Fetching Product Collection with GraphQL in Magento 2
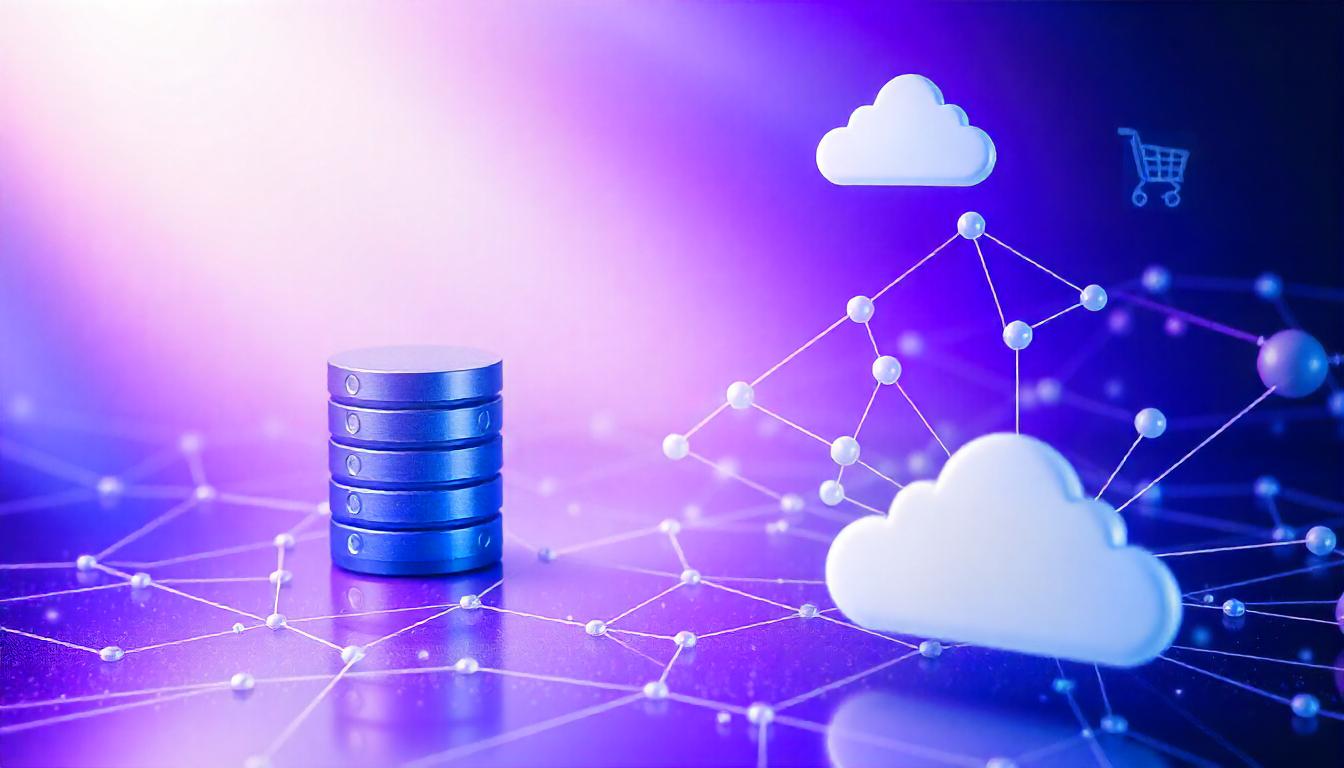
Fetching Product Collection with GraphQL in Magento 2
Magento 2.3 introduced GraphQL, a powerful storefront API for building robust and flexible eCommerce solutions. This guide demonstrates how to retrieve product collections using GraphQL in Magento 2 by creating a custom module.
Table Of Content
Key Features of GraphQL in Magento
GraphQL has revolutionized how Magento integrates with modern storefronts. It offers unique capabilities that cater to the demands of dynamic eCommerce environments. Let’s dive into its essential features and their benefits.
