Implementing an Event Observer in Magento 2.4 with PHP 8.2 Compatibility
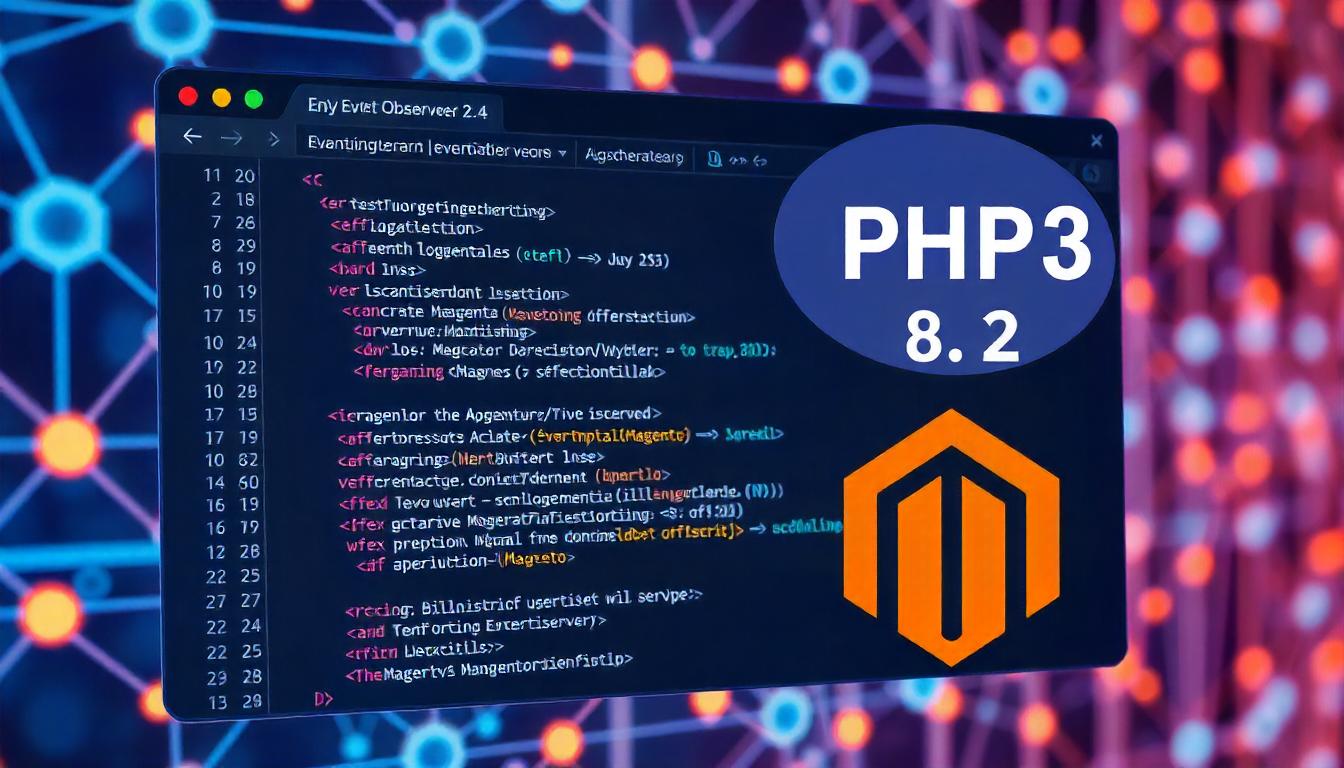
Implementing an Event Observer in Magento 2.4 with PHP 8.2 Compatibility
Event observers in Magento 2 are a flexible way to modify or add behavior to your store without changing the core files. Here's how you can create and use an observer for the sales_order_save_commit_after event, compatible with Magento 2.4 and PHP 8.2.
Table Of Content
Steps to Create an Observer
Create events.xml Define your observer in the events.xml file. Place it in the appropriate directory based on where the event will be triggered:
- Global:
etc/events.xml
- Frontend:
etc/frontend/events.xml
- Admin:
etc/adminhtml/events.xml
Example events.xml for global scope:
Magento 2: Creating an Event Observer
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="sales_order_save_commit_after">
<observer name="custom_sales_order_observer" instance="Vendor\Module\Observer\OrderSaveCommitAfter" />
</event>
</config>
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Create the Observer Class In the Vendor\Module\Observer directory, create OrderSaveCommitAfter.php implementing the ObserverInterface.
Magento 2 Observer: Order Save Commit
<?php
namespace Vendor\Module\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
class OrderSaveCommitAfter implements ObserverInterface
{
public function execute(Observer $observer)
{
$order = $observer->getEvent()->getOrder();
if ($order) {
// Log order ID for debugging purposes
$orderId = $order->getId();
error_log("Order ID: " . $orderId);
// Add your custom logic here
}
}
}
Register Your Module Ensure your module is registered with Magento by defining module.xml
in Vendor/Module/etc
and registration.php
in your module's root directory.
- Keep observer logic minimal and efficient.
- Avoid including business logic directly in the observer. Use service classes or helpers for complex operations.
- Use the correct events.xml file location based on the scope (global, frontend, admin, etc.).
FAQs
What Is an Event Observer in Magento 2.4?
An Event Observer in Magento 2.4 is a mechanism that allows developers to execute custom logic in response to specific events triggered within the Magento system. It’s a key part of Magento’s event-driven architecture.
Why Should I Use an Event Observer?
Using an Event Observer allows you to extend Magento's functionality without modifying the core code. This approach helps in maintaining compatibility during upgrades and adhering to Magento’s modular architecture.
How Do I Create an Event Observer in Magento 2.4?
To create an Event Observer, you need to define an event in `events.xml` and create a corresponding observer class implementing the required logic. Place these files in your custom module following Magento's directory structure.
Is Magento 2.4 Compatible with PHP 8.2?
Yes, Magento 2.4 supports PHP 8.2. Ensure your code, including custom modules and observers, is compatible with PHP 8.2 to prevent errors during runtime.
How Do I Register an Event in Magento 2.4?
Register an event by adding an `events.xml` file in your module’s `etc` directory. Specify the event name and the observer class that handles the event logic.
What Are Common Issues When Implementing Observers?
Common issues include incorrectly defined event names, missing observer class declarations, or syntax errors in XML files. Additionally, ensure compatibility with PHP 8.2 to avoid deprecated function usage.
Can I Use Observers to Modify Checkout Behavior?
Yes, you can use observers to modify checkout behavior by listening to events triggered during the checkout process. For example, you can update cart details or apply custom discounts dynamically.
Where Can I Learn More About Event Observers in Magento 2?
Magento's DevDocs and community forums are excellent resources for learning about Event Observers. Additionally, many developer blogs and tutorials provide step-by-step guides on implementing custom observers.