Step-by-Step Guide to Creating a Product Programmatically in Magento 2
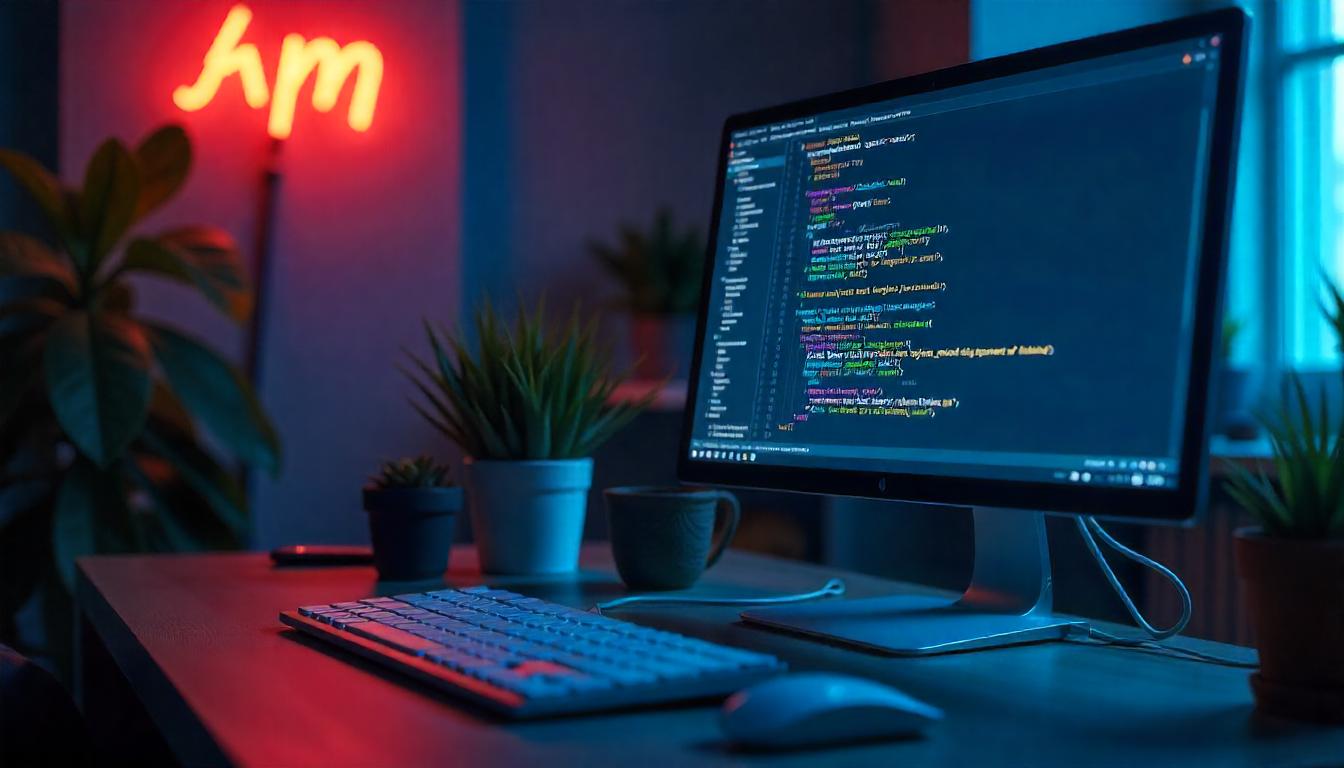
Step-by-Step Guide to Creating a Product Programmatically in Magento 2
If you're looking to create products in Magento 2 programmatically, follow these instructions to streamline your catalog setup. This method is efficient for adding multiple products without manually inputting data through the admin panel, making it ideal for larger eCommerce sites.
Table Of Content
Building a New Product in Magento with Factory Method
This method provides a practical way to create a product programmatically in Magento using the Factory Method. Magento’s ProductFactory enables creating products dynamically in code, which can be particularly useful for automating product creation and updates. Let’s look at how you can create a simple product with specific attributes and configuration settings.
Code Walkthrough:
Below is a sample code using Magento's ProductFactory class to generate a new product. We’ve ensured essential product properties are set, like SKU, price, and stock availability, with room to expand settings as needed.
