How to Pass Data from Controller to View Template Files in Magento 2?
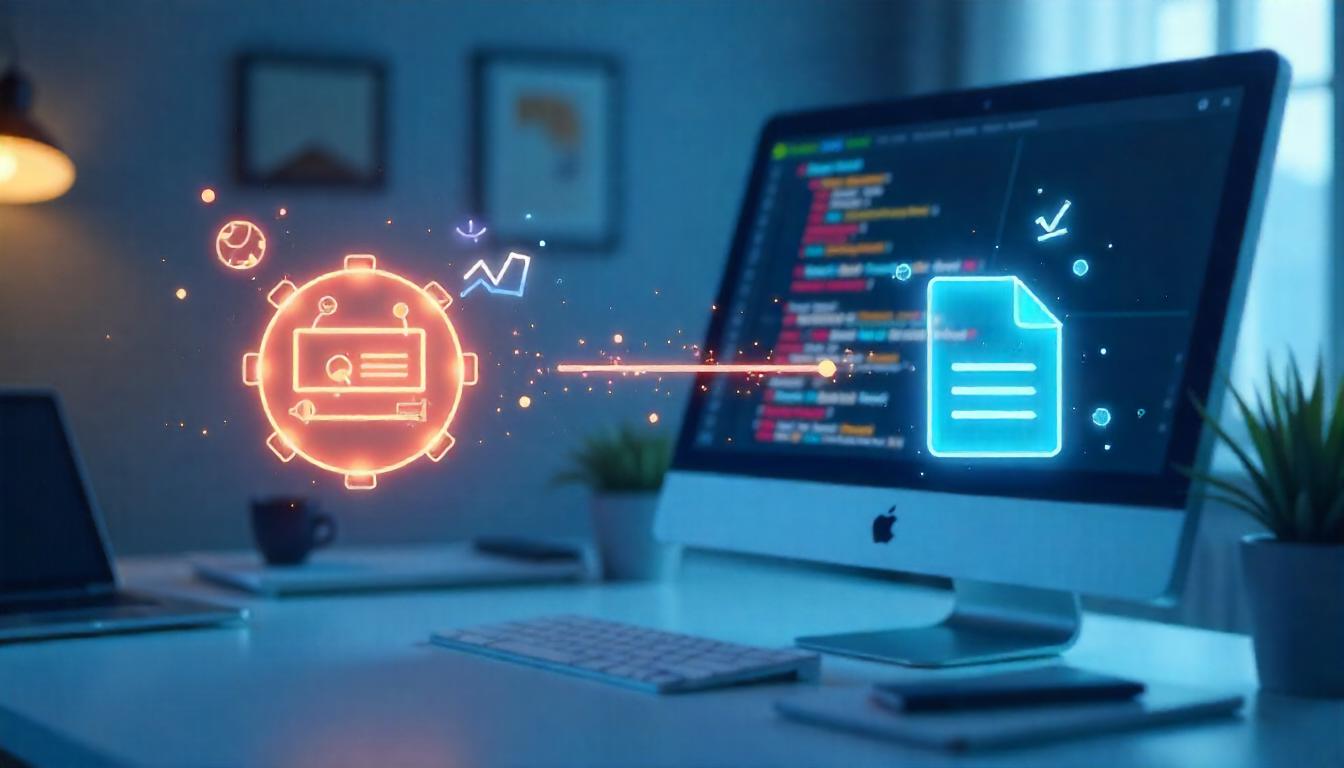
How to Pass Data from Controller to View Template Files in Magento 2?
In Magento 2, passing data from a Controller action to a View Template file is not a direct process due to its MVC (Model-View-Controller) architecture. Instead, this is achieved by leveraging the Block class as an intermediary. This approach promotes clean separation of logic and ensures maintainability.
This guide will walk you through the steps to pass data from the Controller to the View template using DataPersistorInterface
and Block classes.
Table Of Content
Why Can't You Pass Data Directly?
Magento enforces a modular architecture to maintain clean code structure and separation of concerns. Here's why direct data passing is not allowed:
MVC Component | Responsibility |
---|---|
Controller | Handles HTTP requests and directs workflow to the appropriate components. |
Block Class | Acts as an intermediary, preparing data for the View Template. |
View Template (PHTML) | Focuses solely on rendering output without business logic. |
Directly passing data from the Controller to the View Template violates this structure, leading to tightly coupled code, poor maintainability, and reduced scalability.
