How to Get Review Collection by ID in Magento 2.4.7
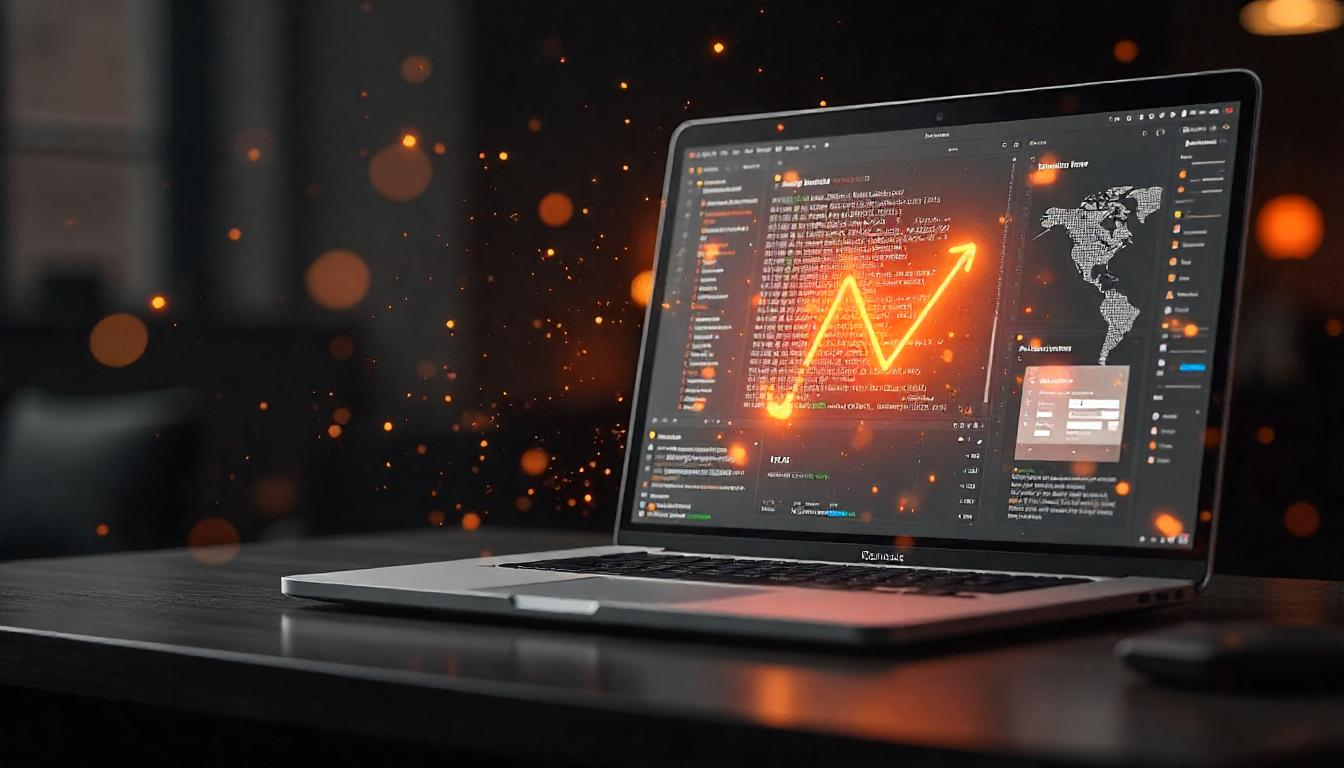
How to Get Review Collection by ID in Magento 2.4.7
Retrieving product review details by review ID in Magento 2.4.7 is crucial for displaying or processing customer feedback efficiently. This guide explains how to fetch review details using Magento\Review\Model\ReviewFactory
.
Table Of Content
Magento 2 Review Management: Retrieving Review Details by ID
Magento 2 provides a robust review system that allows customers to leave reviews on product pages. These reviews contribute to customer engagement and trust. However, retrieving specific review details—such as rating, customer information, and status—requires efficient handling through Magento’s ReviewFactory and Review Model.