Code Example:
Magento 2 Product Creator Class
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Model\ProductFactory;
class ProductCreator
{
protected $_productLoader;
public function __construct(ProductFactory $productLoader)
{
$this->_productLoader = $productLoader;
}
public function createProduct()
{
$_product = $this->_productLoader->create();
$_product->setName('Test Product'); // Set Product Name
$_product->setTypeId('simple'); // Define as Simple Product
$_product->setAttributeSetId(4); // Set Attribute Set ID
$_product->setSku('test-SKU'); // Set SKU
$_product->setWebsiteIds([1]); // Set Website IDs
$_product->setVisibility(4); // Set Visibility
$_product->setPrice(400); // Set Price
$_product->setImage('/simpleproduct/test.jpg');
$_product->setSmallImage('/simpleproduct/test.jpg');
$_product->setThumbnail('/simpleproduct/test.jpg');
$_product->setStockData([
'use_config_manage_stock' => 0,
'manage_stock' => 1,
'min_sale_qty' => 1,
'max_sale_qty' => 2,
'is_in_stock' => 1,
'qty' => 1000
]);
$_product->save();
echo "Product ID: " . $_product->getId();
}
}
Key Variables Explained:
Variable
Description
$this->_productloader
Product loader object
setName('Test Product')
Name assigned to the new product
setTypeId('simple')
Sets product type to simple
setAttributeSetId(4)
Attribute set ID (use appropriate ID)
Effective Product Updates Using Magento 2's Factory Pattern
Variable | Description |
---|---|
$this->_productloader | Product loader object |
setName('Test Product') | Name assigned to the new product |
setTypeId('simple') | Sets product type to simple |
setAttributeSetId(4) | Attribute set ID (use appropriate ID) |
Effective Product Updates Using Magento 2's Factory Pattern
In Magento 2, the Factory Method is a design pattern that creates instances of a class dynamically. By using factories, you simplify object management, enhancing flexibility and maintainability in your code. When updating product attributes, the Factory Pattern allows you to instantiate and modify objects without manually managing object dependencies—a best practice in Magento development.
Example Code with Factory Implementation
PHP Code Example
protected $_product;
public function __construct(
\Magento\Catalog\Model\ProductFactory $_product
) {
$this->_productloader = $_productloader;
}
public function updateProduct()
{
$_product = $this->_productloader->create()->load(2047);
$_product->setName('First Test Product Updated');
$_product->save();
}
In this example:
- Dependency Injection: The ProductFactory is injected through the constructor, enhancing code flexibility and adhering to Magento’s DI approach.
- Product Loading and Validation: Before making updates, it verifies that the product ID exists to prevent errors.
- Exception Handling: Errors during the save process are caught and displayed, providing clear feedback for debugging.
Step-by-Step Code Example
Instead of the Object Manager, we’ll use the ProductRepositoryInterface. This method follows Magento’s best practices, making code easier to manage and safer for long-term use. Below is an example of updating a product name:
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Does It Mean to Create a Product Programmatically in Magento 2?
Creating a product programmatically in Magento 2 means using code to automate the addition of products directly into the store without manual input. This approach is helpful for bulk imports or for custom modules that need to create products automatically.
Why Would I Need to Programmatically Create Products in Magento 2?
Programmatically creating products can save time and reduce errors when handling large inventories or complex product attributes. It’s ideal for stores needing frequent product updates, bulk additions, or integrations with other systems that add products dynamically.
Which Classes and Interfaces Are Essential for Product Creation?
To create products programmatically in Magento 2, you’ll primarily use the `ProductFactory` and `ProductRepositoryInterface` classes. These enable product instantiation, attribute setting, and saving the product to the database efficiently.
How Do I Set Basic Product Attributes Programmatically?
To set basic attributes, use methods like `setName()`, `setSku()`, and `setPrice()` within the product object. Each method allows you to define the corresponding attribute directly within the code.
Can I Set Custom Attributes When Creating Products Programmatically?
Yes, Magento 2 allows you to set custom attributes programmatically. Use the `setCustomAttribute()` method, specifying the attribute code and value to customize each product.
How Do I Handle Product Images When Creating Products Programmatically?
Product images can be added by uploading them to the media gallery programmatically. Use the Magento `ImageProcessor` class to upload, resize, and associate images with a product efficiently.
What Are Common Errors When Creating Products Programmatically?
Common issues include missing required attributes, validation errors, or file permission issues for image uploads. It’s essential to check each attribute and ensure file permissions are correctly configured to avoid errors.
Where Can I Find Additional Resources on Programmatically Creating Products?
Check Magento's official documentation, Magento DevDocs, and developer forums for in-depth guidance and troubleshooting tips on creating products programmatically in Magento 2.