How to Use Retry Functionality for Specific Failed API Calls in PHP Magento 2?
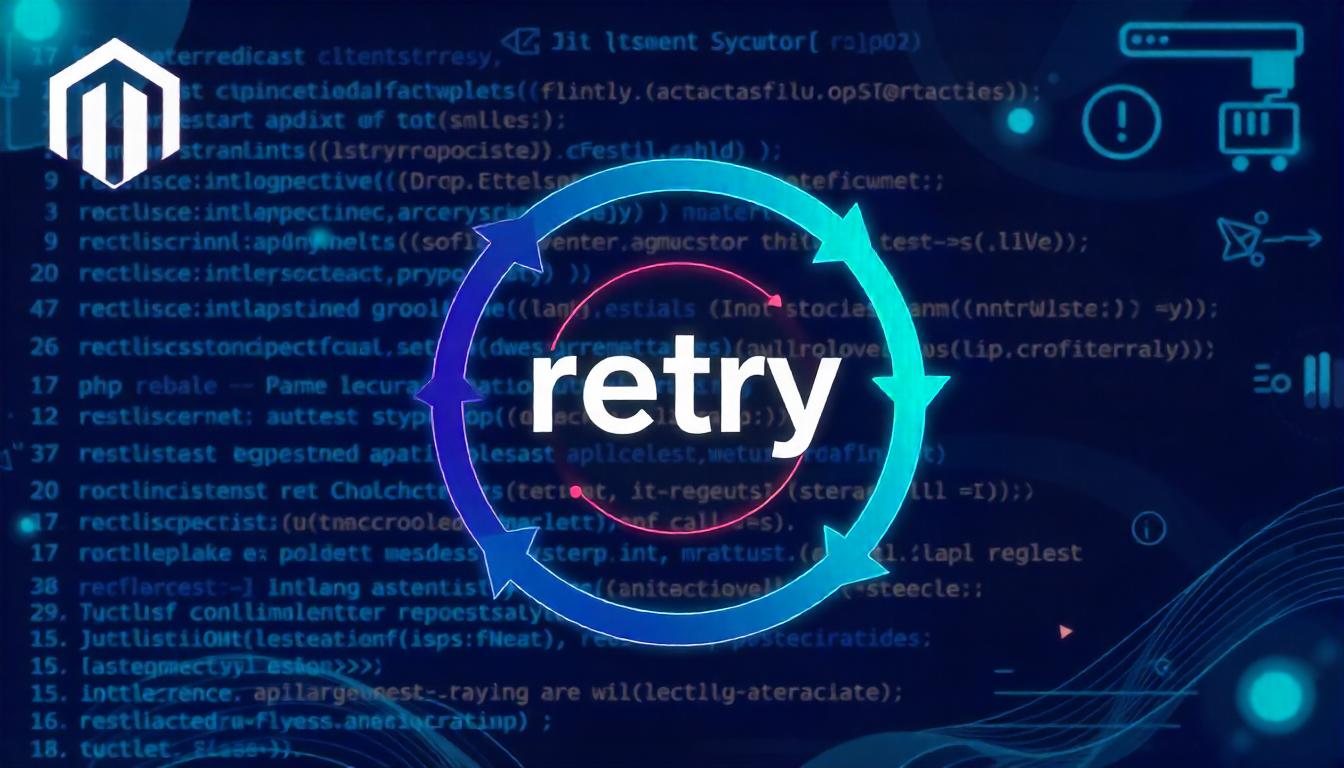
How to Use Retry Functionality for Specific Failed API Calls in PHP Magento 2?
When working with third-party APIs in Magento 2, you may encounter situations where an API call fails due to transient issues like server downtime or network instability. Instead of failing outright, implementing a retry mechanism allows the system to reattempt the API call, ensuring better resilience and reliability.
This article provides a comprehensive guide to using the retry functionality for failed API calls, demonstrating how to implement progressive delays and structured retries.
Table Of Content
Understanding the Importance of Retry Functionality in Applications
Retry functionality is a key aspect of building resilient systems, especially when dealing with third-party APIs. Temporary disruptions can occur for various reasons, and a robust retry mechanism ensures that such issues do not disrupt the application's workflow. Here's an enhanced breakdown of its significance:
Key Benefits of Retry Functionality
1. Improved Reliability
- Purpose: Reattempts failed API calls, increasing the likelihood of successful communication.
