How to Retrieve Product Categories in Magento 2: A Complete Guide
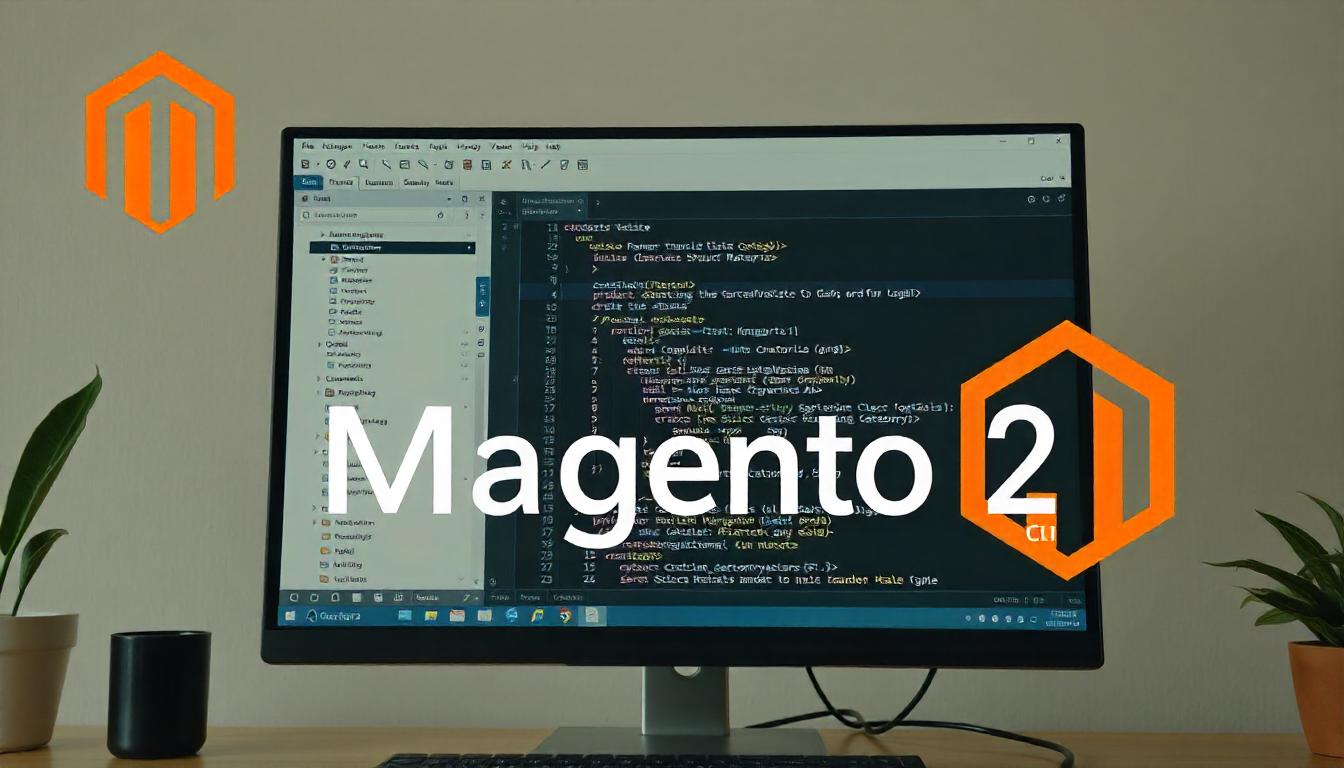
How to Retrieve Product Categories in Magento 2: A Complete Guide
Retrieving product categories in Magento 2 programmatically can help you customize and manage category data effectively. Here are different methods you can use, based on best practices:
Table Of Content
Retrieving Magento 2 Product Categories: A Simple Guide
Efficiently managing product categories in Magento 2 is essential for smooth catalog operations. Let’s dive into how to get product categories from a product object using Magento 2. The examples below include code snippets that can help you achieve this quickly. This approach avoids common errors users have faced when improperly retrieving categories.
Retrieving Categories for a Single Product
In the constructor, initialize the product factory:
protected $_productFactory;
public function __construct(
\Magento\Catalog\Model\ProductFactory $productFactory
) {
$this->_productFactory = $productFactory;
}
public function getProductCategories($pid)
{
$product = $this->_productFactory->create()->load($pid);
return $product->getCategoryIds(); // Returns an array of category IDs
}
This function returns an array of category IDs associated with the product. Ensure the $pid is valid to avoid null results.
Fetching Categories from a Product Collection
If you need categories for multiple products in a collection, use the following method:
protected $_productCollectionFactory;
protected $_productloader;
public function __construct(
\Magento\Catalog\Model\ResourceModel\Product\CollectionFactory $productCollectionFactory,
\Magento\Catalog\Model\ProductFactory $productloader
) {
$this->_productCollectionFactory = $productCollectionFactory;
$this->_productloader = $productloader;
}
Here’s the method to retrieve categories for all products in the collection:
Key Points to Remember
- Avoid Null Results: Ensure product IDs are valid and products exist in the catalog.
- Performance Optimization: Loading product objects in a loop can be resource-intensive. For larger collections, consider custom queries to improve efficiency.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Product Categories for a Single Product?
To get categories for a single product in Magento 2, use the getCategoryIds()
method on a product object. For example:
$product = $this->_productFactory->create()->load($pid); $categories = $product->getCategoryIds();
This returns an array of category IDs assigned to the product.
How Do I Retrieve Categories from a Product Collection?
For a collection of products, you can loop through the products and retrieve their categories:
$productCollection = $this->_productCollectionFactory->create(); $catIds = []; foreach ($productCollection as $product) { $loadedProduct = $this->_productloader->create()->load($product->getId()); $catIds = array_merge($catIds, $loadedProduct->getCategoryIds()); } $finalCatIds = array_unique($catIds);
This provides a unique list of all category IDs in the collection.
What Are the Key Steps to Avoid Errors When Retrieving Categories?
To avoid errors, ensure the following:
- The product ID is valid and exists in the catalog.
- The field
category_ids
is properly defined in the product entity. - Performance is optimized for large collections by limiting product loading in loops.
How Do I Optimize Performance When Retrieving Categories?
Loading product objects in a loop can slow down performance. For larger catalogs, consider these approaches:
- Use direct SQL queries for bulk category retrieval.
- Filter collections to only include necessary products.
- Leverage caching for frequently accessed data.
Can I Use Custom Logic for Retrieving Categories?
Yes, you can implement custom logic for specific use cases. For instance, to retrieve categories by specific product attributes, modify the collection filter:
$productCollection = $this->_productCollectionFactory->create() ->addFieldToFilter('attribute_code', ['eq' => 'value']);
This approach helps refine the results based on your requirements.
What Are Common Challenges in Retrieving Categories?
Challenges include:
- Missing or invalid product IDs.
- Loading performance issues with large collections.
- Incorrect or outdated field mappings.
Double-check your setup and test code thoroughly to resolve these issues.
What Is the Output of These Methods?
Both methods return an array of category IDs. The single product method focuses on one product, while the collection method aggregates unique category IDs across multiple products.