Fetch CMS Blocks Collection Using GraphQL in Magento 2
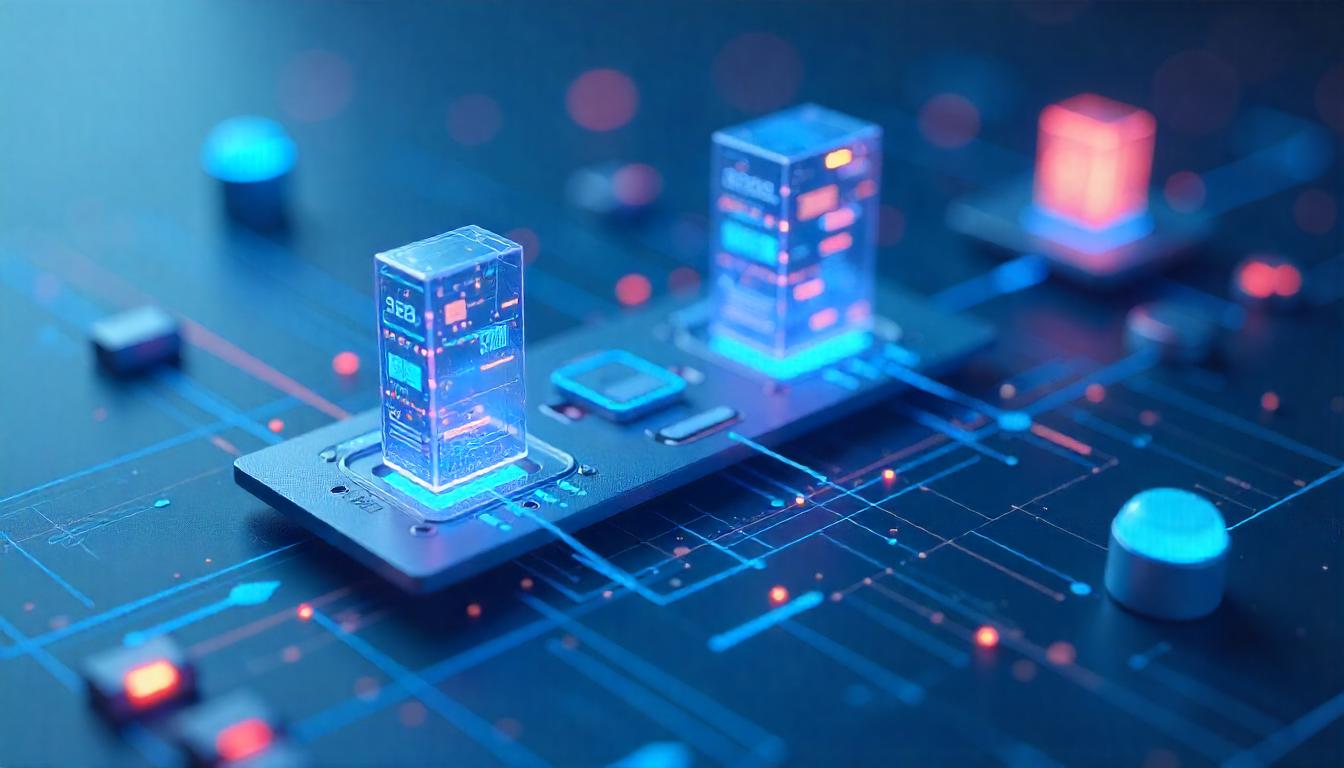
Fetch CMS Blocks Collection Using GraphQL in Magento 2
In Magento 2, fetching CMS static block collections with GraphQL can significantly improve the flexibility and performance of your storefront. By creating a custom module, you can tailor GraphQL queries to retrieve relevant CMS block data efficiently. Here’s how you can achieve it:
Why Fetch CMS Blocks with GraphQL?
- Enhanced Performance: GraphQL allows selective data fetching, reducing the payload and improving page load speed.
- Customization: Tailor queries to fetch only the data you need.
- Flexibility: Easily integrate CMS content into headless applications or custom frontend frameworks.
Table Of Content
Overview of CMS Block Query via GraphQL in Magento 2
GraphQL in Magento 2 provides an efficient and flexible way to query CMS blocks. It allows developers to fetch only the required data, enhancing performance and enabling integration with modern frontend frameworks. This guide dives into the process of querying CMS blocks using GraphQL, creating custom resolvers, and optimizing your module for specific use cases.
Why Use GraphQL for CMS Blocks?
