How to Programmatically Rename Files in Magento 2
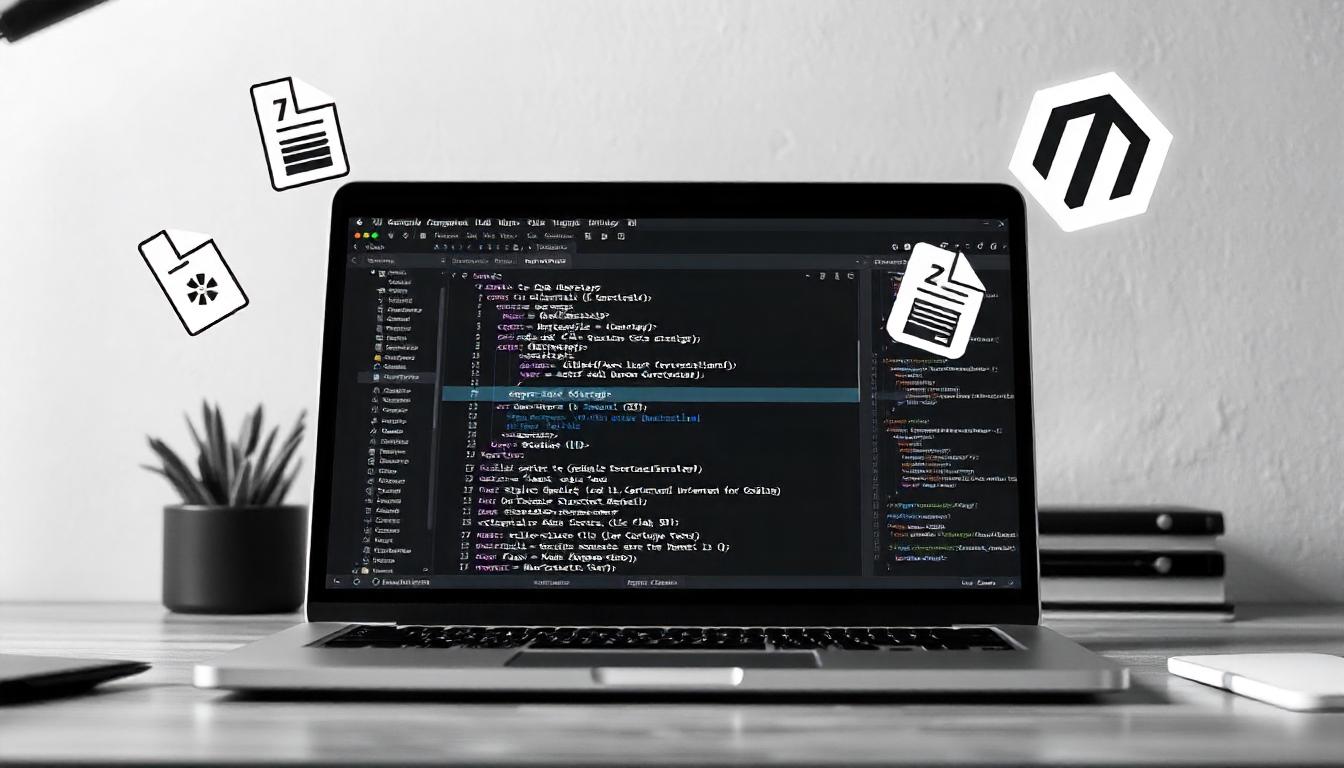
How to Programmatically Rename Files in Magento 2
Renaming files programmatically in Magento 2 is a common requirement for developers when dealing with file uploads, image management, or automated workflows. Here's a step-by-step guide to help you achieve this efficiently.
Table Of Content
How to Programmatically Rename Files in Magento 2
Renaming files programmatically in Magento 2 involves specifying the source and destination paths. This can be particularly useful when working with files under the pub directory or elsewhere. The process uses Magento's Filesystem API, specifically the renameFile() method provided by the Magento\Framework\Filesystem\Directory\Write
class.