Flexibility
GraphQL provides unparalleled flexibility by allowing you to query only the data you need. Unlike traditional APIs, where predefined responses may include unnecessary information, GraphQL enables developers to tailor requests to specific requirements.
Benefits:
- Reduces data transfer overhead.
- Simplifies front-end development with precise data availability.
Performance
One of the standout features of GraphQL is its ability to consolidate multiple resource requests into a single API call. This reduces the number of server interactions, making it more efficient than REST or SOAP APIs.
Benefits:
- Faster page load times.
- Lower server resource consumption.
Compatibility
GraphQL is inherently compatible with modern technologies such as Progressive Web Applications (PWAs) and headless storefronts. Its ability to provide structured, real-time data makes it ideal for these cutting-edge implementations.
Benefits:
- Seamless integration with headless CMS platforms.
- Enhanced support for dynamic, interactive user interfaces.
Comparison of GraphQL, REST, and SOAP
Here’s a quick comparison of GraphQL with traditional APIs:
Feature | GraphQL | SOAP |
---|---|---|
Data Fetching | Customizable queries; fetch exactly what you need | Predefined responses; limited flexibility |
Performance | Single request for multiple resources | Multiple requests; slower due to complex structure |
Modern Compatibility | Optimized for PWA and headless storefronts | Not ideal for modern storefronts |
GraphQL's flexibility, performance, and compatibility make it a cornerstone for Magento's future-ready eCommerce solutions. Whether you are building a PWA or optimizing an existing storefront, GraphQL offers the tools needed to deliver a seamless customer experience.
Exploring the Product Query in Magento
GraphQL in Magento enables developers to fetch product data effortlessly. While Magento provides a default product query with access to all available fields, creating a custom module allows for more precise queries tailored to your specific needs. This guide explores the default product query and how it can be customized.
Understanding the Default Product Query
The default product query in Magento retrieves a comprehensive set of fields such as name, SKU, price, and more. This query can be utilized out of the box by enabling GraphQL in your Magento store, providing flexibility to fetch product collections directly.
Example: Default Product Query
Below is an example of the default product query:
query {
products(search: "shirt") {
items {
sku
name
price {
regularPrice {
amount {
value
currency
}
}
}
}
total_count
page_info {
current_page
page_size
}
}
}
Why Customize the Product Query?
While the default query offers extensive data, it may fetch more information than required, increasing response size and load times. Customizing the query ensures you retrieve only the data you need, improving performance and reducing redundancy.
Key Benefits of Custom Queries:
- Enhanced performance by limiting unnecessary data.
- Precise control over the query structure.
- Ability to include conditions to filter product collections.
Comparison Table: Default Query vs. Custom Query
Aspect | Default Product Query | Custom Product Query |
---|---|---|
Fields Fetched | All available fields | Selected fields based on needs |
Performance | May include unnecessary data | Optimized for specific requirements |
Flexibility | Limited to predefined structure | Highly flexible with custom filters |
Custom Product Query
To fetch a specific product collection with selected fields, you can define a custom query. Here’s an example:
query {
products(filter: {price: {from: "50", to: "100"}}) {
items {
name
sku
price {
regularPrice {
amount {
value
currency
}
}
}
}
}
}
Steps to Customize the Product Query
- Create a Custom Module: Set up a new Magento module to define the custom query.
- Define Schema: Add a
schema.graphqls
file to declare the query structure. - Implement Resolver: Use a resolver class to process and return the desired data.
Magento's GraphQL API offers both a robust default product query and the ability to craft customized queries. By creating a custom module, you can enhance performance, tailor data responses, and provide a seamless experience for developers and end-users alike.
Comprehensive Guide to Creating a Custom Module in Magento 2
Magento 2 is known for its modular architecture, allowing developers to extend and customize its functionality with ease. One of the fundamental tasks in Magento development is creating a custom module. This guide walks you through the steps to build a custom module effectively.
Step 1: Set Up the Basic Structure of Your Custom Module
Creating a custom module in Magento 2 requires setting up the foundational files and configurations. Below, we outline the necessary steps to define your module structure.