This guide explains how to retrieve review details using ReviewFactory, best practices for performance optimization, and security considerations.
How to Retrieve Review Details by Review ID
Using ReviewFactory to Load Review Data
Magento 2 provides ReviewFactory, which enables developers to fetch specific reviews by their unique review ID. The following PHP code demonstrates how to achieve this:
<?php
namespace Vendor\Module\Model;
use Magento\Review\Model\ReviewFactory;
class ReviewLoader
{
protected $reviewFactory;
public function __construct(
ReviewFactory $reviewFactory
) {
$this->reviewFactory = $reviewFactory;
}
/**
* Load Review by ID
*
* @param int $reviewId
* @return \Magento\Review\Model\Review
*/
public function getReviewById($reviewId)
{
$review = $this->reviewFactory->create();
$review->load($reviewId);
return $review->getData();
}
}
Calling the Function
To retrieve a review in a template file or another class, use:
<?php
$reviewData = $this->getReviewById(10); // Replace 10 with the actual review ID
print_r($reviewData);
?>
Understanding Magento 2 Review Data Structure
In Magento 2, product reviews are stored in multiple database tables, each holding specific details about reviews, ratings, and status. When you fetch a review using ReviewFactory, it retrieves a structured dataset containing various fields. Understanding these fields is crucial for filtering, displaying, and managing reviews efficiently.
Key Review Data Fields
The following table outlines the core fields retrieved when loading a review in Magento 2:
Field Name | Description |
---|---|
review_id | Unique identifier for the review. |
entity_id | The type of entity being reviewed (e.g., product, customer). |
entity_pk_value | The actual product or customer ID associated with the review. |
title | The review title submitted by the customer. |
detail | The full review text written by the customer. |
nickname | The name of the reviewer (either registered customer or guest). |
customer_id | The ID of the registered customer who submitted the review. |
store_id | The store view where the review was submitted (useful for multi-store setups). |
status_id | The current status of the review (Pending, Approved, or Rejected). |
created_at | Timestamp indicating when the review was submitted. |
rating_votes | The rating values associated with the review (e.g., star ratings). |
Magento also maintains separate tables to store aggregated review data, status information, and store-level associations.
Review Database Tables in Magento 2
Magento stores review data across multiple tables, each serving a specific purpose.
Table Name | Purpose |
---|---|
review | Stores the basic review details (e.g., rating, title, customer ID). |
review_detail | Contains the full review text and metadata like reviewer name and store ID. |
review_status | Holds different review status values (Pending, Approved, Rejected). |
review_entity | Defines the type of entity being reviewed (product, customer, etc.). |
review_store | Associates reviews with store views in a multi-store Magento setup. |
review_aggregate | Stores pre-calculated rating summaries for performance optimization. |
customer_review | Maps reviews to registered customers for easy tracking. |
review_votes | Stores customer reactions to reviews (e.g., upvotes, downvotes). |
Review Status in Magento 2
Magento categorizes reviews based on their approval status. This helps manage which reviews appear publicly.
Status ID | Status Name | Description |
---|---|---|
1 | Pending | The review is awaiting admin approval. |
2 | Approved | The review has been published and is visible to customers. |
3 | Rejected | The review was rejected due to spam, policy violations, or inappropriate content. |
Example: Fetching Only Approved Reviews
<?php
$reviewCollection = $this->reviewFactory->create()->getCollection()
->addEntityFilter($productId, 'product')
->addStatusFilter(2); // Fetch only approved reviews
->setDateOrder();
?>
Filtering and Sorting Review Data
Magento’s Review Model provides built-in methods for filtering and sorting reviews efficiently. These methods help refine the review collection based on specific criteria.
