Image Resizing in Magento 2: Techniques and Methods
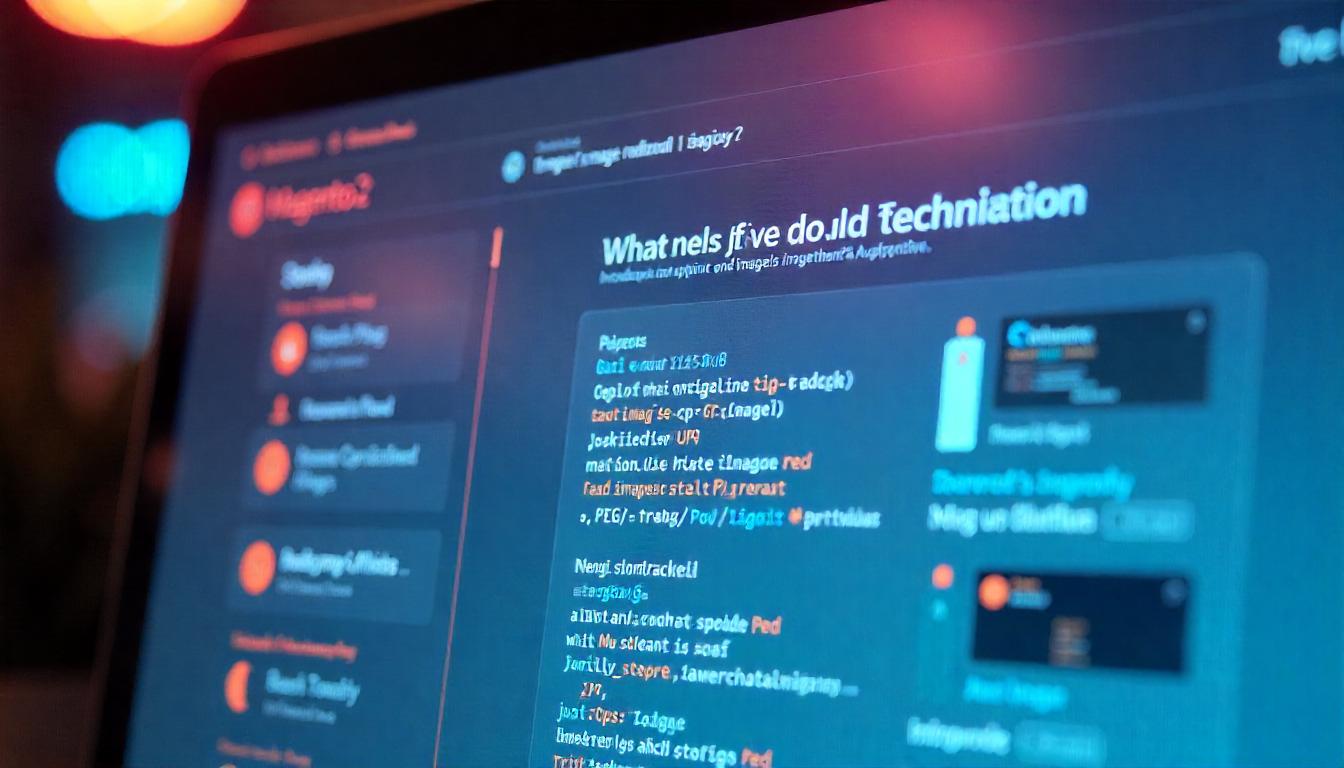
Image Resizing in Magento 2: Techniques and Methods
You can resize images directly in Magento 2 template files (e.g., .phtml files), allowing you to specify unique dimensions for product images or other visual assets. Use the following code structure to set custom dimensions, maintaining the aspect ratio for optimal display:
Table Of Content
How to Resize an Image in Your Custom Magento 2 Module
Resizing images in a custom Magento 2 module is straightforward once you know the right classes to use. We rely on the Filesystem class for accessing the file system and the Image\AdapterFactory class for handling image manipulation.