Benefits of Using Block Classes in Magento 2
In Magento 2, Block classes play a pivotal role in facilitating the interaction between the Controller and View Template (PHTML files). They serve as intermediaries, bridging the data flow from backend logic to the frontend display. Using Block classes offers a structured approach that aligns with Magento's MVC architecture.
Key Benefits of Using Block Classes
Block classes are more than just data carriers; they are essential for ensuring code quality, scalability, and maintainability in Magento 2 development.
Advantages and Descriptions
table highlighting the benefits of using Block classes:
Benefit | Description |
---|---|
Separation of Concerns | Keeps business logic out of templates, ensuring better maintainability and adherence to MVC principles. |
Reusability | Block methods can be reused across multiple templates, reducing redundancy and promoting cleaner code. |
Scalability | Simplifies the extension and modification of data-passing logic without disrupting template structure or existing workflows. |
Testability | Enhances test coverage by allowing logic to be tested separately from the template files. |
Debugging | Centralizes data preparation, making it easier to debug and identify issues in the data flow. |
Consistency | Provides a standardized way of passing data, reducing errors and improving developer collaboration. |
Practical Applications
Dynamic Content Rendering: Use Block classes to fetch dynamic data such as product details, customer information, or promotional banners.>
API Integration: Facilitate API response handling by processing and formatting data in the Block before rendering it in templates.
Conditional Logic: Implement conditional display logic in Block classes to keep the View layer clean and focused on presentation.
Passing Data to Templates
Let’s consider a scenario where you need to pass a list of featured products from the Controller to the template. By leveraging Block classes:
- The Controller fetches the data.
- The Block processes the data, adds additional logic if necessary, and prepares it for rendering.
- The View Template focuses solely on iterating over the prepared data for display.
Using Block classes in Magento 2 is essential for maintaining a clean, scalable, and efficient codebase. Their role in separating concerns, improving reusability, and enhancing debugging makes them indispensable for any Magento 2 developer. By understanding and implementing these benefits, you can streamline your development process while ensuring long-term maintainability of your projects.
A Comprehensive Guide to DataPersistorInterface
in Magento 2
The DataPersistorInterface in Magento 2 is an essential component for managing temporary data during the lifecycle of an HTTP request. It provides a robust mechanism to store and retrieve data seamlessly, making it invaluable for use cases like form submissions, error handling, and more. By enabling temporary storage of data between requests, it ensures a smoother and more intuitive user experience.
Why Use DataPersistorInterface?
Using DataPersistorInterface
comes with numerous advantages, especially in scenarios where maintaining data consistency between requests is crucial. Here are some key reasons to use it:
- Seamless Data Management: Helps in storing form data when validation fails, allowing users to correct and resubmit without re-entering everything.
- Session-Independent: Unlike sessions, the DataPersistorInterface is designed for short-lived storage that clears automatically after usage.
- Simplifies Development: Reduces the need for custom temporary storage logic, adhering to Magento’s standard practices.
- Improves User Experience: Ensures that data is not lost during a failed operation or page reload.
Methods Provided by DataPersistorInterface
Here is a detailed breakdown of the methods available in DataPersistorInterface
and their use:
Method | Description |
---|---|
set($key, $value) |
Stores data using a unique key, enabling retrieval during the request lifecycle. |
get($key) |
Retrieves data associated with the provided key. |
clear($key) |
Removes data associated with the key from the temporary storage. |
getAndClear($key) |
Fetches the data and clears it simultaneously to prevent reuse. |
How Does DataPersistorInterface Work?
The interface operates as a bridge between requests, leveraging Magento’s session and registry mechanisms under the hood. Here’s how it works:
- Data Storage: Data is temporarily stored in a unique key-value format.
- Request Transition: The stored data persists through subsequent requests until cleared.
- Automatic Clearing: Data is automatically removed when explicitly cleared or when the request cycle ends.
Common Use Cases of DataPersistorInterface
1. Form Submission Handling
- Scenario: A user submits a form, but validation fails. Instead of losing all entered data, it is temporarily stored using DataPersistorInterface, allowing the user to make corrections.
2. Error Handling
- Scenario: While processing a request, errors occur. The interface stores relevant data to prepopulate fields or display relevant messages during a retry.
3. Workflow Optimization
- Scenario: Use it to save temporary states or intermediary data during complex workflows involving multiple steps.