Files to Create for Module Definition:
registration.php
This file registers your module within Magento’s system and should be placed in the root of your module directory.PHP Code for Module Registration
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Emmo_ProductsGraphQl',
__DIR__
);
module.xml
This configuration file is required for Magento to recognize the module. It should be placed inside theetc
directory of your module. The file also defines module dependencies.XML Configuration for Module
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Emmo_ProductsGraphQl">
<sequence>
<module name="Magento_Catalog"/>
<module name="Magento_GraphQl"/>
</sequence>
</module>
</config>
- Explanation
Magento_Catalog
: Ensures your module can interact with the catalog data.Magento_GraphQl
: Integrates GraphQL functionality for the custom module.
Directory Structure
Here’s how your module directory should be structured at this point:
Module Directory Structure
app/code/Emmo/ProductsGraphQl
├── registration.php
└── etc
└── module.xml
By defining these files, you’ve completed the first step of module creation. In the next steps, you will add more functionality, such as custom GraphQL queries, models, and other logic to extend your Magento store.
Step 2: Defining the GraphQL Schema
To enable custom GraphQL queries in your Magento 2 module, you need to define the schema. This schema will specify the structure and resolvers for the data that will be exposed via GraphQL.
Create the schema.graphqls
File
This file defines the GraphQL types and queries that your module will expose. It is essential to link your query to the appropriate resolver, which will handle the logic for fetching the data.
Here is how you can create the schema.graphqls
for your module:
GraphQL Schema Definition
schema.graphqls
type Query {
productCollection: ProductCollection @resolver(class: "Emmo\\ProductsGraphQl\\Model\\Resolver\\ProductsResolver") @doc(description: "Fetch product collection for the store")
}
type ProductCollection @doc(description: "A collection of products from the store") {
allProducts: [ProductRecord] @doc(description: "Detailed product information")
}
type ProductRecord {
sku: String @doc(description: "Product SKU")
name: String @doc(description: "Product Name")
price: Float @doc(description: "Product Price")
}
Explanation of the Schema
Type/Field | Description |
---|---|
Query | Defines the root query for fetching data. |
productCollection | The custom query to retrieve the product collection. Links to the resolver. |
ProductCollection | Represents a collection of products. |
allProducts | An array of product records, each containing information like SKU, name, and price. |
ProductRecord | Defines the structure of each individual product, including its SKU, name, and price. |
This schema enables a custom GraphQL query productCollection
, which can be used to fetch product records in your store. The query is linked to the resolver ProductsResolver
, which contains the logic for retrieving the actual data.
With this schema in place, you have laid the foundation for your custom GraphQL functionality. The next step is to implement the resolver logic to fetch product data.
Step 3: Implementing the Resolver Model for Product Fetching
In this step, we’ll create a resolver model that contains the logic necessary to fetch product data based on specific conditions. This resolver will interface with the Magento catalog API to retrieve a list of products.
The Resolver Model: ProductsResolver.php
ProductsResolver PHP Class
declare(strict_types=1);
namespace Emmo\ProductsGraphQl\Model\Resolver;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Magento\Framework\Api\SearchCriteriaBuilder;
use Magento\Framework\GraphQl\Config\Element\Field;
use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException;
use Magento\Framework\GraphQl\Query\ResolverInterface;
use Magento\Framework\GraphQl\Schema\Type\ResolveInfo;
class ProductsResolver implements ResolverInterface
{
public function __construct(
private readonly ProductRepositoryInterface $productRepository,
private readonly SearchCriteriaBuilder $searchCriteriaBuilder
) {}
public function resolve(
Field $field,
$context,
ResolveInfo $info,
array $value = null,
array $args = null
) {
// Fetch the product data using custom logic
$productsData = $this->getProductsData();
return $productsData;
}
private function getProductsData(): array
{
try {
// Build the search criteria for fetching products
$searchCriteria = $this->searchCriteriaBuilder->addFilter('entity_id', 1, 'gteq')->create();
$products = $this->productRepository->getList($searchCriteria)->getItems();
$productRecord['allProducts'] = [];
// Loop through the product collection and prepare the data
foreach ($products as $product) {
$productRecord['allProducts'][] = [
'sku' => $product->getSku(),
'name' => $product->getName(),
'price' => $product->getPrice(),
];
}
} catch (NoSuchEntityException $e) {
// Handle the case when no product is found
throw new GraphQlNoSuchEntityException(__($e->getMessage()), $e);
}
return $productRecord;
}
}
Key Components of the Resolver Model
This resolver interacts with the Magento catalog API to retrieve product data. Below is an explanation of the essential parts of the code.
