How to Use addFieldToFilter in Magento 2 for Flexible Queries
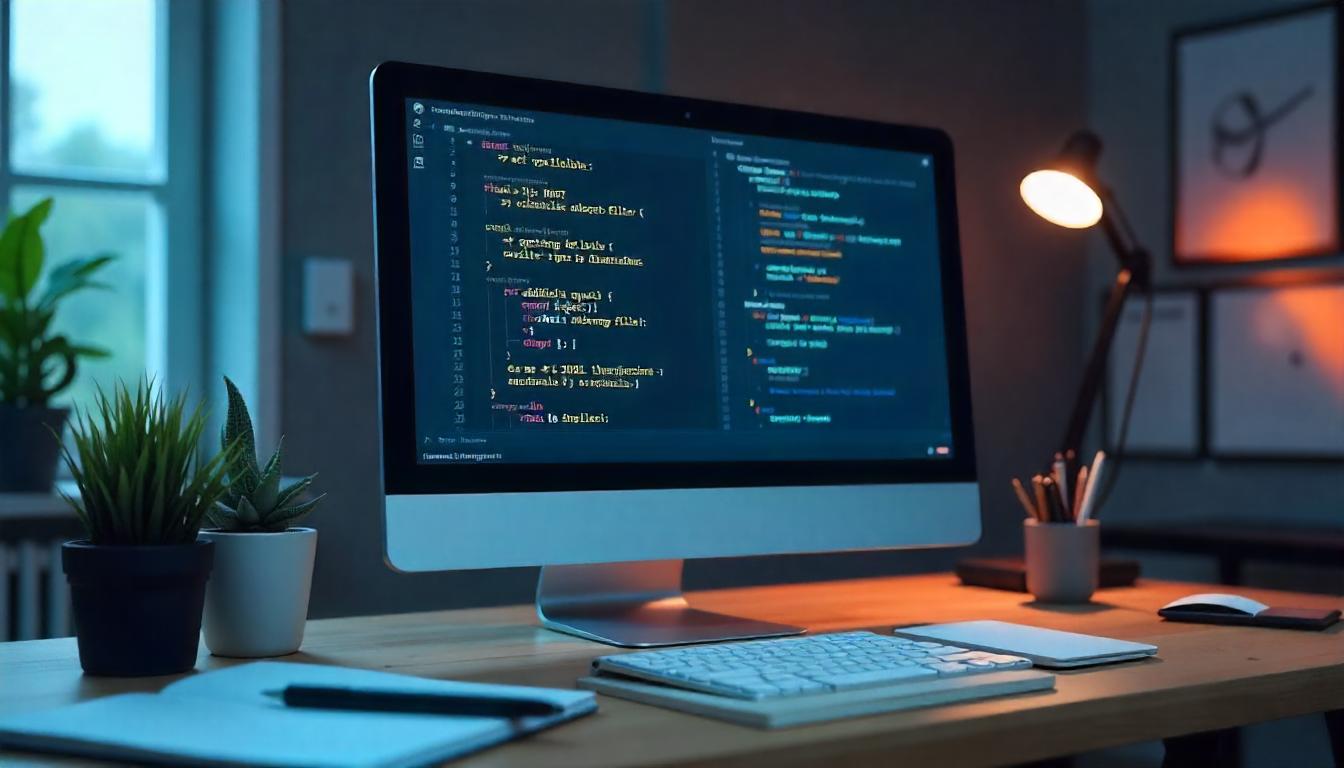
How to Use addFieldToFilter in Magento 2 for Flexible Queries
Magento 2's addFieldToFilter method is a powerful way to add conditions to collections in your application. Whether you're filtering for exact matches, ranges, or handling complex conditions like OR or NULL values, this function simplifies database queries.
Table Of Content
Understanding and Using addFieldToFilter in Magento 2
In Magento 2, addFieldToFilter is a powerful method for refining product collections. It supports various conditions like equals, not equals, like, in, and others. This flexibility lets developers precisely filter data while creating dynamic and efficient queries. Below, we'll explore these conditions in detail, with practical examples to help you implement them effectively.