- Example Scenario: When a payment gateway experiences brief downtime, retries ensure transactions are processed smoothly.
2. Reduced Downtime Impact
- Purpose: Automatically handles temporary server outages without requiring manual intervention.
- Example Scenario: E-commerce platforms remain functional even if product data APIs face intermittent failures.
3. Enhanced User Experience
- Purpose: Prevents immediate errors from being displayed to users, maintaining a seamless operational flow.
- Example Scenario: Retry mechanisms in messaging apps ensure that messages are sent even during network instability.
Core Components of a Retry Mechanism
Retry Count
- Limits the number of retries to prevent excessive API load.
Backoff Strategy
- Implements delays between retries (e.g., exponential backoff) to avoid overwhelming the server.
Timeout Settings
- Establishes a maximum wait time for responses, ensuring the system does not hang indefinitely.
Error Handling
- Provides clear messages when retries fail after the limit is reached.
Retry Mechanism Approaches
Aspect | Simple Retry | Exponential Backoff |
---|---|---|
Delay Between Attempts | Fixed duration (e.g., 5 seconds) | Gradually increases with each retry (e.g., 2, 4, 8 seconds). |
Server Load | May overwhelm the server during downtime. | Reduces server strain by spacing out retries. |
Best Use Case | Stable APIs with rare failures. | APIs prone to frequent or temporary disruptions. |
Advanced Considerations for Retry Functionality
Idempotent Requests:
Ensure API requests are idempotent (repeated calls yield the same result) to prevent duplicate actions.
Logging and Monitoring:
Log retries and failures for analysis, enabling proactive debugging and optimizations.
Custom Retry Policies:
Tailor retry logic for specific API endpoints or error codes (e.g., retry only for 503 Service Unavailable errors).
Circuit Breaker Pattern:
Implement a circuit breaker to stop retries if the failure rate exceeds a predefined threshold, protecting the system from overload.
By integrating a well-thought-out retry mechanism, developers can enhance system resilience, improve reliability, and provide a smoother experience for end-users.
Key Features of the Retry Mechanism
Progressive Delay:
>- Definition: The time between retries increases after each failure to avoid overwhelming the server.
- Example: Initial delay of 5 seconds, doubling to 10 seconds, then 15 seconds, and so on.
Limited Attempts:
- Definition: Ensures the retry process doesn't continue indefinitely.
- Example: A maximum of 3 retries is commonly configured.
Customizable Configuration:
- Flexibility: Tailor retry settings, including delay intervals, maximum retries, and failure thresholds.
- Application-Specific: Adjust configurations based on the criticality of the API and expected downtime patterns.
Error Handling Integration:
- Log details of failed attempts for troubleshooting.
- Provide fallback mechanisms in case retries exhaust without success.
Progressive Delay vs. Fixed Delay
Aspect | Progressive Delay | Fixed Delay |
---|---|---|
Delay Pattern | Increases incrementally (e.g., 5s, 10s, 20s) | Constant interval between attempts (e.g., 10s each time) |
Server Impact | Reduces server strain over time. | May overwhelm the server during outages. |
Use Case | APIs with high traffic or prone to transient errors. | Stable APIs with predictable failures. |
Explanation of How the Retry Functionality Works
Step-by-Step Process:
Initial Request:
- The application sends an API request to the server.
- The response is immediately validated.
Failure Handling:
- If the API response indicates a failure (e.g., HTTP status code 500 or timeout), the retry mechanism is triggered.
Progressive Delay Application:
- The system waits for an initial delay before retrying the request.
- Delay duration progressively increases, applying exponential backoff strategies if configured.
Retry Limit Check:
- The system verifies whether the maximum retry limit has been reached.
- If the limit is exceeded, an exception or custom error response is generated.
Success or Exit:
- On a successful API response, the process terminates and returns the result.
- If all retries fail, the mechanism transitions to error-handling protocols.
Best Practices for Implementing Retry Functionality
Set Reasonable Limits:
Avoid excessive retries to prevent resource wastage or prolonged downtime.
Use Exponential Backoff:
Adopt a delay pattern that progressively increases to balance responsiveness and server stability.
Incorporate Fallback Options:
Use cached data or alternate APIs when retries fail.