Component | Description |
---|---|
ProductRepositoryInterface | Used to interact with the product data in Magento's catalog system. It retrieves product information based on search criteria. |
SearchCriteriaBuilder | Helps build search queries for filtering and retrieving specific product data, like filtering by product ID or price range. |
Field | Represents the field that the GraphQL query requests, such as productCollection. It’s used for resolving the data. |
GraphQlNoSuchEntityException | An exception thrown when no entity (product) is found matching the query criteria, providing a meaningful error message. |
resolve() | The main function that is triggered when the GraphQL query is executed. It fetches the product data and returns it. |
getProductsData() | A custom function to get the list of products from the catalog based on defined criteria. It formats the product information before returning it. |
By following this structure, you’ve created a resolver that retrieves product data in a flexible and customizable way. This setup can be extended to implement more complex querying logic as needed.
Querying the Product Collection
To fetch product details such as SKU, name, and price from your Magento store using GraphQL, you can utilize GraphQL client tools like Altair GraphQL or ChromeiQL. These tools allow you to create and send queries to your Magento server and view the responses in a structured format.
Example GraphQL Query
Below is an example of a GraphQL query to retrieve a list of products, including their SKU, name, and price:
Request:
GraphQL Query for Product Collection
{
productCollection {
allProducts {
sku
name
price
}
}
}
Sample Response
After executing the query, you should receive a response similar to this:
Response:
GraphQL Query for Product Collection
{
"data": {
"productCollection": {
"allProducts": [
{
"sku": "Test Product 1",
"name": "Test Product 1",
"price": 100
},
{
"sku": "Test Product 2",
"name": "Test Product 2",
"price": 199.5
}
]
}
}
}
This response contains the data requested in the query. Each product is returned with the sku, name, and price
values, which can then be used for further processing or displayed on the frontend.
Essential Tips for GraphQL Developers
When working with GraphQL in Magento, there are several best practices and tips that can help improve the efficiency, flexibility, and debugging of your queries. Here are some key strategies:
1. Implement Custom Conditions in the Resolver
Enhance your query by adding custom filters in the resolver. This allows you to fetch specific products based on various criteria, such as product attributes, categories, or custom fields. For instance, you can filter products by their availability or price range to deliver more targeted data to the client.
2. Expand Your GraphQL Schema
GraphQL schemas are flexible and can be extended to include additional fields. You can add new fields to existing types or create completely new custom types. This expansion allows your GraphQL queries to provide richer and more specific data, improving the user experience for consumers and admins alike.
3. Leverage Magento's Debugging and Logging Tools
Debugging is an essential part of working with GraphQL in Magento. Utilize Magento's built-in logging tools to track errors and troubleshoot issues in your queries. Whether it's a missing field or a failure to fetch data, effective logging can significantly speed up your debugging process.
Summary of Tips
Tip | Description |
---|---|
Custom Conditions | Add filters in the resolver to fetch specific products based on defined criteria, improving query precision. |
Schema Expansion | Extend the GraphQL schema by adding new fields or types to meet evolving data requirements. |
Debugging | Use Magento's logging and debugging tools to troubleshoot errors in GraphQL queries, improving development efficiency. |
By incorporating these tips into your development workflow, you can build more powerful, efficient, and maintainable GraphQL queries for your Magento projects.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
To summarize, the process of creating a custom GraphQL module in Magento enhances the flexibility and performance of an eCommerce site. By defining the module structure with essential files like registration.php and module.xml, developers lay the foundation for seamless integration with existing Magento components. The schema creation and resolver implementation provide an opportunity to customize data retrieval by defining precise queries and leveraging product-related data with ease.