GraphQL provides numerous benefits over traditional REST or SOAP APIs when working with CMS blocks:
- Selective Data Retrieval: Fetch only the data you need, reducing response size.
- Modern Integration: Seamlessly connect with headless storefronts and single-page applications.
- Improved Performance: Reduce API calls with GraphQL’s single-query approach.
- Scalability: Handle complex data relationships efficiently.
CMS Block Query: Features and Benefits
Feature | Benefit |
---|---|
Fetch All CMS Blocks | Retrieve complete CMS block collections for dynamic content management. |
Filter CMS Blocks | Query blocks based on identifiers, titles, or specific fields. |
Optimized Payload | Return only the fields you need (e.g., identifier, title, content). |
Integration Support | Compatible with React, Vue, or other modern frontend frameworks. |
Steps to Implement a Custom CMS Block Query in Magento 2
Creating a custom CMS block query with GraphQL in Magento 2 involves defining a custom module, setting up its configuration, and implementing the necessary resolvers. Follow these detailed steps to build your module.
Step 1: Step 1: Create the Module
Creating a custom module in Magento 2 requires setting up the foundational files and configurations. Below, we outline the necessary steps to define your module structure.
Files to Create for Module Definition:
registration.php
This file registers your module within Magento’s system and should be placed in the root of your module directory.Path:
app/code/Emmo/CmsBlocksGraphQl/registration.php
PHP Code for Module Registration
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Emmo_CmsBlocksGraphQl',
__DIR__
);
module.xml
This configuration file is required for Magento to recognize the module. It should be placed inside theetc
directory of your module. The file also defines module dependencies.Path:
app/code/Emmo/CmsBlocksGraphQl/etc/module.xml
XML Configuration for Module
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Emmo_CmsBlocksGraphQll">
<sequence>
<module name="Magento_Cms"/>
<module name="Magento_GraphQl"/>
</sequence>
</module>
</config>
- Explanation
Magento_Cms
: Ensures your module can interact with the cms data.Magento_GraphQl
: Integrates GraphQL functionality for the custom module.
Directory Structure
Here’s how your module directory should be structured at this point:
Module Directory Structure
app/code/Emmo/CmsBlocksGraphQl
├── registration.php
└── etc
└── module.xml
By defining these files, you’ve completed the first step of module creation. In the next steps, you will add more functionality, such as custom GraphQL queries, models, and other logic to extend your Magento store.
Step 2: Define the Schema for CMS Blocks Query
To enable GraphQL to retrieve CMS blocks, you need to define a schema file. This file outlines the structure of the query, the type of data it will return, and any additional metadata like descriptions.
Createschema.graphqls
File Path:app/code/Emmo/CmsBlocksGraphQl/etc/schema.graphqls
This file defines the query and data types used in your GraphQL implementation.
GraphQL Schema Definition
schema.graphqls
type Query {
cmsBlocks: CmsBlocks @resolver(class: "Emmo\\CmsBlocksGraphQl\\Model\\Resolver\\CmsBlock") @doc(description: "Retrieve all CMS static blocks.")
}
type CmsBlocks @doc(description: "Details of all CMS static blocks") {
allBlocks: [BlockRecord] @doc(description: "Array of all CMS static blocks.")
}
type BlockRecord {
identifier: String @doc(description: "Unique identifier of the CMS block.")
name: String @doc(description: "Name of the CMS block.")
content: String @doc(description: "Content of the CMS block.")
}
Key Elements of the Schema
1. Root Query (cmsBlocks)
- Purpose: Fetch CMS block data.
- Resolver: A class handles the backend logic for fetching the blocks.
- Description: Provides metadata for better documentation of GraphQL API.
2. Type:CmsBlocks
- Purpose: Groups the results as a single object.
- Fields:
allBlocks
: An array containing CMS block details.
Type:BlockRecord
- Purpose: Defines the structure of each CMS block in the result.
- Fields:
identifier:
Unique key for identifying the CMS block.name:
The display name of the CMS block.content:
The actual HTML or text content stored in the block.
Step 3: Implement the Resolver
The resolver is the backbone of the GraphQL functionality, as it defines how data from Magento’s back-end is fetched and transformed into the required format.
Purpose of the Resolver
The resolver bridges GraphQL queries with the Magento 2 CMS block repository. It handles the logic to retrieve, filter, and structure CMS block data for GraphQL responses.