Using DataPersistorInterface
Controller Example
public function execute()
{
$dataPersistor = $this->dataPersistorFactory->create();
$dataPersistor->set('form_data', $this->getRequest()->getPostValue());
if ($this->validationFails()) {
// Redirect with stored data
return $this->_redirect('*/*/edit');
}
$dataPersistor->clear('form_data');
}
Template Example
$formData = $dataPersistor->getAndClear('form_data');
if ($formData) {
// Populate form fields with $formData
}
Benefits and Drawbacks of DataPersistorInterface
Benefits
- Ease of Use: Simple key-value interface for storing temporary data.
- Standardized: Reduces the need for custom solutions.
- Integrated: Works seamlessly with Magento’s existing request lifecycle.
Drawbacks
- Temporary Nature: Not suitable for long-term storage.
- Session Dependency: Relies on session handling, which might not align with stateless architectures.
Comparison of DataPersistorInterface with Sessions
Below is a comparison highlighting where DataPersistorInterface excels over traditional session handling:
Feature | DataPersistorInterface | Session |
---|---|---|
Use Case | Temporary storage between requests | Persistent storage across multiple sessions |
Data Lifetime | Short-lived | Long-lived |
Ease of Clearing | Automatic or explicit | Manual clearing required |
Storage Scope | Request-specific | Session-specific |
The DataPersistorInterface in Magento 2 is an indispensable tool for managing temporary data effectively. Its simplicity, combined with Magento’s robust architecture, makes it a go-to solution for scenarios like form handling, error management, and workflow optimizations. By understanding its methods and use cases, developers can harness its potential to build more user-friendly and maintainable applications.
Step-by-Step Implementation
In Magento 2, passing data from a Controller to a View Template is crucial for dynamic content rendering. This is especially important when you need to preserve user inputs across multiple pages or after form submissions. Magento 2 uses the MVC (Model-View-Controller) architecture, which separates data handling (Controller), presentation (View Template), and the intermediary (Block). This structure enhances code maintainability and scalability.
This guide will walk you through the steps to pass data from a Controller action to a View Template file in Magento 2. We will make use of DataPersistorInterface and Block classes for data storage and retrieval.
Step 1: Set Data in the Controller Action
The first step is to store data in the Controller action using the DataPersistorInterface. In this example, the Controller captures form data from the HTTP request, stores it, and makes it available for retrieval later.
<?php
declare(strict_types=1);
namespace Emmo\Blog\Controller\Index;
use Magento\Framework\App\Request\DataPersistorInterface;
class PassDataToTemplate
{
public function __construct(
private DataPersistorInterface $dataPersistor
) {
}
public function execute()
{
// Store form data in DataPersistor
$this->dataPersistor->set('form_data', $this->getRequest()->getParams());
}
}
set()
method: This method stores the data (form_data
) from the request parameters into theDataPersistorInterface
. The keyform_data
is used to uniquely identify this data for later retrieval.- Request Parameters: In this case, we use
$this->getRequest()->getParams()
to retrieve all request parameters, which typically include form inputs.
Step 2: Retrieve Data in the Block Class
Once the data is stored in the Controller, the next step is to retrieve that data in the Block class. Block classes serve as the intermediary, fetching data from various sources and passing it to the template for rendering.
<?php
declare(strict_types=1);
namespace Emmo\Blog\Block;
use Magento\Framework\View\Element\Template;
use Magento\Framework\App\Request\DataPersistorInterface;
class FormBlock extends Template
{
public function __construct(
Template\Context $context,
private DataPersistorInterface $dataPersistor,
array $data = []
) {
parent::__construct($context, $data);
}
public function getFormData(): array
{
// Retrieve form data using the key
return (array) $this->dataPersistor->get('form_data');
}
}
getFormData()
method: This method fetches the storedform_data
using the key provided during storage (form_data
). The data is returned as an array.- Injecting
DataPersistorInterface
: TheDataPersistorInterface
is injected into the Block class, enabling it to retrieve the stored data when needed.
Step 3: Display Data in the Template File
Finally, after retrieving the data in the Block class, it is time to display it in the View Template. The View Template file is responsible for presenting the data to the user.
<?php
$formData = $block->getFormData();
?>
<div>
<h3>Submitted Form Data:</h3>
<pre><?php print_r($formData); ?></pre>
</div>
- Calling the
getFormData()
method: The template calls thegetFormData()
method from the Block class to retrieve the stored form data. - Displaying Data: The
print_r()
function is used to display the form data in a human-readable format. The<pre>
tag ensures the output is formatted in a way that’s easy to read.