Step-by-Step Guide to Resize Images
To begin, you’ll need to create a method for resizing the image in your block or helper class. Here's an example where the method is created within the block:
protected $_filesystem;
protected $_imageFactory;
public function __construct(
\Magento\Framework\Filesystem $filesystem,
\Magento\Framework\Image\AdapterFactory $imageFactory
) {
$this->_filesystem = $filesystem;
$this->_imageFactory = $imageFactory;
}
public function resize($image, $width = null, $height = null)
{
$absolutePath = $this->_filesystem-> getDirectoryRead(\Magento\Framework\App\Filesystem\DirectoryList::MEDIA)
->getAbsolutePath('helloworld/images/') . $image;
$imageResized = $this->_filesystem->getDirectoryRead(\Magento\Framework\App\Filesystem\DirectoryList::MEDIA)
->getAbsolutePath('resized/' . $width . '/') . $image;
$imageResize = $this->_imageFactory->create();
$imageResize->open($absolutePath);
$imageResize->constrainOnly(true);
$imageResize->keepTransparency(true);
$imageResize->keepFrame(false);
$imageResize->keepAspectRatio(true);
$imageResize->resize($width, $height);
$destination = $imageResized;
$imageResize->save($destination);
$resizedURL = $this->_storeManager->getStore()->getBaseUrl(\Magento\Framework\UrlInterface::URL_TYPE_MEDIA) . 'resized/' . $width . '/' . $image;
return $resizedURL;
}
Calling the Resize Method in PHTML
Once the method is in place, you can easily call it from your .phtml file:
$block->resize('test.jpg', 500, 400);
Key Details to Keep in Mind
- Filesystem Handling: The Filesystem class is crucial for obtaining the absolute path of images stored in the media directory. It ensures you work with the correct file path.
- Image Resizing: The Image\AdapterFactory is used to resize the image, maintaining aspects like transparency and aspect ratio. You can specify the width and height, and the image will be resized accordingly.
- Resized Image Location: The resized image is saved in the resized/ folder and can be accessed via a URL, which is returned after the image is processed.
Summary of the Process
Step | Action |
---|---|
1. Initialize Classes | Use Filesystem and Image\AdapterFactory for image handling. |
2. Create Resize Method | Define the resize method in your block or helper class. |
3. Call Method in PHTML | Call the method using $block->resize('image.jpg', width, height);. |
4. Save Resized Image | The resized image is saved in a new folder and accessible via URL. |
By following these steps, you can resize images effectively in your custom Magento 2 module. This method is both flexible and efficient for managing image sizes in various parts of your store.
FAQs
What Is Image Resizing in Magento 2?
Image resizing in Magento 2 refers to the process of adjusting images to specific dimensions, which can optimize page load times and improve the user experience. Magento provides tools to programmatically resize images using its Filesystem and Image\AdapterFactory classes.
Why Is Image Resizing Important for My Magento Store?
Resizing images helps reduce file sizes without compromising on visual quality. This leads to faster loading times, which is crucial for improving SEO rankings and user engagement on your Magento store.
How Can I Resize Images in My Custom Magento Module?
In Magento 2, you can resize images by creating a method in a block or helper, using the Filesystem and AdapterFactory classes to access and modify the image dimensions. The resized images are saved to a new directory for easy access and use.
What Classes Are Used for Image Resizing in Magento 2?
Magento 2 uses the \Magento\Framework\Filesystem class for managing file paths and the \Magento\Framework\Image\AdapterFactory class to perform the image manipulation. These classes work together to load, resize, and save images in Magento modules.
Can I Resize Images Using Any Magento Version?
Image resizing techniques apply to all Magento 2 versions, but certain methods or features may vary between versions. Always ensure your Magento version supports the necessary classes and functionality for image manipulation.
What Should I Consider When Resizing Images for My Magento Store?
Before resizing, consider the image quality, file size, and dimensions to balance aesthetics and performance. It's also important to check whether your resized images maintain their transparency and aspect ratio to ensure they display correctly on the site.
How Do I Handle Different Image Resizing Requirements for My Magento Store?
Magento allows you to customize the resizing process for different image types (e.g., product images, category images) by specifying width and height parameters. You can create a flexible resizing function in your custom module to handle multiple image types efficiently.
Where Can I Find More Information on Resizing Images in Magento 2?
For in-depth tutorials and resources, check out Magento's official documentation, developer blogs, and community forums. These resources provide detailed instructions on how to implement image resizing techniques effectively in your store.