Notify users about the temporary unavailability of certain features.
Monitor and Log Failures:
Enable detailed logging to analyze patterns in API failures and optimize retry settings accordingly.
Adapt to Specific API Behavior:
Configure retry policies based on API-specific characteristics, including expected latency and error patterns.
Combine with Circuit Breakers:
Prevent retry storms during extended downtimes by integrating retry mechanisms with circuit breaker patterns.
By following these steps and configurations, the retry functionality can significantly enhance application reliability and user experience.
Code Implementation for Retry Functionality
Here’s the full implementation of a robust retry mechanism in PHP, along with explanations for each key part:
<?php declare(strict_types=1);
namespace Emmo\Blog\Model;
use Psr\Log\LoggerInterface;
class RetryApiCall
{
private const MAX_ERROR_RETRY = 3; // Maximum retry attempts
private const DEFAULT_DELAY = 5; // Default delay in seconds
private LoggerInterface $logger;
public function __construct(LoggerInterface $logger)
{
$this->logger = $logger;
}
/**
* Handles API calls with retry functionality
*
* @return array Response data from API
* @throws \Exception If maximum retries are exceeded
*/
public function callThirdPartyAPI(): array
{
$retries = 0;
$response = [];
try {
do {
try {
$response = $this->makeApiRequest();
$statusCode = (int) $response['status'];
if ($statusCode === 200) {
$this->logger->info("API call successful.");
$shouldRetry = false;
} else {
$this->logger->warning("API call failed with status $statusCode. Retrying...");
$shouldRetry = true;
$this->waitOnRetry(++$retries, $statusCode);
}
} catch (\Exception $e) {
$this->logger->error("Error during API call: " . $e->getMessage());
throw $e;
}
} while ($shouldRetry);
} catch (\Exception $exception) {
$this->logger->critical("Maximum retry attempts reached: " . $exception->getMessage());
throw $exception;
}
return $response;
}
/**
* Simulates an API request
*
* @return array Simulated API response
*/
private function makeApiRequest(): array
{
return ['status' => rand(200, 500), 'body' => []];
}
/**
* Implements delay between retries
*
* @param int $retries Current retry count
* @param int $status Response status code
* @throws \Exception If maximum retries are exceeded
*/
private function waitOnRetry(int $retries, int $status): void
{
if ($retries <= self::MAX_ERROR_RETRY) {
$delay = self::DEFAULT_DELAY * $retries;
$this->logger->info("Waiting $delay seconds before retry #$retries...");
sleep($delay);
} else {
throw new \Exception("Maximum retry attempts ($retries) reached. Last status: $status");
}
}
}
Explanation
Retry Logic:
- The
callThirdPartyAPI
method implements a loop with retries for failed API calls. It stops once a successful response (200
) is received or the retry limit is exceeded.
Progressive Delay:
- The
waitOnRetry
method calculates delays based on the current retry count. For example, retry #1 waits for 5 seconds, retry #2 for 10 seconds, and so on.
Logging Integration:=
- The
LoggerInterface
tracks each attempt, providing insights into errors and retries for debugging.
Simulation of API Calls:
- The
makeApiRequest
method simulates an API response. Replace this with actual API call logic using libraries like cURL or Guzzle.
Exception Handling:
- Ensures that clear error messages are logged and exceptions are thrown when retries fail after reaching the limit.
Features to Note
- Customizable Parameters: Retry limits and delays can be adjusted for specific APIs.
- Extensibility: Easy to integrate with circuit breakers or other failover mechanisms.
- Logging: Provides detailed insights into the retry process for debugging.
By using this code, you ensure a robust mechanism to handle transient API failures, improving overall system reliability.
Step-by-Step Guide to Implementing Retry Functionality
Implementing retry functionality in API calls ensures reliability and resilience in applications. Below is an enhanced guide that delves into each step with more details, practical tips, and a comprehensive table summarizing key points.
Step 1: Setup API Call
Before implementing retry logic, ensure the API call is correctly set up. Use libraries like cURL, Guzzle, or other HTTP clients to make requests.
Example
- cURL: Use
curl_exec()
to execute the API call and handle the response. - Guzzle: Simplify the process using the
GuzzleHttp\Client
class.