File Details
File Name:CmsBlock.php
Pathapp/code/Emmo/CmsBlocksGraphQl/Model/Resolver/CmsBlock.php
Key Components
Dependencies and Their Roles
Dependency | Purpose |
---|---|
BlockRepositoryInterface | Accesses CMS blocks stored in the Magento database. |
SearchCriteriaBuilder | Builds search criteria for filtering the CMS blocks. |
Field, ResolveInfo | Provide GraphQL schema and field information for query resolution. |
GraphQlNoSuchEntityException | Manages exceptions when the requested CMS block does not exist. |
Code Explanation
Resolver Implementation
<?php
declare(strict_types=1);
namespace Emmo\CmsBlocksGraphQl\Model\Resolver;
use Magento\Framework\Api\SearchCriteriaBuilder;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\GraphQl\Config\Element\Field;
use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException;
use Magento\Framework\GraphQl\Query\ResolverInterface;
use Magento\Framework\GraphQl\Schema\Type\ResolveInfo;
use Magento\Cms\Api\BlockRepositoryInterface;
class CmsBlock implements ResolverInterface
{
private BlockRepositoryInterface $blockRepository;
private SearchCriteriaBuilder $searchCriteriaBuilder;
public function __construct(
BlockRepositoryInterface $blockRepository,
SearchCriteriaBuilder $searchCriteriaBuilder
) {
$this->blockRepository = $blockRepository;
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
}
public function resolve(
Field $field,
$context,
ResolveInfo $info,
array $value = null,
array $args = null
) {
return $this->getBlocksData();
}
private function getBlocksData(): array
{
try {
$searchCriteria = $this->searchCriteriaBuilder->addFilter('block_id', 1, 'gteq')->create();
$blocks = $this->blockRepository->getList($searchCriteria)->getItems();
$cmsBlocks = ['allBlocks' => []];
foreach ($blocks as $block) {
$cmsBlocks['allBlocks'][] = [
'identifier' => $block->getIdentifier(),
'name' => $block->getTitle(),
'content' => $block->getContent(),
];
}
return $cmsBlocks;
} catch (NoSuchEntityException $e) {
throw new GraphQlNoSuchEntityException(__($e->getMessage()), $e);
}
}
}
Breakdown of Key Methods
1. Constructor
The constructor injects dependencies:
Constructor Implementation
public function __construct(
BlockRepositoryInterface $blockRepository,
SearchCriteriaBuilder $searchCriteriaBuilder
) {
$this->blockRepository = $blockRepository;
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
}
2. resolve
Method
This method handles the query resolution and delegates the work to the getBlocksData()
method:
Resolver Method
public function resolve(
Field $field,
$context,
ResolveInfo $info,
array $value = null,
array $args = null
) {
return $this->getBlocksData();
}
3. getBlocksData
Method
This method fetches CMS blocks from the repository and formats the data:
Private Method: getBlocksData
private function getBlocksData(): array
{
try {
$searchCriteria = $this->searchCriteriaBuilder
->addFilter('block_id', 1, 'gteq')
->create();
$blocks = $this->blockRepository->getList($searchCriteria)->getItems();
$cmsBlocks = ['allBlocks' => []];
foreach ($blocks as $block) {
$cmsBlocks['allBlocks'][] = [
'identifier' => $block->getIdentifier(),
'name' => $block->getTitle(),
'content' => $block->getContent(),
];
}
return $cmsBlocks;
} catch (NoSuchEntityException $e) {
throw new GraphQlNoSuchEntityException(__($e->getMessage()), $e);
}
}
Error Handling
This resolver uses exception handling to manage errors gracefully:
- If a block is not found,
NoSuchEntityException
is thrown. - This is rethrown as
GraphQlNoSuchEntityException
for GraphQL compatibility.
Step 4: Run Magento Setup Commands
After implementing the module code, it’s essential to activate and configure it properly within the Magento framework. Running the setup commands ensures the module is registered, database changes (if any) are applied, and the system cache is refreshed for the changes to take effect.
Commands Overview
Command | Purpose |
---|---|
php bin/magento setup:upgrade |
Registers the module and applies any database schema or data changes. |
php bin/magento cache:flush |
Clears the Magento cache to ensure the system reflects the latest changes. |
Steps to Run the Commands
Open Terminal
Navigate to your Magento installation directory using the terminal or command prompt.
Run the Setup Upgrade Command
php bin/magento setup:upgrade
Purpose:This command scans the app/code
directory, updates the setup_module
table in the database, and applies any schema or data patches.