Benefits of Using DataPersistorInterface
1. Data Persistence Across Requests
- DataPersistorInterface allows you to persist form data between HTTP requests. This ensures that data remains available even after page reloads, redirects, or form validation errors. This is essential in multi-step forms and for workflows requiring user inputs to be retained across pages.
2. Separation of Concerns
- The use of a Block class for data passing promotes a clean separation of business logic (handled by the Controller) from presentation logic (handled by the View Template). This structure enhances the maintainability and scalability of the code.
3. Consistency and Reusability
- By centralizing data management, DataPersistorInterface ensures consistent data handling across your Magento 2 project. Block methods that fetch data can be reused in multiple templates, making your code more modular and DRY (Don't Repeat Yourself).
4. Better Debugging and Error Handling
- The use of DataPersistorInterface facilitates easy debugging and logging. When combined with proper logging, you can track when and where data is persisted, helping diagnose any issues that may arise during the process.
5. No Need for Custom Session Handling
- Using
DataPersistorInterface
allows you to persist data across requests without having to manually manage sessions. This provides a cleaner, simpler alternative to handling session variables directly in Magento 2.
Troubleshooting Common Issues
Data Not Persisting:
- If you notice that data is not persisting as expected, ensure that the
set()
method is being called correctly and the correct key (form_data
) is being used in both the Controller and the Block. - Check that your
DataPersistorInterface
is properly injected into the Block class.
Data Retrieval Issues:
- If the data is not being retrieved correctly in the Block, confirm that the correct key (
form_data
) is passed to theget()
method ofDataPersistorInterface
. - Ensure that you are calling
getFormData()
from the correct Block instance and that no data-clearing actions (e.g.,$dataPersistor->clear()
) are happening prematurely.
Data Format Issues:
- If the data is not being displayed correctly in the template, ensure that the format used in
getFormData()
(such as casting to an array) is appropriate for your use case.
When to Use DataPersistorInterface
Common Scenarios:
Common Scenarios:
- Form Handling: If you're working with forms (e.g., user registration, checkout), using
DataPersistorInterface
ensures that users don't lose their data due to form submission errors or page reloads. - Error Handling: When form validation fails,
DataPersistorInterface
allows you to retain the user input and present the form with the correct values for easy correction. - Multi-step Processes: In workflows that span multiple pages or steps, such as multi-page checkout or survey forms, DataPersistor helps retain the state of the form data across different stages.
Practical Example: Magento_Contact Module
The Magento_Contact module offers a built-in feature to manage contact form submissions. When a customer submits a contact form, Magento follows the MVC (Model-View-Controller) architecture to handle the data flow. In this practical example, we’ll illustrate how data is passed from the controller to the view template using the principles of DataPersistorInterface and Block classes.
Flow Summary: Controller to Template
Here’s how the data flows from the controller to the view template in the Magento_Contact module:
Step | Component | Description |
---|---|---|
1 | Store Data | Controller Action - The controller action stores the submitted form data using DataPersistorInterface. The form data is saved with a unique key. |
2 | Fetch Data | Block Class - The Block class retrieves the stored data using the unique key and prepares it for the view template. |
3 | Render Data | View Template (.phtml) - The view template calls the block method to fetch the data and displays it to the customer in the frontend. |
This process ensures that the contact form data is passed and rendered correctly in the Magento framework, adhering to the MVC architecture.
Step 1: Storing Data in the Controller
In the Magento_Contact module, the controller handles the form submission. It stores the form data using the DataPersistorInterface. This allows the data to persist across page loads.
<?php
declare(strict_types=1);
namespace Magento\Contact\Controller\Index;
use Magento\Framework\App\Request\DataPersistorInterface;
use Magento\Framework\Controller\ResultFactory;
class Post extends \Magento\Contact\Controller\Index\AbstractIndex
{
public function __construct(
\Magento\Framework\App\Action\Context $context,
private DataPersistorInterface $dataPersistor
) {
parent::__construct($context);
}
public function execute()
{
// Retrieve the form data from the request
$formData = $this->getRequest()->getPostValue();
// Store the form data in DataPersistor
$this->dataPersistor->set('contact_form_data', $formData);
// Handle form submission logic here
return $this->resultFactory->create(ResultFactory::TYPE_REDIRECT)->setUrl('/thank-you');
}
}
Step 2: Fetching Data in the Block Class
Once the form data is stored in DataPersistorInterface, the next step is to retrieve this data in the Block class. The Block class serves as an intermediary between the controller and the view template.
