Developing a Magento 2 Plugin for Post-Order Placement Actions
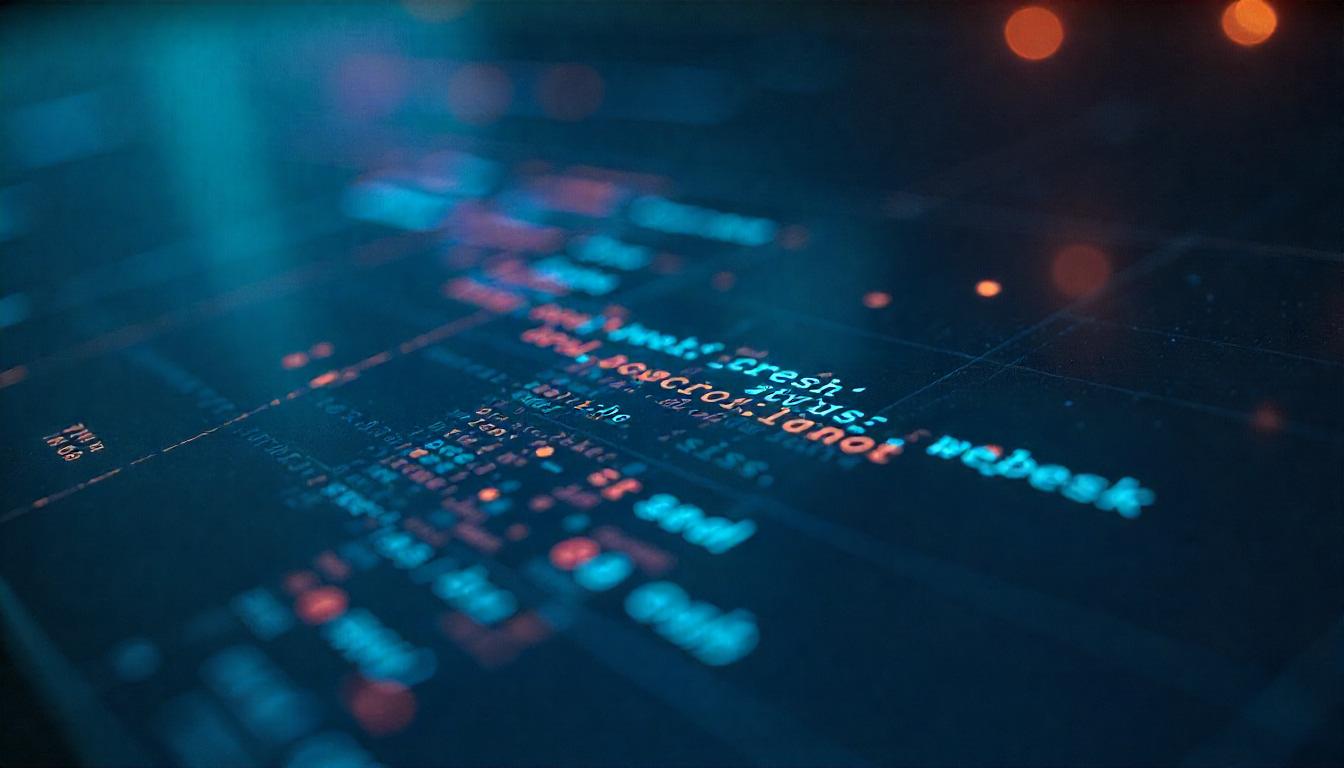
Developing a Magento 2 Plugin for Post-Order Placement Actions
Creating a Magento 2 plugin for post-order placement actions allows you to execute custom logic immediately after an order is placed. By intercepting the place() method of the OrderManagementInterface, you can modify order data, trigger external API calls, or store additional information.
Table Of Content
Developing a Magento 2 Plugin for Post-Order Placement Actions
To execute custom logic immediately after an order is placed in Magento 2, you can create a plugin that intercepts the place()
method of the OrderManagementInterface
. This approach allows you to perform additional operations following the standard order placement process.
Step 1: Define the Plugin in di.xml
Begin by declaring your plugin in the di.xml
file located at app/code/YourVendor/YourModule/etc/di.xml
:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Sales\Api\OrderManagementInterface">
<plugin name="after_place_order_plugin"
type="YourVendor\YourModule\Plugin\OrderManagementPlugin"/>
</type>
</config>
This configuration tells Magento to use your plugin after the place()
method of the OrderManagementInterface
is called.