Method | Description |
---|---|
addStatusFilter($status) |
Retrieve reviews based on their status (Pending, Approved, Rejected). |
addEntityFilter($entityId, $entityType) |
Fetch reviews for a specific product or customer. |
addStoreFilter($storeId) |
Get reviews for a specific store view (useful in multi-store setups). |
setDateOrder() |
Order reviews based on the date they were submitted. |
addRateVotes() |
Fetch rating information along with review details. |
Example: Retrieving Reviews for a Specific Store and Sorting by Date
<?php
$storeId = 1; // Example store ID
$reviewCollection = $this->reviewFactory->create()->getCollection()
->addStoreFilter($storeId)
->setDateOrder(); // Orders reviews based on submission date
?>
Performance Optimization for Review Retrieval
When dealing with large review datasets, optimizing performance is essential to avoid slow queries and high database load.
Best Practices for Performance Optimization
Strategy | Benefit |
---|---|
Lazy Loading | Loads review details only when needed, reducing unnecessary queries. |
Indexing | Improves retrieval speed by pre-calculating review summaries. |
Pagination | Prevents loading too many reviews at once, improving UI performance. |
Caching | Stores frequently accessed reviews in cache to minimize database queries. |
Example: Implementing Pagination
$reviewCollection->setPageSize(10); // Load only 10 reviews per request
Best Practices for Handling Reviews
Managing reviews effectively requires validation, security, and error handling.
1. Data Validation
- Always validate input data before processing it.
- Ensure that review IDs exist before querying them.
2. Security & Access Control
- Restrict review data access to authorized admin users using Magento ACL (Access Control Lists).
- Prevent unauthorized data exposure by securing API calls and restricting direct database access.
3. Error Handling
Common Issue | Possible Cause | Solution |
---|---|---|
NoSuchEntityException | Invalid or non-existent review ID | Validate review existence before querying. |
Review returns empty data | Review might be assigned to a different store view | Use addStoreFilter($storeId) to get the correct data. |
Review is not visible on frontend | Review status is Pending or Rejected | Ensure the status is set to Approved (status_id = 2 ). |
Retrieving and managing reviews in Magento 2 requires a structured approach to querying, filtering, and security controls. By leveraging ReviewFactory, performance optimizations, and best practices, you can efficiently manage reviews while maintaining fast performance and data security.
Steps to Retrieve Review Details by ID in Magento 2
To fetch specific review details based on the review ID, follow these steps:
1. Inject the ReviewFactory into Your Class
Magento 2 follows Dependency Injection (DI) principles, so injecting ReviewFactory
into your custom model or class ensures proper object instantiation.
2. Load the Review Using the Review ID
Once injected, use ReviewFactory
to load the review data.
3. Retrieve Required Review Fields
Extract key fields such as review_id
, title
, nickname
, detail
, status_id
, and others to display or process further.
Magento 2 Review Data Retrieval Code
Below is a well-structured Magento 2 model for fetching review data efficiently:
<?php
namespace Jesadiya\Review\Model;
use Magento\Review\Model\ReviewFactory;
use Magento\Framework\Exception\LocalizedException;
class ReviewData
{
/**
* @var ReviewFactory
*/
private $reviewFactory;
/**
* Constructor
*
* @param ReviewFactory $reviewFactory
*/
public function __construct(ReviewFactory $reviewFactory)
{
$this->reviewFactory = $reviewFactory;
}
/**
* Get Review Data by ID
*
* @param int $reviewId
* @return \Magento\Review\Model\Review
* @throws LocalizedException
*/
public function getReviewData($reviewId)
{
try {
$review = $this->reviewFactory->create()->load($reviewId);
if (!$review->getId()) {
throw new LocalizedException(__('Review not found.'));
}
return $review;
} catch (LocalizedException $exception) {
throw new LocalizedException(__($exception->getMessage()));
}
}
}
Calling the Function in a Template or PHP Class
To fetch and display the review details, call the function in your template or another PHP class as follows:
$reviewModel = $this->getReviewData(1);
echo '<pre>' . print_r($reviewModel->getData(), true) . '</pre>';
Sample Output of Review Data Retrieval
Review Attribute | Value |
---|---|
Review ID | 1 |
Created At | 2025-03-03 12:00:00 |
Entity ID | 1 |
Entity PK Value | 1 |
Status ID | 1 (Pending) |
Store ID | 1 |
Title | "Great Quality" |
Detail | "The product is excellent and meets expectations." |
Nickname | "JohnDoe" |
Customer ID | 25 |
Stores | [1,2] |
Additional Enhancements
Filtering Reviews Based on Status and Store View
<?php
$reviewCollection = $this->reviewFactory->create()->getCollection()
->addFieldToFilter('status_id', 2) // Fetch only approved reviews
->addFieldToFilter('store_id', 1) // Fetch reviews for store ID 1
->setOrder('created_at', 'DESC'); // Sort by latest reviews
?>
Tips for Working with Review Data
- Ensure Review ID Exists: Always check if the review exists before retrieving data to avoid errors.
