Passing Data from Controller to Template in Magento 2
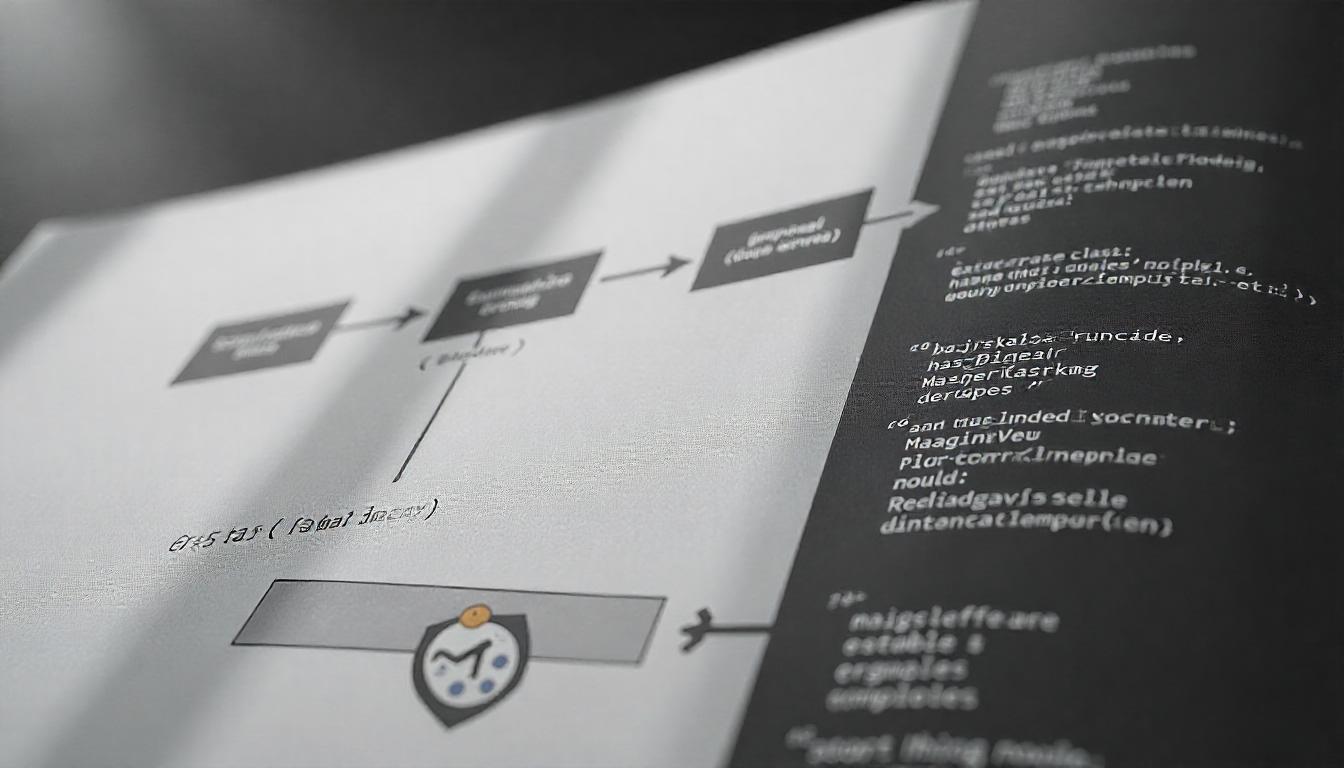
Passing Data from Controller to Template in Magento 2
When building a Magento 2 module, passing data from the controller to the template is a common task. This process allows you to share dynamic or processed information with your view layer for rendering. Here’s a step-by-step guide to achieve it:
Table Of Content
Passing Data from Controller to Template in Magento 2
In Magento 2, you can't pass data directly from a controller to a template. Instead, you use a block class to handle this. Here's how to do it:
1. Controller Action
<?php
declare(strict_types=1);
namespace YourNamespace\YourModule\Controller\Index;
use Magento\Framework\App\Request\DataPersistorInterface;
use Magento\Framework\App\Action\Action;
use Magento\Framework\App\Action\Context;
class YourController extends Action
{
private DataPersistorInterface $dataPersistor;
public function __construct(
Context $context,
DataPersistorInterface $dataPersistor
) {
parent::__construct($context);
$this->dataPersistor = $dataPersistor;
}
public function execute()
{
// Your logic here
$data = ['key' => 'value']; // Replace with your actual data
$this->dataPersistor->set('your_data_key', $data);
// Return your result
return $this->resultFactory->create(ResultFactory::TYPE_PAGE);
}
}
2. Block Class
Create a block class to retrieve the data.