Step 2: Implement the Plugin Class
Next, create the plugin class at app/code/YourVendor/YourModule/Plugin/OrderManagementPlugin.php
<:
<?php
namespace YourVendor\YourModule\Plugin;
use Magento\Sales\Api\Data\OrderInterface;
use Magento\Sales\Api\OrderManagementInterface;
class OrderManagementPlugin
{
/**
* Execute custom logic after an order is placed.
*
* @param OrderManagementInterface $subject
* @param OrderInterface $result
* @return OrderInterface
*/
public function afterPlace(
OrderManagementInterface $subject,
OrderInterface $result
) {
$orderId = $result->getEntityId();
if ($orderId) {
// Insert your custom logic here
}
return $result;
}
}
In this method, $result
represents the placed order object, from which you can retrieve the order ID and other details to implement your custom functionality.
Alternative Approach: Using Observers
If you prefer using observers, Magento 2 provides events that you can listen to after an order is placed. One such event is checkout_submit_all_after
. To utilize this event, define it in your module's events.xml file:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="checkout_submit_all_after">
<observer name="your_observer_name"
instance="YourVendor\YourModule\Observer\OrderSuccessObserver"/>
</event>
</config>
Then, implement the observer class at app/code/YourVendor/YourModule/Observer/OrderSuccessObserver.php
:
<?php
namespace YourVendor\YourModule\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
class OrderSuccessObserver implements ObserverInterface
{
/**
* Execute observer.
*
* @param Observer $observer
* @return void
*/
public function execute(Observer $observer)
{
$order = $observer->getEvent()->getOrder();
if ($order) {
$orderId = $order->getEntityId();
// Insert your custom logic here
}
}
}
This observer will trigger after the order submission process, allowing you to add your custom logic accordingly.
Key Considerations
- Choosing Between Plugins and Observers: Plugins are suitable for modifying or extending specific class behaviors, while observers are ideal for reacting to events dispatched by Magento. Select the approach that best fits your requirements.
- Accessing Order Data: Ensure that the order data you need is available at the point where your plugin or observer executes. For instance, some order information might not be fully populated during certain events.
- Performance Implications: Be mindful of the performance impact of your custom logic, especially if it involves external API calls or complex computations.
By following these steps, you can effectively implement custom actions that execute immediately after an order is placed in Magento 2, tailoring the platform's functionality to your business needs.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Do I Execute Custom Logic After an Order Is Placed in Magento 2?
You can create a plugin to intercept the place()
method of the OrderManagementInterface
.
app/code/YourVendor/YourModule/etc/di.xml
How Can I Define the Plugin in di.xml?
Add the following configuration to your module’s di.xml
:
<type name="Magento\Sales\Api\OrderManagementInterface">
<plugin name="after_place_order_plugin"
type="YourVendor\YourModule\Plugin\OrderManagementPlugin"/>
</type>
Where Should I Implement the Plugin Class?
Create the plugin class in the following path:
app/code/YourVendor/YourModule/Plugin/OrderManagementPlugin.php
What Should Be in OrderManagementPlugin.php?
Add the following method to execute custom logic after an order is placed:
public function afterPlace(
OrderManagementInterface $subject,
OrderInterface $result
) {
$orderId = $result->getEntityId();
if ($orderId) {
// Custom logic here
}
return $result;
}
Can I Use an Observer Instead of a Plugin?
Yes, you can use the checkout_submit_all_after
event.
app/code/YourVendor/YourModule/etc/events.xml
How Do I Define an Observer in events.xml?
Add the following configuration:
<event name="checkout_submit_all_after">
<observer name="your_observer_name"
instance="YourVendor\YourModule\Observer\OrderSuccessObserver"/>
</event>
Where Should I Place My Custom Observer Class?
Create it in the following directory:
app/code/YourVendor/YourModule/Observer/OrderSuccessObserver.php
What Should Be in OrderSuccessObserver.php?
Implement the observer logic as follows:
public function execute(Observer $observer)
{
$order = $observer->getEvent()->getOrder();
if ($order) {
$orderId = $order->getEntityId();
// Custom logic here
}
}
How Can I Clear Cache After Adding My Plugin or Observer?
Run the following commands to refresh Magento's cache:
php bin/magento cache:clean
php bin/magento cache:flush
What If My Custom Logic Doesn’t Work?
Check the following:
- Ensure your plugin is correctly defined in
di.xml
. - Verify the observer is registered in
events.xml
. - Run
php bin/magento setup:upgrade
if working within a module. - Check file permissions:
chmod -R 775 app/code/YourVendor/YourModule