- Use Dependency Injection: Inject
ReviewFactory
instead of usingObjectManager
for better performance and maintainability. - Handle Exceptions: Wrap the code in a
try-catch
block to handle missing or invalid review IDs. - Filter by Status: Use
getStatusId()
to filter approved or pending reviews.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Effectively retrieving and managing product reviews in Magento 2 is essential for enhancing customer trust and improving the shopping experience. By leveraging ReviewFactory
, applying filters for store views and statuses, and handling exceptions properly, you ensure accurate and efficient data retrieval. Implementing best practices like lazy loading, caching, and indexing further optimizes performance, reducing unnecessary database queries and enhancing UI responsiveness.
Additionally, understanding common issues—such as incorrect store filters or unapproved reviews—helps in troubleshooting and maintaining a seamless review system. By structuring your code efficiently and following Magento’s best practices, you can streamline review management, improve store performance, and provide valuable insights to both customers and administrators.
With a well-optimized review retrieval system, your Magento 2 store will not only enhance user engagement but also boost SEO rankings, build brand credibility, and drive higher conversion rates.
FAQs
What are pending reviews in Magento 2?
Pending reviews are customer reviews that have been submitted but have not yet been approved by the site administrator. They remain hidden until moderated.
How can I retrieve a review by its ID in Magento 2?
You can retrieve a review by its ID using ReviewFactory in Magento 2. Load the review model and fetch the details by calling the load($reviewId)
method.
What does the status_id field indicate in review data?
The status_id
field represents the review's approval state. Common values include 1 (Pending), 2 (Approved), and 3 (Rejected).
Why is my review not visible on the frontend?
If your review is not visible, check if its status is set to Approved (status_id = 2
). Also, ensure it is associated with the correct store view.
How do I filter reviews by store view in Magento 2?
You can use the addStoreFilter($storeId)
method in the review collection to fetch reviews specific to a store view.
What is the purpose of the entity_id field in reviews?
The entity_id
field determines the type of entity being reviewed, such as a product or customer, allowing proper review association.
Can I retrieve review ratings along with review details?
Yes, you can use the addRateVotes()
method to include rating information when fetching review details.
How does Magento 2 optimize review retrieval performance?
Magento 2 uses caching, indexing, pagination, and lazy loading to optimize review retrieval and minimize database load.
How do I check if a review exists before loading it?
Before loading a review, you can check if it exists by calling getId()
on the loaded review model to avoid exceptions.
What should I do if I get a NoSuchEntityException error?
The error occurs when the review ID is invalid or does not exist. Validate the review ID before querying to prevent this issue.
How do I sort reviews by date in Magento 2?
You can use the setDateOrder()
method in the review collection to order reviews by their submission date.
Can I restrict review management access to certain admins?
Yes, you can use Magento's ACL (Access Control Lists) to restrict review management access to specific admin roles.
How can I improve review loading speed for large stores?
Implement pagination, indexing, caching, and lazy loading techniques to optimize review loading speed and reduce database queries.