Common Conditions for addFieldToFilter
The table below summarizes common conditions used with addFieldToFilter and their syntax:
Condition | Operator | Example |
---|---|---|
Equals | eq | $collection->addFieldToFilter('status', ['eq' => 1]); |
Not Equals | neq | $collection->addFieldToFilter('sku', ['neq' => 'test-product']); |
Like | like | $collection->addFieldToFilter('sku', ['like' => 'UX%']); |
Not Like | nlike | $collection->addFieldToFilter('sku', ['nlike' => 'err-prod%']); |
In | in | $collection->addFieldToFilter('entity_id', ['in' => [1, 4, 98]]); |
Not In | nin | $collection->addFieldToFilter('entity_id', ['nin' => [1, 4, 98]]); |
Null | Null | $collection->addFieldToFilter('description', ['null' => true]); |
Not Null | notnull | $collection->addFieldToFilter('description', ['notnull' => true]); |
Greater Than | gt | $collection->addFieldToFilter('entity_id', ['gt' => 5]); |
Less Than | lt | $collection->addFieldToFilter('entity_id', ['lt' => 5]); |
Greater or Equal | gteq | $collection->addFieldToFilter('entity_id', ['gteq' => 5]); |
Less or Equal | lteq | $collection->addFieldToFilter('entity_id', ['lteq' => 5]); |
Practical Implementation
Here's an example of how you can create and filter a product collection in Magento 2 using these conditions:
Magento 2 Product Observer Class
protected $_productCollectionFactory;
public function __construct(
\Magento\Catalog\Model\ResourceModel\Product\CollectionFactory $productFactory
) {
$this->_productCollectionFactory = $productFactory;
}
public function getProductCollection()
{
return $this->_productCollectionFactory->create()
->addAttributeToSelect('*')
->addFieldToFilter('sku', ['eq' => 'test']);
}
Examples of Specific Conditions
1. Filtering Products by Status
Use the eq condition to filter products with a specific status:
$collection->addFieldToFilter('status', ['eq' => 1]);
2. Excluding Specific SKUs
Use the neq condition to exclude products with certain SKUs:
$collection->addFieldToFilter('sku', ['neq' => 'excluded-sku']);
3. Partial Matches
Use the like condition to find SKUs that start with "UX":
$collection->addFieldToFilter('sku', ['like' => 'UX%']);
4. Filtering for NULL Values
To find products without a description:
$collection->addFieldToFilter('description', ['null' => true]);
5. Range Filtering
Filter products with IDs greater than 5:
$collection->addFieldToFilter('entity_id', ['gt' => 5]);
Debugging Common Issues
If the collection isn't filtering as expected, check these common errors:
- Invalid Field Name: Ensure the field exists in the database table.
- Incorrect Syntax: Double-check the array structure for conditions.
- Data Type Mismatch: Verify that the values match the expected data type.
By understanding and correctly applying addFieldToFilter, you can streamline your product collection queries, improve performance, and build more dynamic features.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is addFieldToFilter in Magento 2?
addFieldToFilter is a method in Magento 2 that lets you refine collections by applying flexible conditions to query data. It’s commonly used for filtering product collections.
What Are the Common Conditions for addFieldToFilter?
Conditions include eq (equals), neq (not equals), like, nlike, in, nin, null, notnull, gt (greater than), lt (less than), and their variations like gteq (greater than or equal to).
How Do You Use addFieldToFilter with Equals Condition?
To filter by equals, use:
$collection->addFieldToFilter('status', ['eq' => 1]);
This retrieves items where the status is exactly 1.
How Do You Filter by Not Equals in Magento 2?
Use neq to exclude specific values:
$collection->addFieldToFilter('sku', ['neq' => 'excluded-sku']);
This filters out items with the SKU "excluded-sku."
Can addFieldToFilter Handle Partial Matches?
Yes, the like condition supports partial matches:
$collection->addFieldToFilter('sku', ['like' => 'UX%']);
This retrieves SKUs starting with "UX."
How Do You Filter for NULL or NOT NULL Values?
For NULL values, use:
$collection->addFieldToFilter('description', ['null' => true]);
For NOT NULL values, use:
$collection->addFieldToFilter('description', ['notnull' => true]);
How Do You Filter with Range Conditions?
For greater than, use:
$collection->addFieldToFilter('entity_id', ['gt' => 5]);
For less than, use:
$collection->addFieldToFilter('entity_id', ['lt' => 5]);
What Are Common Issues When Using addFieldToFilter?
Common issues include:
- Using an invalid field name not present in the database.
- Incorrect syntax for the condition array.
- Data type mismatches causing unexpected results.
Double-check your field names, syntax, and data types to avoid errors.