser developer tools to inspect overridden methods and check for JavaScript errors in the console.
What If My Mixin Doesn’t Work?
Check the following:
- Ensure the mixin file path is correct.
- Verify
requirejs-config.js
is properly configured. - Flush cache and redeploy static content.
Do Mixins Work with Knockout.js Components?
Yes, you can apply mixins to Knockout.js components in Magento 2.
Where Can I Learn More About Magento 2 Mixins?
Refer to the official Magento developer documentation for an in-depth guide on mixins.