Flush the Cache
php bin/magento cache:flush
Purpose:Clears all cached data, ensuring the changes from the newly installed module are applied immediately. This avoids serving outdated content or configurations.
Troubleshooting Common Issues
Issue | Solution |
---|---|
Permission Denied error | Ensure you have the necessary permissions to run commands. Use sudo if required. |
Module not appearing in setup:upgrade log | Check the module.xml and registration.php files for correct configuration. |
Cache not clearing | Manually delete cache directories in var/cache and var/page_cache. |
Step 5: Test the GraphQL Query
Testing your GraphQL query is essential to ensure that the integration works as expected and returns the desired results. By using tools like ChromeiQL or Altair GraphQL Client, you can easily test your GraphQL queries and inspect the responses from the server.
GraphQL Query Example
To retrieve all CMS blocks, execute the following query:
GraphQL Query
{"
cmsBlocks {
allBlocks {
name
identifier
content
}
}
}
This query will fetch the name, identifier, and content for all available CMS blocks.
Expected Response:
If the query is successful, you should receive a response similar to the one below:
Expected Response
{
"data": {
"cmsBlocks": {
"allBlocks": [
{
"name": "Contact Us Info",
"identifier": "contact-us-info",
"content": "<div class="contact-info">We love hearing from you!</div>"
},
{
"name": "Footer Links Block",
"identifier": "footer-links-block",
"content": "<ul><li>About Us</li><li>Customer Service</li></ul>"
}
]
}
}
}
Additional Tips
- Custom Filters: Add filters in the
getBlocksData()
method for more refined results. - Pagination: Modify the query to include pagination for large collections.
- Error Handling: Enhance error-handling logic in the resolver for better debugging.
This implementation provides a robust way to fetch CMS static blocks in Magento 2 using GraphQL. Customize the module as needed to suit your project requirements!
Common Use Cases for CMS Block Queries
GraphQL queries are commonly used in various Magento applications, especially when you need to retrieve or manage CMS block data. Below are some common use cases that illustrate how CMS block queries can be utilized:
1. Dynamic Landing Pages
CMS blocks are crucial for displaying dynamic content on landing pages. By fetching CMS blocks via GraphQL, you can populate landing pages with content dynamically based on the query parameters or user input, making the pages highly customizable.
Example Use Case:
- A dynamic landing page where the CMS block content varies based on the user’s region, language, or promotions.
2. Headless CMS Integration
One of the most powerful use cases of GraphQL in Magento is the integration of CMS data into headless architectures. Using GraphQL, you can fetch CMS block data and display it in various frontend applications built with frameworks like React, Vue.js, or Angular.
Example Use Case:
- A headless Magento storefront built with React, where CMS blocks like "Contact Us" or "About Us" are fetched via GraphQL and rendered in the UI.
3. Mobile Optimization
GraphQL allows for optimized payload delivery, which is essential for mobile applications. By querying only the necessary CMS block data, you can reduce the size of the response and improve the loading speed for mobile users.
Example Use Case:
- A mobile app for a Magento store, fetching CMS block content like promotions or product details using a minimal GraphQL query optimized for mobile data usage.
4. Personalized Content
By leveraging GraphQL queries with customer attributes, you can deliver personalized content to users. For instance, you can query for CMS blocks based on customer data such as purchase history or user preferences.
Example Use Case:
- A personalized homepage for logged-in users, displaying different CMS blocks or promotional banners based on their browsing history or previous purchases.
Tips for Optimizing GraphQL Queries
When working with GraphQL in Magento, it’s essential to optimize queries to ensure that the system performs efficiently, especially when dealing with large datasets. Here are some key tips for improving your GraphQL queries:
1. Paginate Results
For queries that retrieve large collections, you can implement pagination to ensure that your system doesn't overload by fetching too many records at once. GraphQL allows you to use limit
and offset
parameters to control the number of items returned in a single query, making the query response faster and reducing the load on the server.
Example Pagination Query:
{
cmsBlocks(pageSize: 10, currentPage: 1) {
items {
name
identifier
content
}
}
}
2. Caching
Magento provides several caching mechanisms to enhance the performance of GraphQL queries. Caching allows you to reduce database load by storing query results for a specified period, ensuring that repeat requests are served faster.
- Full Page Cache (FPC): Magento uses FPC to cache entire pages, including GraphQL results, for faster rendering.
- Query Response Caching: You can also cache specific GraphQL queries based on the data returned and frequency of change.
3. Add Authorization
GraphQL allows you to implement authorization at the query level, ensuring that only authorized users can access certain data. This is especially useful when building storefronts where sensitive data, such as customer orders or account details, should only be accessible to the respective user.
