Resolving 'Typed Property Must Not Be Accessed Before Initialization' in PHP
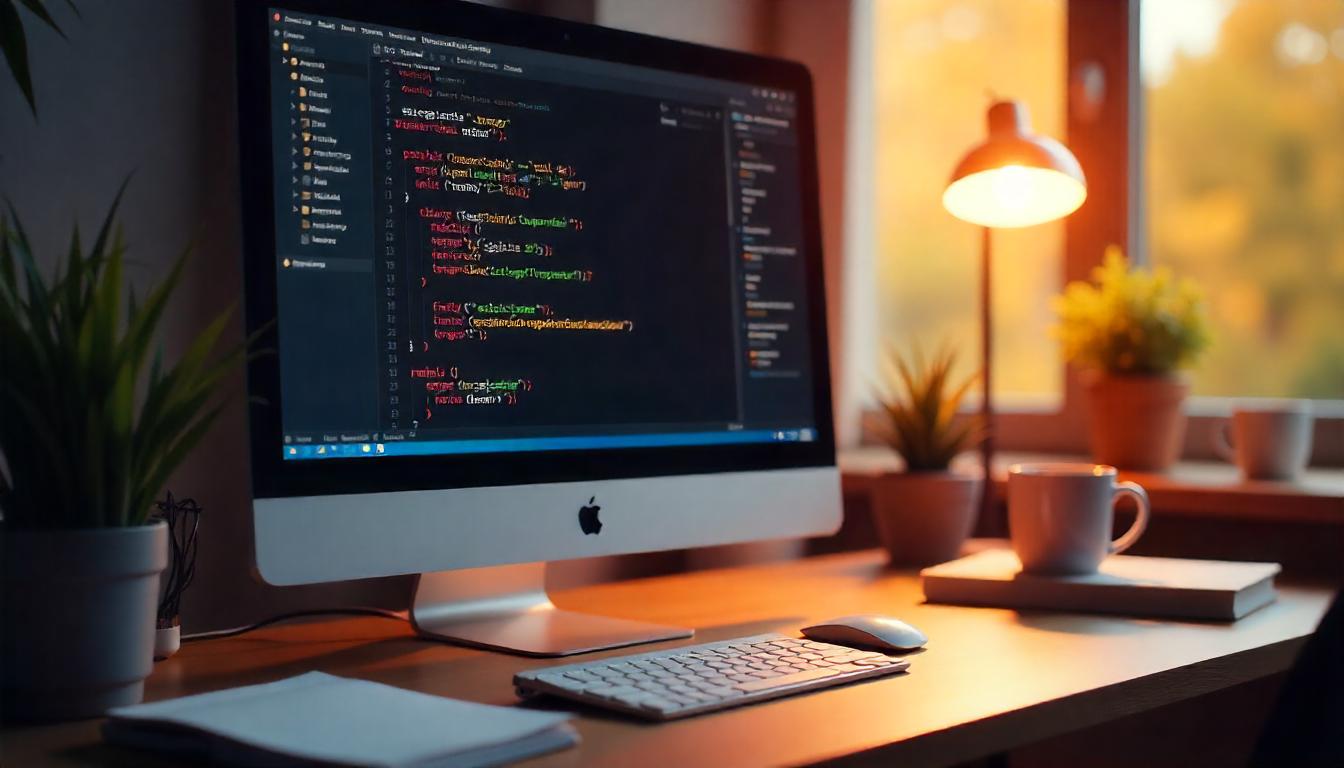
Resolving 'Typed Property Must Not Be Accessed Before Initialization' in PHP
In PHP, the error Typed property must not be accessed before initialization occurs when you try to access a typed property of a class that hasn't been assigned a value yet. Since PHP 7.4 introduced typed properties, this error ensures better type safety but can lead to runtime exceptions if not handled properly. Here's a guide to understanding and resolving this issue.
Resolving 'Typed Property Must Not Be Accessed Before Initialization' in PHP
In PHP 7.4 and later, uninitialized typed properties can cause errors when accessed. To prevent this, always assign a default value to typed properties. For instance, declare a nullable array property as private ?array $loadedData = null;. This ensures the property is properly initialized before use.
Example Implementation:
<?php declare(strict_types=1);
namespace Rbj\Blog\Model;
class DataProvider {
private ?array $loadedData = null;
public function getData(): array
{
if ($this->loadedData !== null) {
return $this->loadedData;
}
// Logic to load data and assign it to $loadedData
$this->loadedData = $this->fetchData();
return $this->loadedData;
}
private function fetchData(): array
{
// Replace with actua data fetching logic
return [];
}
}
In this setup, getData() checks if $loadedData is initialized. If not, it calls fetchData() to assign a value. This approach ensures that $loadedData is always initialized before access, preventing errors.
Key Points:
- Always initialize typed properties to avoid access errors.
- Use nullable types (
e.g., ?array
) when a property can be null. - Implement checks in your methods to ensure properties are initialized before use.
By following these practices, you can effectively manage typed properties in PHP and prevent initialization errors.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is Retry Logic for API Calls in PHP?
Retry logic is a technique used to reattempt failed API calls after a short delay. It’s particularly useful for handling temporary network issues or server unavailability by retrying the request multiple times before giving up.
Why Should I Implement Retry Logic in My PHP Application?
Implementing retry logic helps improve the reliability of your application when interacting with third-party APIs. It reduces the chances of a request failing due to temporary issues and enhances the user experience by reducing downtime.
How Does Retry Logic Work in PHP?
Retry logic works by attempting a failed API request a predefined number of times. Each time the request fails, the system waits for a few seconds before retrying, with the delay increasing after each failed attempt. If all attempts fail, an error is thrown.
How Do I Implement Retry Logic for API Calls in PHP?
You can implement retry logic by using a loop that tracks the number of attempts. If the API returns an error status, the logic waits for a specified period before retrying. After the maximum retries are reached, it throws an exception.
public function callApiWithRetry() {
$retries = 0;
while ($shouldRetry && $retries < self::MAX_RETRIES) {
// API call logic
// If API returns error, retry with delay
}
}
What Is Exponential Backoff in Retry Logic?
Exponential backoff is a retry strategy where the delay between retries increases exponentially with each failure. For example, the first retry might wait 1 second, the second retry waits 2 seconds, and so on. This reduces the likelihood of overloading the API server.
What Are the Benefits of Using Retry Logic in PHP?
Retry logic helps ensure that temporary failures don’t result in permanent errors. It also reduces the need for manual intervention, improves system reliability, and ensures smooth interactions with third-party APIs.
What Happens If Retry Logic Is Not Implemented?
Without retry logic, failed API calls may result in an incomplete transaction or an error that disrupts the user experience. Users may see error messages or experience delays, reducing the reliability of your system.
How Can I Avoid Hitting API Rate Limits with Retry Logic?
To avoid hitting API rate limits, ensure that your retry logic includes a delay between retries, such as exponential backoff. You can also check the API’s documentation for rate-limiting guidelines and adjust your retry strategy accordingly.
How Many Times Should I Retry a Failed API Call?
It’s generally recommended to retry a failed API call between 2 and 5 times, depending on the API’s nature and the severity of the issue. If retries continue to fail, it's best to log the error and alert the system administrator.
Can Retry Logic Be Used for Other Types of Network Failures?
Yes, retry logic can be used for any type of network failure, including timeouts, server errors, and connectivity issues. However, be sure to include a limit on retries to prevent infinite loops and resource wastage.