$client = new \GuzzleHttp\Client();
$response = $client->request('GET', 'https://api.example.com/data');
Tips
- Ensure you capture both the response body and the HTTP status code.
- Log each request to trace errors if issues occur.
Step 2: Define Retry Logic
Establish conditions under which retries are triggered. For instance:
- API response status code is not 200 (OK).
- Specific error codes like 500 (Internal Server Error) or 503 (Service Unavailable).
Best Practices
- Define error handling rules for various HTTP status codes.
- Avoid retrying for client-side errors (e.g., 400 series).
Step 3: Implement Progressive Delays
Progressive delays prevent overwhelming the API server. For instance:
- Use exponential backoff: Delay increases with each retry attempt (e.g., 2, 4, 8 seconds).
- Use the
sleep()
function for delays in PHP.
Example
$delay = 2; // Initial delay
for ($i = 0; $i < $maxRetries; $i++) {
sleep($delay);
$delay *= 2; // Double the delay on each retry
}
Advantages
- Reduces the likelihood of API throttling.
- Provides servers time to recover.
Step 4: Limit Retry Attempts
To avoid infinite loops, set a maximum number of retries. Typically, 3-5 attempts is a reasonable range.
Why It Matters
- Prevents unnecessary use of resources.
- Ensures a fallback mechanism in case of persistent failure.
Practical Tip
Log the retry count and the status code for better debugging.
Step 5: Handle Exceptions
If the retry attempts fail, throw a descriptive exception. This makes debugging easier and ensures your application handles errors gracefully.
Example
if ($retries >= $maxRetries) {
throw new \Exception("Max retries reached. Last status code: $statusCode");
}
Exception Logging
- Log exceptions with detailed error messages.
- Include the API endpoint, parameters, and status code for context.
Summary of Steps
Step | Description | Example |
---|---|---|
Setup API Call | Use libraries like cURL or Guzzle to make API requests. | $response = $client->request('GET', 'https://api.example.com'); |
Define Retry Logic | Trigger retries for non-200 HTTP status codes. | if ($statusCode !== 200) { retry(); } |
Implement Progressive Delays | Add wait times between retries to avoid API overload. | sleep(2 * $retryCount); |
Limit Retry Attempts | Set a cap on retries to avoid infinite loops. | if ($retries > 3) { throw new \Exception(); } |
Handle Exceptions | Throw exceptions if retries fail after the maximum attempts. | throw new \Exception("API failure"); |
Additional Considerations
Logging
- Maintain logs for every API call, retry attempt, and exception.
- Use a structured format like JSON for better analysis.
Monitoring
- Integrate tools like New Relic or Datadog to monitor API call success rates.
Testing
- Simulate different API responses (e.g., 500, 503) to ensure the retry logic works as intended.
By following these steps, you can implement a robust retry mechanism for API calls, ensuring your application remains reliable and scalable even when facing external API failures.
Configurable Retry Parameters
To make the retry functionality more flexible and adaptable to various use cases, you can externalize the settings into a configuration file. This allows for easy customization of retry behavior without modifying the application code directly. Below is an example of the parameters you can define and how they impact the retry functionality:
Parameter | Default Value | Description |
---|---|---|
max_retry_attempts | 3 | The maximum number of retry attempts allowed before failing. |
retry_delay_seconds | 5 | The initial delay between retries in seconds. |
incremental_delay | true | Whether the delay increases progressively with each retry. |
log_failed_attempts | true | Whether to log each failed attempt for debugging purposes. |
These configurable parameters make it easy to adjust retry behavior for different environments and API conditions.
Progressive Retry Delays
In the case of progressive retry delays, the delay between each retry increases. This is useful for handling temporary issues that may resolve over time. Here's an example of how the delay progresses over multiple retry attempts:
Retry Attempt | Delay (Seconds) | Total Wait Time (Seconds) |
---|---|---|
1st Attempt | 5 | 5 |
2nd Attempt | 10 | 15 |
3rd Attempt | 15 | 30 |
This approach ensures that the system doesn’t overwhelm the server by retrying too quickly, and provides a better chance of success as time passes.
Advanced Enhancements
Beyond the basic retry functionality, several advanced features can be implemented to improve efficiency, reduce server load, and ensure a more robust retry mechanism. Here are some advanced enhancements you can consider:
- Logging: Record each retry attempt for better debugging and monitoring. By keeping track of each failed attempt, you can analyze patterns and determine if retries are being attempted too often or if certain errors are more frequent.