- Use Magento’s built-in user roles and permissions to restrict query access.
4. Use Debugging Tools
GraphQL is a flexible and powerful query language, but debugging complex queries can be challenging. Tools like GraphQL Playground and Chrome DevTools are invaluable for testing and debugging GraphQL queries.
- GraphQL Playground: A GraphQL IDE that allows you to test queries, view schema documentation, and explore different GraphQL operations.
- Chrome DevTools: Use the network tab in Chrome to inspect GraphQL requests and responses, and test queries directly from the console.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
In this guide, we've explored the process of fetching CMS block collections in Magento 2 using GraphQL. By creating a custom module, defining the schema, and implementing the resolver, we can efficiently retrieve CMS static block data, providing enhanced flexibility and performance for Magento-based stores.
We also covered key aspects like adding custom filters, implementing pagination for large datasets, and handling errors for a smoother and more reliable experience. This setup not only improves query efficiency but also integrates seamlessly with Magento's GraphQL capabilities.
As Magento 2 continues to evolve, leveraging GraphQL for data retrieval empowers developers to build faster, more scalable eCommerce solutions, offering better control and optimization for complex CMS block management. Whether you're building a custom frontend, API integrations, or simply improving data handling, this approach offers a robust and future-proof solution.
With this module in place, you can easily extend its functionality to meet the specific needs of your projects, making it a vital tool for developers working with Magento 2. Happy coding!
FAQs
What is GraphQL in Magento 2?
GraphQL is a query language for APIs that enables clients to request exactly the data they need, making it more efficient than traditional REST APIs. Magento 2 supports GraphQL for enhanced data fetching and flexibility.
How does GraphQL improve data fetching in Magento 2?
GraphQL allows clients to request specific data in a single query, reducing the amount of data transferred and improving performance compared to traditional REST APIs where multiple requests may be needed.
How can I fetch CMS blocks using GraphQL in Magento 2?
To fetch CMS blocks in Magento 2, you need to create a custom GraphQL module, define a schema with a CMS block query, and implement a resolver to return the CMS block data.
What is the purpose of a custom resolver in GraphQL?
A custom resolver in GraphQL is responsible for handling the business logic of fetching data for a specific query. In Magento 2, it connects the schema to the data source (e.g., CMS block repository) and formats the response.
What is the use of the `@resolver` directive in the GraphQL schema?
The `@resolver` directive in the schema binds a specific query to a custom resolver class that contains the logic for fetching data. It ensures that the query will execute the appropriate PHP logic to return the desired data.
What dependencies are required for a CMS blocks GraphQL module in Magento 2?
The module requires dependencies on the `Magento_Cms` and `Magento_GraphQl` modules. These are essential for accessing CMS block data and utilizing Magento’s GraphQL functionality.
Can I filter CMS blocks using GraphQL?
Yes, you can filter CMS blocks by adding conditions in the custom resolver, such as filtering by block identifier or other attributes, to return a subset of CMS blocks based on specific criteria.
What is the structure of the GraphQL query to fetch CMS blocks?
The GraphQL query to fetch CMS blocks typically looks like this:
{ cmsBlocks { allBlocks { name identifier content } } }
This query retrieves all CMS blocks, returning their name, identifier, and content.
How can I paginate CMS block results in GraphQL?
To paginate CMS blocks, you can modify the resolver to include pagination arguments, such as `pageSize` and `currentPage`, and adjust the query to return paginated results based on those parameters.
What are the advantages of using GraphQL over REST in Magento 2?
GraphQL allows for more efficient data fetching, as clients can request only the specific data they need. This reduces the number of requests and the amount of data transferred compared to traditional REST APIs.
How do I test GraphQL queries in Magento 2?
You can test GraphQL queries using tools like ChromeiQL or Altair GraphQL Client. These tools allow you to send queries to your Magento store's GraphQL endpoint and view the responses in real-time.
What error handling mechanisms should be implemented in a GraphQL resolver?
Error handling in GraphQL resolvers can be done by throwing appropriate exceptions, such as `GraphQlNoSuchEntityException`, for cases where data is not found, or custom error messages for other scenarios, ensuring clear and helpful error reporting.
Can I use GraphQL to fetch other types of data besides CMS blocks?
Yes, GraphQL in Magento 2 allows you to fetch a wide variety of data types, such as products, categories, orders, and more, by creating custom queries and resolvers for each data type.