Key Steps to Rename Files
Source and Target Paths
Identify the file's current location (source path) and where you want to move or rename it (destination path). For example:
- Source Path:
pub/media/sitemap/sitemap.xml
- Target Path:
pub/media/sitemap/demo.xml
$this->directory = $filesystem->getDirectoryWrite(DirectoryList::PUB);
Using renameFile()
This method accepts two arguments:
$path
(current file path)$newPath
(new file path)
Error Handling
Implement exception handling to catch errors during the renaming process.
Code Example
Here’s an updated and extended example of how to create a custom model for renaming files:
<?php
declare(strict_types=1);
namespace Custom\Module\Model;
use Magento\Framework\App\Filesystem\DirectoryList;
use Magento\Framework\Filesystem\Directory\WriteInterface;
use Magento\Framework\Exception\LocalizedException;
use Magento\Framework\Filesystem;
class RenameFile
{
private Filesystem $filesystem;
private WriteInterface $directory;
public function __construct(
Filesystem $filesystem
) {
$this->filesystem = $filesystem;
$this->directory = $this->filesystem->getDirectoryWrite(DirectoryList::PUB);
}
public function renameFile(string $currentPath, string $newPath): void
{
try {
if (!$this->directory->isFile($currentPath)) {
throw new LocalizedException(__('The file "%1" does not exist.', $currentPath));
}
$this->directory->renameFile($currentPath, $newPath);
} catch (\Exception $e) {
throw new LocalizedException(
__('Failed to rename the file: %1', $e->getMessage()),
$e
);
}
}
}
Example Usage
To rename a file, instantiate the RenameFile class and call the renameFile() method with the source and target paths:
$renameFile = $objectManager->create(\Custom\Module\Model\RenameFile::class);
$renameFile->renameFile('media/sitemap/sitemap.xml', 'media/sitemap/demo.xml');
Additional Notes
- Custom Directories: If you need to work with files outside pub, modify the DirectoryList argument.
- Common Errors: Ensure the file paths are correct and the Magento file system has the necessary permissions.
- Extensibility: Add more methods to handle other file operations, such as copying or deleting files.
Method | Purpose | Example |
---|---|---|
renameFile($path, $newPath) |
Renames or moves a file to a new location. | renameFile('old.xml', 'new.xml') |
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Purpose of Renaming Files Programmatically in Magento 2?
Renaming files programmatically in Magento 2 allows developers to automate file management tasks, ensuring efficiency and accuracy, especially for dynamic file operations under the pub or other directories.
Which Method Is Used to Rename Files in Magento 2?
Magento 2 uses the renameFile()
method from the Magento\Framework\Filesystem\Directory\Write
class. It simplifies renaming by accepting the source and destination file paths as arguments.
Can Files Outside the Pub Directory Be Renamed?
Yes, files outside the pub directory can be renamed by modifying the $this->directory
argument in the constructor to point to the desired directory. For example, replace DirectoryList::PUB
with the appropriate directory constant.
How Do You Define Source and Target Paths for Renaming?
Source and target paths define the current file location and its new location or name. For instance:
- Source Path:
pub/media/sitemap/sitemap.xml
- Target Path:
pub/media/sitemap/demo.xml
Can You Share a Code Example for Renaming Files?
filesystem = $filesystem;
$this->directory = $filesystem->getDirectoryWrite(DirectoryList::PUB);
}
public function renameFile()
{
$path = 'media/sitemap/sitemap.xml';
$destination = 'media/sitemap/demo.xml';
try {
$this->directory->renameFile($path, $destination);
} catch (\Exception $e) {
throw new LocalizedException(__('File rename failed.'), $e);
}
}
}
What Errors Should You Watch For When Renaming Files?
If the file rename fails, check:
- The source and target paths are correct.
- The directory permissions allow file modifications.
- The logs for exceptions or error messages.
Why Is Exception Handling Important in File Operations?
Exception handling ensures that errors during file operations are captured and logged, preventing the application from crashing and aiding in debugging issues.
Is This Approach Suitable for Large-Scale File Operations?
Yes, programmatically renaming files using Magento 2’s APIs ensures scalability and consistency, making it suitable for large-scale projects or repetitive tasks.
What Best Practices Should You Follow for File Renaming?
Follow these best practices:
- Ensure file paths are dynamically determined when possible.
- Implement robust exception handling for error scenarios.
- Log all file operations for traceability and debugging.