<?php
declare(strict_types=1);
namespace YourNamespace\YourModule\Block;
use Magento\Framework\App\Request\DataPersistorInterface;
use Magento\Framework\View\Element\Template;
class YourBlock extends Template
}
private DataPersistorInterface $dataPersistor;
public function __construct(
Template\Context $context,
DataPersistorInterface $dataPersistor,
array $data = []
) {
parent::__construct($context, $data);
$this->dataPersistor = $dataPersistor;
}
public function getYourData()
{
return $this->dataPersistor->get('your_data_key');
}
}
3. Template File
In your template file, access the data through the block.
<?php
/** @var \YourNamespace\YourModule\Block\YourBlock $block */
$yourData = $block->getYourData();
if ($yourData) {
// Process your data
echo $yourData['key']; // Replace with your actual data processing
}
Alternative Method: Using ViewModel
Another approach is to use a ViewModel, which is a more modern and recommended method in Magento 2.
1. ViewModel Class
<?php
declare(strict_types=1);
namespace YourNamespace\YourModule\ViewModel;
use Magento\Framework\View\Element\Block\ArgumentInterface;
class YourViewModel implements ArgumentInterface
{
public function getYourData()
{
// Your logic to fetch data
return ['key' => 'value']; // Replace with your actual data
}
}
2. Layout XML
Define the ViewModel in your layout XML.
<block class="YourNamespace\YourModule\Block\YourBlock" name="your.block.name">
<arguments>
<argument name="view_model" xsi:type="object">YourNamespace\YourModule\ViewModel\YourViewModel</argument>
</arguments>
</block>
3. Template File
Access the data in your template.
/** @var \YourNamespace\YourModule\Block\YourBlock $block */
$yourData = $block->getYourData();
if ($yourData) {
// Process your data
echo $yourData['key']; // Replace with your actual data processing
}
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Role of a Block Class in Magento 2?
The block class in Magento 2 serves as a bridge between the controller and the template. It enables the transfer of data to the template files while maintaining separation of concerns.
Why Can’t Data Be Passed Directly from Controller to Template?
Magento 2 enforces a strict MVC structure where the controller's primary role is to handle requests. To maintain clean architecture, data must be passed through the block class instead of directly to the template.
How Do You Use DataPersistorInterface to Pass Data?
DataPersistorInterface is used to store and retrieve data in Magento 2. In the controller, you use the `set()` method to save data, and in the block class, the `get()` method retrieves it.
Can You Provide an Example of Controller Code for Passing Data?
dataPersistor->set('key_name', ['data_key' => 'value']);
}
}
This code saves data with the key `key_name` for later retrieval.
How Can Data Be Retrieved in a Block Class?
Data can be retrieved in a block class by using the `get()` method of DataPersistorInterface. Here's an example:
dataPersistor->get('key_name');
}
}
Why Is Using a Block Class a Best Practice?
Using a block class adheres to Magento 2's MVC architecture, ensuring better code organization, maintainability, and scalability.
Can Data Be Passed Directly to Template Without a Block Class?
No, Magento 2 enforces strict MVC principles. A block class must be used to handle the data transfer to templates.
What Are the Benefits of Using DataPersistorInterface?
DataPersistorInterface simplifies data handling by providing methods for storing and retrieving data. It ensures data consistency and reduces the complexity of custom solutions.
What Should I Check If Data Transfer Fails?
If data transfer fails, verify the key used in DataPersistorInterface is consistent, and ensure the block class and template files are correctly implemented. Check logs for errors.
Is This Approach Suitable for Large Projects?
Yes, passing data via the block class ensures scalability and maintainability, making it suitable for projects of any size.