<?php
declare(strict_types=1);
namespace Magento\Contact\Block;
use Magento\Framework\View\Element\Template;
use Magento\Framework\App\Request\DataPersistorInterface;
class ContactFormBlock extends Template
{
private DataPersistorInterface $dataPersistor;
public function __construct(
Template\Context $context,
DataPersistorInterface $dataPersistor,
array $data = []
) {
parent::__construct($context, $data);
$this->dataPersistor = $dataPersistor;
}
public function getContactFormData(): array
{
// Retrieve form data from DataPersistor
return (array) $this->dataPersistor->get('contact_form_data');
}
}
Step 3: Rendering Data in the View Template
In the View Template (.phtml), the data is displayed by calling the Block class's method to fetch the stored data. This ensures that the form data is available for display in the frontend.
<?php
$formData = $block->getContactFormData();
?>
<div>
<h3>Submitted Contact Form Data:</h3>
<pre><?php print_r($formData); ?></pre>
</div>
Benefits of Using DataPersistorInterface in Magento
The DataPersistorInterface in Magento offers several advantages for data management, especially for use cases like form submissions. Here are some key benefits:
Benefit | Description |
---|---|
Data Retention | Data is stored temporarily across multiple page requests, ensuring persistence without re-submission. |
Separation of Concerns | By using the DataPersistor, business logic remains separate from view logic, maintaining the MVC structure. |
Simplifies Form Handling | It streamlines handling multi-step or multi-page forms, where data needs to be carried over between form submissions. |
Enhanced User Experience | Reduces the need for users to re-enter data in case of errors or validation failures. |
By following the steps outlined in this example, Magento developers can easily pass data between the controller, block, and view template, while adhering to Magento's MVC architecture. Using DataPersistorInterface ensures that data remains persistent across page loads and improves the overall maintainability of the system.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Passing data from the Controller to View Template files in Magento 2 is a fundamental part of developing dynamic and interactive eCommerce websites. By adhering to Magento’s MVC architecture and leveraging tools like DataPersistorInterface and Block classes, you can maintain a clean and scalable codebase.
This approach ensures a clear separation of concerns, making your application easier to understand, maintain, and extend. Whether you are handling form submissions, user inputs, or other types of dynamic data, following this method guarantees robust and reliable data handling.
By implementing these best practices, you align with Magento 2's framework philosophy, enhancing both developer productivity and the overall quality of your code. Always remember to test your workflows thoroughly and handle edge cases gracefully to provide a seamless user experience.
FAQs
What is DataPersistorInterface in Magento 2?
DataPersistorInterface is a Magento framework interface that allows temporary storage and retrieval of data using key-value pairs, making it easier to pass data between controllers and blocks.
How can I pass data from a Controller to a template file in Magento 2?
Data from a Controller can be passed to a template file using a Block class that retrieves the data via DataPersistorInterface or directly from methods in the Block class.
What is the purpose of the Block class in Magento 2?
The Block class in Magento 2 acts as a bridge between the Controller and the View template. It holds business logic and retrieves or manipulates data for the template files.
How does the MVC architecture work in Magento 2?
The MVC (Model-View-Controller) architecture in Magento 2 separates the application logic, data handling, and presentation. Controllers handle requests, Models manage data, and Views present data through templates and Block classes.
What are the key methods of DataPersistorInterface?
The key methods of DataPersistorInterface are set()
for storing data, get()
for retrieving data, and clear()
for removing stored data by key.
Why can't data be passed directly from the Controller to the template?
Magento 2 uses the Block class as an intermediary to ensure adherence to its MVC architecture, which promotes separation of concerns and maintainability of code.
What is the role of the template file in Magento 2?
The template file in Magento 2 is responsible for rendering the HTML content on the frontend, using data provided by the associated Block class.
How can I retrieve data in a template file from a Block class?
To retrieve data in a template file, call the relevant method from the associated Block class, such as $block->getFormData()
in the PHP template.
Can I reuse a Block class for multiple templates?
Yes, a Block class can be reused across multiple templates, provided it contains the required logic or data retrieval methods for those templates.
What are the advantages of using DataPersistorInterface?
DataPersistorInterface provides a clean and standardized way to temporarily store and access data, improving code organization and reducing dependencies between components.
What is an example of using DataPersistorInterface in Magento 2?
An example would be storing form data with set()
in a Controller and retrieving it with get()
in a Block class to display the data in a template.
How does Magento 2 handle temporary data storage?
Magento 2 handles temporary data storage using tools like DataPersistorInterface and session management to maintain and retrieve data across requests.