- Error Categorization: Retry only on specific errors, such as server-side errors (e.g., HTTP 500 or 503), while ignoring others like client-side errors (e.g., HTTP 400). This prevents unnecessary retries on errors that are unlikely to resolve through retrying.
- Dynamic Delays: Use exponential backoff or jitter to randomize delays between retries. This reduces the risk of causing a server overload by having multiple clients retrying at the same time. Exponential backoff increases the delay after each retry, and jitter introduces randomness to spread retries across time.
Common Use Cases for Retry Functionality
Retry functionality is particularly useful in environments where temporary failures are common, such as when dealing with third-party services. Here are a few common use cases for implementing retry logic:
- Order Synchronization: In an eCommerce system, failed requests to update order statuses with third-party logistics APIs can be retried. This ensures that the order status remains accurate even if the API service is temporarily unavailable.
- Inventory Updates: When syncing stock levels with external systems, retrying failed calls ensures that inventory data is eventually updated correctly without losing synchronization with suppliers or warehouse systems.
- Payment Gateways: Payment processing systems are prone to temporary issues such as timeouts or service interruptions. Implementing retry logic allows for automatic reattempts when payment gateway APIs experience temporary failures.
By incorporating these configurable parameters and advanced enhancements, you can significantly improve the robustness of your retry functionality and ensure smoother integration with third-party services.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Implementing retry functionality is an essential aspect of building robust and resilient applications that interact with third-party APIs. By adding a structured mechanism for retrying failed requests, you ensure that temporary disruptions or errors don’t disrupt your application’s critical operations.
Key strategies like progressive delays, limiting the number of retries, and customizing retry parameters enable you to fine-tune the retry logic to suit the specific needs of your system. With enhancements such as dynamic delays (like exponential backoff), error categorization, and detailed logging, you can create an even more efficient and fault-tolerant system.
By adopting these best practices, your application will not only become more reliable but will also enhance user experience by minimizing disruptions caused by temporary API failures. Whether you're handling order synchronization, updating inventory, or
FAQs
What is retry functionality in API integration?
Retry functionality is the mechanism that automatically reattempts an API call if it fails due to temporary issues, improving the system's resilience and ensuring uninterrupted operations.
Why is retry functionality important in applications?
Retry functionality ensures that temporary issues with third-party APIs don’t disrupt critical processes, improving reliability, reducing downtime impact, and enhancing user experience by avoiding immediate errors.
What is the difference between simple retry and exponential backoff?
Simple retry uses a fixed delay between attempts, while exponential backoff gradually increases the delay with each retry, reducing server strain and improving efficiency in case of frequent or temporary disruptions.
What are the benefits of exponential backoff over simple retry?
Exponential backoff reduces server strain by spacing out retries, making it ideal for APIs prone to temporary disruptions. Simple retry, on the other hand, may overwhelm the server during downtime.
How does progressive delay work in retry functionality?
Progressive delay increases the wait time between retries, ensuring that repeated attempts are spaced out over time, preventing resource overuse and reducing the impact on the server.
What happens when the maximum retry attempts are reached?
When the maximum retry attempts are reached, the system throws an exception, indicating that the retries were unsuccessful and providing the last status code for debugging purposes.
How can I configure retry parameters in my application?
You can externalize retry parameters into a configuration file, allowing flexibility to adjust settings such as maximum retry attempts, initial delay, incremental delay, and whether to log failed attempts.
What is the role of logging in retry functionality?
Logging failed attempts helps track retries, enabling better debugging and monitoring. It also provides insights into why certain API calls are failing and can guide improvements in retry strategies.
What are common use cases for implementing retry functionality?
Common use cases include order synchronization with third-party APIs, inventory updates, and handling issues with payment gateways, ensuring smooth operations even during temporary failures.
What is dynamic delay in retry functionality?
Dynamic delay, such as exponential backoff or jitter, randomizes the delay between retries to reduce API server load and improve the chances of a successful retry during temporary disruptions.
How can I implement retry functionality in PHP?
In PHP, you can use libraries like cURL or Guzzle to make the API call, then implement retry logic with conditions to check for failures, apply delays, and retry the request up to a maximum number of attempts.