GraphQL's extensibility allows developers to tailor the API to meet specific business needs. Whether it’s by adding custom filters in the resolver to target certain products or expanding the schema with new fields to capture additional information, the possibilities are vast. Furthermore, Magento’s built-in logging and debugging tools ensure that issues can be resolved efficiently, making the development process smoother and faster.
By utilizing tools like Altair GraphQL or ChromeiQL for query testing, developers can ensure that the queries work as expected and optimize performance before deployment. In addition, understanding and implementing best practices in query optimization, error handling, and modular design will result in a highly maintainable and scalable solution.
Ultimately, mastering the Magento GraphQL API opens up countless opportunities for customizations that align with specific business requirements. Whether you're working with product collections or building complex queries, the knowledge of how to create, test, and optimize GraphQL requests will provide a competitive edge and drive long-term success for your online store. With Magento’s flexibility, combined with the power of GraphQL, you can ensure a future-proof, data-driven, and seamless shopping experience for your customers.
FAQs
What is GraphQL in Magento 2?
GraphQL is a query language for APIs that enables clients to request exactly the data they need, making it more efficient than traditional REST APIs. Magento 2 supports GraphQL for enhanced data fetching and flexibility.
How do I enable GraphQL in Magento 2?
GraphQL is enabled by default in Magento 2. To start using it, you simply need to create the necessary schema files and define the resolvers in your custom module.
What are resolvers in GraphQL?
Resolvers are responsible for fetching the data when a GraphQL query is executed. In Magento 2, resolvers can be used to define custom logic for retrieving data from the database or other sources.
How do I create a custom GraphQL query in Magento 2?
To create a custom GraphQL query, you need to define the query structure in a schema file (`schema.graphqls`) and implement the resolver logic in a custom PHP class.
What is the purpose of the `schema.graphqls` file?
The `schema.graphqls` file defines the structure of the GraphQL queries and types. It specifies what queries can be made and what fields can be fetched, serving as the contract between the client and server.
Can I filter products using GraphQL in Magento 2?
Yes, you can filter products using GraphQL in Magento 2 by adding filters in the resolver. Filters allow you to retrieve products based on specific criteria such as SKU, price range, or category.
How do I handle errors in GraphQL queries?
Errors in GraphQL queries can be handled by throwing exceptions in the resolver. For example, the `GraphQlNoSuchEntityException` can be thrown when a product or other entity cannot be found.
What tools can I use to test GraphQL queries?
Tools like Altair GraphQL, GraphiQL, or Postman can be used to test GraphQL queries. These tools provide an interface for writing and testing queries, making it easier to debug and refine your GraphQL requests.
Can GraphQL be used for product details in Magento 2?
Yes, GraphQL can be used to retrieve detailed product information, including attributes like SKU, name, price, and more, by defining the necessary fields in the schema and fetching them via the resolver.
What is a custom resolver in Magento 2?
A custom resolver is a PHP class in Magento 2 that contains the logic to fetch data for a GraphQL query. It processes the query and returns the requested data to the client, allowing for customized data fetching.
How can I extend the GraphQL schema in Magento 2?
You can extend the GraphQL schema by adding new types, queries, or fields in the `schema.graphqls` file. This allows you to support custom data structures and functionality beyond the default Magento 2 GraphQL API.
Is GraphQL better than REST API in Magento 2?
GraphQL offers several advantages over REST APIs, including more precise data fetching, reduced over-fetching of data, and the ability to query multiple resources in a single request. However, the choice depends on your specific use case.
What is a GraphQL mutation in Magento 2?
A GraphQL mutation is a type of operation used to modify data, such as creating, updating, or deleting resources. In Magento 2, mutations can be used to perform actions like adding products to the cart or updating customer information.